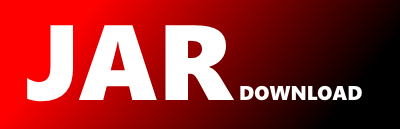
org.tensorflow.metadata.v0.BinaryClassification Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow_metadata/proto/v0/problem_statement.proto
// Protobuf Java Version: 3.25.4
package org.tensorflow.metadata.v0;
/**
*
* Configuration for a binary classification task.
* The output is one of two possible class labels, encoded as the same type
* as the label column.
* BinaryClassification is the same as MultiClassClassification with
* n_classes = 2.
*
*
* Protobuf type {@code tensorflow.metadata.v0.BinaryClassification}
*/
public final class BinaryClassification extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.BinaryClassification)
BinaryClassificationOrBuilder {
private static final long serialVersionUID = 0L;
// Use BinaryClassification.newBuilder() to construct.
private BinaryClassification(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BinaryClassification() {
exampleWeight_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new BinaryClassification();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.BinaryClassification.class, org.tensorflow.metadata.v0.BinaryClassification.Builder.class);
}
public interface PositiveNegativeSpecOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec)
com.google.protobuf.MessageOrBuilder {
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
* @return Whether the positiveClassValue field is set.
*/
boolean hasPositiveClassValue();
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
* @return The positiveClassValue.
*/
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getPositiveClassValue();
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder getPositiveClassValueOrBuilder();
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
* @return Whether the negativeClassValue field is set.
*/
boolean hasNegativeClassValue();
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
* @return The negativeClassValue.
*/
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getNegativeClassValue();
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder getNegativeClassValueOrBuilder();
}
/**
*
* Defines which label value is the positive and/or negative class.
*
*
* Protobuf type {@code tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec}
*/
public static final class PositiveNegativeSpec extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec)
PositiveNegativeSpecOrBuilder {
private static final long serialVersionUID = 0L;
// Use PositiveNegativeSpec.newBuilder() to construct.
private PositiveNegativeSpec(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PositiveNegativeSpec() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new PositiveNegativeSpec();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.class, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.Builder.class);
}
public interface LabelValueOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue)
com.google.protobuf.MessageOrBuilder {
/**
* string string_value = 1;
* @return Whether the stringValue field is set.
*/
boolean hasStringValue();
/**
* string string_value = 1;
* @return The stringValue.
*/
java.lang.String getStringValue();
/**
* string string_value = 1;
* @return The bytes for stringValue.
*/
com.google.protobuf.ByteString
getStringValueBytes();
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.ValueTypeCase getValueTypeCase();
}
/**
*
* Specifies a label's value which can be used for positive/negative class
* specification.
*
*
* Protobuf type {@code tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue}
*/
public static final class LabelValue extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue)
LabelValueOrBuilder {
private static final long serialVersionUID = 0L;
// Use LabelValue.newBuilder() to construct.
private LabelValue(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LabelValue() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LabelValue();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_LabelValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_LabelValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.class, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder.class);
}
private int valueTypeCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object valueType_;
public enum ValueTypeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
STRING_VALUE(1),
VALUETYPE_NOT_SET(0);
private final int value;
private ValueTypeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ValueTypeCase valueOf(int value) {
return forNumber(value);
}
public static ValueTypeCase forNumber(int value) {
switch (value) {
case 1: return STRING_VALUE;
case 0: return VALUETYPE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ValueTypeCase
getValueTypeCase() {
return ValueTypeCase.forNumber(
valueTypeCase_);
}
public static final int STRING_VALUE_FIELD_NUMBER = 1;
/**
* string string_value = 1;
* @return Whether the stringValue field is set.
*/
public boolean hasStringValue() {
return valueTypeCase_ == 1;
}
/**
* string string_value = 1;
* @return The stringValue.
*/
public java.lang.String getStringValue() {
java.lang.Object ref = "";
if (valueTypeCase_ == 1) {
ref = valueType_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueTypeCase_ == 1) {
valueType_ = s;
}
return s;
}
}
/**
* string string_value = 1;
* @return The bytes for stringValue.
*/
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = "";
if (valueTypeCase_ == 1) {
ref = valueType_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueTypeCase_ == 1) {
valueType_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (valueTypeCase_ == 1) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, valueType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (valueTypeCase_ == 1) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, valueType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue other = (org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue) obj;
if (!getValueTypeCase().equals(other.getValueTypeCase())) return false;
switch (valueTypeCase_) {
case 1:
if (!getStringValue()
.equals(other.getStringValue())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (valueTypeCase_) {
case 1:
hash = (37 * hash) + STRING_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getStringValue().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Specifies a label's value which can be used for positive/negative class
* specification.
*
*
* Protobuf type {@code tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue)
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_LabelValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_LabelValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.class, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
valueTypeCase_ = 0;
valueType_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_LabelValue_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue build() {
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue buildPartial() {
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue result = new org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue result) {
int from_bitField0_ = bitField0_;
}
private void buildPartialOneofs(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue result) {
result.valueTypeCase_ = valueTypeCase_;
result.valueType_ = this.valueType_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue) {
return mergeFrom((org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue other) {
if (other == org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance()) return this;
switch (other.getValueTypeCase()) {
case STRING_VALUE: {
valueTypeCase_ = 1;
valueType_ = other.valueType_;
onChanged();
break;
}
case VALUETYPE_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
valueTypeCase_ = 1;
valueType_ = s;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int valueTypeCase_ = 0;
private java.lang.Object valueType_;
public ValueTypeCase
getValueTypeCase() {
return ValueTypeCase.forNumber(
valueTypeCase_);
}
public Builder clearValueType() {
valueTypeCase_ = 0;
valueType_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
* string string_value = 1;
* @return Whether the stringValue field is set.
*/
@java.lang.Override
public boolean hasStringValue() {
return valueTypeCase_ == 1;
}
/**
* string string_value = 1;
* @return The stringValue.
*/
@java.lang.Override
public java.lang.String getStringValue() {
java.lang.Object ref = "";
if (valueTypeCase_ == 1) {
ref = valueType_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (valueTypeCase_ == 1) {
valueType_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string string_value = 1;
* @return The bytes for stringValue.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getStringValueBytes() {
java.lang.Object ref = "";
if (valueTypeCase_ == 1) {
ref = valueType_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (valueTypeCase_ == 1) {
valueType_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string string_value = 1;
* @param value The stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValue(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
valueTypeCase_ = 1;
valueType_ = value;
onChanged();
return this;
}
/**
* string string_value = 1;
* @return This builder for chaining.
*/
public Builder clearStringValue() {
if (valueTypeCase_ == 1) {
valueTypeCase_ = 0;
valueType_ = null;
onChanged();
}
return this;
}
/**
* string string_value = 1;
* @param value The bytes for stringValue to set.
* @return This builder for chaining.
*/
public Builder setStringValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
valueTypeCase_ = 1;
valueType_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue)
private static final org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue();
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LabelValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int POSITIVE_CLASS_VALUE_FIELD_NUMBER = 1;
private org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positiveClassValue_;
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
* @return Whether the positiveClassValue field is set.
*/
@java.lang.Override
public boolean hasPositiveClassValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
* @return The positiveClassValue.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getPositiveClassValue() {
return positiveClassValue_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : positiveClassValue_;
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder getPositiveClassValueOrBuilder() {
return positiveClassValue_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : positiveClassValue_;
}
public static final int NEGATIVE_CLASS_VALUE_FIELD_NUMBER = 2;
private org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negativeClassValue_;
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
* @return Whether the negativeClassValue field is set.
*/
@java.lang.Override
public boolean hasNegativeClassValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
* @return The negativeClassValue.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getNegativeClassValue() {
return negativeClassValue_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : negativeClassValue_;
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder getNegativeClassValueOrBuilder() {
return negativeClassValue_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : negativeClassValue_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getPositiveClassValue());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getNegativeClassValue());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getPositiveClassValue());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getNegativeClassValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec other = (org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec) obj;
if (hasPositiveClassValue() != other.hasPositiveClassValue()) return false;
if (hasPositiveClassValue()) {
if (!getPositiveClassValue()
.equals(other.getPositiveClassValue())) return false;
}
if (hasNegativeClassValue() != other.hasNegativeClassValue()) return false;
if (hasNegativeClassValue()) {
if (!getNegativeClassValue()
.equals(other.getNegativeClassValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasPositiveClassValue()) {
hash = (37 * hash) + POSITIVE_CLASS_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getPositiveClassValue().hashCode();
}
if (hasNegativeClassValue()) {
hash = (37 * hash) + NEGATIVE_CLASS_VALUE_FIELD_NUMBER;
hash = (53 * hash) + getNegativeClassValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Defines which label value is the positive and/or negative class.
*
*
* Protobuf type {@code tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec)
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpecOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.class, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPositiveClassValueFieldBuilder();
getNegativeClassValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
positiveClassValue_ = null;
if (positiveClassValueBuilder_ != null) {
positiveClassValueBuilder_.dispose();
positiveClassValueBuilder_ = null;
}
negativeClassValue_ = null;
if (negativeClassValueBuilder_ != null) {
negativeClassValueBuilder_.dispose();
negativeClassValueBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_PositiveNegativeSpec_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec build() {
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec buildPartial() {
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec result = new org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.positiveClassValue_ = positiveClassValueBuilder_ == null
? positiveClassValue_
: positiveClassValueBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.negativeClassValue_ = negativeClassValueBuilder_ == null
? negativeClassValue_
: negativeClassValueBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec) {
return mergeFrom((org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec other) {
if (other == org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.getDefaultInstance()) return this;
if (other.hasPositiveClassValue()) {
mergePositiveClassValue(other.getPositiveClassValue());
}
if (other.hasNegativeClassValue()) {
mergeNegativeClassValue(other.getNegativeClassValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getPositiveClassValueFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getNegativeClassValueFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positiveClassValue_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder> positiveClassValueBuilder_;
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
* @return Whether the positiveClassValue field is set.
*/
public boolean hasPositiveClassValue() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
* @return The positiveClassValue.
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getPositiveClassValue() {
if (positiveClassValueBuilder_ == null) {
return positiveClassValue_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : positiveClassValue_;
} else {
return positiveClassValueBuilder_.getMessage();
}
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
public Builder setPositiveClassValue(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue value) {
if (positiveClassValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
positiveClassValue_ = value;
} else {
positiveClassValueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
public Builder setPositiveClassValue(
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder builderForValue) {
if (positiveClassValueBuilder_ == null) {
positiveClassValue_ = builderForValue.build();
} else {
positiveClassValueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
public Builder mergePositiveClassValue(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue value) {
if (positiveClassValueBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
positiveClassValue_ != null &&
positiveClassValue_ != org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance()) {
getPositiveClassValueBuilder().mergeFrom(value);
} else {
positiveClassValue_ = value;
}
} else {
positiveClassValueBuilder_.mergeFrom(value);
}
if (positiveClassValue_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
public Builder clearPositiveClassValue() {
bitField0_ = (bitField0_ & ~0x00000001);
positiveClassValue_ = null;
if (positiveClassValueBuilder_ != null) {
positiveClassValueBuilder_.dispose();
positiveClassValueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder getPositiveClassValueBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getPositiveClassValueFieldBuilder().getBuilder();
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder getPositiveClassValueOrBuilder() {
if (positiveClassValueBuilder_ != null) {
return positiveClassValueBuilder_.getMessageOrBuilder();
} else {
return positiveClassValue_ == null ?
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : positiveClassValue_;
}
}
/**
*
* This value is the positive class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue positive_class_value = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder>
getPositiveClassValueFieldBuilder() {
if (positiveClassValueBuilder_ == null) {
positiveClassValueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder>(
getPositiveClassValue(),
getParentForChildren(),
isClean());
positiveClassValue_ = null;
}
return positiveClassValueBuilder_;
}
private org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negativeClassValue_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder> negativeClassValueBuilder_;
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
* @return Whether the negativeClassValue field is set.
*/
public boolean hasNegativeClassValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
* @return The negativeClassValue.
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue getNegativeClassValue() {
if (negativeClassValueBuilder_ == null) {
return negativeClassValue_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : negativeClassValue_;
} else {
return negativeClassValueBuilder_.getMessage();
}
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
public Builder setNegativeClassValue(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue value) {
if (negativeClassValueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
negativeClassValue_ = value;
} else {
negativeClassValueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
public Builder setNegativeClassValue(
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder builderForValue) {
if (negativeClassValueBuilder_ == null) {
negativeClassValue_ = builderForValue.build();
} else {
negativeClassValueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
public Builder mergeNegativeClassValue(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue value) {
if (negativeClassValueBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
negativeClassValue_ != null &&
negativeClassValue_ != org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance()) {
getNegativeClassValueBuilder().mergeFrom(value);
} else {
negativeClassValue_ = value;
}
} else {
negativeClassValueBuilder_.mergeFrom(value);
}
if (negativeClassValue_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
public Builder clearNegativeClassValue() {
bitField0_ = (bitField0_ & ~0x00000002);
negativeClassValue_ = null;
if (negativeClassValueBuilder_ != null) {
negativeClassValueBuilder_.dispose();
negativeClassValueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder getNegativeClassValueBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getNegativeClassValueFieldBuilder().getBuilder();
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder getNegativeClassValueOrBuilder() {
if (negativeClassValueBuilder_ != null) {
return negativeClassValueBuilder_.getMessageOrBuilder();
} else {
return negativeClassValue_ == null ?
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.getDefaultInstance() : negativeClassValue_;
}
}
/**
*
* This value is the negative class.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue negative_class_value = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder>
getNegativeClassValueFieldBuilder() {
if (negativeClassValueBuilder_ == null) {
negativeClassValueBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValue.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.LabelValueOrBuilder>(
getNegativeClassValue(),
getParentForChildren(),
isClean());
negativeClassValue_ = null;
}
return negativeClassValueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec)
private static final org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec();
}
public static org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PositiveNegativeSpec parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int labelIdCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object labelId_;
public enum LabelIdCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
LABEL(1),
LABEL_PATH(3),
LABELID_NOT_SET(0);
private final int value;
private LabelIdCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LabelIdCase valueOf(int value) {
return forNumber(value);
}
public static LabelIdCase forNumber(int value) {
switch (value) {
case 1: return LABEL;
case 3: return LABEL_PATH;
case 0: return LABELID_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public LabelIdCase
getLabelIdCase() {
return LabelIdCase.forNumber(
labelIdCase_);
}
public static final int LABEL_FIELD_NUMBER = 1;
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @return Whether the label field is set.
*/
public boolean hasLabel() {
return labelIdCase_ == 1;
}
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @return The label.
*/
public java.lang.String getLabel() {
java.lang.Object ref = "";
if (labelIdCase_ == 1) {
ref = labelId_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (labelIdCase_ == 1) {
labelId_ = s;
}
return s;
}
}
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @return The bytes for label.
*/
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = "";
if (labelIdCase_ == 1) {
ref = labelId_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (labelIdCase_ == 1) {
labelId_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LABEL_PATH_FIELD_NUMBER = 3;
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
* @return Whether the labelPath field is set.
*/
@java.lang.Override
public boolean hasLabelPath() {
return labelIdCase_ == 3;
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
* @return The labelPath.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.Path getLabelPath() {
if (labelIdCase_ == 3) {
return (org.tensorflow.metadata.v0.Path) labelId_;
}
return org.tensorflow.metadata.v0.Path.getDefaultInstance();
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.PathOrBuilder getLabelPathOrBuilder() {
if (labelIdCase_ == 3) {
return (org.tensorflow.metadata.v0.Path) labelId_;
}
return org.tensorflow.metadata.v0.Path.getDefaultInstance();
}
public static final int EXAMPLE_WEIGHT_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object exampleWeight_ = "";
/**
*
* (optional) The weight column.
*
*
* string example_weight = 2;
* @return The exampleWeight.
*/
@java.lang.Override
public java.lang.String getExampleWeight() {
java.lang.Object ref = exampleWeight_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exampleWeight_ = s;
return s;
}
}
/**
*
* (optional) The weight column.
*
*
* string example_weight = 2;
* @return The bytes for exampleWeight.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExampleWeightBytes() {
java.lang.Object ref = exampleWeight_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exampleWeight_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POSITIVE_NEGATIVE_SPEC_FIELD_NUMBER = 4;
private org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positiveNegativeSpec_;
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
* @return Whether the positiveNegativeSpec field is set.
*/
@java.lang.Override
public boolean hasPositiveNegativeSpec() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
* @return The positiveNegativeSpec.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec getPositiveNegativeSpec() {
return positiveNegativeSpec_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.getDefaultInstance() : positiveNegativeSpec_;
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpecOrBuilder getPositiveNegativeSpecOrBuilder() {
return positiveNegativeSpec_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.getDefaultInstance() : positiveNegativeSpec_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (labelIdCase_ == 1) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, labelId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(exampleWeight_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, exampleWeight_);
}
if (labelIdCase_ == 3) {
output.writeMessage(3, (org.tensorflow.metadata.v0.Path) labelId_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(4, getPositiveNegativeSpec());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (labelIdCase_ == 1) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, labelId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(exampleWeight_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, exampleWeight_);
}
if (labelIdCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (org.tensorflow.metadata.v0.Path) labelId_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPositiveNegativeSpec());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.BinaryClassification)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.BinaryClassification other = (org.tensorflow.metadata.v0.BinaryClassification) obj;
if (!getExampleWeight()
.equals(other.getExampleWeight())) return false;
if (hasPositiveNegativeSpec() != other.hasPositiveNegativeSpec()) return false;
if (hasPositiveNegativeSpec()) {
if (!getPositiveNegativeSpec()
.equals(other.getPositiveNegativeSpec())) return false;
}
if (!getLabelIdCase().equals(other.getLabelIdCase())) return false;
switch (labelIdCase_) {
case 1:
if (!getLabel()
.equals(other.getLabel())) return false;
break;
case 3:
if (!getLabelPath()
.equals(other.getLabelPath())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + EXAMPLE_WEIGHT_FIELD_NUMBER;
hash = (53 * hash) + getExampleWeight().hashCode();
if (hasPositiveNegativeSpec()) {
hash = (37 * hash) + POSITIVE_NEGATIVE_SPEC_FIELD_NUMBER;
hash = (53 * hash) + getPositiveNegativeSpec().hashCode();
}
switch (labelIdCase_) {
case 1:
hash = (37 * hash) + LABEL_FIELD_NUMBER;
hash = (53 * hash) + getLabel().hashCode();
break;
case 3:
hash = (37 * hash) + LABEL_PATH_FIELD_NUMBER;
hash = (53 * hash) + getLabelPath().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.BinaryClassification parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.BinaryClassification prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Configuration for a binary classification task.
* The output is one of two possible class labels, encoded as the same type
* as the label column.
* BinaryClassification is the same as MultiClassClassification with
* n_classes = 2.
*
*
* Protobuf type {@code tensorflow.metadata.v0.BinaryClassification}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.BinaryClassification)
org.tensorflow.metadata.v0.BinaryClassificationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.BinaryClassification.class, org.tensorflow.metadata.v0.BinaryClassification.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.BinaryClassification.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPositiveNegativeSpecFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (labelPathBuilder_ != null) {
labelPathBuilder_.clear();
}
exampleWeight_ = "";
positiveNegativeSpec_ = null;
if (positiveNegativeSpecBuilder_ != null) {
positiveNegativeSpecBuilder_.dispose();
positiveNegativeSpecBuilder_ = null;
}
labelIdCase_ = 0;
labelId_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.ProblemStatementOuterClass.internal_static_tensorflow_metadata_v0_BinaryClassification_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.BinaryClassification.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification build() {
org.tensorflow.metadata.v0.BinaryClassification result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification buildPartial() {
org.tensorflow.metadata.v0.BinaryClassification result = new org.tensorflow.metadata.v0.BinaryClassification(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(org.tensorflow.metadata.v0.BinaryClassification result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.exampleWeight_ = exampleWeight_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.positiveNegativeSpec_ = positiveNegativeSpecBuilder_ == null
? positiveNegativeSpec_
: positiveNegativeSpecBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(org.tensorflow.metadata.v0.BinaryClassification result) {
result.labelIdCase_ = labelIdCase_;
result.labelId_ = this.labelId_;
if (labelIdCase_ == 3 &&
labelPathBuilder_ != null) {
result.labelId_ = labelPathBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.BinaryClassification) {
return mergeFrom((org.tensorflow.metadata.v0.BinaryClassification)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.BinaryClassification other) {
if (other == org.tensorflow.metadata.v0.BinaryClassification.getDefaultInstance()) return this;
if (!other.getExampleWeight().isEmpty()) {
exampleWeight_ = other.exampleWeight_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasPositiveNegativeSpec()) {
mergePositiveNegativeSpec(other.getPositiveNegativeSpec());
}
switch (other.getLabelIdCase()) {
case LABEL: {
labelIdCase_ = 1;
labelId_ = other.labelId_;
onChanged();
break;
}
case LABEL_PATH: {
mergeLabelPath(other.getLabelPath());
break;
}
case LABELID_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
labelIdCase_ = 1;
labelId_ = s;
break;
} // case 10
case 18: {
exampleWeight_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 18
case 26: {
input.readMessage(
getLabelPathFieldBuilder().getBuilder(),
extensionRegistry);
labelIdCase_ = 3;
break;
} // case 26
case 34: {
input.readMessage(
getPositiveNegativeSpecFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int labelIdCase_ = 0;
private java.lang.Object labelId_;
public LabelIdCase
getLabelIdCase() {
return LabelIdCase.forNumber(
labelIdCase_);
}
public Builder clearLabelId() {
labelIdCase_ = 0;
labelId_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @return Whether the label field is set.
*/
@java.lang.Override
public boolean hasLabel() {
return labelIdCase_ == 1;
}
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @return The label.
*/
@java.lang.Override
public java.lang.String getLabel() {
java.lang.Object ref = "";
if (labelIdCase_ == 1) {
ref = labelId_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (labelIdCase_ == 1) {
labelId_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @return The bytes for label.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = "";
if (labelIdCase_ == 1) {
ref = labelId_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (labelIdCase_ == 1) {
labelId_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @param value The label to set.
* @return This builder for chaining.
*/
public Builder setLabel(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
labelIdCase_ = 1;
labelId_ = value;
onChanged();
return this;
}
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @return This builder for chaining.
*/
public Builder clearLabel() {
if (labelIdCase_ == 1) {
labelIdCase_ = 0;
labelId_ = null;
onChanged();
}
return this;
}
/**
*
* The name of the label. Assumes the label is a flat, top-level field.
*
*
* string label = 1;
* @param value The bytes for label to set.
* @return This builder for chaining.
*/
public Builder setLabelBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
labelIdCase_ = 1;
labelId_ = value;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Path, org.tensorflow.metadata.v0.Path.Builder, org.tensorflow.metadata.v0.PathOrBuilder> labelPathBuilder_;
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
* @return Whether the labelPath field is set.
*/
@java.lang.Override
public boolean hasLabelPath() {
return labelIdCase_ == 3;
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
* @return The labelPath.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.Path getLabelPath() {
if (labelPathBuilder_ == null) {
if (labelIdCase_ == 3) {
return (org.tensorflow.metadata.v0.Path) labelId_;
}
return org.tensorflow.metadata.v0.Path.getDefaultInstance();
} else {
if (labelIdCase_ == 3) {
return labelPathBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.Path.getDefaultInstance();
}
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
public Builder setLabelPath(org.tensorflow.metadata.v0.Path value) {
if (labelPathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
labelId_ = value;
onChanged();
} else {
labelPathBuilder_.setMessage(value);
}
labelIdCase_ = 3;
return this;
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
public Builder setLabelPath(
org.tensorflow.metadata.v0.Path.Builder builderForValue) {
if (labelPathBuilder_ == null) {
labelId_ = builderForValue.build();
onChanged();
} else {
labelPathBuilder_.setMessage(builderForValue.build());
}
labelIdCase_ = 3;
return this;
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
public Builder mergeLabelPath(org.tensorflow.metadata.v0.Path value) {
if (labelPathBuilder_ == null) {
if (labelIdCase_ == 3 &&
labelId_ != org.tensorflow.metadata.v0.Path.getDefaultInstance()) {
labelId_ = org.tensorflow.metadata.v0.Path.newBuilder((org.tensorflow.metadata.v0.Path) labelId_)
.mergeFrom(value).buildPartial();
} else {
labelId_ = value;
}
onChanged();
} else {
if (labelIdCase_ == 3) {
labelPathBuilder_.mergeFrom(value);
} else {
labelPathBuilder_.setMessage(value);
}
}
labelIdCase_ = 3;
return this;
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
public Builder clearLabelPath() {
if (labelPathBuilder_ == null) {
if (labelIdCase_ == 3) {
labelIdCase_ = 0;
labelId_ = null;
onChanged();
}
} else {
if (labelIdCase_ == 3) {
labelIdCase_ = 0;
labelId_ = null;
}
labelPathBuilder_.clear();
}
return this;
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
public org.tensorflow.metadata.v0.Path.Builder getLabelPathBuilder() {
return getLabelPathFieldBuilder().getBuilder();
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.PathOrBuilder getLabelPathOrBuilder() {
if ((labelIdCase_ == 3) && (labelPathBuilder_ != null)) {
return labelPathBuilder_.getMessageOrBuilder();
} else {
if (labelIdCase_ == 3) {
return (org.tensorflow.metadata.v0.Path) labelId_;
}
return org.tensorflow.metadata.v0.Path.getDefaultInstance();
}
}
/**
*
* A path can be used instead of a flat string if the label is nested.
*
*
* .tensorflow.metadata.v0.Path label_path = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Path, org.tensorflow.metadata.v0.Path.Builder, org.tensorflow.metadata.v0.PathOrBuilder>
getLabelPathFieldBuilder() {
if (labelPathBuilder_ == null) {
if (!(labelIdCase_ == 3)) {
labelId_ = org.tensorflow.metadata.v0.Path.getDefaultInstance();
}
labelPathBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Path, org.tensorflow.metadata.v0.Path.Builder, org.tensorflow.metadata.v0.PathOrBuilder>(
(org.tensorflow.metadata.v0.Path) labelId_,
getParentForChildren(),
isClean());
labelId_ = null;
}
labelIdCase_ = 3;
onChanged();
return labelPathBuilder_;
}
private java.lang.Object exampleWeight_ = "";
/**
*
* (optional) The weight column.
*
*
* string example_weight = 2;
* @return The exampleWeight.
*/
public java.lang.String getExampleWeight() {
java.lang.Object ref = exampleWeight_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exampleWeight_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* (optional) The weight column.
*
*
* string example_weight = 2;
* @return The bytes for exampleWeight.
*/
public com.google.protobuf.ByteString
getExampleWeightBytes() {
java.lang.Object ref = exampleWeight_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exampleWeight_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* (optional) The weight column.
*
*
* string example_weight = 2;
* @param value The exampleWeight to set.
* @return This builder for chaining.
*/
public Builder setExampleWeight(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
exampleWeight_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* (optional) The weight column.
*
*
* string example_weight = 2;
* @return This builder for chaining.
*/
public Builder clearExampleWeight() {
exampleWeight_ = getDefaultInstance().getExampleWeight();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* (optional) The weight column.
*
*
* string example_weight = 2;
* @param value The bytes for exampleWeight to set.
* @return This builder for chaining.
*/
public Builder setExampleWeightBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
exampleWeight_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positiveNegativeSpec_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpecOrBuilder> positiveNegativeSpecBuilder_;
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
* @return Whether the positiveNegativeSpec field is set.
*/
public boolean hasPositiveNegativeSpec() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
* @return The positiveNegativeSpec.
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec getPositiveNegativeSpec() {
if (positiveNegativeSpecBuilder_ == null) {
return positiveNegativeSpec_ == null ? org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.getDefaultInstance() : positiveNegativeSpec_;
} else {
return positiveNegativeSpecBuilder_.getMessage();
}
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
public Builder setPositiveNegativeSpec(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec value) {
if (positiveNegativeSpecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
positiveNegativeSpec_ = value;
} else {
positiveNegativeSpecBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
public Builder setPositiveNegativeSpec(
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.Builder builderForValue) {
if (positiveNegativeSpecBuilder_ == null) {
positiveNegativeSpec_ = builderForValue.build();
} else {
positiveNegativeSpecBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
public Builder mergePositiveNegativeSpec(org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec value) {
if (positiveNegativeSpecBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
positiveNegativeSpec_ != null &&
positiveNegativeSpec_ != org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.getDefaultInstance()) {
getPositiveNegativeSpecBuilder().mergeFrom(value);
} else {
positiveNegativeSpec_ = value;
}
} else {
positiveNegativeSpecBuilder_.mergeFrom(value);
}
if (positiveNegativeSpec_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
public Builder clearPositiveNegativeSpec() {
bitField0_ = (bitField0_ & ~0x00000008);
positiveNegativeSpec_ = null;
if (positiveNegativeSpecBuilder_ != null) {
positiveNegativeSpecBuilder_.dispose();
positiveNegativeSpecBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.Builder getPositiveNegativeSpecBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getPositiveNegativeSpecFieldBuilder().getBuilder();
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
public org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpecOrBuilder getPositiveNegativeSpecOrBuilder() {
if (positiveNegativeSpecBuilder_ != null) {
return positiveNegativeSpecBuilder_.getMessageOrBuilder();
} else {
return positiveNegativeSpec_ == null ?
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.getDefaultInstance() : positiveNegativeSpec_;
}
}
/**
*
* (optional) specification of the positive and/or negative class value.
*
*
* .tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec positive_negative_spec = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpecOrBuilder>
getPositiveNegativeSpecFieldBuilder() {
if (positiveNegativeSpecBuilder_ == null) {
positiveNegativeSpecBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpec.Builder, org.tensorflow.metadata.v0.BinaryClassification.PositiveNegativeSpecOrBuilder>(
getPositiveNegativeSpec(),
getParentForChildren(),
isClean());
positiveNegativeSpec_ = null;
}
return positiveNegativeSpecBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.BinaryClassification)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.BinaryClassification)
private static final org.tensorflow.metadata.v0.BinaryClassification DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.BinaryClassification();
}
public static org.tensorflow.metadata.v0.BinaryClassification getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BinaryClassification parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.BinaryClassification getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy