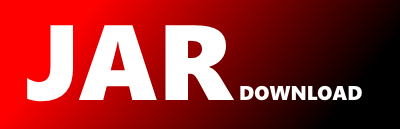
org.tensorflow.metadata.v0.CommonStatisticsOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow_metadata/proto/v0/statistics.proto
// Protobuf Java Version: 3.25.4
package org.tensorflow.metadata.v0;
public interface CommonStatisticsOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.metadata.v0.CommonStatistics)
com.google.protobuf.MessageOrBuilder {
/**
*
* The number of examples that include this feature. Note that this includes
* examples that contain this feature with an explicitly empty list of values,
* which may be permitted for variable length features.
*
*
* uint64 num_non_missing = 1;
* @return The numNonMissing.
*/
long getNumNonMissing();
/**
*
* The number of examples missing this feature.
*
*
* uint64 num_missing = 2;
* @return The numMissing.
*/
long getNumMissing();
/**
*
* The minimum number of values in a single example for this feature.
*
*
* uint64 min_num_values = 3;
* @return The minNumValues.
*/
long getMinNumValues();
/**
*
* The maximum number of values in a single example for this feature.
*
*
* uint64 max_num_values = 4;
* @return The maxNumValues.
*/
long getMaxNumValues();
/**
*
* The average number of values in a single example for this feature.
*
*
* float avg_num_values = 5;
* @return The avgNumValues.
*/
float getAvgNumValues();
/**
*
* tot_num_values = avg_num_values * num_non_missing.
* This is calculated directly, so should have less numerical error.
*
*
* uint64 tot_num_values = 8;
* @return The totNumValues.
*/
long getTotNumValues();
/**
*
* The quantiles histogram for the number of values in this feature.
*
*
* .tensorflow.metadata.v0.Histogram num_values_histogram = 6;
* @return Whether the numValuesHistogram field is set.
*/
boolean hasNumValuesHistogram();
/**
*
* The quantiles histogram for the number of values in this feature.
*
*
* .tensorflow.metadata.v0.Histogram num_values_histogram = 6;
* @return The numValuesHistogram.
*/
org.tensorflow.metadata.v0.Histogram getNumValuesHistogram();
/**
*
* The quantiles histogram for the number of values in this feature.
*
*
* .tensorflow.metadata.v0.Histogram num_values_histogram = 6;
*/
org.tensorflow.metadata.v0.HistogramOrBuilder getNumValuesHistogramOrBuilder();
/**
* .tensorflow.metadata.v0.WeightedCommonStatistics weighted_common_stats = 7;
* @return Whether the weightedCommonStats field is set.
*/
boolean hasWeightedCommonStats();
/**
* .tensorflow.metadata.v0.WeightedCommonStatistics weighted_common_stats = 7;
* @return The weightedCommonStats.
*/
org.tensorflow.metadata.v0.WeightedCommonStatistics getWeightedCommonStats();
/**
* .tensorflow.metadata.v0.WeightedCommonStatistics weighted_common_stats = 7;
*/
org.tensorflow.metadata.v0.WeightedCommonStatisticsOrBuilder getWeightedCommonStatsOrBuilder();
/**
*
* The histogram for the number of features in the feature list (only set if
* this feature is a non-context feature from a tf.SequenceExample).
* This is different from num_values_histogram, as num_values_histogram tracks
* the count of all values for a feature in an example, whereas this tracks
* the length of the feature list for this feature in an example (where each
* feature list can contain multiple values).
*
*
* .tensorflow.metadata.v0.Histogram feature_list_length_histogram = 9;
* @return Whether the featureListLengthHistogram field is set.
*/
boolean hasFeatureListLengthHistogram();
/**
*
* The histogram for the number of features in the feature list (only set if
* this feature is a non-context feature from a tf.SequenceExample).
* This is different from num_values_histogram, as num_values_histogram tracks
* the count of all values for a feature in an example, whereas this tracks
* the length of the feature list for this feature in an example (where each
* feature list can contain multiple values).
*
*
* .tensorflow.metadata.v0.Histogram feature_list_length_histogram = 9;
* @return The featureListLengthHistogram.
*/
org.tensorflow.metadata.v0.Histogram getFeatureListLengthHistogram();
/**
*
* The histogram for the number of features in the feature list (only set if
* this feature is a non-context feature from a tf.SequenceExample).
* This is different from num_values_histogram, as num_values_histogram tracks
* the count of all values for a feature in an example, whereas this tracks
* the length of the feature list for this feature in an example (where each
* feature list can contain multiple values).
*
*
* .tensorflow.metadata.v0.Histogram feature_list_length_histogram = 9;
*/
org.tensorflow.metadata.v0.HistogramOrBuilder getFeatureListLengthHistogramOrBuilder();
/**
*
* Contains presence and valency stats for each nest level of the feature.
* The first item corresponds to the outermost level, and by definition,
* the stats it contains equals to the corresponding stats defined above.
* May not be populated if the feature is of nest level 1.
*
*
* repeated .tensorflow.metadata.v0.PresenceAndValencyStatistics presence_and_valency_stats = 10;
*/
java.util.List
getPresenceAndValencyStatsList();
/**
*
* Contains presence and valency stats for each nest level of the feature.
* The first item corresponds to the outermost level, and by definition,
* the stats it contains equals to the corresponding stats defined above.
* May not be populated if the feature is of nest level 1.
*
*
* repeated .tensorflow.metadata.v0.PresenceAndValencyStatistics presence_and_valency_stats = 10;
*/
org.tensorflow.metadata.v0.PresenceAndValencyStatistics getPresenceAndValencyStats(int index);
/**
*
* Contains presence and valency stats for each nest level of the feature.
* The first item corresponds to the outermost level, and by definition,
* the stats it contains equals to the corresponding stats defined above.
* May not be populated if the feature is of nest level 1.
*
*
* repeated .tensorflow.metadata.v0.PresenceAndValencyStatistics presence_and_valency_stats = 10;
*/
int getPresenceAndValencyStatsCount();
/**
*
* Contains presence and valency stats for each nest level of the feature.
* The first item corresponds to the outermost level, and by definition,
* the stats it contains equals to the corresponding stats defined above.
* May not be populated if the feature is of nest level 1.
*
*
* repeated .tensorflow.metadata.v0.PresenceAndValencyStatistics presence_and_valency_stats = 10;
*/
java.util.List extends org.tensorflow.metadata.v0.PresenceAndValencyStatisticsOrBuilder>
getPresenceAndValencyStatsOrBuilderList();
/**
*
* Contains presence and valency stats for each nest level of the feature.
* The first item corresponds to the outermost level, and by definition,
* the stats it contains equals to the corresponding stats defined above.
* May not be populated if the feature is of nest level 1.
*
*
* repeated .tensorflow.metadata.v0.PresenceAndValencyStatistics presence_and_valency_stats = 10;
*/
org.tensorflow.metadata.v0.PresenceAndValencyStatisticsOrBuilder getPresenceAndValencyStatsOrBuilder(
int index);
/**
*
* If not empty, it's parallel to presence_and_valency_stats.
*
*
* repeated .tensorflow.metadata.v0.WeightedCommonStatistics weighted_presence_and_valency_stats = 11;
*/
java.util.List
getWeightedPresenceAndValencyStatsList();
/**
*
* If not empty, it's parallel to presence_and_valency_stats.
*
*
* repeated .tensorflow.metadata.v0.WeightedCommonStatistics weighted_presence_and_valency_stats = 11;
*/
org.tensorflow.metadata.v0.WeightedCommonStatistics getWeightedPresenceAndValencyStats(int index);
/**
*
* If not empty, it's parallel to presence_and_valency_stats.
*
*
* repeated .tensorflow.metadata.v0.WeightedCommonStatistics weighted_presence_and_valency_stats = 11;
*/
int getWeightedPresenceAndValencyStatsCount();
/**
*
* If not empty, it's parallel to presence_and_valency_stats.
*
*
* repeated .tensorflow.metadata.v0.WeightedCommonStatistics weighted_presence_and_valency_stats = 11;
*/
java.util.List extends org.tensorflow.metadata.v0.WeightedCommonStatisticsOrBuilder>
getWeightedPresenceAndValencyStatsOrBuilderList();
/**
*
* If not empty, it's parallel to presence_and_valency_stats.
*
*
* repeated .tensorflow.metadata.v0.WeightedCommonStatistics weighted_presence_and_valency_stats = 11;
*/
org.tensorflow.metadata.v0.WeightedCommonStatisticsOrBuilder getWeightedPresenceAndValencyStatsOrBuilder(
int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy