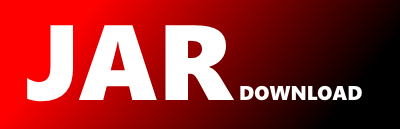
org.tensorflow.metadata.v0.AnomalyInfo Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow_metadata/proto/v0/anomalies.proto
// Protobuf Java Version: 3.25.4
package org.tensorflow.metadata.v0;
/**
*
* Message to represent information about an individual anomaly.
*
*
* Protobuf type {@code tensorflow.metadata.v0.AnomalyInfo}
*/
public final class AnomalyInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.AnomalyInfo)
AnomalyInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use AnomalyInfo.newBuilder() to construct.
private AnomalyInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AnomalyInfo() {
severity_ = 0;
description_ = "";
shortDescription_ = "";
diffRegions_ = java.util.Collections.emptyList();
reason_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AnomalyInfo();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.AnomalyInfo.class, org.tensorflow.metadata.v0.AnomalyInfo.Builder.class);
}
/**
* Protobuf enum {@code tensorflow.metadata.v0.AnomalyInfo.Severity}
*/
public enum Severity
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN = 0;
*/
UNKNOWN(0),
/**
* WARNING = 1;
*/
WARNING(1),
/**
* ERROR = 2;
*/
ERROR(2),
;
/**
* UNKNOWN = 0;
*/
public static final int UNKNOWN_VALUE = 0;
/**
* WARNING = 1;
*/
public static final int WARNING_VALUE = 1;
/**
* ERROR = 2;
*/
public static final int ERROR_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Severity valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Severity forNumber(int value) {
switch (value) {
case 0: return UNKNOWN;
case 1: return WARNING;
case 2: return ERROR;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Severity> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Severity findValueByNumber(int number) {
return Severity.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tensorflow.metadata.v0.AnomalyInfo.getDescriptor().getEnumTypes().get(0);
}
private static final Severity[] VALUES = values();
public static Severity valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Severity(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.metadata.v0.AnomalyInfo.Severity)
}
/**
*
* Next ID: 89
* LINT.IfChange
*
*
* Protobuf enum {@code tensorflow.metadata.v0.AnomalyInfo.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_TYPE = 0;
*/
UNKNOWN_TYPE(0),
/**
*
* Multiple reasons for anomaly.
*
*
* MULTIPLE_REASONS = 82;
*/
MULTIPLE_REASONS(82),
/**
*
* Integer larger than 1
*
*
* BOOL_TYPE_BIG_INT = 1;
*/
BOOL_TYPE_BIG_INT(1),
/**
*
* BYTES type when expected INT type
*
*
* BOOL_TYPE_BYTES_NOT_INT = 2;
*/
BOOL_TYPE_BYTES_NOT_INT(2),
/**
*
* BYTES type when expected STRING type
*
*
* BOOL_TYPE_BYTES_NOT_STRING = 3;
*/
BOOL_TYPE_BYTES_NOT_STRING(3),
/**
*
* FLOAT type when expected INT type
*
*
* BOOL_TYPE_FLOAT_NOT_INT = 4;
*/
BOOL_TYPE_FLOAT_NOT_INT(4),
/**
*
* FLOAT type when expected STRING type
*
*
* BOOL_TYPE_FLOAT_NOT_STRING = 5;
*/
BOOL_TYPE_FLOAT_NOT_STRING(5),
/**
*
* INT type when expected STRING type
*
*
* BOOL_TYPE_INT_NOT_STRING = 6;
*/
BOOL_TYPE_INT_NOT_STRING(6),
/**
*
* Integer smaller than 0
*
*
* BOOL_TYPE_SMALL_INT = 7;
*/
BOOL_TYPE_SMALL_INT(7),
/**
*
* STRING type when expected INT type
*
*
* BOOL_TYPE_STRING_NOT_INT = 8;
*/
BOOL_TYPE_STRING_NOT_INT(8),
/**
*
* Expected a string, but not the string seen
*
*
* BOOL_TYPE_UNEXPECTED_STRING = 9;
*/
BOOL_TYPE_UNEXPECTED_STRING(9),
/**
*
* Boolean had float values other than 0 and 1.
*
*
* BOOL_TYPE_UNEXPECTED_FLOAT = 52;
*/
BOOL_TYPE_UNEXPECTED_FLOAT(52),
/**
*
* BoolDomain has invalid configuration.
*
*
* BOOL_TYPE_INVALID_CONFIG = 88;
*/
BOOL_TYPE_INVALID_CONFIG(88),
/**
*
* BYTES type when expected STRING type
*
*
* ENUM_TYPE_BYTES_NOT_STRING = 10;
*/
ENUM_TYPE_BYTES_NOT_STRING(10),
/**
*
* FLOAT type when expected STRING type
*
*
* ENUM_TYPE_FLOAT_NOT_STRING = 11;
*/
ENUM_TYPE_FLOAT_NOT_STRING(11),
/**
*
* INT type when expected STRING type
*
*
* ENUM_TYPE_INT_NOT_STRING = 12;
*/
ENUM_TYPE_INT_NOT_STRING(12),
/**
*
* Invalid UTF8 string observed
*
*
* ENUM_TYPE_INVALID_UTF8 = 13;
*/
ENUM_TYPE_INVALID_UTF8(13),
/**
*
* Unexpected string values
*
*
* ENUM_TYPE_UNEXPECTED_STRING_VALUES = 14;
*/
ENUM_TYPE_UNEXPECTED_STRING_VALUES(14),
/**
*
* The number of values in a given example is too large
*
*
* FEATURE_TYPE_HIGH_NUMBER_VALUES = 15;
*/
FEATURE_TYPE_HIGH_NUMBER_VALUES(15),
/**
*
* The fraction of examples containing a feature is too small
*
*
* FEATURE_TYPE_LOW_FRACTION_PRESENT = 16;
*/
FEATURE_TYPE_LOW_FRACTION_PRESENT(16),
/**
*
* The number of examples containing a feature is too small
*
*
* FEATURE_TYPE_LOW_NUMBER_PRESENT = 17;
*/
FEATURE_TYPE_LOW_NUMBER_PRESENT(17),
/**
*
* The number of values in a given example is too small
*
*
* FEATURE_TYPE_LOW_NUMBER_VALUES = 18;
*/
FEATURE_TYPE_LOW_NUMBER_VALUES(18),
/**
*
* No examples contain the value
*
*
* FEATURE_TYPE_NOT_PRESENT = 19;
*/
FEATURE_TYPE_NOT_PRESENT(19),
/**
*
* The feature is present as an empty list
*
*
* FEATURE_TYPE_NO_VALUES = 20;
*/
FEATURE_TYPE_NO_VALUES(20),
/**
*
* The feature is repeated in an example, but was expected to be a singleton
*
*
* FEATURE_TYPE_UNEXPECTED_REPEATED = 21;
*/
FEATURE_TYPE_UNEXPECTED_REPEATED(21),
/**
*
* The feature had too many unique values (string and categorical features
* only).
*
*
* FEATURE_TYPE_HIGH_UNIQUE = 59;
*/
FEATURE_TYPE_HIGH_UNIQUE(59),
/**
*
* The feature had too few unique values (string and categorical features
* only).
*
*
* FEATURE_TYPE_LOW_UNIQUE = 60;
*/
FEATURE_TYPE_LOW_UNIQUE(60),
/**
*
* The feature has a constraint on the number of unique values but is not of
* a type that has the number of unique values counted (i.e., is not string
* or categorical).
*
*
* FEATURE_TYPE_NO_UNIQUE = 61;
*/
FEATURE_TYPE_NO_UNIQUE(61),
/**
*
* There is a float value that is too high
*
*
* FLOAT_TYPE_BIG_FLOAT = 22;
*/
FLOAT_TYPE_BIG_FLOAT(22),
/**
*
* The type is not FLOAT
*
*
* FLOAT_TYPE_NOT_FLOAT = 23;
*/
FLOAT_TYPE_NOT_FLOAT(23),
/**
*
* There is a float value that is too low
*
*
* FLOAT_TYPE_SMALL_FLOAT = 24;
*/
FLOAT_TYPE_SMALL_FLOAT(24),
/**
*
* The feature is supposed to be floats encoded as strings, but there is
* a string that is not a float
*
*
* FLOAT_TYPE_STRING_NOT_FLOAT = 25;
*/
FLOAT_TYPE_STRING_NOT_FLOAT(25),
/**
*
* The feature is supposed to be floats encoded as strings, but it was
* some other type (INT, BYTES, FLOAT)
*
*
* FLOAT_TYPE_NON_STRING = 26;
*/
FLOAT_TYPE_NON_STRING(26),
/**
*
* The type is completely unknown
*
*
* FLOAT_TYPE_UNKNOWN_TYPE_NUMBER = 27;
*/
FLOAT_TYPE_UNKNOWN_TYPE_NUMBER(27),
/**
*
* Float feature includes NaN values.
*
*
* FLOAT_TYPE_HAS_NAN = 53;
*/
FLOAT_TYPE_HAS_NAN(53),
/**
*
* Float feature includes Inf or -Inf values.
*
*
* FLOAT_TYPE_HAS_INF = 62;
*/
FLOAT_TYPE_HAS_INF(62),
/**
*
* There is an unexpectedly large integer
*
*
* INT_TYPE_BIG_INT = 28;
*/
INT_TYPE_BIG_INT(28),
/**
*
* The type was supposed to be INT, but it was not.
*
*
* INT_TYPE_INT_EXPECTED = 29;
*/
INT_TYPE_INT_EXPECTED(29),
/**
*
* The feature is supposed to be ints encoded as strings, but some string
* was not an int.
*
*
* INT_TYPE_NOT_INT_STRING = 30;
*/
INT_TYPE_NOT_INT_STRING(30),
/**
*
* The type was supposed to be STRING, but it was not.
*
*
* INT_TYPE_NOT_STRING = 31;
*/
INT_TYPE_NOT_STRING(31),
/**
*
* There is an unexpectedly small integer
*
*
* INT_TYPE_SMALL_INT = 32;
*/
INT_TYPE_SMALL_INT(32),
/**
*
* The feature is supposed to be ints encoded as strings, but it was
* some other type (INT, BYTES, FLOAT)
*
*
* INT_TYPE_STRING_EXPECTED = 33;
*/
INT_TYPE_STRING_EXPECTED(33),
/**
*
* Unknown type in stats proto
*
*
* INT_TYPE_UNKNOWN_TYPE_NUMBER = 34;
*/
INT_TYPE_UNKNOWN_TYPE_NUMBER(34),
/**
*
* The fraction of examples containing TensorFlow supported images is lower
* than the threshold set in the Schema.
*
*
* LOW_SUPPORTED_IMAGE_FRACTION = 64;
*/
LOW_SUPPORTED_IMAGE_FRACTION(64),
/**
*
* There are no stats for a column at all
*
*
* SCHEMA_MISSING_COLUMN = 35;
*/
SCHEMA_MISSING_COLUMN(35),
/**
*
* There is a new column that is not in the schema.
*
*
* SCHEMA_NEW_COLUMN = 36;
*/
SCHEMA_NEW_COLUMN(36),
/**
*
* Training serving skew issue
*
*
* SCHEMA_TRAINING_SERVING_SKEW = 37;
*/
SCHEMA_TRAINING_SERVING_SKEW(37),
/**
*
* Expected STRING type, but it was FLOAT.
*
*
* STRING_TYPE_NOW_FLOAT = 38;
*/
STRING_TYPE_NOW_FLOAT(38),
/**
*
* Expected STRING type, but it was INT.
*
*
* STRING_TYPE_NOW_INT = 39;
*/
STRING_TYPE_NOW_INT(39),
/**
*
* Control data is missing (either scoring data or previous day).
*
*
* COMPARATOR_CONTROL_DATA_MISSING = 40;
*/
COMPARATOR_CONTROL_DATA_MISSING(40),
/**
*
* Treatment data is missing (either treatment data or current day).
*
*
* COMPARATOR_TREATMENT_DATA_MISSING = 41;
*/
COMPARATOR_TREATMENT_DATA_MISSING(41),
/**
*
* L infinity between treatment and control is high.
*
*
* COMPARATOR_L_INFTY_HIGH = 42;
*/
COMPARATOR_L_INFTY_HIGH(42),
/**
*
* Approximate Jensen-Shannon divergence between treatment and control is
* high.
*
*
* COMPARATOR_JENSEN_SHANNON_DIVERGENCE_HIGH = 63;
*/
COMPARATOR_JENSEN_SHANNON_DIVERGENCE_HIGH(63),
/**
*
* The normalized absolute difference between treatment and control is high.
*
*
* COMPARATOR_NORMALIZED_ABSOLUTE_DIFFERENCE_HIGH = 87;
*/
COMPARATOR_NORMALIZED_ABSOLUTE_DIFFERENCE_HIGH(87),
/**
*
* No examples in the span.
*
*
* NO_DATA_IN_SPAN = 43;
*/
NO_DATA_IN_SPAN(43),
/**
*
* The value feature of a sparse feature is missing and at least one
* feature defining the sparse feature is present.
*
*
* SPARSE_FEATURE_MISSING_VALUE = 44;
*/
SPARSE_FEATURE_MISSING_VALUE(44),
/**
*
* An index feature of a sparse feature is missing and at least one
* feature defining the sparse feature is present.
*
*
* SPARSE_FEATURE_MISSING_INDEX = 45;
*/
SPARSE_FEATURE_MISSING_INDEX(45),
/**
*
* The length of the features representing a sparse feature does not match.
*
*
* SPARSE_FEATURE_LENGTH_MISMATCH = 46;
*/
SPARSE_FEATURE_LENGTH_MISMATCH(46),
/**
*
* Name collision between a sparse feature and raw feature.
*
*
* SPARSE_FEATURE_NAME_COLLISION = 47;
*/
SPARSE_FEATURE_NAME_COLLISION(47),
/**
*
* Invalid custom semantic domain.
*
*
* SEMANTIC_DOMAIN_UPDATE = 48;
*/
SEMANTIC_DOMAIN_UPDATE(48),
/**
*
* There are not enough examples in the current data as compared to a
* control dataset.
*
*
* COMPARATOR_LOW_NUM_EXAMPLES = 49;
*/
COMPARATOR_LOW_NUM_EXAMPLES(49),
/**
*
* There are too many examples in the current data as compared to a control
* dataset.
*
*
* COMPARATOR_HIGH_NUM_EXAMPLES = 50;
*/
COMPARATOR_HIGH_NUM_EXAMPLES(50),
/**
*
* There are not enough examples in the dataset.
*
*
* DATASET_LOW_NUM_EXAMPLES = 51;
*/
DATASET_LOW_NUM_EXAMPLES(51),
/**
*
* There are too many examples in the dataset.
*
*
* DATASET_HIGH_NUM_EXAMPLES = 58;
*/
DATASET_HIGH_NUM_EXAMPLES(58),
/**
*
* Name collision between a weighted feature and a raw feature.
*
*
* WEIGHTED_FEATURE_NAME_COLLISION = 54;
*/
WEIGHTED_FEATURE_NAME_COLLISION(54),
/**
*
* The value feature of a weighted feature is missing on examples where the
* weight feature is present.
*
*
* WEIGHTED_FEATURE_MISSING_VALUE = 55;
*/
WEIGHTED_FEATURE_MISSING_VALUE(55),
/**
*
* The weight feature of a weighted feature is missing on examples where the
* value feature is present.
*
*
* WEIGHTED_FEATURE_MISSING_WEIGHT = 56;
*/
WEIGHTED_FEATURE_MISSING_WEIGHT(56),
/**
*
* The length of the features representing a weighted feature does not
* match.
*
*
* WEIGHTED_FEATURE_LENGTH_MISMATCH = 57;
*/
WEIGHTED_FEATURE_LENGTH_MISMATCH(57),
/**
*
* The nesting level of the feature values does not match.
*
*
* VALUE_NESTEDNESS_MISMATCH = 65;
*/
VALUE_NESTEDNESS_MISMATCH(65),
/**
*
* The domain specified is not compatible with the physical type.
*
*
* DOMAIN_INVALID_FOR_TYPE = 66;
*/
DOMAIN_INVALID_FOR_TYPE(66),
/**
*
* Feature on schema has no name.
*
*
* FEATURE_MISSING_NAME = 67;
*/
FEATURE_MISSING_NAME(67),
/**
*
* Feature on schema has no type.
*
*
* FEATURE_MISSING_TYPE = 68;
*/
FEATURE_MISSING_TYPE(68),
/**
*
* Triggered for invalid schema specifications, e.g. min_fraction < 0.
*
*
* INVALID_SCHEMA_SPECIFICATION = 69;
*/
INVALID_SCHEMA_SPECIFICATION(69),
/**
*
* Triggered for invalid domain specifications in schema.
*
*
* INVALID_DOMAIN_SPECIFICATION = 81;
*/
INVALID_DOMAIN_SPECIFICATION(81),
/**
*
* The type of the data is inconsistent with the specified type.
*
*
* UNEXPECTED_DATA_TYPE = 70;
*/
UNEXPECTED_DATA_TYPE(70),
/**
*
* A value did not show up the min number of times within a sequence.
*
*
* SEQUENCE_VALUE_TOO_FEW_OCCURRENCES = 71;
*/
SEQUENCE_VALUE_TOO_FEW_OCCURRENCES(71),
/**
*
* A value showed up more the max number of times within a sequence.
*
*
* SEQUENCE_VALUE_TOO_MANY_OCCURRENCES = 72;
*/
SEQUENCE_VALUE_TOO_MANY_OCCURRENCES(72),
/**
*
* A value did not show up in at least the min fraction of sequences.
*
*
* SEQUENCE_VALUE_TOO_SMALL_FRACTION = 73;
*/
SEQUENCE_VALUE_TOO_SMALL_FRACTION(73),
/**
*
* A value showed up in greater than the max fraction of sequences.
*
*
* SEQUENCE_VALUE_TOO_LARGE_FRACTION = 74;
*/
SEQUENCE_VALUE_TOO_LARGE_FRACTION(74),
/**
*
* Too small a fraction of feature values matched vocab entries.
*
*
* FEATURE_COVERAGE_TOO_LOW = 75;
*/
FEATURE_COVERAGE_TOO_LOW(75),
/**
*
* The average token length was too short.
*
*
* FEATURE_COVERAGE_TOO_SHORT_AVG_TOKEN_LENGTH = 76;
*/
FEATURE_COVERAGE_TOO_SHORT_AVG_TOKEN_LENGTH(76),
/**
*
* A sequence violated the location constraint.
*
*
* NLP_WRONG_LOCATION = 77;
*/
NLP_WRONG_LOCATION(77),
/**
*
* A feature was specified as an embedding but was not a fixed dimension.
*
*
* EMBEDDING_SHAPE_INVALID = 78;
*/
EMBEDDING_SHAPE_INVALID(78),
/**
*
* A feature contains an image that has more bytes than the max byte size.
*
*
* MAX_IMAGE_BYTE_SIZE_EXCEEDED = 79;
*/
MAX_IMAGE_BYTE_SIZE_EXCEEDED(79),
/**
*
* A feature is supposed to be of a fixed shape but its valency stats
* do not agree.
*
*
* INVALID_FEATURE_SHAPE = 80;
*/
INVALID_FEATURE_SHAPE(80),
/**
*
* Constraints are specified within the but cannot be verified because the
* corresponding stats are not available.
*
*
* STATS_NOT_AVAILABLE = 83;
*/
STATS_NOT_AVAILABLE(83),
/**
*
* The following are experimental and subject to change.
*
*
* DERIVED_FEATURE_BAD_LIFECYCLE = 84;
*/
DERIVED_FEATURE_BAD_LIFECYCLE(84),
/**
*
* A derived feature is represented in the schema with an invalid or missing
* validation_derived_source.
*
*
* DERIVED_FEATURE_INVALID_SOURCE = 85;
*/
DERIVED_FEATURE_INVALID_SOURCE(85),
/**
*
* The following type is experimental and subject to change.
* The statistics did not specify a custom validation condition.
*
*
* CUSTOM_VALIDATION = 86;
*/
CUSTOM_VALIDATION(86),
;
/**
* UNKNOWN_TYPE = 0;
*/
public static final int UNKNOWN_TYPE_VALUE = 0;
/**
*
* Multiple reasons for anomaly.
*
*
* MULTIPLE_REASONS = 82;
*/
public static final int MULTIPLE_REASONS_VALUE = 82;
/**
*
* Integer larger than 1
*
*
* BOOL_TYPE_BIG_INT = 1;
*/
public static final int BOOL_TYPE_BIG_INT_VALUE = 1;
/**
*
* BYTES type when expected INT type
*
*
* BOOL_TYPE_BYTES_NOT_INT = 2;
*/
public static final int BOOL_TYPE_BYTES_NOT_INT_VALUE = 2;
/**
*
* BYTES type when expected STRING type
*
*
* BOOL_TYPE_BYTES_NOT_STRING = 3;
*/
public static final int BOOL_TYPE_BYTES_NOT_STRING_VALUE = 3;
/**
*
* FLOAT type when expected INT type
*
*
* BOOL_TYPE_FLOAT_NOT_INT = 4;
*/
public static final int BOOL_TYPE_FLOAT_NOT_INT_VALUE = 4;
/**
*
* FLOAT type when expected STRING type
*
*
* BOOL_TYPE_FLOAT_NOT_STRING = 5;
*/
public static final int BOOL_TYPE_FLOAT_NOT_STRING_VALUE = 5;
/**
*
* INT type when expected STRING type
*
*
* BOOL_TYPE_INT_NOT_STRING = 6;
*/
public static final int BOOL_TYPE_INT_NOT_STRING_VALUE = 6;
/**
*
* Integer smaller than 0
*
*
* BOOL_TYPE_SMALL_INT = 7;
*/
public static final int BOOL_TYPE_SMALL_INT_VALUE = 7;
/**
*
* STRING type when expected INT type
*
*
* BOOL_TYPE_STRING_NOT_INT = 8;
*/
public static final int BOOL_TYPE_STRING_NOT_INT_VALUE = 8;
/**
*
* Expected a string, but not the string seen
*
*
* BOOL_TYPE_UNEXPECTED_STRING = 9;
*/
public static final int BOOL_TYPE_UNEXPECTED_STRING_VALUE = 9;
/**
*
* Boolean had float values other than 0 and 1.
*
*
* BOOL_TYPE_UNEXPECTED_FLOAT = 52;
*/
public static final int BOOL_TYPE_UNEXPECTED_FLOAT_VALUE = 52;
/**
*
* BoolDomain has invalid configuration.
*
*
* BOOL_TYPE_INVALID_CONFIG = 88;
*/
public static final int BOOL_TYPE_INVALID_CONFIG_VALUE = 88;
/**
*
* BYTES type when expected STRING type
*
*
* ENUM_TYPE_BYTES_NOT_STRING = 10;
*/
public static final int ENUM_TYPE_BYTES_NOT_STRING_VALUE = 10;
/**
*
* FLOAT type when expected STRING type
*
*
* ENUM_TYPE_FLOAT_NOT_STRING = 11;
*/
public static final int ENUM_TYPE_FLOAT_NOT_STRING_VALUE = 11;
/**
*
* INT type when expected STRING type
*
*
* ENUM_TYPE_INT_NOT_STRING = 12;
*/
public static final int ENUM_TYPE_INT_NOT_STRING_VALUE = 12;
/**
*
* Invalid UTF8 string observed
*
*
* ENUM_TYPE_INVALID_UTF8 = 13;
*/
public static final int ENUM_TYPE_INVALID_UTF8_VALUE = 13;
/**
*
* Unexpected string values
*
*
* ENUM_TYPE_UNEXPECTED_STRING_VALUES = 14;
*/
public static final int ENUM_TYPE_UNEXPECTED_STRING_VALUES_VALUE = 14;
/**
*
* The number of values in a given example is too large
*
*
* FEATURE_TYPE_HIGH_NUMBER_VALUES = 15;
*/
public static final int FEATURE_TYPE_HIGH_NUMBER_VALUES_VALUE = 15;
/**
*
* The fraction of examples containing a feature is too small
*
*
* FEATURE_TYPE_LOW_FRACTION_PRESENT = 16;
*/
public static final int FEATURE_TYPE_LOW_FRACTION_PRESENT_VALUE = 16;
/**
*
* The number of examples containing a feature is too small
*
*
* FEATURE_TYPE_LOW_NUMBER_PRESENT = 17;
*/
public static final int FEATURE_TYPE_LOW_NUMBER_PRESENT_VALUE = 17;
/**
*
* The number of values in a given example is too small
*
*
* FEATURE_TYPE_LOW_NUMBER_VALUES = 18;
*/
public static final int FEATURE_TYPE_LOW_NUMBER_VALUES_VALUE = 18;
/**
*
* No examples contain the value
*
*
* FEATURE_TYPE_NOT_PRESENT = 19;
*/
public static final int FEATURE_TYPE_NOT_PRESENT_VALUE = 19;
/**
*
* The feature is present as an empty list
*
*
* FEATURE_TYPE_NO_VALUES = 20;
*/
public static final int FEATURE_TYPE_NO_VALUES_VALUE = 20;
/**
*
* The feature is repeated in an example, but was expected to be a singleton
*
*
* FEATURE_TYPE_UNEXPECTED_REPEATED = 21;
*/
public static final int FEATURE_TYPE_UNEXPECTED_REPEATED_VALUE = 21;
/**
*
* The feature had too many unique values (string and categorical features
* only).
*
*
* FEATURE_TYPE_HIGH_UNIQUE = 59;
*/
public static final int FEATURE_TYPE_HIGH_UNIQUE_VALUE = 59;
/**
*
* The feature had too few unique values (string and categorical features
* only).
*
*
* FEATURE_TYPE_LOW_UNIQUE = 60;
*/
public static final int FEATURE_TYPE_LOW_UNIQUE_VALUE = 60;
/**
*
* The feature has a constraint on the number of unique values but is not of
* a type that has the number of unique values counted (i.e., is not string
* or categorical).
*
*
* FEATURE_TYPE_NO_UNIQUE = 61;
*/
public static final int FEATURE_TYPE_NO_UNIQUE_VALUE = 61;
/**
*
* There is a float value that is too high
*
*
* FLOAT_TYPE_BIG_FLOAT = 22;
*/
public static final int FLOAT_TYPE_BIG_FLOAT_VALUE = 22;
/**
*
* The type is not FLOAT
*
*
* FLOAT_TYPE_NOT_FLOAT = 23;
*/
public static final int FLOAT_TYPE_NOT_FLOAT_VALUE = 23;
/**
*
* There is a float value that is too low
*
*
* FLOAT_TYPE_SMALL_FLOAT = 24;
*/
public static final int FLOAT_TYPE_SMALL_FLOAT_VALUE = 24;
/**
*
* The feature is supposed to be floats encoded as strings, but there is
* a string that is not a float
*
*
* FLOAT_TYPE_STRING_NOT_FLOAT = 25;
*/
public static final int FLOAT_TYPE_STRING_NOT_FLOAT_VALUE = 25;
/**
*
* The feature is supposed to be floats encoded as strings, but it was
* some other type (INT, BYTES, FLOAT)
*
*
* FLOAT_TYPE_NON_STRING = 26;
*/
public static final int FLOAT_TYPE_NON_STRING_VALUE = 26;
/**
*
* The type is completely unknown
*
*
* FLOAT_TYPE_UNKNOWN_TYPE_NUMBER = 27;
*/
public static final int FLOAT_TYPE_UNKNOWN_TYPE_NUMBER_VALUE = 27;
/**
*
* Float feature includes NaN values.
*
*
* FLOAT_TYPE_HAS_NAN = 53;
*/
public static final int FLOAT_TYPE_HAS_NAN_VALUE = 53;
/**
*
* Float feature includes Inf or -Inf values.
*
*
* FLOAT_TYPE_HAS_INF = 62;
*/
public static final int FLOAT_TYPE_HAS_INF_VALUE = 62;
/**
*
* There is an unexpectedly large integer
*
*
* INT_TYPE_BIG_INT = 28;
*/
public static final int INT_TYPE_BIG_INT_VALUE = 28;
/**
*
* The type was supposed to be INT, but it was not.
*
*
* INT_TYPE_INT_EXPECTED = 29;
*/
public static final int INT_TYPE_INT_EXPECTED_VALUE = 29;
/**
*
* The feature is supposed to be ints encoded as strings, but some string
* was not an int.
*
*
* INT_TYPE_NOT_INT_STRING = 30;
*/
public static final int INT_TYPE_NOT_INT_STRING_VALUE = 30;
/**
*
* The type was supposed to be STRING, but it was not.
*
*
* INT_TYPE_NOT_STRING = 31;
*/
public static final int INT_TYPE_NOT_STRING_VALUE = 31;
/**
*
* There is an unexpectedly small integer
*
*
* INT_TYPE_SMALL_INT = 32;
*/
public static final int INT_TYPE_SMALL_INT_VALUE = 32;
/**
*
* The feature is supposed to be ints encoded as strings, but it was
* some other type (INT, BYTES, FLOAT)
*
*
* INT_TYPE_STRING_EXPECTED = 33;
*/
public static final int INT_TYPE_STRING_EXPECTED_VALUE = 33;
/**
*
* Unknown type in stats proto
*
*
* INT_TYPE_UNKNOWN_TYPE_NUMBER = 34;
*/
public static final int INT_TYPE_UNKNOWN_TYPE_NUMBER_VALUE = 34;
/**
*
* The fraction of examples containing TensorFlow supported images is lower
* than the threshold set in the Schema.
*
*
* LOW_SUPPORTED_IMAGE_FRACTION = 64;
*/
public static final int LOW_SUPPORTED_IMAGE_FRACTION_VALUE = 64;
/**
*
* There are no stats for a column at all
*
*
* SCHEMA_MISSING_COLUMN = 35;
*/
public static final int SCHEMA_MISSING_COLUMN_VALUE = 35;
/**
*
* There is a new column that is not in the schema.
*
*
* SCHEMA_NEW_COLUMN = 36;
*/
public static final int SCHEMA_NEW_COLUMN_VALUE = 36;
/**
*
* Training serving skew issue
*
*
* SCHEMA_TRAINING_SERVING_SKEW = 37;
*/
public static final int SCHEMA_TRAINING_SERVING_SKEW_VALUE = 37;
/**
*
* Expected STRING type, but it was FLOAT.
*
*
* STRING_TYPE_NOW_FLOAT = 38;
*/
public static final int STRING_TYPE_NOW_FLOAT_VALUE = 38;
/**
*
* Expected STRING type, but it was INT.
*
*
* STRING_TYPE_NOW_INT = 39;
*/
public static final int STRING_TYPE_NOW_INT_VALUE = 39;
/**
*
* Control data is missing (either scoring data or previous day).
*
*
* COMPARATOR_CONTROL_DATA_MISSING = 40;
*/
public static final int COMPARATOR_CONTROL_DATA_MISSING_VALUE = 40;
/**
*
* Treatment data is missing (either treatment data or current day).
*
*
* COMPARATOR_TREATMENT_DATA_MISSING = 41;
*/
public static final int COMPARATOR_TREATMENT_DATA_MISSING_VALUE = 41;
/**
*
* L infinity between treatment and control is high.
*
*
* COMPARATOR_L_INFTY_HIGH = 42;
*/
public static final int COMPARATOR_L_INFTY_HIGH_VALUE = 42;
/**
*
* Approximate Jensen-Shannon divergence between treatment and control is
* high.
*
*
* COMPARATOR_JENSEN_SHANNON_DIVERGENCE_HIGH = 63;
*/
public static final int COMPARATOR_JENSEN_SHANNON_DIVERGENCE_HIGH_VALUE = 63;
/**
*
* The normalized absolute difference between treatment and control is high.
*
*
* COMPARATOR_NORMALIZED_ABSOLUTE_DIFFERENCE_HIGH = 87;
*/
public static final int COMPARATOR_NORMALIZED_ABSOLUTE_DIFFERENCE_HIGH_VALUE = 87;
/**
*
* No examples in the span.
*
*
* NO_DATA_IN_SPAN = 43;
*/
public static final int NO_DATA_IN_SPAN_VALUE = 43;
/**
*
* The value feature of a sparse feature is missing and at least one
* feature defining the sparse feature is present.
*
*
* SPARSE_FEATURE_MISSING_VALUE = 44;
*/
public static final int SPARSE_FEATURE_MISSING_VALUE_VALUE = 44;
/**
*
* An index feature of a sparse feature is missing and at least one
* feature defining the sparse feature is present.
*
*
* SPARSE_FEATURE_MISSING_INDEX = 45;
*/
public static final int SPARSE_FEATURE_MISSING_INDEX_VALUE = 45;
/**
*
* The length of the features representing a sparse feature does not match.
*
*
* SPARSE_FEATURE_LENGTH_MISMATCH = 46;
*/
public static final int SPARSE_FEATURE_LENGTH_MISMATCH_VALUE = 46;
/**
*
* Name collision between a sparse feature and raw feature.
*
*
* SPARSE_FEATURE_NAME_COLLISION = 47;
*/
public static final int SPARSE_FEATURE_NAME_COLLISION_VALUE = 47;
/**
*
* Invalid custom semantic domain.
*
*
* SEMANTIC_DOMAIN_UPDATE = 48;
*/
public static final int SEMANTIC_DOMAIN_UPDATE_VALUE = 48;
/**
*
* There are not enough examples in the current data as compared to a
* control dataset.
*
*
* COMPARATOR_LOW_NUM_EXAMPLES = 49;
*/
public static final int COMPARATOR_LOW_NUM_EXAMPLES_VALUE = 49;
/**
*
* There are too many examples in the current data as compared to a control
* dataset.
*
*
* COMPARATOR_HIGH_NUM_EXAMPLES = 50;
*/
public static final int COMPARATOR_HIGH_NUM_EXAMPLES_VALUE = 50;
/**
*
* There are not enough examples in the dataset.
*
*
* DATASET_LOW_NUM_EXAMPLES = 51;
*/
public static final int DATASET_LOW_NUM_EXAMPLES_VALUE = 51;
/**
*
* There are too many examples in the dataset.
*
*
* DATASET_HIGH_NUM_EXAMPLES = 58;
*/
public static final int DATASET_HIGH_NUM_EXAMPLES_VALUE = 58;
/**
*
* Name collision between a weighted feature and a raw feature.
*
*
* WEIGHTED_FEATURE_NAME_COLLISION = 54;
*/
public static final int WEIGHTED_FEATURE_NAME_COLLISION_VALUE = 54;
/**
*
* The value feature of a weighted feature is missing on examples where the
* weight feature is present.
*
*
* WEIGHTED_FEATURE_MISSING_VALUE = 55;
*/
public static final int WEIGHTED_FEATURE_MISSING_VALUE_VALUE = 55;
/**
*
* The weight feature of a weighted feature is missing on examples where the
* value feature is present.
*
*
* WEIGHTED_FEATURE_MISSING_WEIGHT = 56;
*/
public static final int WEIGHTED_FEATURE_MISSING_WEIGHT_VALUE = 56;
/**
*
* The length of the features representing a weighted feature does not
* match.
*
*
* WEIGHTED_FEATURE_LENGTH_MISMATCH = 57;
*/
public static final int WEIGHTED_FEATURE_LENGTH_MISMATCH_VALUE = 57;
/**
*
* The nesting level of the feature values does not match.
*
*
* VALUE_NESTEDNESS_MISMATCH = 65;
*/
public static final int VALUE_NESTEDNESS_MISMATCH_VALUE = 65;
/**
*
* The domain specified is not compatible with the physical type.
*
*
* DOMAIN_INVALID_FOR_TYPE = 66;
*/
public static final int DOMAIN_INVALID_FOR_TYPE_VALUE = 66;
/**
*
* Feature on schema has no name.
*
*
* FEATURE_MISSING_NAME = 67;
*/
public static final int FEATURE_MISSING_NAME_VALUE = 67;
/**
*
* Feature on schema has no type.
*
*
* FEATURE_MISSING_TYPE = 68;
*/
public static final int FEATURE_MISSING_TYPE_VALUE = 68;
/**
*
* Triggered for invalid schema specifications, e.g. min_fraction < 0.
*
*
* INVALID_SCHEMA_SPECIFICATION = 69;
*/
public static final int INVALID_SCHEMA_SPECIFICATION_VALUE = 69;
/**
*
* Triggered for invalid domain specifications in schema.
*
*
* INVALID_DOMAIN_SPECIFICATION = 81;
*/
public static final int INVALID_DOMAIN_SPECIFICATION_VALUE = 81;
/**
*
* The type of the data is inconsistent with the specified type.
*
*
* UNEXPECTED_DATA_TYPE = 70;
*/
public static final int UNEXPECTED_DATA_TYPE_VALUE = 70;
/**
*
* A value did not show up the min number of times within a sequence.
*
*
* SEQUENCE_VALUE_TOO_FEW_OCCURRENCES = 71;
*/
public static final int SEQUENCE_VALUE_TOO_FEW_OCCURRENCES_VALUE = 71;
/**
*
* A value showed up more the max number of times within a sequence.
*
*
* SEQUENCE_VALUE_TOO_MANY_OCCURRENCES = 72;
*/
public static final int SEQUENCE_VALUE_TOO_MANY_OCCURRENCES_VALUE = 72;
/**
*
* A value did not show up in at least the min fraction of sequences.
*
*
* SEQUENCE_VALUE_TOO_SMALL_FRACTION = 73;
*/
public static final int SEQUENCE_VALUE_TOO_SMALL_FRACTION_VALUE = 73;
/**
*
* A value showed up in greater than the max fraction of sequences.
*
*
* SEQUENCE_VALUE_TOO_LARGE_FRACTION = 74;
*/
public static final int SEQUENCE_VALUE_TOO_LARGE_FRACTION_VALUE = 74;
/**
*
* Too small a fraction of feature values matched vocab entries.
*
*
* FEATURE_COVERAGE_TOO_LOW = 75;
*/
public static final int FEATURE_COVERAGE_TOO_LOW_VALUE = 75;
/**
*
* The average token length was too short.
*
*
* FEATURE_COVERAGE_TOO_SHORT_AVG_TOKEN_LENGTH = 76;
*/
public static final int FEATURE_COVERAGE_TOO_SHORT_AVG_TOKEN_LENGTH_VALUE = 76;
/**
*
* A sequence violated the location constraint.
*
*
* NLP_WRONG_LOCATION = 77;
*/
public static final int NLP_WRONG_LOCATION_VALUE = 77;
/**
*
* A feature was specified as an embedding but was not a fixed dimension.
*
*
* EMBEDDING_SHAPE_INVALID = 78;
*/
public static final int EMBEDDING_SHAPE_INVALID_VALUE = 78;
/**
*
* A feature contains an image that has more bytes than the max byte size.
*
*
* MAX_IMAGE_BYTE_SIZE_EXCEEDED = 79;
*/
public static final int MAX_IMAGE_BYTE_SIZE_EXCEEDED_VALUE = 79;
/**
*
* A feature is supposed to be of a fixed shape but its valency stats
* do not agree.
*
*
* INVALID_FEATURE_SHAPE = 80;
*/
public static final int INVALID_FEATURE_SHAPE_VALUE = 80;
/**
*
* Constraints are specified within the but cannot be verified because the
* corresponding stats are not available.
*
*
* STATS_NOT_AVAILABLE = 83;
*/
public static final int STATS_NOT_AVAILABLE_VALUE = 83;
/**
*
* The following are experimental and subject to change.
*
*
* DERIVED_FEATURE_BAD_LIFECYCLE = 84;
*/
public static final int DERIVED_FEATURE_BAD_LIFECYCLE_VALUE = 84;
/**
*
* A derived feature is represented in the schema with an invalid or missing
* validation_derived_source.
*
*
* DERIVED_FEATURE_INVALID_SOURCE = 85;
*/
public static final int DERIVED_FEATURE_INVALID_SOURCE_VALUE = 85;
/**
*
* The following type is experimental and subject to change.
* The statistics did not specify a custom validation condition.
*
*
* CUSTOM_VALIDATION = 86;
*/
public static final int CUSTOM_VALIDATION_VALUE = 86;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Type forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_TYPE;
case 82: return MULTIPLE_REASONS;
case 1: return BOOL_TYPE_BIG_INT;
case 2: return BOOL_TYPE_BYTES_NOT_INT;
case 3: return BOOL_TYPE_BYTES_NOT_STRING;
case 4: return BOOL_TYPE_FLOAT_NOT_INT;
case 5: return BOOL_TYPE_FLOAT_NOT_STRING;
case 6: return BOOL_TYPE_INT_NOT_STRING;
case 7: return BOOL_TYPE_SMALL_INT;
case 8: return BOOL_TYPE_STRING_NOT_INT;
case 9: return BOOL_TYPE_UNEXPECTED_STRING;
case 52: return BOOL_TYPE_UNEXPECTED_FLOAT;
case 88: return BOOL_TYPE_INVALID_CONFIG;
case 10: return ENUM_TYPE_BYTES_NOT_STRING;
case 11: return ENUM_TYPE_FLOAT_NOT_STRING;
case 12: return ENUM_TYPE_INT_NOT_STRING;
case 13: return ENUM_TYPE_INVALID_UTF8;
case 14: return ENUM_TYPE_UNEXPECTED_STRING_VALUES;
case 15: return FEATURE_TYPE_HIGH_NUMBER_VALUES;
case 16: return FEATURE_TYPE_LOW_FRACTION_PRESENT;
case 17: return FEATURE_TYPE_LOW_NUMBER_PRESENT;
case 18: return FEATURE_TYPE_LOW_NUMBER_VALUES;
case 19: return FEATURE_TYPE_NOT_PRESENT;
case 20: return FEATURE_TYPE_NO_VALUES;
case 21: return FEATURE_TYPE_UNEXPECTED_REPEATED;
case 59: return FEATURE_TYPE_HIGH_UNIQUE;
case 60: return FEATURE_TYPE_LOW_UNIQUE;
case 61: return FEATURE_TYPE_NO_UNIQUE;
case 22: return FLOAT_TYPE_BIG_FLOAT;
case 23: return FLOAT_TYPE_NOT_FLOAT;
case 24: return FLOAT_TYPE_SMALL_FLOAT;
case 25: return FLOAT_TYPE_STRING_NOT_FLOAT;
case 26: return FLOAT_TYPE_NON_STRING;
case 27: return FLOAT_TYPE_UNKNOWN_TYPE_NUMBER;
case 53: return FLOAT_TYPE_HAS_NAN;
case 62: return FLOAT_TYPE_HAS_INF;
case 28: return INT_TYPE_BIG_INT;
case 29: return INT_TYPE_INT_EXPECTED;
case 30: return INT_TYPE_NOT_INT_STRING;
case 31: return INT_TYPE_NOT_STRING;
case 32: return INT_TYPE_SMALL_INT;
case 33: return INT_TYPE_STRING_EXPECTED;
case 34: return INT_TYPE_UNKNOWN_TYPE_NUMBER;
case 64: return LOW_SUPPORTED_IMAGE_FRACTION;
case 35: return SCHEMA_MISSING_COLUMN;
case 36: return SCHEMA_NEW_COLUMN;
case 37: return SCHEMA_TRAINING_SERVING_SKEW;
case 38: return STRING_TYPE_NOW_FLOAT;
case 39: return STRING_TYPE_NOW_INT;
case 40: return COMPARATOR_CONTROL_DATA_MISSING;
case 41: return COMPARATOR_TREATMENT_DATA_MISSING;
case 42: return COMPARATOR_L_INFTY_HIGH;
case 63: return COMPARATOR_JENSEN_SHANNON_DIVERGENCE_HIGH;
case 87: return COMPARATOR_NORMALIZED_ABSOLUTE_DIFFERENCE_HIGH;
case 43: return NO_DATA_IN_SPAN;
case 44: return SPARSE_FEATURE_MISSING_VALUE;
case 45: return SPARSE_FEATURE_MISSING_INDEX;
case 46: return SPARSE_FEATURE_LENGTH_MISMATCH;
case 47: return SPARSE_FEATURE_NAME_COLLISION;
case 48: return SEMANTIC_DOMAIN_UPDATE;
case 49: return COMPARATOR_LOW_NUM_EXAMPLES;
case 50: return COMPARATOR_HIGH_NUM_EXAMPLES;
case 51: return DATASET_LOW_NUM_EXAMPLES;
case 58: return DATASET_HIGH_NUM_EXAMPLES;
case 54: return WEIGHTED_FEATURE_NAME_COLLISION;
case 55: return WEIGHTED_FEATURE_MISSING_VALUE;
case 56: return WEIGHTED_FEATURE_MISSING_WEIGHT;
case 57: return WEIGHTED_FEATURE_LENGTH_MISMATCH;
case 65: return VALUE_NESTEDNESS_MISMATCH;
case 66: return DOMAIN_INVALID_FOR_TYPE;
case 67: return FEATURE_MISSING_NAME;
case 68: return FEATURE_MISSING_TYPE;
case 69: return INVALID_SCHEMA_SPECIFICATION;
case 81: return INVALID_DOMAIN_SPECIFICATION;
case 70: return UNEXPECTED_DATA_TYPE;
case 71: return SEQUENCE_VALUE_TOO_FEW_OCCURRENCES;
case 72: return SEQUENCE_VALUE_TOO_MANY_OCCURRENCES;
case 73: return SEQUENCE_VALUE_TOO_SMALL_FRACTION;
case 74: return SEQUENCE_VALUE_TOO_LARGE_FRACTION;
case 75: return FEATURE_COVERAGE_TOO_LOW;
case 76: return FEATURE_COVERAGE_TOO_SHORT_AVG_TOKEN_LENGTH;
case 77: return NLP_WRONG_LOCATION;
case 78: return EMBEDDING_SHAPE_INVALID;
case 79: return MAX_IMAGE_BYTE_SIZE_EXCEEDED;
case 80: return INVALID_FEATURE_SHAPE;
case 83: return STATS_NOT_AVAILABLE;
case 84: return DERIVED_FEATURE_BAD_LIFECYCLE;
case 85: return DERIVED_FEATURE_INVALID_SOURCE;
case 86: return CUSTOM_VALIDATION;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tensorflow.metadata.v0.AnomalyInfo.getDescriptor().getEnumTypes().get(1);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.metadata.v0.AnomalyInfo.Type)
}
public interface ReasonOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.metadata.v0.AnomalyInfo.Reason)
com.google.protobuf.MessageOrBuilder {
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @return Whether the type field is set.
*/
boolean hasType();
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @return The type.
*/
org.tensorflow.metadata.v0.AnomalyInfo.Type getType();
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return Whether the shortDescription field is set.
*/
boolean hasShortDescription();
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return The shortDescription.
*/
java.lang.String getShortDescription();
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return The bytes for shortDescription.
*/
com.google.protobuf.ByteString
getShortDescriptionBytes();
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return Whether the description field is set.
*/
boolean hasDescription();
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return The description.
*/
java.lang.String getDescription();
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return The bytes for description.
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
*
* LINT.ThenChange(//tensorflow_data_validation/g3doc/anomalies.md)
* Reason for the anomaly. There may be more than one reason,
* e.g. the field might be missing sometimes AND a new value is
* present.
*
*
* Protobuf type {@code tensorflow.metadata.v0.AnomalyInfo.Reason}
*/
public static final class Reason extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.AnomalyInfo.Reason)
ReasonOrBuilder {
private static final long serialVersionUID = 0L;
// Use Reason.newBuilder() to construct.
private Reason(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Reason() {
type_ = 0;
shortDescription_ = "";
description_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Reason();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_Reason_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_Reason_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.AnomalyInfo.Reason.class, org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder.class);
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_ = 0;
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @return The type.
*/
@java.lang.Override public org.tensorflow.metadata.v0.AnomalyInfo.Type getType() {
org.tensorflow.metadata.v0.AnomalyInfo.Type result = org.tensorflow.metadata.v0.AnomalyInfo.Type.forNumber(type_);
return result == null ? org.tensorflow.metadata.v0.AnomalyInfo.Type.UNKNOWN_TYPE : result;
}
public static final int SHORT_DESCRIPTION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object shortDescription_ = "";
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return Whether the shortDescription field is set.
*/
@java.lang.Override
public boolean hasShortDescription() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return The shortDescription.
*/
@java.lang.Override
public java.lang.String getShortDescription() {
java.lang.Object ref = shortDescription_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortDescription_ = s;
}
return s;
}
}
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return The bytes for shortDescription.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getShortDescriptionBytes() {
java.lang.Object ref = shortDescription_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
}
}
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, shortDescription_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, description_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, shortDescription_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, description_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.AnomalyInfo.Reason)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.AnomalyInfo.Reason other = (org.tensorflow.metadata.v0.AnomalyInfo.Reason) obj;
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasShortDescription() != other.hasShortDescription()) return false;
if (hasShortDescription()) {
if (!getShortDescription()
.equals(other.getShortDescription())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription()
.equals(other.getDescription())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasShortDescription()) {
hash = (37 * hash) + SHORT_DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getShortDescription().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.AnomalyInfo.Reason prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* LINT.ThenChange(//tensorflow_data_validation/g3doc/anomalies.md)
* Reason for the anomaly. There may be more than one reason,
* e.g. the field might be missing sometimes AND a new value is
* present.
*
*
* Protobuf type {@code tensorflow.metadata.v0.AnomalyInfo.Reason}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.AnomalyInfo.Reason)
org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_Reason_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_Reason_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.AnomalyInfo.Reason.class, org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.AnomalyInfo.Reason.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
type_ = 0;
shortDescription_ = "";
description_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_Reason_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.Reason getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.AnomalyInfo.Reason.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.Reason build() {
org.tensorflow.metadata.v0.AnomalyInfo.Reason result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.Reason buildPartial() {
org.tensorflow.metadata.v0.AnomalyInfo.Reason result = new org.tensorflow.metadata.v0.AnomalyInfo.Reason(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tensorflow.metadata.v0.AnomalyInfo.Reason result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.shortDescription_ = shortDescription_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.AnomalyInfo.Reason) {
return mergeFrom((org.tensorflow.metadata.v0.AnomalyInfo.Reason)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.AnomalyInfo.Reason other) {
if (other == org.tensorflow.metadata.v0.AnomalyInfo.Reason.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasShortDescription()) {
shortDescription_ = other.shortDescription_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int tmpRaw = input.readEnum();
org.tensorflow.metadata.v0.AnomalyInfo.Type tmpValue =
org.tensorflow.metadata.v0.AnomalyInfo.Type.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(1, tmpRaw);
} else {
type_ = tmpRaw;
bitField0_ |= 0x00000001;
}
break;
} // case 8
case 18: {
shortDescription_ = input.readBytes();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
description_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int type_ = 0;
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @return The type.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.Type getType() {
org.tensorflow.metadata.v0.AnomalyInfo.Type result = org.tensorflow.metadata.v0.AnomalyInfo.Type.forNumber(type_);
return result == null ? org.tensorflow.metadata.v0.AnomalyInfo.Type.UNKNOWN_TYPE : result;
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.tensorflow.metadata.v0.AnomalyInfo.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Type type = 1 [default = UNKNOWN_TYPE];
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
private java.lang.Object shortDescription_ = "";
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return Whether the shortDescription field is set.
*/
public boolean hasShortDescription() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return The shortDescription.
*/
public java.lang.String getShortDescription() {
java.lang.Object ref = shortDescription_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortDescription_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return The bytes for shortDescription.
*/
public com.google.protobuf.ByteString
getShortDescriptionBytes() {
java.lang.Object ref = shortDescription_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @param value The shortDescription to set.
* @return This builder for chaining.
*/
public Builder setShortDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
shortDescription_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @return This builder for chaining.
*/
public Builder clearShortDescription() {
shortDescription_ = getDefaultInstance().getShortDescription();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* A short description of an anomaly, suitable for UI presentation.
*
*
* optional string short_description = 2;
* @param value The bytes for shortDescription to set.
* @return This builder for chaining.
*/
public Builder setShortDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
shortDescription_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* A longer description of an anomaly.
*
*
* optional string description = 3;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.AnomalyInfo.Reason)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.AnomalyInfo.Reason)
private static final org.tensorflow.metadata.v0.AnomalyInfo.Reason DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.AnomalyInfo.Reason();
}
public static org.tensorflow.metadata.v0.AnomalyInfo.Reason getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Reason parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.Reason getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int PATH_FIELD_NUMBER = 8;
private org.tensorflow.metadata.v0.Path path_;
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
* @return Whether the path field is set.
*/
@java.lang.Override
public boolean hasPath() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
* @return The path.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.Path getPath() {
return path_ == null ? org.tensorflow.metadata.v0.Path.getDefaultInstance() : path_;
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.PathOrBuilder getPathOrBuilder() {
return path_ == null ? org.tensorflow.metadata.v0.Path.getDefaultInstance() : path_;
}
public static final int SEVERITY_FIELD_NUMBER = 5;
private int severity_ = 0;
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Severity severity = 5;
* @return Whether the severity field is set.
*/
@java.lang.Override public boolean hasSeverity() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Severity severity = 5;
* @return The severity.
*/
@java.lang.Override public org.tensorflow.metadata.v0.AnomalyInfo.Severity getSeverity() {
org.tensorflow.metadata.v0.AnomalyInfo.Severity result = org.tensorflow.metadata.v0.AnomalyInfo.Severity.forNumber(severity_);
return result == null ? org.tensorflow.metadata.v0.AnomalyInfo.Severity.UNKNOWN : result;
}
public static final int DESCRIPTION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
}
}
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SHORT_DESCRIPTION_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object shortDescription_ = "";
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @return Whether the shortDescription field is set.
*/
@java.lang.Override
public boolean hasShortDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @return The shortDescription.
*/
@java.lang.Override
public java.lang.String getShortDescription() {
java.lang.Object ref = shortDescription_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortDescription_ = s;
}
return s;
}
}
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @return The bytes for shortDescription.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getShortDescriptionBytes() {
java.lang.Object ref = shortDescription_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DIFF_REGIONS_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private java.util.List diffRegions_;
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
@java.lang.Override
public java.util.List getDiffRegionsList() {
return diffRegions_;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
@java.lang.Override
public java.util.List extends org.tensorflow.metadata.v0.DiffRegionOrBuilder>
getDiffRegionsOrBuilderList() {
return diffRegions_;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
@java.lang.Override
public int getDiffRegionsCount() {
return diffRegions_.size();
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.DiffRegion getDiffRegions(int index) {
return diffRegions_.get(index);
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.DiffRegionOrBuilder getDiffRegionsOrBuilder(
int index) {
return diffRegions_.get(index);
}
public static final int REASON_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List reason_;
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
@java.lang.Override
public java.util.List getReasonList() {
return reason_;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
@java.lang.Override
public java.util.List extends org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder>
getReasonOrBuilderList() {
return reason_;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
@java.lang.Override
public int getReasonCount() {
return reason_.size();
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.Reason getReason(int index) {
return reason_.get(index);
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder getReasonOrBuilder(
int index) {
return reason_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, description_);
}
for (int i = 0; i < diffRegions_.size(); i++) {
output.writeMessage(4, diffRegions_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeEnum(5, severity_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, shortDescription_);
}
for (int i = 0; i < reason_.size(); i++) {
output.writeMessage(7, reason_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(8, getPath());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, description_);
}
for (int i = 0; i < diffRegions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, diffRegions_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, severity_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, shortDescription_);
}
for (int i = 0; i < reason_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, reason_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getPath());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.AnomalyInfo)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.AnomalyInfo other = (org.tensorflow.metadata.v0.AnomalyInfo) obj;
if (hasPath() != other.hasPath()) return false;
if (hasPath()) {
if (!getPath()
.equals(other.getPath())) return false;
}
if (hasSeverity() != other.hasSeverity()) return false;
if (hasSeverity()) {
if (severity_ != other.severity_) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription()
.equals(other.getDescription())) return false;
}
if (hasShortDescription() != other.hasShortDescription()) return false;
if (hasShortDescription()) {
if (!getShortDescription()
.equals(other.getShortDescription())) return false;
}
if (!getDiffRegionsList()
.equals(other.getDiffRegionsList())) return false;
if (!getReasonList()
.equals(other.getReasonList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
if (hasSeverity()) {
hash = (37 * hash) + SEVERITY_FIELD_NUMBER;
hash = (53 * hash) + severity_;
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasShortDescription()) {
hash = (37 * hash) + SHORT_DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getShortDescription().hashCode();
}
if (getDiffRegionsCount() > 0) {
hash = (37 * hash) + DIFF_REGIONS_FIELD_NUMBER;
hash = (53 * hash) + getDiffRegionsList().hashCode();
}
if (getReasonCount() > 0) {
hash = (37 * hash) + REASON_FIELD_NUMBER;
hash = (53 * hash) + getReasonList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.AnomalyInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.AnomalyInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Message to represent information about an individual anomaly.
*
*
* Protobuf type {@code tensorflow.metadata.v0.AnomalyInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.AnomalyInfo)
org.tensorflow.metadata.v0.AnomalyInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.AnomalyInfo.class, org.tensorflow.metadata.v0.AnomalyInfo.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.AnomalyInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPathFieldBuilder();
getDiffRegionsFieldBuilder();
getReasonFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
path_ = null;
if (pathBuilder_ != null) {
pathBuilder_.dispose();
pathBuilder_ = null;
}
severity_ = 0;
description_ = "";
shortDescription_ = "";
if (diffRegionsBuilder_ == null) {
diffRegions_ = java.util.Collections.emptyList();
} else {
diffRegions_ = null;
diffRegionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (reasonBuilder_ == null) {
reason_ = java.util.Collections.emptyList();
} else {
reason_ = null;
reasonBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.AnomaliesOuterClass.internal_static_tensorflow_metadata_v0_AnomalyInfo_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.AnomalyInfo.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo build() {
org.tensorflow.metadata.v0.AnomalyInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo buildPartial() {
org.tensorflow.metadata.v0.AnomalyInfo result = new org.tensorflow.metadata.v0.AnomalyInfo(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.tensorflow.metadata.v0.AnomalyInfo result) {
if (diffRegionsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
diffRegions_ = java.util.Collections.unmodifiableList(diffRegions_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.diffRegions_ = diffRegions_;
} else {
result.diffRegions_ = diffRegionsBuilder_.build();
}
if (reasonBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
reason_ = java.util.Collections.unmodifiableList(reason_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.reason_ = reason_;
} else {
result.reason_ = reasonBuilder_.build();
}
}
private void buildPartial0(org.tensorflow.metadata.v0.AnomalyInfo result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.path_ = pathBuilder_ == null
? path_
: pathBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.severity_ = severity_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.shortDescription_ = shortDescription_;
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.AnomalyInfo) {
return mergeFrom((org.tensorflow.metadata.v0.AnomalyInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.AnomalyInfo other) {
if (other == org.tensorflow.metadata.v0.AnomalyInfo.getDefaultInstance()) return this;
if (other.hasPath()) {
mergePath(other.getPath());
}
if (other.hasSeverity()) {
setSeverity(other.getSeverity());
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasShortDescription()) {
shortDescription_ = other.shortDescription_;
bitField0_ |= 0x00000008;
onChanged();
}
if (diffRegionsBuilder_ == null) {
if (!other.diffRegions_.isEmpty()) {
if (diffRegions_.isEmpty()) {
diffRegions_ = other.diffRegions_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureDiffRegionsIsMutable();
diffRegions_.addAll(other.diffRegions_);
}
onChanged();
}
} else {
if (!other.diffRegions_.isEmpty()) {
if (diffRegionsBuilder_.isEmpty()) {
diffRegionsBuilder_.dispose();
diffRegionsBuilder_ = null;
diffRegions_ = other.diffRegions_;
bitField0_ = (bitField0_ & ~0x00000010);
diffRegionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDiffRegionsFieldBuilder() : null;
} else {
diffRegionsBuilder_.addAllMessages(other.diffRegions_);
}
}
}
if (reasonBuilder_ == null) {
if (!other.reason_.isEmpty()) {
if (reason_.isEmpty()) {
reason_ = other.reason_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureReasonIsMutable();
reason_.addAll(other.reason_);
}
onChanged();
}
} else {
if (!other.reason_.isEmpty()) {
if (reasonBuilder_.isEmpty()) {
reasonBuilder_.dispose();
reasonBuilder_ = null;
reason_ = other.reason_;
bitField0_ = (bitField0_ & ~0x00000020);
reasonBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getReasonFieldBuilder() : null;
} else {
reasonBuilder_.addAllMessages(other.reason_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18: {
description_ = input.readBytes();
bitField0_ |= 0x00000004;
break;
} // case 18
case 34: {
org.tensorflow.metadata.v0.DiffRegion m =
input.readMessage(
org.tensorflow.metadata.v0.DiffRegion.PARSER,
extensionRegistry);
if (diffRegionsBuilder_ == null) {
ensureDiffRegionsIsMutable();
diffRegions_.add(m);
} else {
diffRegionsBuilder_.addMessage(m);
}
break;
} // case 34
case 40: {
int tmpRaw = input.readEnum();
org.tensorflow.metadata.v0.AnomalyInfo.Severity tmpValue =
org.tensorflow.metadata.v0.AnomalyInfo.Severity.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(5, tmpRaw);
} else {
severity_ = tmpRaw;
bitField0_ |= 0x00000002;
}
break;
} // case 40
case 50: {
shortDescription_ = input.readBytes();
bitField0_ |= 0x00000008;
break;
} // case 50
case 58: {
org.tensorflow.metadata.v0.AnomalyInfo.Reason m =
input.readMessage(
org.tensorflow.metadata.v0.AnomalyInfo.Reason.PARSER,
extensionRegistry);
if (reasonBuilder_ == null) {
ensureReasonIsMutable();
reason_.add(m);
} else {
reasonBuilder_.addMessage(m);
}
break;
} // case 58
case 66: {
input.readMessage(
getPathFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 66
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.tensorflow.metadata.v0.Path path_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Path, org.tensorflow.metadata.v0.Path.Builder, org.tensorflow.metadata.v0.PathOrBuilder> pathBuilder_;
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
* @return Whether the path field is set.
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
* @return The path.
*/
public org.tensorflow.metadata.v0.Path getPath() {
if (pathBuilder_ == null) {
return path_ == null ? org.tensorflow.metadata.v0.Path.getDefaultInstance() : path_;
} else {
return pathBuilder_.getMessage();
}
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
public Builder setPath(org.tensorflow.metadata.v0.Path value) {
if (pathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
path_ = value;
} else {
pathBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
public Builder setPath(
org.tensorflow.metadata.v0.Path.Builder builderForValue) {
if (pathBuilder_ == null) {
path_ = builderForValue.build();
} else {
pathBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
public Builder mergePath(org.tensorflow.metadata.v0.Path value) {
if (pathBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
path_ != null &&
path_ != org.tensorflow.metadata.v0.Path.getDefaultInstance()) {
getPathBuilder().mergeFrom(value);
} else {
path_ = value;
}
} else {
pathBuilder_.mergeFrom(value);
}
if (path_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
public Builder clearPath() {
bitField0_ = (bitField0_ & ~0x00000001);
path_ = null;
if (pathBuilder_ != null) {
pathBuilder_.dispose();
pathBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
public org.tensorflow.metadata.v0.Path.Builder getPathBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getPathFieldBuilder().getBuilder();
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
public org.tensorflow.metadata.v0.PathOrBuilder getPathOrBuilder() {
if (pathBuilder_ != null) {
return pathBuilder_.getMessageOrBuilder();
} else {
return path_ == null ?
org.tensorflow.metadata.v0.Path.getDefaultInstance() : path_;
}
}
/**
*
* A path indicating where the anomaly occurred.
* Dataset-level anomalies do not have a path.
*
*
* optional .tensorflow.metadata.v0.Path path = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Path, org.tensorflow.metadata.v0.Path.Builder, org.tensorflow.metadata.v0.PathOrBuilder>
getPathFieldBuilder() {
if (pathBuilder_ == null) {
pathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Path, org.tensorflow.metadata.v0.Path.Builder, org.tensorflow.metadata.v0.PathOrBuilder>(
getPath(),
getParentForChildren(),
isClean());
path_ = null;
}
return pathBuilder_;
}
private int severity_ = 0;
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Severity severity = 5;
* @return Whether the severity field is set.
*/
@java.lang.Override public boolean hasSeverity() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Severity severity = 5;
* @return The severity.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo.Severity getSeverity() {
org.tensorflow.metadata.v0.AnomalyInfo.Severity result = org.tensorflow.metadata.v0.AnomalyInfo.Severity.forNumber(severity_);
return result == null ? org.tensorflow.metadata.v0.AnomalyInfo.Severity.UNKNOWN : result;
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Severity severity = 5;
* @param value The severity to set.
* @return This builder for chaining.
*/
public Builder setSeverity(org.tensorflow.metadata.v0.AnomalyInfo.Severity value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
severity_ = value.getNumber();
onChanged();
return this;
}
/**
* optional .tensorflow.metadata.v0.AnomalyInfo.Severity severity = 5;
* @return This builder for chaining.
*/
public Builder clearSeverity() {
bitField0_ = (bitField0_ & ~0x00000002);
severity_ = 0;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* A description of the entire anomaly.
*
*
* optional string description = 2;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object shortDescription_ = "";
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @return Whether the shortDescription field is set.
*/
public boolean hasShortDescription() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @return The shortDescription.
*/
public java.lang.String getShortDescription() {
java.lang.Object ref = shortDescription_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
shortDescription_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @return The bytes for shortDescription.
*/
public com.google.protobuf.ByteString
getShortDescriptionBytes() {
java.lang.Object ref = shortDescription_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
shortDescription_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @param value The shortDescription to set.
* @return This builder for chaining.
*/
public Builder setShortDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
shortDescription_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @return This builder for chaining.
*/
public Builder clearShortDescription() {
shortDescription_ = getDefaultInstance().getShortDescription();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* A shorter description, suitable for UI presentation.
* If there is a single reason for the anomaly, identical to
* reason[0].short_description. Otherwise, summarizes all the reasons.
*
*
* optional string short_description = 6;
* @param value The bytes for shortDescription to set.
* @return This builder for chaining.
*/
public Builder setShortDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
shortDescription_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.util.List diffRegions_ =
java.util.Collections.emptyList();
private void ensureDiffRegionsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
diffRegions_ = new java.util.ArrayList(diffRegions_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.DiffRegion, org.tensorflow.metadata.v0.DiffRegion.Builder, org.tensorflow.metadata.v0.DiffRegionOrBuilder> diffRegionsBuilder_;
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public java.util.List getDiffRegionsList() {
if (diffRegionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(diffRegions_);
} else {
return diffRegionsBuilder_.getMessageList();
}
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public int getDiffRegionsCount() {
if (diffRegionsBuilder_ == null) {
return diffRegions_.size();
} else {
return diffRegionsBuilder_.getCount();
}
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public org.tensorflow.metadata.v0.DiffRegion getDiffRegions(int index) {
if (diffRegionsBuilder_ == null) {
return diffRegions_.get(index);
} else {
return diffRegionsBuilder_.getMessage(index);
}
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder setDiffRegions(
int index, org.tensorflow.metadata.v0.DiffRegion value) {
if (diffRegionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDiffRegionsIsMutable();
diffRegions_.set(index, value);
onChanged();
} else {
diffRegionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder setDiffRegions(
int index, org.tensorflow.metadata.v0.DiffRegion.Builder builderForValue) {
if (diffRegionsBuilder_ == null) {
ensureDiffRegionsIsMutable();
diffRegions_.set(index, builderForValue.build());
onChanged();
} else {
diffRegionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder addDiffRegions(org.tensorflow.metadata.v0.DiffRegion value) {
if (diffRegionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDiffRegionsIsMutable();
diffRegions_.add(value);
onChanged();
} else {
diffRegionsBuilder_.addMessage(value);
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder addDiffRegions(
int index, org.tensorflow.metadata.v0.DiffRegion value) {
if (diffRegionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDiffRegionsIsMutable();
diffRegions_.add(index, value);
onChanged();
} else {
diffRegionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder addDiffRegions(
org.tensorflow.metadata.v0.DiffRegion.Builder builderForValue) {
if (diffRegionsBuilder_ == null) {
ensureDiffRegionsIsMutable();
diffRegions_.add(builderForValue.build());
onChanged();
} else {
diffRegionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder addDiffRegions(
int index, org.tensorflow.metadata.v0.DiffRegion.Builder builderForValue) {
if (diffRegionsBuilder_ == null) {
ensureDiffRegionsIsMutable();
diffRegions_.add(index, builderForValue.build());
onChanged();
} else {
diffRegionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder addAllDiffRegions(
java.lang.Iterable extends org.tensorflow.metadata.v0.DiffRegion> values) {
if (diffRegionsBuilder_ == null) {
ensureDiffRegionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, diffRegions_);
onChanged();
} else {
diffRegionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder clearDiffRegions() {
if (diffRegionsBuilder_ == null) {
diffRegions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
diffRegionsBuilder_.clear();
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public Builder removeDiffRegions(int index) {
if (diffRegionsBuilder_ == null) {
ensureDiffRegionsIsMutable();
diffRegions_.remove(index);
onChanged();
} else {
diffRegionsBuilder_.remove(index);
}
return this;
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public org.tensorflow.metadata.v0.DiffRegion.Builder getDiffRegionsBuilder(
int index) {
return getDiffRegionsFieldBuilder().getBuilder(index);
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public org.tensorflow.metadata.v0.DiffRegionOrBuilder getDiffRegionsOrBuilder(
int index) {
if (diffRegionsBuilder_ == null) {
return diffRegions_.get(index); } else {
return diffRegionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public java.util.List extends org.tensorflow.metadata.v0.DiffRegionOrBuilder>
getDiffRegionsOrBuilderList() {
if (diffRegionsBuilder_ != null) {
return diffRegionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(diffRegions_);
}
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public org.tensorflow.metadata.v0.DiffRegion.Builder addDiffRegionsBuilder() {
return getDiffRegionsFieldBuilder().addBuilder(
org.tensorflow.metadata.v0.DiffRegion.getDefaultInstance());
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public org.tensorflow.metadata.v0.DiffRegion.Builder addDiffRegionsBuilder(
int index) {
return getDiffRegionsFieldBuilder().addBuilder(
index, org.tensorflow.metadata.v0.DiffRegion.getDefaultInstance());
}
/**
*
* The comparison between the existing schema and the fixed schema.
*
*
* repeated .tensorflow.metadata.v0.DiffRegion diff_regions = 4;
*/
public java.util.List
getDiffRegionsBuilderList() {
return getDiffRegionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.DiffRegion, org.tensorflow.metadata.v0.DiffRegion.Builder, org.tensorflow.metadata.v0.DiffRegionOrBuilder>
getDiffRegionsFieldBuilder() {
if (diffRegionsBuilder_ == null) {
diffRegionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.DiffRegion, org.tensorflow.metadata.v0.DiffRegion.Builder, org.tensorflow.metadata.v0.DiffRegionOrBuilder>(
diffRegions_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
diffRegions_ = null;
}
return diffRegionsBuilder_;
}
private java.util.List reason_ =
java.util.Collections.emptyList();
private void ensureReasonIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
reason_ = new java.util.ArrayList(reason_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.AnomalyInfo.Reason, org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder, org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder> reasonBuilder_;
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public java.util.List getReasonList() {
if (reasonBuilder_ == null) {
return java.util.Collections.unmodifiableList(reason_);
} else {
return reasonBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public int getReasonCount() {
if (reasonBuilder_ == null) {
return reason_.size();
} else {
return reasonBuilder_.getCount();
}
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public org.tensorflow.metadata.v0.AnomalyInfo.Reason getReason(int index) {
if (reasonBuilder_ == null) {
return reason_.get(index);
} else {
return reasonBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder setReason(
int index, org.tensorflow.metadata.v0.AnomalyInfo.Reason value) {
if (reasonBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReasonIsMutable();
reason_.set(index, value);
onChanged();
} else {
reasonBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder setReason(
int index, org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder builderForValue) {
if (reasonBuilder_ == null) {
ensureReasonIsMutable();
reason_.set(index, builderForValue.build());
onChanged();
} else {
reasonBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder addReason(org.tensorflow.metadata.v0.AnomalyInfo.Reason value) {
if (reasonBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReasonIsMutable();
reason_.add(value);
onChanged();
} else {
reasonBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder addReason(
int index, org.tensorflow.metadata.v0.AnomalyInfo.Reason value) {
if (reasonBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureReasonIsMutable();
reason_.add(index, value);
onChanged();
} else {
reasonBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder addReason(
org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder builderForValue) {
if (reasonBuilder_ == null) {
ensureReasonIsMutable();
reason_.add(builderForValue.build());
onChanged();
} else {
reasonBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder addReason(
int index, org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder builderForValue) {
if (reasonBuilder_ == null) {
ensureReasonIsMutable();
reason_.add(index, builderForValue.build());
onChanged();
} else {
reasonBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder addAllReason(
java.lang.Iterable extends org.tensorflow.metadata.v0.AnomalyInfo.Reason> values) {
if (reasonBuilder_ == null) {
ensureReasonIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, reason_);
onChanged();
} else {
reasonBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder clearReason() {
if (reasonBuilder_ == null) {
reason_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
reasonBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public Builder removeReason(int index) {
if (reasonBuilder_ == null) {
ensureReasonIsMutable();
reason_.remove(index);
onChanged();
} else {
reasonBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder getReasonBuilder(
int index) {
return getReasonFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder getReasonOrBuilder(
int index) {
if (reasonBuilder_ == null) {
return reason_.get(index); } else {
return reasonBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public java.util.List extends org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder>
getReasonOrBuilderList() {
if (reasonBuilder_ != null) {
return reasonBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(reason_);
}
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder addReasonBuilder() {
return getReasonFieldBuilder().addBuilder(
org.tensorflow.metadata.v0.AnomalyInfo.Reason.getDefaultInstance());
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder addReasonBuilder(
int index) {
return getReasonFieldBuilder().addBuilder(
index, org.tensorflow.metadata.v0.AnomalyInfo.Reason.getDefaultInstance());
}
/**
* repeated .tensorflow.metadata.v0.AnomalyInfo.Reason reason = 7;
*/
public java.util.List
getReasonBuilderList() {
return getReasonFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.AnomalyInfo.Reason, org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder, org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder>
getReasonFieldBuilder() {
if (reasonBuilder_ == null) {
reasonBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.AnomalyInfo.Reason, org.tensorflow.metadata.v0.AnomalyInfo.Reason.Builder, org.tensorflow.metadata.v0.AnomalyInfo.ReasonOrBuilder>(
reason_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
reason_ = null;
}
return reasonBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.AnomalyInfo)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.AnomalyInfo)
private static final org.tensorflow.metadata.v0.AnomalyInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.AnomalyInfo();
}
public static org.tensorflow.metadata.v0.AnomalyInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AnomalyInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.AnomalyInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy