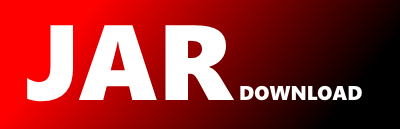
org.tensorflow.metadata.v0.Feature Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow_metadata/proto/v0/schema.proto
// Protobuf Java Version: 3.25.4
package org.tensorflow.metadata.v0;
/**
*
* Describes schema-level information about a specific feature.
* NextID: 36
*
*
* Protobuf type {@code tensorflow.metadata.v0.Feature}
*/
public final class Feature extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.Feature)
FeatureOrBuilder {
private static final long serialVersionUID = 0L;
// Use Feature.newBuilder() to construct.
private Feature(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Feature() {
name_ = "";
type_ = 0;
inEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
notInEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
lifecycleStage_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Feature();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_Feature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_Feature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.Feature.class, org.tensorflow.metadata.v0.Feature.Builder.class);
}
private int bitField0_;
private int presenceConstraintsCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object presenceConstraints_;
public enum PresenceConstraintsCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PRESENCE(14),
GROUP_PRESENCE(17),
PRESENCECONSTRAINTS_NOT_SET(0);
private final int value;
private PresenceConstraintsCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PresenceConstraintsCase valueOf(int value) {
return forNumber(value);
}
public static PresenceConstraintsCase forNumber(int value) {
switch (value) {
case 14: return PRESENCE;
case 17: return GROUP_PRESENCE;
case 0: return PRESENCECONSTRAINTS_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public PresenceConstraintsCase
getPresenceConstraintsCase() {
return PresenceConstraintsCase.forNumber(
presenceConstraintsCase_);
}
private int shapeTypeCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object shapeType_;
public enum ShapeTypeCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
SHAPE(23),
VALUE_COUNT(5),
VALUE_COUNTS(32),
SHAPETYPE_NOT_SET(0);
private final int value;
private ShapeTypeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ShapeTypeCase valueOf(int value) {
return forNumber(value);
}
public static ShapeTypeCase forNumber(int value) {
switch (value) {
case 23: return SHAPE;
case 5: return VALUE_COUNT;
case 32: return VALUE_COUNTS;
case 0: return SHAPETYPE_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ShapeTypeCase
getShapeTypeCase() {
return ShapeTypeCase.forNumber(
shapeTypeCase_);
}
private int domainInfoCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object domainInfo_;
public enum DomainInfoCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
DOMAIN(7),
INT_DOMAIN(9),
FLOAT_DOMAIN(10),
STRING_DOMAIN(11),
BOOL_DOMAIN(13),
STRUCT_DOMAIN(29),
NATURAL_LANGUAGE_DOMAIN(24),
IMAGE_DOMAIN(25),
MID_DOMAIN(26),
URL_DOMAIN(27),
TIME_DOMAIN(28),
TIME_OF_DAY_DOMAIN(30),
DOMAININFO_NOT_SET(0);
private final int value;
private DomainInfoCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DomainInfoCase valueOf(int value) {
return forNumber(value);
}
public static DomainInfoCase forNumber(int value) {
switch (value) {
case 7: return DOMAIN;
case 9: return INT_DOMAIN;
case 10: return FLOAT_DOMAIN;
case 11: return STRING_DOMAIN;
case 13: return BOOL_DOMAIN;
case 29: return STRUCT_DOMAIN;
case 24: return NATURAL_LANGUAGE_DOMAIN;
case 25: return IMAGE_DOMAIN;
case 26: return MID_DOMAIN;
case 27: return URL_DOMAIN;
case 28: return TIME_DOMAIN;
case 30: return TIME_OF_DAY_DOMAIN;
case 0: return DOMAININFO_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public DomainInfoCase
getDomainInfoCase() {
return DomainInfoCase.forNumber(
domainInfoCase_);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEPRECATED_FIELD_NUMBER = 2;
private boolean deprecated_ = false;
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.Feature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=153
* @return Whether the deprecated field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasDeprecated() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.Feature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=153
* @return The deprecated.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getDeprecated() {
return deprecated_;
}
public static final int PRESENCE_FIELD_NUMBER = 14;
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
* @return Whether the presence field is set.
*/
@java.lang.Override
public boolean hasPresence() {
return presenceConstraintsCase_ == 14;
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
* @return The presence.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresence getPresence() {
if (presenceConstraintsCase_ == 14) {
return (org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance();
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresenceOrBuilder getPresenceOrBuilder() {
if (presenceConstraintsCase_ == 14) {
return (org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance();
}
public static final int GROUP_PRESENCE_FIELD_NUMBER = 17;
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
* @return Whether the groupPresence field is set.
*/
@java.lang.Override
public boolean hasGroupPresence() {
return presenceConstraintsCase_ == 17;
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
* @return The groupPresence.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresenceWithinGroup getGroupPresence() {
if (presenceConstraintsCase_ == 17) {
return (org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.getDefaultInstance();
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresenceWithinGroupOrBuilder getGroupPresenceOrBuilder() {
if (presenceConstraintsCase_ == 17) {
return (org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.getDefaultInstance();
}
public static final int SHAPE_FIELD_NUMBER = 23;
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
* @return Whether the shape field is set.
*/
@java.lang.Override
public boolean hasShape() {
return shapeTypeCase_ == 23;
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
* @return The shape.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FixedShape getShape() {
if (shapeTypeCase_ == 23) {
return (org.tensorflow.metadata.v0.FixedShape) shapeType_;
}
return org.tensorflow.metadata.v0.FixedShape.getDefaultInstance();
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FixedShapeOrBuilder getShapeOrBuilder() {
if (shapeTypeCase_ == 23) {
return (org.tensorflow.metadata.v0.FixedShape) shapeType_;
}
return org.tensorflow.metadata.v0.FixedShape.getDefaultInstance();
}
public static final int VALUE_COUNT_FIELD_NUMBER = 5;
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
* @return Whether the valueCount field is set.
*/
@java.lang.Override
public boolean hasValueCount() {
return shapeTypeCase_ == 5;
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
* @return The valueCount.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCount getValueCount() {
if (shapeTypeCase_ == 5) {
return (org.tensorflow.metadata.v0.ValueCount) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCount.getDefaultInstance();
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCountOrBuilder getValueCountOrBuilder() {
if (shapeTypeCase_ == 5) {
return (org.tensorflow.metadata.v0.ValueCount) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCount.getDefaultInstance();
}
public static final int VALUE_COUNTS_FIELD_NUMBER = 32;
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
* @return Whether the valueCounts field is set.
*/
@java.lang.Override
public boolean hasValueCounts() {
return shapeTypeCase_ == 32;
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
* @return The valueCounts.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCountList getValueCounts() {
if (shapeTypeCase_ == 32) {
return (org.tensorflow.metadata.v0.ValueCountList) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCountList.getDefaultInstance();
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCountListOrBuilder getValueCountsOrBuilder() {
if (shapeTypeCase_ == 32) {
return (org.tensorflow.metadata.v0.ValueCountList) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCountList.getDefaultInstance();
}
public static final int TYPE_FIELD_NUMBER = 6;
private int type_ = 0;
/**
*
* Physical type of the feature's values.
* Note that you can have:
* type: BYTES
* int_domain: {
* min: 0
* max: 3
* }
* This would be a field that is syntactically BYTES (i.e. strings), but
* semantically an int, i.e. it would be "0", "1", "2", or "3".
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 6;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Physical type of the feature's values.
* Note that you can have:
* type: BYTES
* int_domain: {
* min: 0
* max: 3
* }
* This would be a field that is syntactically BYTES (i.e. strings), but
* semantically an int, i.e. it would be "0", "1", "2", or "3".
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 6;
* @return The type.
*/
@java.lang.Override public org.tensorflow.metadata.v0.FeatureType getType() {
org.tensorflow.metadata.v0.FeatureType result = org.tensorflow.metadata.v0.FeatureType.forNumber(type_);
return result == null ? org.tensorflow.metadata.v0.FeatureType.TYPE_UNKNOWN : result;
}
public static final int DOMAIN_FIELD_NUMBER = 7;
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @return Whether the domain field is set.
*/
public boolean hasDomain() {
return domainInfoCase_ == 7;
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @return The domain.
*/
public java.lang.String getDomain() {
java.lang.Object ref = "";
if (domainInfoCase_ == 7) {
ref = domainInfo_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8() && (domainInfoCase_ == 7)) {
domainInfo_ = s;
}
return s;
}
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @return The bytes for domain.
*/
public com.google.protobuf.ByteString
getDomainBytes() {
java.lang.Object ref = "";
if (domainInfoCase_ == 7) {
ref = domainInfo_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (domainInfoCase_ == 7) {
domainInfo_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INT_DOMAIN_FIELD_NUMBER = 9;
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
* @return Whether the intDomain field is set.
*/
@java.lang.Override
public boolean hasIntDomain() {
return domainInfoCase_ == 9;
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
* @return The intDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.IntDomain getIntDomain() {
if (domainInfoCase_ == 9) {
return (org.tensorflow.metadata.v0.IntDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.IntDomain.getDefaultInstance();
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.IntDomainOrBuilder getIntDomainOrBuilder() {
if (domainInfoCase_ == 9) {
return (org.tensorflow.metadata.v0.IntDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.IntDomain.getDefaultInstance();
}
public static final int FLOAT_DOMAIN_FIELD_NUMBER = 10;
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
* @return Whether the floatDomain field is set.
*/
@java.lang.Override
public boolean hasFloatDomain() {
return domainInfoCase_ == 10;
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
* @return The floatDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FloatDomain getFloatDomain() {
if (domainInfoCase_ == 10) {
return (org.tensorflow.metadata.v0.FloatDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.FloatDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FloatDomainOrBuilder getFloatDomainOrBuilder() {
if (domainInfoCase_ == 10) {
return (org.tensorflow.metadata.v0.FloatDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.FloatDomain.getDefaultInstance();
}
public static final int STRING_DOMAIN_FIELD_NUMBER = 11;
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
* @return Whether the stringDomain field is set.
*/
@java.lang.Override
public boolean hasStringDomain() {
return domainInfoCase_ == 11;
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
* @return The stringDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StringDomain getStringDomain() {
if (domainInfoCase_ == 11) {
return (org.tensorflow.metadata.v0.StringDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StringDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StringDomainOrBuilder getStringDomainOrBuilder() {
if (domainInfoCase_ == 11) {
return (org.tensorflow.metadata.v0.StringDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StringDomain.getDefaultInstance();
}
public static final int BOOL_DOMAIN_FIELD_NUMBER = 13;
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
* @return Whether the boolDomain field is set.
*/
@java.lang.Override
public boolean hasBoolDomain() {
return domainInfoCase_ == 13;
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
* @return The boolDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BoolDomain getBoolDomain() {
if (domainInfoCase_ == 13) {
return (org.tensorflow.metadata.v0.BoolDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.BoolDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BoolDomainOrBuilder getBoolDomainOrBuilder() {
if (domainInfoCase_ == 13) {
return (org.tensorflow.metadata.v0.BoolDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.BoolDomain.getDefaultInstance();
}
public static final int STRUCT_DOMAIN_FIELD_NUMBER = 29;
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
* @return Whether the structDomain field is set.
*/
@java.lang.Override
public boolean hasStructDomain() {
return domainInfoCase_ == 29;
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
* @return The structDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StructDomain getStructDomain() {
if (domainInfoCase_ == 29) {
return (org.tensorflow.metadata.v0.StructDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StructDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StructDomainOrBuilder getStructDomainOrBuilder() {
if (domainInfoCase_ == 29) {
return (org.tensorflow.metadata.v0.StructDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StructDomain.getDefaultInstance();
}
public static final int NATURAL_LANGUAGE_DOMAIN_FIELD_NUMBER = 24;
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
* @return Whether the naturalLanguageDomain field is set.
*/
@java.lang.Override
public boolean hasNaturalLanguageDomain() {
return domainInfoCase_ == 24;
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
* @return The naturalLanguageDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.NaturalLanguageDomain getNaturalLanguageDomain() {
if (domainInfoCase_ == 24) {
return (org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.NaturalLanguageDomain.getDefaultInstance();
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.NaturalLanguageDomainOrBuilder getNaturalLanguageDomainOrBuilder() {
if (domainInfoCase_ == 24) {
return (org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.NaturalLanguageDomain.getDefaultInstance();
}
public static final int IMAGE_DOMAIN_FIELD_NUMBER = 25;
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
* @return Whether the imageDomain field is set.
*/
@java.lang.Override
public boolean hasImageDomain() {
return domainInfoCase_ == 25;
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
* @return The imageDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ImageDomain getImageDomain() {
if (domainInfoCase_ == 25) {
return (org.tensorflow.metadata.v0.ImageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.ImageDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ImageDomainOrBuilder getImageDomainOrBuilder() {
if (domainInfoCase_ == 25) {
return (org.tensorflow.metadata.v0.ImageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.ImageDomain.getDefaultInstance();
}
public static final int MID_DOMAIN_FIELD_NUMBER = 26;
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
* @return Whether the midDomain field is set.
*/
@java.lang.Override
public boolean hasMidDomain() {
return domainInfoCase_ == 26;
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
* @return The midDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.MIDDomain getMidDomain() {
if (domainInfoCase_ == 26) {
return (org.tensorflow.metadata.v0.MIDDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.MIDDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.MIDDomainOrBuilder getMidDomainOrBuilder() {
if (domainInfoCase_ == 26) {
return (org.tensorflow.metadata.v0.MIDDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.MIDDomain.getDefaultInstance();
}
public static final int URL_DOMAIN_FIELD_NUMBER = 27;
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
* @return Whether the urlDomain field is set.
*/
@java.lang.Override
public boolean hasUrlDomain() {
return domainInfoCase_ == 27;
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
* @return The urlDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.URLDomain getUrlDomain() {
if (domainInfoCase_ == 27) {
return (org.tensorflow.metadata.v0.URLDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.URLDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.URLDomainOrBuilder getUrlDomainOrBuilder() {
if (domainInfoCase_ == 27) {
return (org.tensorflow.metadata.v0.URLDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.URLDomain.getDefaultInstance();
}
public static final int TIME_DOMAIN_FIELD_NUMBER = 28;
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
* @return Whether the timeDomain field is set.
*/
@java.lang.Override
public boolean hasTimeDomain() {
return domainInfoCase_ == 28;
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
* @return The timeDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeDomain getTimeDomain() {
if (domainInfoCase_ == 28) {
return (org.tensorflow.metadata.v0.TimeDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeDomainOrBuilder getTimeDomainOrBuilder() {
if (domainInfoCase_ == 28) {
return (org.tensorflow.metadata.v0.TimeDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeDomain.getDefaultInstance();
}
public static final int TIME_OF_DAY_DOMAIN_FIELD_NUMBER = 30;
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
* @return Whether the timeOfDayDomain field is set.
*/
@java.lang.Override
public boolean hasTimeOfDayDomain() {
return domainInfoCase_ == 30;
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
* @return The timeOfDayDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeOfDayDomain getTimeOfDayDomain() {
if (domainInfoCase_ == 30) {
return (org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeOfDayDomain.getDefaultInstance();
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeOfDayDomainOrBuilder getTimeOfDayDomainOrBuilder() {
if (domainInfoCase_ == 30) {
return (org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeOfDayDomain.getDefaultInstance();
}
public static final int DISTRIBUTION_CONSTRAINTS_FIELD_NUMBER = 15;
private org.tensorflow.metadata.v0.DistributionConstraints distributionConstraints_;
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
* @return Whether the distributionConstraints field is set.
*/
@java.lang.Override
public boolean hasDistributionConstraints() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
* @return The distributionConstraints.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.DistributionConstraints getDistributionConstraints() {
return distributionConstraints_ == null ? org.tensorflow.metadata.v0.DistributionConstraints.getDefaultInstance() : distributionConstraints_;
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.DistributionConstraintsOrBuilder getDistributionConstraintsOrBuilder() {
return distributionConstraints_ == null ? org.tensorflow.metadata.v0.DistributionConstraints.getDefaultInstance() : distributionConstraints_;
}
public static final int ANNOTATION_FIELD_NUMBER = 16;
private org.tensorflow.metadata.v0.Annotation annotation_;
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
* @return Whether the annotation field is set.
*/
@java.lang.Override
public boolean hasAnnotation() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
* @return The annotation.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.Annotation getAnnotation() {
return annotation_ == null ? org.tensorflow.metadata.v0.Annotation.getDefaultInstance() : annotation_;
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.AnnotationOrBuilder getAnnotationOrBuilder() {
return annotation_ == null ? org.tensorflow.metadata.v0.Annotation.getDefaultInstance() : annotation_;
}
public static final int SKEW_COMPARATOR_FIELD_NUMBER = 18;
private org.tensorflow.metadata.v0.FeatureComparator skewComparator_;
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
* @return Whether the skewComparator field is set.
*/
@java.lang.Override
public boolean hasSkewComparator() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
* @return The skewComparator.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeatureComparator getSkewComparator() {
return skewComparator_ == null ? org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : skewComparator_;
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeatureComparatorOrBuilder getSkewComparatorOrBuilder() {
return skewComparator_ == null ? org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : skewComparator_;
}
public static final int DRIFT_COMPARATOR_FIELD_NUMBER = 21;
private org.tensorflow.metadata.v0.FeatureComparator driftComparator_;
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
* @return Whether the driftComparator field is set.
*/
@java.lang.Override
public boolean hasDriftComparator() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
* @return The driftComparator.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeatureComparator getDriftComparator() {
return driftComparator_ == null ? org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : driftComparator_;
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeatureComparatorOrBuilder getDriftComparatorOrBuilder() {
return driftComparator_ == null ? org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : driftComparator_;
}
public static final int IN_ENVIRONMENT_FIELD_NUMBER = 20;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList inEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @return A list containing the inEnvironment.
*/
public com.google.protobuf.ProtocolStringList
getInEnvironmentList() {
return inEnvironment_;
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @return The count of inEnvironment.
*/
public int getInEnvironmentCount() {
return inEnvironment_.size();
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param index The index of the element to return.
* @return The inEnvironment at the given index.
*/
public java.lang.String getInEnvironment(int index) {
return inEnvironment_.get(index);
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param index The index of the value to return.
* @return The bytes of the inEnvironment at the given index.
*/
public com.google.protobuf.ByteString
getInEnvironmentBytes(int index) {
return inEnvironment_.getByteString(index);
}
public static final int NOT_IN_ENVIRONMENT_FIELD_NUMBER = 19;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList notInEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @return A list containing the notInEnvironment.
*/
public com.google.protobuf.ProtocolStringList
getNotInEnvironmentList() {
return notInEnvironment_;
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @return The count of notInEnvironment.
*/
public int getNotInEnvironmentCount() {
return notInEnvironment_.size();
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param index The index of the element to return.
* @return The notInEnvironment at the given index.
*/
public java.lang.String getNotInEnvironment(int index) {
return notInEnvironment_.get(index);
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param index The index of the value to return.
* @return The bytes of the notInEnvironment at the given index.
*/
public com.google.protobuf.ByteString
getNotInEnvironmentBytes(int index) {
return notInEnvironment_.getByteString(index);
}
public static final int LIFECYCLE_STAGE_FIELD_NUMBER = 22;
private int lifecycleStage_ = 0;
/**
*
* The lifecycle stage of a feature. It can also apply to its descendants.
* i.e., if a struct is DEPRECATED, its children are implicitly deprecated.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 22;
* @return Whether the lifecycleStage field is set.
*/
@java.lang.Override public boolean hasLifecycleStage() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The lifecycle stage of a feature. It can also apply to its descendants.
* i.e., if a struct is DEPRECATED, its children are implicitly deprecated.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 22;
* @return The lifecycleStage.
*/
@java.lang.Override public org.tensorflow.metadata.v0.LifecycleStage getLifecycleStage() {
org.tensorflow.metadata.v0.LifecycleStage result = org.tensorflow.metadata.v0.LifecycleStage.forNumber(lifecycleStage_);
return result == null ? org.tensorflow.metadata.v0.LifecycleStage.UNKNOWN_STAGE : result;
}
public static final int UNIQUE_CONSTRAINTS_FIELD_NUMBER = 31;
private org.tensorflow.metadata.v0.UniqueConstraints uniqueConstraints_;
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
* @return Whether the uniqueConstraints field is set.
*/
@java.lang.Override
public boolean hasUniqueConstraints() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
* @return The uniqueConstraints.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.UniqueConstraints getUniqueConstraints() {
return uniqueConstraints_ == null ? org.tensorflow.metadata.v0.UniqueConstraints.getDefaultInstance() : uniqueConstraints_;
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.UniqueConstraintsOrBuilder getUniqueConstraintsOrBuilder() {
return uniqueConstraints_ == null ? org.tensorflow.metadata.v0.UniqueConstraints.getDefaultInstance() : uniqueConstraints_;
}
public static final int VALIDATION_DERIVED_SOURCE_FIELD_NUMBER = 34;
private org.tensorflow.metadata.v0.DerivedFeatureSource validationDerivedSource_;
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
* @return Whether the validationDerivedSource field is set.
*/
@java.lang.Override
public boolean hasValidationDerivedSource() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
* @return The validationDerivedSource.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.DerivedFeatureSource getValidationDerivedSource() {
return validationDerivedSource_ == null ? org.tensorflow.metadata.v0.DerivedFeatureSource.getDefaultInstance() : validationDerivedSource_;
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.DerivedFeatureSourceOrBuilder getValidationDerivedSourceOrBuilder() {
return validationDerivedSource_ == null ? org.tensorflow.metadata.v0.DerivedFeatureSource.getDefaultInstance() : validationDerivedSource_;
}
public static final int SEQUENCE_METADATA_FIELD_NUMBER = 35;
private org.tensorflow.metadata.v0.SequenceMetadata sequenceMetadata_;
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
* @return Whether the sequenceMetadata field is set.
*/
@java.lang.Override
public boolean hasSequenceMetadata() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
* @return The sequenceMetadata.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.SequenceMetadata getSequenceMetadata() {
return sequenceMetadata_ == null ? org.tensorflow.metadata.v0.SequenceMetadata.getDefaultInstance() : sequenceMetadata_;
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.SequenceMetadataOrBuilder getSequenceMetadataOrBuilder() {
return sequenceMetadata_ == null ? org.tensorflow.metadata.v0.SequenceMetadata.getDefaultInstance() : sequenceMetadata_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(2, deprecated_);
}
if (shapeTypeCase_ == 5) {
output.writeMessage(5, (org.tensorflow.metadata.v0.ValueCount) shapeType_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeEnum(6, type_);
}
if (domainInfoCase_ == 7) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, domainInfo_);
}
if (domainInfoCase_ == 9) {
output.writeMessage(9, (org.tensorflow.metadata.v0.IntDomain) domainInfo_);
}
if (domainInfoCase_ == 10) {
output.writeMessage(10, (org.tensorflow.metadata.v0.FloatDomain) domainInfo_);
}
if (domainInfoCase_ == 11) {
output.writeMessage(11, (org.tensorflow.metadata.v0.StringDomain) domainInfo_);
}
if (domainInfoCase_ == 13) {
output.writeMessage(13, (org.tensorflow.metadata.v0.BoolDomain) domainInfo_);
}
if (presenceConstraintsCase_ == 14) {
output.writeMessage(14, (org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(15, getDistributionConstraints());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(16, getAnnotation());
}
if (presenceConstraintsCase_ == 17) {
output.writeMessage(17, (org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(18, getSkewComparator());
}
for (int i = 0; i < notInEnvironment_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, notInEnvironment_.getRaw(i));
}
for (int i = 0; i < inEnvironment_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, inEnvironment_.getRaw(i));
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(21, getDriftComparator());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeEnum(22, lifecycleStage_);
}
if (shapeTypeCase_ == 23) {
output.writeMessage(23, (org.tensorflow.metadata.v0.FixedShape) shapeType_);
}
if (domainInfoCase_ == 24) {
output.writeMessage(24, (org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_);
}
if (domainInfoCase_ == 25) {
output.writeMessage(25, (org.tensorflow.metadata.v0.ImageDomain) domainInfo_);
}
if (domainInfoCase_ == 26) {
output.writeMessage(26, (org.tensorflow.metadata.v0.MIDDomain) domainInfo_);
}
if (domainInfoCase_ == 27) {
output.writeMessage(27, (org.tensorflow.metadata.v0.URLDomain) domainInfo_);
}
if (domainInfoCase_ == 28) {
output.writeMessage(28, (org.tensorflow.metadata.v0.TimeDomain) domainInfo_);
}
if (domainInfoCase_ == 29) {
output.writeMessage(29, (org.tensorflow.metadata.v0.StructDomain) domainInfo_);
}
if (domainInfoCase_ == 30) {
output.writeMessage(30, (org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(31, getUniqueConstraints());
}
if (shapeTypeCase_ == 32) {
output.writeMessage(32, (org.tensorflow.metadata.v0.ValueCountList) shapeType_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(34, getValidationDerivedSource());
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(35, getSequenceMetadata());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, deprecated_);
}
if (shapeTypeCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (org.tensorflow.metadata.v0.ValueCount) shapeType_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, type_);
}
if (domainInfoCase_ == 7) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, domainInfo_);
}
if (domainInfoCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, (org.tensorflow.metadata.v0.IntDomain) domainInfo_);
}
if (domainInfoCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (org.tensorflow.metadata.v0.FloatDomain) domainInfo_);
}
if (domainInfoCase_ == 11) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, (org.tensorflow.metadata.v0.StringDomain) domainInfo_);
}
if (domainInfoCase_ == 13) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, (org.tensorflow.metadata.v0.BoolDomain) domainInfo_);
}
if (presenceConstraintsCase_ == 14) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, (org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, getDistributionConstraints());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getAnnotation());
}
if (presenceConstraintsCase_ == 17) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, (org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getSkewComparator());
}
{
int dataSize = 0;
for (int i = 0; i < notInEnvironment_.size(); i++) {
dataSize += computeStringSizeNoTag(notInEnvironment_.getRaw(i));
}
size += dataSize;
size += 2 * getNotInEnvironmentList().size();
}
{
int dataSize = 0;
for (int i = 0; i < inEnvironment_.size(); i++) {
dataSize += computeStringSizeNoTag(inEnvironment_.getRaw(i));
}
size += dataSize;
size += 2 * getInEnvironmentList().size();
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getDriftComparator());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(22, lifecycleStage_);
}
if (shapeTypeCase_ == 23) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, (org.tensorflow.metadata.v0.FixedShape) shapeType_);
}
if (domainInfoCase_ == 24) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, (org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_);
}
if (domainInfoCase_ == 25) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(25, (org.tensorflow.metadata.v0.ImageDomain) domainInfo_);
}
if (domainInfoCase_ == 26) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(26, (org.tensorflow.metadata.v0.MIDDomain) domainInfo_);
}
if (domainInfoCase_ == 27) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(27, (org.tensorflow.metadata.v0.URLDomain) domainInfo_);
}
if (domainInfoCase_ == 28) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(28, (org.tensorflow.metadata.v0.TimeDomain) domainInfo_);
}
if (domainInfoCase_ == 29) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(29, (org.tensorflow.metadata.v0.StructDomain) domainInfo_);
}
if (domainInfoCase_ == 30) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(30, (org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(31, getUniqueConstraints());
}
if (shapeTypeCase_ == 32) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(32, (org.tensorflow.metadata.v0.ValueCountList) shapeType_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(34, getValidationDerivedSource());
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(35, getSequenceMetadata());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.Feature)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.Feature other = (org.tensorflow.metadata.v0.Feature) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasDeprecated() != other.hasDeprecated()) return false;
if (hasDeprecated()) {
if (getDeprecated()
!= other.getDeprecated()) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (hasDistributionConstraints() != other.hasDistributionConstraints()) return false;
if (hasDistributionConstraints()) {
if (!getDistributionConstraints()
.equals(other.getDistributionConstraints())) return false;
}
if (hasAnnotation() != other.hasAnnotation()) return false;
if (hasAnnotation()) {
if (!getAnnotation()
.equals(other.getAnnotation())) return false;
}
if (hasSkewComparator() != other.hasSkewComparator()) return false;
if (hasSkewComparator()) {
if (!getSkewComparator()
.equals(other.getSkewComparator())) return false;
}
if (hasDriftComparator() != other.hasDriftComparator()) return false;
if (hasDriftComparator()) {
if (!getDriftComparator()
.equals(other.getDriftComparator())) return false;
}
if (!getInEnvironmentList()
.equals(other.getInEnvironmentList())) return false;
if (!getNotInEnvironmentList()
.equals(other.getNotInEnvironmentList())) return false;
if (hasLifecycleStage() != other.hasLifecycleStage()) return false;
if (hasLifecycleStage()) {
if (lifecycleStage_ != other.lifecycleStage_) return false;
}
if (hasUniqueConstraints() != other.hasUniqueConstraints()) return false;
if (hasUniqueConstraints()) {
if (!getUniqueConstraints()
.equals(other.getUniqueConstraints())) return false;
}
if (hasValidationDerivedSource() != other.hasValidationDerivedSource()) return false;
if (hasValidationDerivedSource()) {
if (!getValidationDerivedSource()
.equals(other.getValidationDerivedSource())) return false;
}
if (hasSequenceMetadata() != other.hasSequenceMetadata()) return false;
if (hasSequenceMetadata()) {
if (!getSequenceMetadata()
.equals(other.getSequenceMetadata())) return false;
}
if (!getPresenceConstraintsCase().equals(other.getPresenceConstraintsCase())) return false;
switch (presenceConstraintsCase_) {
case 14:
if (!getPresence()
.equals(other.getPresence())) return false;
break;
case 17:
if (!getGroupPresence()
.equals(other.getGroupPresence())) return false;
break;
case 0:
default:
}
if (!getShapeTypeCase().equals(other.getShapeTypeCase())) return false;
switch (shapeTypeCase_) {
case 23:
if (!getShape()
.equals(other.getShape())) return false;
break;
case 5:
if (!getValueCount()
.equals(other.getValueCount())) return false;
break;
case 32:
if (!getValueCounts()
.equals(other.getValueCounts())) return false;
break;
case 0:
default:
}
if (!getDomainInfoCase().equals(other.getDomainInfoCase())) return false;
switch (domainInfoCase_) {
case 7:
if (!getDomain()
.equals(other.getDomain())) return false;
break;
case 9:
if (!getIntDomain()
.equals(other.getIntDomain())) return false;
break;
case 10:
if (!getFloatDomain()
.equals(other.getFloatDomain())) return false;
break;
case 11:
if (!getStringDomain()
.equals(other.getStringDomain())) return false;
break;
case 13:
if (!getBoolDomain()
.equals(other.getBoolDomain())) return false;
break;
case 29:
if (!getStructDomain()
.equals(other.getStructDomain())) return false;
break;
case 24:
if (!getNaturalLanguageDomain()
.equals(other.getNaturalLanguageDomain())) return false;
break;
case 25:
if (!getImageDomain()
.equals(other.getImageDomain())) return false;
break;
case 26:
if (!getMidDomain()
.equals(other.getMidDomain())) return false;
break;
case 27:
if (!getUrlDomain()
.equals(other.getUrlDomain())) return false;
break;
case 28:
if (!getTimeDomain()
.equals(other.getTimeDomain())) return false;
break;
case 30:
if (!getTimeOfDayDomain()
.equals(other.getTimeOfDayDomain())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasDeprecated()) {
hash = (37 * hash) + DEPRECATED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDeprecated());
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasDistributionConstraints()) {
hash = (37 * hash) + DISTRIBUTION_CONSTRAINTS_FIELD_NUMBER;
hash = (53 * hash) + getDistributionConstraints().hashCode();
}
if (hasAnnotation()) {
hash = (37 * hash) + ANNOTATION_FIELD_NUMBER;
hash = (53 * hash) + getAnnotation().hashCode();
}
if (hasSkewComparator()) {
hash = (37 * hash) + SKEW_COMPARATOR_FIELD_NUMBER;
hash = (53 * hash) + getSkewComparator().hashCode();
}
if (hasDriftComparator()) {
hash = (37 * hash) + DRIFT_COMPARATOR_FIELD_NUMBER;
hash = (53 * hash) + getDriftComparator().hashCode();
}
if (getInEnvironmentCount() > 0) {
hash = (37 * hash) + IN_ENVIRONMENT_FIELD_NUMBER;
hash = (53 * hash) + getInEnvironmentList().hashCode();
}
if (getNotInEnvironmentCount() > 0) {
hash = (37 * hash) + NOT_IN_ENVIRONMENT_FIELD_NUMBER;
hash = (53 * hash) + getNotInEnvironmentList().hashCode();
}
if (hasLifecycleStage()) {
hash = (37 * hash) + LIFECYCLE_STAGE_FIELD_NUMBER;
hash = (53 * hash) + lifecycleStage_;
}
if (hasUniqueConstraints()) {
hash = (37 * hash) + UNIQUE_CONSTRAINTS_FIELD_NUMBER;
hash = (53 * hash) + getUniqueConstraints().hashCode();
}
if (hasValidationDerivedSource()) {
hash = (37 * hash) + VALIDATION_DERIVED_SOURCE_FIELD_NUMBER;
hash = (53 * hash) + getValidationDerivedSource().hashCode();
}
if (hasSequenceMetadata()) {
hash = (37 * hash) + SEQUENCE_METADATA_FIELD_NUMBER;
hash = (53 * hash) + getSequenceMetadata().hashCode();
}
switch (presenceConstraintsCase_) {
case 14:
hash = (37 * hash) + PRESENCE_FIELD_NUMBER;
hash = (53 * hash) + getPresence().hashCode();
break;
case 17:
hash = (37 * hash) + GROUP_PRESENCE_FIELD_NUMBER;
hash = (53 * hash) + getGroupPresence().hashCode();
break;
case 0:
default:
}
switch (shapeTypeCase_) {
case 23:
hash = (37 * hash) + SHAPE_FIELD_NUMBER;
hash = (53 * hash) + getShape().hashCode();
break;
case 5:
hash = (37 * hash) + VALUE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getValueCount().hashCode();
break;
case 32:
hash = (37 * hash) + VALUE_COUNTS_FIELD_NUMBER;
hash = (53 * hash) + getValueCounts().hashCode();
break;
case 0:
default:
}
switch (domainInfoCase_) {
case 7:
hash = (37 * hash) + DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getDomain().hashCode();
break;
case 9:
hash = (37 * hash) + INT_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getIntDomain().hashCode();
break;
case 10:
hash = (37 * hash) + FLOAT_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getFloatDomain().hashCode();
break;
case 11:
hash = (37 * hash) + STRING_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getStringDomain().hashCode();
break;
case 13:
hash = (37 * hash) + BOOL_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getBoolDomain().hashCode();
break;
case 29:
hash = (37 * hash) + STRUCT_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getStructDomain().hashCode();
break;
case 24:
hash = (37 * hash) + NATURAL_LANGUAGE_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getNaturalLanguageDomain().hashCode();
break;
case 25:
hash = (37 * hash) + IMAGE_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getImageDomain().hashCode();
break;
case 26:
hash = (37 * hash) + MID_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getMidDomain().hashCode();
break;
case 27:
hash = (37 * hash) + URL_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getUrlDomain().hashCode();
break;
case 28:
hash = (37 * hash) + TIME_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getTimeDomain().hashCode();
break;
case 30:
hash = (37 * hash) + TIME_OF_DAY_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getTimeOfDayDomain().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.Feature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.Feature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.Feature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.Feature prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Describes schema-level information about a specific feature.
* NextID: 36
*
*
* Protobuf type {@code tensorflow.metadata.v0.Feature}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.Feature)
org.tensorflow.metadata.v0.FeatureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_Feature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_Feature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.Feature.class, org.tensorflow.metadata.v0.Feature.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.Feature.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDistributionConstraintsFieldBuilder();
getAnnotationFieldBuilder();
getSkewComparatorFieldBuilder();
getDriftComparatorFieldBuilder();
getUniqueConstraintsFieldBuilder();
getValidationDerivedSourceFieldBuilder();
getSequenceMetadataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
deprecated_ = false;
if (presenceBuilder_ != null) {
presenceBuilder_.clear();
}
if (groupPresenceBuilder_ != null) {
groupPresenceBuilder_.clear();
}
if (shapeBuilder_ != null) {
shapeBuilder_.clear();
}
if (valueCountBuilder_ != null) {
valueCountBuilder_.clear();
}
if (valueCountsBuilder_ != null) {
valueCountsBuilder_.clear();
}
type_ = 0;
if (intDomainBuilder_ != null) {
intDomainBuilder_.clear();
}
if (floatDomainBuilder_ != null) {
floatDomainBuilder_.clear();
}
if (stringDomainBuilder_ != null) {
stringDomainBuilder_.clear();
}
if (boolDomainBuilder_ != null) {
boolDomainBuilder_.clear();
}
if (structDomainBuilder_ != null) {
structDomainBuilder_.clear();
}
if (naturalLanguageDomainBuilder_ != null) {
naturalLanguageDomainBuilder_.clear();
}
if (imageDomainBuilder_ != null) {
imageDomainBuilder_.clear();
}
if (midDomainBuilder_ != null) {
midDomainBuilder_.clear();
}
if (urlDomainBuilder_ != null) {
urlDomainBuilder_.clear();
}
if (timeDomainBuilder_ != null) {
timeDomainBuilder_.clear();
}
if (timeOfDayDomainBuilder_ != null) {
timeOfDayDomainBuilder_.clear();
}
distributionConstraints_ = null;
if (distributionConstraintsBuilder_ != null) {
distributionConstraintsBuilder_.dispose();
distributionConstraintsBuilder_ = null;
}
annotation_ = null;
if (annotationBuilder_ != null) {
annotationBuilder_.dispose();
annotationBuilder_ = null;
}
skewComparator_ = null;
if (skewComparatorBuilder_ != null) {
skewComparatorBuilder_.dispose();
skewComparatorBuilder_ = null;
}
driftComparator_ = null;
if (driftComparatorBuilder_ != null) {
driftComparatorBuilder_.dispose();
driftComparatorBuilder_ = null;
}
inEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
notInEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
lifecycleStage_ = 0;
uniqueConstraints_ = null;
if (uniqueConstraintsBuilder_ != null) {
uniqueConstraintsBuilder_.dispose();
uniqueConstraintsBuilder_ = null;
}
validationDerivedSource_ = null;
if (validationDerivedSourceBuilder_ != null) {
validationDerivedSourceBuilder_.dispose();
validationDerivedSourceBuilder_ = null;
}
sequenceMetadata_ = null;
if (sequenceMetadataBuilder_ != null) {
sequenceMetadataBuilder_.dispose();
sequenceMetadataBuilder_ = null;
}
presenceConstraintsCase_ = 0;
presenceConstraints_ = null;
shapeTypeCase_ = 0;
shapeType_ = null;
domainInfoCase_ = 0;
domainInfo_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_Feature_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.Feature getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.Feature.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.Feature build() {
org.tensorflow.metadata.v0.Feature result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.Feature buildPartial() {
org.tensorflow.metadata.v0.Feature result = new org.tensorflow.metadata.v0.Feature(this);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(org.tensorflow.metadata.v0.Feature result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.deprecated_ = deprecated_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.distributionConstraints_ = distributionConstraintsBuilder_ == null
? distributionConstraints_
: distributionConstraintsBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.annotation_ = annotationBuilder_ == null
? annotation_
: annotationBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.skewComparator_ = skewComparatorBuilder_ == null
? skewComparator_
: skewComparatorBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.driftComparator_ = driftComparatorBuilder_ == null
? driftComparator_
: driftComparatorBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
inEnvironment_.makeImmutable();
result.inEnvironment_ = inEnvironment_;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
notInEnvironment_.makeImmutable();
result.notInEnvironment_ = notInEnvironment_;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.lifecycleStage_ = lifecycleStage_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.uniqueConstraints_ = uniqueConstraintsBuilder_ == null
? uniqueConstraints_
: uniqueConstraintsBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.validationDerivedSource_ = validationDerivedSourceBuilder_ == null
? validationDerivedSource_
: validationDerivedSourceBuilder_.build();
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x20000000) != 0)) {
result.sequenceMetadata_ = sequenceMetadataBuilder_ == null
? sequenceMetadata_
: sequenceMetadataBuilder_.build();
to_bitField0_ |= 0x00000400;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(org.tensorflow.metadata.v0.Feature result) {
result.presenceConstraintsCase_ = presenceConstraintsCase_;
result.presenceConstraints_ = this.presenceConstraints_;
if (presenceConstraintsCase_ == 14 &&
presenceBuilder_ != null) {
result.presenceConstraints_ = presenceBuilder_.build();
}
if (presenceConstraintsCase_ == 17 &&
groupPresenceBuilder_ != null) {
result.presenceConstraints_ = groupPresenceBuilder_.build();
}
result.shapeTypeCase_ = shapeTypeCase_;
result.shapeType_ = this.shapeType_;
if (shapeTypeCase_ == 23 &&
shapeBuilder_ != null) {
result.shapeType_ = shapeBuilder_.build();
}
if (shapeTypeCase_ == 5 &&
valueCountBuilder_ != null) {
result.shapeType_ = valueCountBuilder_.build();
}
if (shapeTypeCase_ == 32 &&
valueCountsBuilder_ != null) {
result.shapeType_ = valueCountsBuilder_.build();
}
result.domainInfoCase_ = domainInfoCase_;
result.domainInfo_ = this.domainInfo_;
if (domainInfoCase_ == 9 &&
intDomainBuilder_ != null) {
result.domainInfo_ = intDomainBuilder_.build();
}
if (domainInfoCase_ == 10 &&
floatDomainBuilder_ != null) {
result.domainInfo_ = floatDomainBuilder_.build();
}
if (domainInfoCase_ == 11 &&
stringDomainBuilder_ != null) {
result.domainInfo_ = stringDomainBuilder_.build();
}
if (domainInfoCase_ == 13 &&
boolDomainBuilder_ != null) {
result.domainInfo_ = boolDomainBuilder_.build();
}
if (domainInfoCase_ == 29 &&
structDomainBuilder_ != null) {
result.domainInfo_ = structDomainBuilder_.build();
}
if (domainInfoCase_ == 24 &&
naturalLanguageDomainBuilder_ != null) {
result.domainInfo_ = naturalLanguageDomainBuilder_.build();
}
if (domainInfoCase_ == 25 &&
imageDomainBuilder_ != null) {
result.domainInfo_ = imageDomainBuilder_.build();
}
if (domainInfoCase_ == 26 &&
midDomainBuilder_ != null) {
result.domainInfo_ = midDomainBuilder_.build();
}
if (domainInfoCase_ == 27 &&
urlDomainBuilder_ != null) {
result.domainInfo_ = urlDomainBuilder_.build();
}
if (domainInfoCase_ == 28 &&
timeDomainBuilder_ != null) {
result.domainInfo_ = timeDomainBuilder_.build();
}
if (domainInfoCase_ == 30 &&
timeOfDayDomainBuilder_ != null) {
result.domainInfo_ = timeOfDayDomainBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.Feature) {
return mergeFrom((org.tensorflow.metadata.v0.Feature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.Feature other) {
if (other == org.tensorflow.metadata.v0.Feature.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasDeprecated()) {
setDeprecated(other.getDeprecated());
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasDistributionConstraints()) {
mergeDistributionConstraints(other.getDistributionConstraints());
}
if (other.hasAnnotation()) {
mergeAnnotation(other.getAnnotation());
}
if (other.hasSkewComparator()) {
mergeSkewComparator(other.getSkewComparator());
}
if (other.hasDriftComparator()) {
mergeDriftComparator(other.getDriftComparator());
}
if (!other.inEnvironment_.isEmpty()) {
if (inEnvironment_.isEmpty()) {
inEnvironment_ = other.inEnvironment_;
bitField0_ |= 0x01000000;
} else {
ensureInEnvironmentIsMutable();
inEnvironment_.addAll(other.inEnvironment_);
}
onChanged();
}
if (!other.notInEnvironment_.isEmpty()) {
if (notInEnvironment_.isEmpty()) {
notInEnvironment_ = other.notInEnvironment_;
bitField0_ |= 0x02000000;
} else {
ensureNotInEnvironmentIsMutable();
notInEnvironment_.addAll(other.notInEnvironment_);
}
onChanged();
}
if (other.hasLifecycleStage()) {
setLifecycleStage(other.getLifecycleStage());
}
if (other.hasUniqueConstraints()) {
mergeUniqueConstraints(other.getUniqueConstraints());
}
if (other.hasValidationDerivedSource()) {
mergeValidationDerivedSource(other.getValidationDerivedSource());
}
if (other.hasSequenceMetadata()) {
mergeSequenceMetadata(other.getSequenceMetadata());
}
switch (other.getPresenceConstraintsCase()) {
case PRESENCE: {
mergePresence(other.getPresence());
break;
}
case GROUP_PRESENCE: {
mergeGroupPresence(other.getGroupPresence());
break;
}
case PRESENCECONSTRAINTS_NOT_SET: {
break;
}
}
switch (other.getShapeTypeCase()) {
case SHAPE: {
mergeShape(other.getShape());
break;
}
case VALUE_COUNT: {
mergeValueCount(other.getValueCount());
break;
}
case VALUE_COUNTS: {
mergeValueCounts(other.getValueCounts());
break;
}
case SHAPETYPE_NOT_SET: {
break;
}
}
switch (other.getDomainInfoCase()) {
case DOMAIN: {
domainInfoCase_ = 7;
domainInfo_ = other.domainInfo_;
onChanged();
break;
}
case INT_DOMAIN: {
mergeIntDomain(other.getIntDomain());
break;
}
case FLOAT_DOMAIN: {
mergeFloatDomain(other.getFloatDomain());
break;
}
case STRING_DOMAIN: {
mergeStringDomain(other.getStringDomain());
break;
}
case BOOL_DOMAIN: {
mergeBoolDomain(other.getBoolDomain());
break;
}
case STRUCT_DOMAIN: {
mergeStructDomain(other.getStructDomain());
break;
}
case NATURAL_LANGUAGE_DOMAIN: {
mergeNaturalLanguageDomain(other.getNaturalLanguageDomain());
break;
}
case IMAGE_DOMAIN: {
mergeImageDomain(other.getImageDomain());
break;
}
case MID_DOMAIN: {
mergeMidDomain(other.getMidDomain());
break;
}
case URL_DOMAIN: {
mergeUrlDomain(other.getUrlDomain());
break;
}
case TIME_DOMAIN: {
mergeTimeDomain(other.getTimeDomain());
break;
}
case TIME_OF_DAY_DOMAIN: {
mergeTimeOfDayDomain(other.getTimeOfDayDomain());
break;
}
case DOMAININFO_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
deprecated_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
case 42: {
input.readMessage(
getValueCountFieldBuilder().getBuilder(),
extensionRegistry);
shapeTypeCase_ = 5;
break;
} // case 42
case 48: {
int tmpRaw = input.readEnum();
org.tensorflow.metadata.v0.FeatureType tmpValue =
org.tensorflow.metadata.v0.FeatureType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(6, tmpRaw);
} else {
type_ = tmpRaw;
bitField0_ |= 0x00000080;
}
break;
} // case 48
case 58: {
com.google.protobuf.ByteString bs = input.readBytes();
domainInfoCase_ = 7;
domainInfo_ = bs;
break;
} // case 58
case 74: {
input.readMessage(
getIntDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 9;
break;
} // case 74
case 82: {
input.readMessage(
getFloatDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 10;
break;
} // case 82
case 90: {
input.readMessage(
getStringDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 11;
break;
} // case 90
case 106: {
input.readMessage(
getBoolDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 13;
break;
} // case 106
case 114: {
input.readMessage(
getPresenceFieldBuilder().getBuilder(),
extensionRegistry);
presenceConstraintsCase_ = 14;
break;
} // case 114
case 122: {
input.readMessage(
getDistributionConstraintsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00100000;
break;
} // case 122
case 130: {
input.readMessage(
getAnnotationFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00200000;
break;
} // case 130
case 138: {
input.readMessage(
getGroupPresenceFieldBuilder().getBuilder(),
extensionRegistry);
presenceConstraintsCase_ = 17;
break;
} // case 138
case 146: {
input.readMessage(
getSkewComparatorFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00400000;
break;
} // case 146
case 154: {
com.google.protobuf.ByteString bs = input.readBytes();
ensureNotInEnvironmentIsMutable();
notInEnvironment_.add(bs);
break;
} // case 154
case 162: {
com.google.protobuf.ByteString bs = input.readBytes();
ensureInEnvironmentIsMutable();
inEnvironment_.add(bs);
break;
} // case 162
case 170: {
input.readMessage(
getDriftComparatorFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00800000;
break;
} // case 170
case 176: {
int tmpRaw = input.readEnum();
org.tensorflow.metadata.v0.LifecycleStage tmpValue =
org.tensorflow.metadata.v0.LifecycleStage.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(22, tmpRaw);
} else {
lifecycleStage_ = tmpRaw;
bitField0_ |= 0x04000000;
}
break;
} // case 176
case 186: {
input.readMessage(
getShapeFieldBuilder().getBuilder(),
extensionRegistry);
shapeTypeCase_ = 23;
break;
} // case 186
case 194: {
input.readMessage(
getNaturalLanguageDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 24;
break;
} // case 194
case 202: {
input.readMessage(
getImageDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 25;
break;
} // case 202
case 210: {
input.readMessage(
getMidDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 26;
break;
} // case 210
case 218: {
input.readMessage(
getUrlDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 27;
break;
} // case 218
case 226: {
input.readMessage(
getTimeDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 28;
break;
} // case 226
case 234: {
input.readMessage(
getStructDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 29;
break;
} // case 234
case 242: {
input.readMessage(
getTimeOfDayDomainFieldBuilder().getBuilder(),
extensionRegistry);
domainInfoCase_ = 30;
break;
} // case 242
case 250: {
input.readMessage(
getUniqueConstraintsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x08000000;
break;
} // case 250
case 258: {
input.readMessage(
getValueCountsFieldBuilder().getBuilder(),
extensionRegistry);
shapeTypeCase_ = 32;
break;
} // case 258
case 274: {
input.readMessage(
getValidationDerivedSourceFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x10000000;
break;
} // case 274
case 282: {
input.readMessage(
getSequenceMetadataFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x20000000;
break;
} // case 282
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int presenceConstraintsCase_ = 0;
private java.lang.Object presenceConstraints_;
public PresenceConstraintsCase
getPresenceConstraintsCase() {
return PresenceConstraintsCase.forNumber(
presenceConstraintsCase_);
}
public Builder clearPresenceConstraints() {
presenceConstraintsCase_ = 0;
presenceConstraints_ = null;
onChanged();
return this;
}
private int shapeTypeCase_ = 0;
private java.lang.Object shapeType_;
public ShapeTypeCase
getShapeTypeCase() {
return ShapeTypeCase.forNumber(
shapeTypeCase_);
}
public Builder clearShapeType() {
shapeTypeCase_ = 0;
shapeType_ = null;
onChanged();
return this;
}
private int domainInfoCase_ = 0;
private java.lang.Object domainInfo_;
public DomainInfoCase
getDomainInfoCase() {
return DomainInfoCase.forNumber(
domainInfoCase_);
}
public Builder clearDomainInfo() {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The name of the feature.
*
*
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private boolean deprecated_ ;
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.Feature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=153
* @return Whether the deprecated field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasDeprecated() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.Feature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=153
* @return The deprecated.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getDeprecated() {
return deprecated_;
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.Feature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=153
* @param value The deprecated to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setDeprecated(boolean value) {
deprecated_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.Feature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=153
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearDeprecated() {
bitField0_ = (bitField0_ & ~0x00000002);
deprecated_ = false;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresence, org.tensorflow.metadata.v0.FeaturePresence.Builder, org.tensorflow.metadata.v0.FeaturePresenceOrBuilder> presenceBuilder_;
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
* @return Whether the presence field is set.
*/
@java.lang.Override
public boolean hasPresence() {
return presenceConstraintsCase_ == 14;
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
* @return The presence.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresence getPresence() {
if (presenceBuilder_ == null) {
if (presenceConstraintsCase_ == 14) {
return (org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance();
} else {
if (presenceConstraintsCase_ == 14) {
return presenceBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance();
}
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
public Builder setPresence(org.tensorflow.metadata.v0.FeaturePresence value) {
if (presenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
presenceConstraints_ = value;
onChanged();
} else {
presenceBuilder_.setMessage(value);
}
presenceConstraintsCase_ = 14;
return this;
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
public Builder setPresence(
org.tensorflow.metadata.v0.FeaturePresence.Builder builderForValue) {
if (presenceBuilder_ == null) {
presenceConstraints_ = builderForValue.build();
onChanged();
} else {
presenceBuilder_.setMessage(builderForValue.build());
}
presenceConstraintsCase_ = 14;
return this;
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
public Builder mergePresence(org.tensorflow.metadata.v0.FeaturePresence value) {
if (presenceBuilder_ == null) {
if (presenceConstraintsCase_ == 14 &&
presenceConstraints_ != org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance()) {
presenceConstraints_ = org.tensorflow.metadata.v0.FeaturePresence.newBuilder((org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_)
.mergeFrom(value).buildPartial();
} else {
presenceConstraints_ = value;
}
onChanged();
} else {
if (presenceConstraintsCase_ == 14) {
presenceBuilder_.mergeFrom(value);
} else {
presenceBuilder_.setMessage(value);
}
}
presenceConstraintsCase_ = 14;
return this;
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
public Builder clearPresence() {
if (presenceBuilder_ == null) {
if (presenceConstraintsCase_ == 14) {
presenceConstraintsCase_ = 0;
presenceConstraints_ = null;
onChanged();
}
} else {
if (presenceConstraintsCase_ == 14) {
presenceConstraintsCase_ = 0;
presenceConstraints_ = null;
}
presenceBuilder_.clear();
}
return this;
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
public org.tensorflow.metadata.v0.FeaturePresence.Builder getPresenceBuilder() {
return getPresenceFieldBuilder().getBuilder();
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresenceOrBuilder getPresenceOrBuilder() {
if ((presenceConstraintsCase_ == 14) && (presenceBuilder_ != null)) {
return presenceBuilder_.getMessageOrBuilder();
} else {
if (presenceConstraintsCase_ == 14) {
return (org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance();
}
}
/**
*
* Constraints on the presence of this feature in the examples.
*
*
* .tensorflow.metadata.v0.FeaturePresence presence = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresence, org.tensorflow.metadata.v0.FeaturePresence.Builder, org.tensorflow.metadata.v0.FeaturePresenceOrBuilder>
getPresenceFieldBuilder() {
if (presenceBuilder_ == null) {
if (!(presenceConstraintsCase_ == 14)) {
presenceConstraints_ = org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance();
}
presenceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresence, org.tensorflow.metadata.v0.FeaturePresence.Builder, org.tensorflow.metadata.v0.FeaturePresenceOrBuilder>(
(org.tensorflow.metadata.v0.FeaturePresence) presenceConstraints_,
getParentForChildren(),
isClean());
presenceConstraints_ = null;
}
presenceConstraintsCase_ = 14;
onChanged();
return presenceBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresenceWithinGroup, org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.Builder, org.tensorflow.metadata.v0.FeaturePresenceWithinGroupOrBuilder> groupPresenceBuilder_;
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
* @return Whether the groupPresence field is set.
*/
@java.lang.Override
public boolean hasGroupPresence() {
return presenceConstraintsCase_ == 17;
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
* @return The groupPresence.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresenceWithinGroup getGroupPresence() {
if (groupPresenceBuilder_ == null) {
if (presenceConstraintsCase_ == 17) {
return (org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.getDefaultInstance();
} else {
if (presenceConstraintsCase_ == 17) {
return groupPresenceBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.getDefaultInstance();
}
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
public Builder setGroupPresence(org.tensorflow.metadata.v0.FeaturePresenceWithinGroup value) {
if (groupPresenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
presenceConstraints_ = value;
onChanged();
} else {
groupPresenceBuilder_.setMessage(value);
}
presenceConstraintsCase_ = 17;
return this;
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
public Builder setGroupPresence(
org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.Builder builderForValue) {
if (groupPresenceBuilder_ == null) {
presenceConstraints_ = builderForValue.build();
onChanged();
} else {
groupPresenceBuilder_.setMessage(builderForValue.build());
}
presenceConstraintsCase_ = 17;
return this;
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
public Builder mergeGroupPresence(org.tensorflow.metadata.v0.FeaturePresenceWithinGroup value) {
if (groupPresenceBuilder_ == null) {
if (presenceConstraintsCase_ == 17 &&
presenceConstraints_ != org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.getDefaultInstance()) {
presenceConstraints_ = org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.newBuilder((org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_)
.mergeFrom(value).buildPartial();
} else {
presenceConstraints_ = value;
}
onChanged();
} else {
if (presenceConstraintsCase_ == 17) {
groupPresenceBuilder_.mergeFrom(value);
} else {
groupPresenceBuilder_.setMessage(value);
}
}
presenceConstraintsCase_ = 17;
return this;
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
public Builder clearGroupPresence() {
if (groupPresenceBuilder_ == null) {
if (presenceConstraintsCase_ == 17) {
presenceConstraintsCase_ = 0;
presenceConstraints_ = null;
onChanged();
}
} else {
if (presenceConstraintsCase_ == 17) {
presenceConstraintsCase_ = 0;
presenceConstraints_ = null;
}
groupPresenceBuilder_.clear();
}
return this;
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
public org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.Builder getGroupPresenceBuilder() {
return getGroupPresenceFieldBuilder().getBuilder();
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeaturePresenceWithinGroupOrBuilder getGroupPresenceOrBuilder() {
if ((presenceConstraintsCase_ == 17) && (groupPresenceBuilder_ != null)) {
return groupPresenceBuilder_.getMessageOrBuilder();
} else {
if (presenceConstraintsCase_ == 17) {
return (org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_;
}
return org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.getDefaultInstance();
}
}
/**
*
* Only used in the context of a "group" context, e.g., inside a sequence.
*
*
* .tensorflow.metadata.v0.FeaturePresenceWithinGroup group_presence = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresenceWithinGroup, org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.Builder, org.tensorflow.metadata.v0.FeaturePresenceWithinGroupOrBuilder>
getGroupPresenceFieldBuilder() {
if (groupPresenceBuilder_ == null) {
if (!(presenceConstraintsCase_ == 17)) {
presenceConstraints_ = org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.getDefaultInstance();
}
groupPresenceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresenceWithinGroup, org.tensorflow.metadata.v0.FeaturePresenceWithinGroup.Builder, org.tensorflow.metadata.v0.FeaturePresenceWithinGroupOrBuilder>(
(org.tensorflow.metadata.v0.FeaturePresenceWithinGroup) presenceConstraints_,
getParentForChildren(),
isClean());
presenceConstraints_ = null;
}
presenceConstraintsCase_ = 17;
onChanged();
return groupPresenceBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FixedShape, org.tensorflow.metadata.v0.FixedShape.Builder, org.tensorflow.metadata.v0.FixedShapeOrBuilder> shapeBuilder_;
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
* @return Whether the shape field is set.
*/
@java.lang.Override
public boolean hasShape() {
return shapeTypeCase_ == 23;
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
* @return The shape.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FixedShape getShape() {
if (shapeBuilder_ == null) {
if (shapeTypeCase_ == 23) {
return (org.tensorflow.metadata.v0.FixedShape) shapeType_;
}
return org.tensorflow.metadata.v0.FixedShape.getDefaultInstance();
} else {
if (shapeTypeCase_ == 23) {
return shapeBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.FixedShape.getDefaultInstance();
}
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
public Builder setShape(org.tensorflow.metadata.v0.FixedShape value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shapeType_ = value;
onChanged();
} else {
shapeBuilder_.setMessage(value);
}
shapeTypeCase_ = 23;
return this;
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
public Builder setShape(
org.tensorflow.metadata.v0.FixedShape.Builder builderForValue) {
if (shapeBuilder_ == null) {
shapeType_ = builderForValue.build();
onChanged();
} else {
shapeBuilder_.setMessage(builderForValue.build());
}
shapeTypeCase_ = 23;
return this;
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
public Builder mergeShape(org.tensorflow.metadata.v0.FixedShape value) {
if (shapeBuilder_ == null) {
if (shapeTypeCase_ == 23 &&
shapeType_ != org.tensorflow.metadata.v0.FixedShape.getDefaultInstance()) {
shapeType_ = org.tensorflow.metadata.v0.FixedShape.newBuilder((org.tensorflow.metadata.v0.FixedShape) shapeType_)
.mergeFrom(value).buildPartial();
} else {
shapeType_ = value;
}
onChanged();
} else {
if (shapeTypeCase_ == 23) {
shapeBuilder_.mergeFrom(value);
} else {
shapeBuilder_.setMessage(value);
}
}
shapeTypeCase_ = 23;
return this;
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
public Builder clearShape() {
if (shapeBuilder_ == null) {
if (shapeTypeCase_ == 23) {
shapeTypeCase_ = 0;
shapeType_ = null;
onChanged();
}
} else {
if (shapeTypeCase_ == 23) {
shapeTypeCase_ = 0;
shapeType_ = null;
}
shapeBuilder_.clear();
}
return this;
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
public org.tensorflow.metadata.v0.FixedShape.Builder getShapeBuilder() {
return getShapeFieldBuilder().getBuilder();
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FixedShapeOrBuilder getShapeOrBuilder() {
if ((shapeTypeCase_ == 23) && (shapeBuilder_ != null)) {
return shapeBuilder_.getMessageOrBuilder();
} else {
if (shapeTypeCase_ == 23) {
return (org.tensorflow.metadata.v0.FixedShape) shapeType_;
}
return org.tensorflow.metadata.v0.FixedShape.getDefaultInstance();
}
}
/**
*
* The feature has a fixed shape corresponding to a multi-dimensional
* tensor.
*
*
* .tensorflow.metadata.v0.FixedShape shape = 23;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FixedShape, org.tensorflow.metadata.v0.FixedShape.Builder, org.tensorflow.metadata.v0.FixedShapeOrBuilder>
getShapeFieldBuilder() {
if (shapeBuilder_ == null) {
if (!(shapeTypeCase_ == 23)) {
shapeType_ = org.tensorflow.metadata.v0.FixedShape.getDefaultInstance();
}
shapeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FixedShape, org.tensorflow.metadata.v0.FixedShape.Builder, org.tensorflow.metadata.v0.FixedShapeOrBuilder>(
(org.tensorflow.metadata.v0.FixedShape) shapeType_,
getParentForChildren(),
isClean());
shapeType_ = null;
}
shapeTypeCase_ = 23;
onChanged();
return shapeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ValueCount, org.tensorflow.metadata.v0.ValueCount.Builder, org.tensorflow.metadata.v0.ValueCountOrBuilder> valueCountBuilder_;
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
* @return Whether the valueCount field is set.
*/
@java.lang.Override
public boolean hasValueCount() {
return shapeTypeCase_ == 5;
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
* @return The valueCount.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCount getValueCount() {
if (valueCountBuilder_ == null) {
if (shapeTypeCase_ == 5) {
return (org.tensorflow.metadata.v0.ValueCount) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCount.getDefaultInstance();
} else {
if (shapeTypeCase_ == 5) {
return valueCountBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.ValueCount.getDefaultInstance();
}
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
public Builder setValueCount(org.tensorflow.metadata.v0.ValueCount value) {
if (valueCountBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shapeType_ = value;
onChanged();
} else {
valueCountBuilder_.setMessage(value);
}
shapeTypeCase_ = 5;
return this;
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
public Builder setValueCount(
org.tensorflow.metadata.v0.ValueCount.Builder builderForValue) {
if (valueCountBuilder_ == null) {
shapeType_ = builderForValue.build();
onChanged();
} else {
valueCountBuilder_.setMessage(builderForValue.build());
}
shapeTypeCase_ = 5;
return this;
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
public Builder mergeValueCount(org.tensorflow.metadata.v0.ValueCount value) {
if (valueCountBuilder_ == null) {
if (shapeTypeCase_ == 5 &&
shapeType_ != org.tensorflow.metadata.v0.ValueCount.getDefaultInstance()) {
shapeType_ = org.tensorflow.metadata.v0.ValueCount.newBuilder((org.tensorflow.metadata.v0.ValueCount) shapeType_)
.mergeFrom(value).buildPartial();
} else {
shapeType_ = value;
}
onChanged();
} else {
if (shapeTypeCase_ == 5) {
valueCountBuilder_.mergeFrom(value);
} else {
valueCountBuilder_.setMessage(value);
}
}
shapeTypeCase_ = 5;
return this;
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
public Builder clearValueCount() {
if (valueCountBuilder_ == null) {
if (shapeTypeCase_ == 5) {
shapeTypeCase_ = 0;
shapeType_ = null;
onChanged();
}
} else {
if (shapeTypeCase_ == 5) {
shapeTypeCase_ = 0;
shapeType_ = null;
}
valueCountBuilder_.clear();
}
return this;
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
public org.tensorflow.metadata.v0.ValueCount.Builder getValueCountBuilder() {
return getValueCountFieldBuilder().getBuilder();
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCountOrBuilder getValueCountOrBuilder() {
if ((shapeTypeCase_ == 5) && (valueCountBuilder_ != null)) {
return valueCountBuilder_.getMessageOrBuilder();
} else {
if (shapeTypeCase_ == 5) {
return (org.tensorflow.metadata.v0.ValueCount) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCount.getDefaultInstance();
}
}
/**
*
* The feature doesn't have a well defined shape. All we know are limits on
* the minimum and maximum number of values.
*
*
* .tensorflow.metadata.v0.ValueCount value_count = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ValueCount, org.tensorflow.metadata.v0.ValueCount.Builder, org.tensorflow.metadata.v0.ValueCountOrBuilder>
getValueCountFieldBuilder() {
if (valueCountBuilder_ == null) {
if (!(shapeTypeCase_ == 5)) {
shapeType_ = org.tensorflow.metadata.v0.ValueCount.getDefaultInstance();
}
valueCountBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ValueCount, org.tensorflow.metadata.v0.ValueCount.Builder, org.tensorflow.metadata.v0.ValueCountOrBuilder>(
(org.tensorflow.metadata.v0.ValueCount) shapeType_,
getParentForChildren(),
isClean());
shapeType_ = null;
}
shapeTypeCase_ = 5;
onChanged();
return valueCountBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ValueCountList, org.tensorflow.metadata.v0.ValueCountList.Builder, org.tensorflow.metadata.v0.ValueCountListOrBuilder> valueCountsBuilder_;
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
* @return Whether the valueCounts field is set.
*/
@java.lang.Override
public boolean hasValueCounts() {
return shapeTypeCase_ == 32;
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
* @return The valueCounts.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCountList getValueCounts() {
if (valueCountsBuilder_ == null) {
if (shapeTypeCase_ == 32) {
return (org.tensorflow.metadata.v0.ValueCountList) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCountList.getDefaultInstance();
} else {
if (shapeTypeCase_ == 32) {
return valueCountsBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.ValueCountList.getDefaultInstance();
}
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
public Builder setValueCounts(org.tensorflow.metadata.v0.ValueCountList value) {
if (valueCountsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shapeType_ = value;
onChanged();
} else {
valueCountsBuilder_.setMessage(value);
}
shapeTypeCase_ = 32;
return this;
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
public Builder setValueCounts(
org.tensorflow.metadata.v0.ValueCountList.Builder builderForValue) {
if (valueCountsBuilder_ == null) {
shapeType_ = builderForValue.build();
onChanged();
} else {
valueCountsBuilder_.setMessage(builderForValue.build());
}
shapeTypeCase_ = 32;
return this;
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
public Builder mergeValueCounts(org.tensorflow.metadata.v0.ValueCountList value) {
if (valueCountsBuilder_ == null) {
if (shapeTypeCase_ == 32 &&
shapeType_ != org.tensorflow.metadata.v0.ValueCountList.getDefaultInstance()) {
shapeType_ = org.tensorflow.metadata.v0.ValueCountList.newBuilder((org.tensorflow.metadata.v0.ValueCountList) shapeType_)
.mergeFrom(value).buildPartial();
} else {
shapeType_ = value;
}
onChanged();
} else {
if (shapeTypeCase_ == 32) {
valueCountsBuilder_.mergeFrom(value);
} else {
valueCountsBuilder_.setMessage(value);
}
}
shapeTypeCase_ = 32;
return this;
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
public Builder clearValueCounts() {
if (valueCountsBuilder_ == null) {
if (shapeTypeCase_ == 32) {
shapeTypeCase_ = 0;
shapeType_ = null;
onChanged();
}
} else {
if (shapeTypeCase_ == 32) {
shapeTypeCase_ = 0;
shapeType_ = null;
}
valueCountsBuilder_.clear();
}
return this;
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
public org.tensorflow.metadata.v0.ValueCountList.Builder getValueCountsBuilder() {
return getValueCountsFieldBuilder().getBuilder();
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ValueCountListOrBuilder getValueCountsOrBuilder() {
if ((shapeTypeCase_ == 32) && (valueCountsBuilder_ != null)) {
return valueCountsBuilder_.getMessageOrBuilder();
} else {
if (shapeTypeCase_ == 32) {
return (org.tensorflow.metadata.v0.ValueCountList) shapeType_;
}
return org.tensorflow.metadata.v0.ValueCountList.getDefaultInstance();
}
}
/**
*
* Captures the same information as value_count but for features with
* nested values. A ValueCount is provided for each nest level.
*
*
* .tensorflow.metadata.v0.ValueCountList value_counts = 32;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ValueCountList, org.tensorflow.metadata.v0.ValueCountList.Builder, org.tensorflow.metadata.v0.ValueCountListOrBuilder>
getValueCountsFieldBuilder() {
if (valueCountsBuilder_ == null) {
if (!(shapeTypeCase_ == 32)) {
shapeType_ = org.tensorflow.metadata.v0.ValueCountList.getDefaultInstance();
}
valueCountsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ValueCountList, org.tensorflow.metadata.v0.ValueCountList.Builder, org.tensorflow.metadata.v0.ValueCountListOrBuilder>(
(org.tensorflow.metadata.v0.ValueCountList) shapeType_,
getParentForChildren(),
isClean());
shapeType_ = null;
}
shapeTypeCase_ = 32;
onChanged();
return valueCountsBuilder_;
}
private int type_ = 0;
/**
*
* Physical type of the feature's values.
* Note that you can have:
* type: BYTES
* int_domain: {
* min: 0
* max: 3
* }
* This would be a field that is syntactically BYTES (i.e. strings), but
* semantically an int, i.e. it would be "0", "1", "2", or "3".
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 6;
* @return Whether the type field is set.
*/
@java.lang.Override public boolean hasType() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Physical type of the feature's values.
* Note that you can have:
* type: BYTES
* int_domain: {
* min: 0
* max: 3
* }
* This would be a field that is syntactically BYTES (i.e. strings), but
* semantically an int, i.e. it would be "0", "1", "2", or "3".
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 6;
* @return The type.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FeatureType getType() {
org.tensorflow.metadata.v0.FeatureType result = org.tensorflow.metadata.v0.FeatureType.forNumber(type_);
return result == null ? org.tensorflow.metadata.v0.FeatureType.TYPE_UNKNOWN : result;
}
/**
*
* Physical type of the feature's values.
* Note that you can have:
* type: BYTES
* int_domain: {
* min: 0
* max: 3
* }
* This would be a field that is syntactically BYTES (i.e. strings), but
* semantically an int, i.e. it would be "0", "1", "2", or "3".
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 6;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.tensorflow.metadata.v0.FeatureType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Physical type of the feature's values.
* Note that you can have:
* type: BYTES
* int_domain: {
* min: 0
* max: 3
* }
* This would be a field that is syntactically BYTES (i.e. strings), but
* semantically an int, i.e. it would be "0", "1", "2", or "3".
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 6;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000080);
type_ = 0;
onChanged();
return this;
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @return Whether the domain field is set.
*/
@java.lang.Override
public boolean hasDomain() {
return domainInfoCase_ == 7;
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @return The domain.
*/
@java.lang.Override
public java.lang.String getDomain() {
java.lang.Object ref = "";
if (domainInfoCase_ == 7) {
ref = domainInfo_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (domainInfoCase_ == 7) {
if (bs.isValidUtf8()) {
domainInfo_ = s;
}
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @return The bytes for domain.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDomainBytes() {
java.lang.Object ref = "";
if (domainInfoCase_ == 7) {
ref = domainInfo_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (domainInfoCase_ == 7) {
domainInfo_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @param value The domain to set.
* @return This builder for chaining.
*/
public Builder setDomain(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
domainInfoCase_ = 7;
domainInfo_ = value;
onChanged();
return this;
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @return This builder for chaining.
*/
public Builder clearDomain() {
if (domainInfoCase_ == 7) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
return this;
}
/**
*
* Reference to a domain defined at the schema level.
*
*
* string domain = 7;
* @param value The bytes for domain to set.
* @return This builder for chaining.
*/
public Builder setDomainBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
domainInfoCase_ = 7;
domainInfo_ = value;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.IntDomain, org.tensorflow.metadata.v0.IntDomain.Builder, org.tensorflow.metadata.v0.IntDomainOrBuilder> intDomainBuilder_;
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
* @return Whether the intDomain field is set.
*/
@java.lang.Override
public boolean hasIntDomain() {
return domainInfoCase_ == 9;
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
* @return The intDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.IntDomain getIntDomain() {
if (intDomainBuilder_ == null) {
if (domainInfoCase_ == 9) {
return (org.tensorflow.metadata.v0.IntDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.IntDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 9) {
return intDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.IntDomain.getDefaultInstance();
}
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
public Builder setIntDomain(org.tensorflow.metadata.v0.IntDomain value) {
if (intDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
intDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 9;
return this;
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
public Builder setIntDomain(
org.tensorflow.metadata.v0.IntDomain.Builder builderForValue) {
if (intDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
intDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 9;
return this;
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
public Builder mergeIntDomain(org.tensorflow.metadata.v0.IntDomain value) {
if (intDomainBuilder_ == null) {
if (domainInfoCase_ == 9 &&
domainInfo_ != org.tensorflow.metadata.v0.IntDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.IntDomain.newBuilder((org.tensorflow.metadata.v0.IntDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 9) {
intDomainBuilder_.mergeFrom(value);
} else {
intDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 9;
return this;
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
public Builder clearIntDomain() {
if (intDomainBuilder_ == null) {
if (domainInfoCase_ == 9) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 9) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
intDomainBuilder_.clear();
}
return this;
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
public org.tensorflow.metadata.v0.IntDomain.Builder getIntDomainBuilder() {
return getIntDomainFieldBuilder().getBuilder();
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.IntDomainOrBuilder getIntDomainOrBuilder() {
if ((domainInfoCase_ == 9) && (intDomainBuilder_ != null)) {
return intDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 9) {
return (org.tensorflow.metadata.v0.IntDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.IntDomain.getDefaultInstance();
}
}
/**
*
* Inline definitions of domains.
*
*
* .tensorflow.metadata.v0.IntDomain int_domain = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.IntDomain, org.tensorflow.metadata.v0.IntDomain.Builder, org.tensorflow.metadata.v0.IntDomainOrBuilder>
getIntDomainFieldBuilder() {
if (intDomainBuilder_ == null) {
if (!(domainInfoCase_ == 9)) {
domainInfo_ = org.tensorflow.metadata.v0.IntDomain.getDefaultInstance();
}
intDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.IntDomain, org.tensorflow.metadata.v0.IntDomain.Builder, org.tensorflow.metadata.v0.IntDomainOrBuilder>(
(org.tensorflow.metadata.v0.IntDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 9;
onChanged();
return intDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FloatDomain, org.tensorflow.metadata.v0.FloatDomain.Builder, org.tensorflow.metadata.v0.FloatDomainOrBuilder> floatDomainBuilder_;
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
* @return Whether the floatDomain field is set.
*/
@java.lang.Override
public boolean hasFloatDomain() {
return domainInfoCase_ == 10;
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
* @return The floatDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FloatDomain getFloatDomain() {
if (floatDomainBuilder_ == null) {
if (domainInfoCase_ == 10) {
return (org.tensorflow.metadata.v0.FloatDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.FloatDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 10) {
return floatDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.FloatDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
public Builder setFloatDomain(org.tensorflow.metadata.v0.FloatDomain value) {
if (floatDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
floatDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 10;
return this;
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
public Builder setFloatDomain(
org.tensorflow.metadata.v0.FloatDomain.Builder builderForValue) {
if (floatDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
floatDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 10;
return this;
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
public Builder mergeFloatDomain(org.tensorflow.metadata.v0.FloatDomain value) {
if (floatDomainBuilder_ == null) {
if (domainInfoCase_ == 10 &&
domainInfo_ != org.tensorflow.metadata.v0.FloatDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.FloatDomain.newBuilder((org.tensorflow.metadata.v0.FloatDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 10) {
floatDomainBuilder_.mergeFrom(value);
} else {
floatDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 10;
return this;
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
public Builder clearFloatDomain() {
if (floatDomainBuilder_ == null) {
if (domainInfoCase_ == 10) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 10) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
floatDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
public org.tensorflow.metadata.v0.FloatDomain.Builder getFloatDomainBuilder() {
return getFloatDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FloatDomainOrBuilder getFloatDomainOrBuilder() {
if ((domainInfoCase_ == 10) && (floatDomainBuilder_ != null)) {
return floatDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 10) {
return (org.tensorflow.metadata.v0.FloatDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.FloatDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.FloatDomain float_domain = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FloatDomain, org.tensorflow.metadata.v0.FloatDomain.Builder, org.tensorflow.metadata.v0.FloatDomainOrBuilder>
getFloatDomainFieldBuilder() {
if (floatDomainBuilder_ == null) {
if (!(domainInfoCase_ == 10)) {
domainInfo_ = org.tensorflow.metadata.v0.FloatDomain.getDefaultInstance();
}
floatDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FloatDomain, org.tensorflow.metadata.v0.FloatDomain.Builder, org.tensorflow.metadata.v0.FloatDomainOrBuilder>(
(org.tensorflow.metadata.v0.FloatDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 10;
onChanged();
return floatDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.StringDomain, org.tensorflow.metadata.v0.StringDomain.Builder, org.tensorflow.metadata.v0.StringDomainOrBuilder> stringDomainBuilder_;
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
* @return Whether the stringDomain field is set.
*/
@java.lang.Override
public boolean hasStringDomain() {
return domainInfoCase_ == 11;
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
* @return The stringDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StringDomain getStringDomain() {
if (stringDomainBuilder_ == null) {
if (domainInfoCase_ == 11) {
return (org.tensorflow.metadata.v0.StringDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StringDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 11) {
return stringDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.StringDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
public Builder setStringDomain(org.tensorflow.metadata.v0.StringDomain value) {
if (stringDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
stringDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 11;
return this;
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
public Builder setStringDomain(
org.tensorflow.metadata.v0.StringDomain.Builder builderForValue) {
if (stringDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
stringDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 11;
return this;
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
public Builder mergeStringDomain(org.tensorflow.metadata.v0.StringDomain value) {
if (stringDomainBuilder_ == null) {
if (domainInfoCase_ == 11 &&
domainInfo_ != org.tensorflow.metadata.v0.StringDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.StringDomain.newBuilder((org.tensorflow.metadata.v0.StringDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 11) {
stringDomainBuilder_.mergeFrom(value);
} else {
stringDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 11;
return this;
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
public Builder clearStringDomain() {
if (stringDomainBuilder_ == null) {
if (domainInfoCase_ == 11) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 11) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
stringDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
public org.tensorflow.metadata.v0.StringDomain.Builder getStringDomainBuilder() {
return getStringDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StringDomainOrBuilder getStringDomainOrBuilder() {
if ((domainInfoCase_ == 11) && (stringDomainBuilder_ != null)) {
return stringDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 11) {
return (org.tensorflow.metadata.v0.StringDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StringDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.StringDomain string_domain = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.StringDomain, org.tensorflow.metadata.v0.StringDomain.Builder, org.tensorflow.metadata.v0.StringDomainOrBuilder>
getStringDomainFieldBuilder() {
if (stringDomainBuilder_ == null) {
if (!(domainInfoCase_ == 11)) {
domainInfo_ = org.tensorflow.metadata.v0.StringDomain.getDefaultInstance();
}
stringDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.StringDomain, org.tensorflow.metadata.v0.StringDomain.Builder, org.tensorflow.metadata.v0.StringDomainOrBuilder>(
(org.tensorflow.metadata.v0.StringDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 11;
onChanged();
return stringDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BoolDomain, org.tensorflow.metadata.v0.BoolDomain.Builder, org.tensorflow.metadata.v0.BoolDomainOrBuilder> boolDomainBuilder_;
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
* @return Whether the boolDomain field is set.
*/
@java.lang.Override
public boolean hasBoolDomain() {
return domainInfoCase_ == 13;
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
* @return The boolDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BoolDomain getBoolDomain() {
if (boolDomainBuilder_ == null) {
if (domainInfoCase_ == 13) {
return (org.tensorflow.metadata.v0.BoolDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.BoolDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 13) {
return boolDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.BoolDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
public Builder setBoolDomain(org.tensorflow.metadata.v0.BoolDomain value) {
if (boolDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
boolDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 13;
return this;
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
public Builder setBoolDomain(
org.tensorflow.metadata.v0.BoolDomain.Builder builderForValue) {
if (boolDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
boolDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 13;
return this;
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
public Builder mergeBoolDomain(org.tensorflow.metadata.v0.BoolDomain value) {
if (boolDomainBuilder_ == null) {
if (domainInfoCase_ == 13 &&
domainInfo_ != org.tensorflow.metadata.v0.BoolDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.BoolDomain.newBuilder((org.tensorflow.metadata.v0.BoolDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 13) {
boolDomainBuilder_.mergeFrom(value);
} else {
boolDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 13;
return this;
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
public Builder clearBoolDomain() {
if (boolDomainBuilder_ == null) {
if (domainInfoCase_ == 13) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 13) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
boolDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
public org.tensorflow.metadata.v0.BoolDomain.Builder getBoolDomainBuilder() {
return getBoolDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.BoolDomainOrBuilder getBoolDomainOrBuilder() {
if ((domainInfoCase_ == 13) && (boolDomainBuilder_ != null)) {
return boolDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 13) {
return (org.tensorflow.metadata.v0.BoolDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.BoolDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.BoolDomain bool_domain = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BoolDomain, org.tensorflow.metadata.v0.BoolDomain.Builder, org.tensorflow.metadata.v0.BoolDomainOrBuilder>
getBoolDomainFieldBuilder() {
if (boolDomainBuilder_ == null) {
if (!(domainInfoCase_ == 13)) {
domainInfo_ = org.tensorflow.metadata.v0.BoolDomain.getDefaultInstance();
}
boolDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.BoolDomain, org.tensorflow.metadata.v0.BoolDomain.Builder, org.tensorflow.metadata.v0.BoolDomainOrBuilder>(
(org.tensorflow.metadata.v0.BoolDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 13;
onChanged();
return boolDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.StructDomain, org.tensorflow.metadata.v0.StructDomain.Builder, org.tensorflow.metadata.v0.StructDomainOrBuilder> structDomainBuilder_;
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
* @return Whether the structDomain field is set.
*/
@java.lang.Override
public boolean hasStructDomain() {
return domainInfoCase_ == 29;
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
* @return The structDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StructDomain getStructDomain() {
if (structDomainBuilder_ == null) {
if (domainInfoCase_ == 29) {
return (org.tensorflow.metadata.v0.StructDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StructDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 29) {
return structDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.StructDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
public Builder setStructDomain(org.tensorflow.metadata.v0.StructDomain value) {
if (structDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
structDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 29;
return this;
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
public Builder setStructDomain(
org.tensorflow.metadata.v0.StructDomain.Builder builderForValue) {
if (structDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
structDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 29;
return this;
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
public Builder mergeStructDomain(org.tensorflow.metadata.v0.StructDomain value) {
if (structDomainBuilder_ == null) {
if (domainInfoCase_ == 29 &&
domainInfo_ != org.tensorflow.metadata.v0.StructDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.StructDomain.newBuilder((org.tensorflow.metadata.v0.StructDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 29) {
structDomainBuilder_.mergeFrom(value);
} else {
structDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 29;
return this;
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
public Builder clearStructDomain() {
if (structDomainBuilder_ == null) {
if (domainInfoCase_ == 29) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 29) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
structDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
public org.tensorflow.metadata.v0.StructDomain.Builder getStructDomainBuilder() {
return getStructDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.StructDomainOrBuilder getStructDomainOrBuilder() {
if ((domainInfoCase_ == 29) && (structDomainBuilder_ != null)) {
return structDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 29) {
return (org.tensorflow.metadata.v0.StructDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.StructDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.StructDomain struct_domain = 29;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.StructDomain, org.tensorflow.metadata.v0.StructDomain.Builder, org.tensorflow.metadata.v0.StructDomainOrBuilder>
getStructDomainFieldBuilder() {
if (structDomainBuilder_ == null) {
if (!(domainInfoCase_ == 29)) {
domainInfo_ = org.tensorflow.metadata.v0.StructDomain.getDefaultInstance();
}
structDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.StructDomain, org.tensorflow.metadata.v0.StructDomain.Builder, org.tensorflow.metadata.v0.StructDomainOrBuilder>(
(org.tensorflow.metadata.v0.StructDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 29;
onChanged();
return structDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.NaturalLanguageDomain, org.tensorflow.metadata.v0.NaturalLanguageDomain.Builder, org.tensorflow.metadata.v0.NaturalLanguageDomainOrBuilder> naturalLanguageDomainBuilder_;
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
* @return Whether the naturalLanguageDomain field is set.
*/
@java.lang.Override
public boolean hasNaturalLanguageDomain() {
return domainInfoCase_ == 24;
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
* @return The naturalLanguageDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.NaturalLanguageDomain getNaturalLanguageDomain() {
if (naturalLanguageDomainBuilder_ == null) {
if (domainInfoCase_ == 24) {
return (org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.NaturalLanguageDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 24) {
return naturalLanguageDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.NaturalLanguageDomain.getDefaultInstance();
}
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
public Builder setNaturalLanguageDomain(org.tensorflow.metadata.v0.NaturalLanguageDomain value) {
if (naturalLanguageDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
naturalLanguageDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 24;
return this;
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
public Builder setNaturalLanguageDomain(
org.tensorflow.metadata.v0.NaturalLanguageDomain.Builder builderForValue) {
if (naturalLanguageDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
naturalLanguageDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 24;
return this;
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
public Builder mergeNaturalLanguageDomain(org.tensorflow.metadata.v0.NaturalLanguageDomain value) {
if (naturalLanguageDomainBuilder_ == null) {
if (domainInfoCase_ == 24 &&
domainInfo_ != org.tensorflow.metadata.v0.NaturalLanguageDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.NaturalLanguageDomain.newBuilder((org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 24) {
naturalLanguageDomainBuilder_.mergeFrom(value);
} else {
naturalLanguageDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 24;
return this;
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
public Builder clearNaturalLanguageDomain() {
if (naturalLanguageDomainBuilder_ == null) {
if (domainInfoCase_ == 24) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 24) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
naturalLanguageDomainBuilder_.clear();
}
return this;
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
public org.tensorflow.metadata.v0.NaturalLanguageDomain.Builder getNaturalLanguageDomainBuilder() {
return getNaturalLanguageDomainFieldBuilder().getBuilder();
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.NaturalLanguageDomainOrBuilder getNaturalLanguageDomainOrBuilder() {
if ((domainInfoCase_ == 24) && (naturalLanguageDomainBuilder_ != null)) {
return naturalLanguageDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 24) {
return (org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.NaturalLanguageDomain.getDefaultInstance();
}
}
/**
*
* Supported semantic domains.
*
*
* .tensorflow.metadata.v0.NaturalLanguageDomain natural_language_domain = 24;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.NaturalLanguageDomain, org.tensorflow.metadata.v0.NaturalLanguageDomain.Builder, org.tensorflow.metadata.v0.NaturalLanguageDomainOrBuilder>
getNaturalLanguageDomainFieldBuilder() {
if (naturalLanguageDomainBuilder_ == null) {
if (!(domainInfoCase_ == 24)) {
domainInfo_ = org.tensorflow.metadata.v0.NaturalLanguageDomain.getDefaultInstance();
}
naturalLanguageDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.NaturalLanguageDomain, org.tensorflow.metadata.v0.NaturalLanguageDomain.Builder, org.tensorflow.metadata.v0.NaturalLanguageDomainOrBuilder>(
(org.tensorflow.metadata.v0.NaturalLanguageDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 24;
onChanged();
return naturalLanguageDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ImageDomain, org.tensorflow.metadata.v0.ImageDomain.Builder, org.tensorflow.metadata.v0.ImageDomainOrBuilder> imageDomainBuilder_;
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
* @return Whether the imageDomain field is set.
*/
@java.lang.Override
public boolean hasImageDomain() {
return domainInfoCase_ == 25;
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
* @return The imageDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ImageDomain getImageDomain() {
if (imageDomainBuilder_ == null) {
if (domainInfoCase_ == 25) {
return (org.tensorflow.metadata.v0.ImageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.ImageDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 25) {
return imageDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.ImageDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
public Builder setImageDomain(org.tensorflow.metadata.v0.ImageDomain value) {
if (imageDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
imageDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 25;
return this;
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
public Builder setImageDomain(
org.tensorflow.metadata.v0.ImageDomain.Builder builderForValue) {
if (imageDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
imageDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 25;
return this;
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
public Builder mergeImageDomain(org.tensorflow.metadata.v0.ImageDomain value) {
if (imageDomainBuilder_ == null) {
if (domainInfoCase_ == 25 &&
domainInfo_ != org.tensorflow.metadata.v0.ImageDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.ImageDomain.newBuilder((org.tensorflow.metadata.v0.ImageDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 25) {
imageDomainBuilder_.mergeFrom(value);
} else {
imageDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 25;
return this;
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
public Builder clearImageDomain() {
if (imageDomainBuilder_ == null) {
if (domainInfoCase_ == 25) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 25) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
imageDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
public org.tensorflow.metadata.v0.ImageDomain.Builder getImageDomainBuilder() {
return getImageDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.ImageDomainOrBuilder getImageDomainOrBuilder() {
if ((domainInfoCase_ == 25) && (imageDomainBuilder_ != null)) {
return imageDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 25) {
return (org.tensorflow.metadata.v0.ImageDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.ImageDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.ImageDomain image_domain = 25;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ImageDomain, org.tensorflow.metadata.v0.ImageDomain.Builder, org.tensorflow.metadata.v0.ImageDomainOrBuilder>
getImageDomainFieldBuilder() {
if (imageDomainBuilder_ == null) {
if (!(domainInfoCase_ == 25)) {
domainInfo_ = org.tensorflow.metadata.v0.ImageDomain.getDefaultInstance();
}
imageDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.ImageDomain, org.tensorflow.metadata.v0.ImageDomain.Builder, org.tensorflow.metadata.v0.ImageDomainOrBuilder>(
(org.tensorflow.metadata.v0.ImageDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 25;
onChanged();
return imageDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.MIDDomain, org.tensorflow.metadata.v0.MIDDomain.Builder, org.tensorflow.metadata.v0.MIDDomainOrBuilder> midDomainBuilder_;
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
* @return Whether the midDomain field is set.
*/
@java.lang.Override
public boolean hasMidDomain() {
return domainInfoCase_ == 26;
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
* @return The midDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.MIDDomain getMidDomain() {
if (midDomainBuilder_ == null) {
if (domainInfoCase_ == 26) {
return (org.tensorflow.metadata.v0.MIDDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.MIDDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 26) {
return midDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.MIDDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
public Builder setMidDomain(org.tensorflow.metadata.v0.MIDDomain value) {
if (midDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
midDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 26;
return this;
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
public Builder setMidDomain(
org.tensorflow.metadata.v0.MIDDomain.Builder builderForValue) {
if (midDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
midDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 26;
return this;
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
public Builder mergeMidDomain(org.tensorflow.metadata.v0.MIDDomain value) {
if (midDomainBuilder_ == null) {
if (domainInfoCase_ == 26 &&
domainInfo_ != org.tensorflow.metadata.v0.MIDDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.MIDDomain.newBuilder((org.tensorflow.metadata.v0.MIDDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 26) {
midDomainBuilder_.mergeFrom(value);
} else {
midDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 26;
return this;
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
public Builder clearMidDomain() {
if (midDomainBuilder_ == null) {
if (domainInfoCase_ == 26) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 26) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
midDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
public org.tensorflow.metadata.v0.MIDDomain.Builder getMidDomainBuilder() {
return getMidDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.MIDDomainOrBuilder getMidDomainOrBuilder() {
if ((domainInfoCase_ == 26) && (midDomainBuilder_ != null)) {
return midDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 26) {
return (org.tensorflow.metadata.v0.MIDDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.MIDDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.MIDDomain mid_domain = 26;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.MIDDomain, org.tensorflow.metadata.v0.MIDDomain.Builder, org.tensorflow.metadata.v0.MIDDomainOrBuilder>
getMidDomainFieldBuilder() {
if (midDomainBuilder_ == null) {
if (!(domainInfoCase_ == 26)) {
domainInfo_ = org.tensorflow.metadata.v0.MIDDomain.getDefaultInstance();
}
midDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.MIDDomain, org.tensorflow.metadata.v0.MIDDomain.Builder, org.tensorflow.metadata.v0.MIDDomainOrBuilder>(
(org.tensorflow.metadata.v0.MIDDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 26;
onChanged();
return midDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.URLDomain, org.tensorflow.metadata.v0.URLDomain.Builder, org.tensorflow.metadata.v0.URLDomainOrBuilder> urlDomainBuilder_;
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
* @return Whether the urlDomain field is set.
*/
@java.lang.Override
public boolean hasUrlDomain() {
return domainInfoCase_ == 27;
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
* @return The urlDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.URLDomain getUrlDomain() {
if (urlDomainBuilder_ == null) {
if (domainInfoCase_ == 27) {
return (org.tensorflow.metadata.v0.URLDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.URLDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 27) {
return urlDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.URLDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
public Builder setUrlDomain(org.tensorflow.metadata.v0.URLDomain value) {
if (urlDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
urlDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 27;
return this;
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
public Builder setUrlDomain(
org.tensorflow.metadata.v0.URLDomain.Builder builderForValue) {
if (urlDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
urlDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 27;
return this;
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
public Builder mergeUrlDomain(org.tensorflow.metadata.v0.URLDomain value) {
if (urlDomainBuilder_ == null) {
if (domainInfoCase_ == 27 &&
domainInfo_ != org.tensorflow.metadata.v0.URLDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.URLDomain.newBuilder((org.tensorflow.metadata.v0.URLDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 27) {
urlDomainBuilder_.mergeFrom(value);
} else {
urlDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 27;
return this;
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
public Builder clearUrlDomain() {
if (urlDomainBuilder_ == null) {
if (domainInfoCase_ == 27) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 27) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
urlDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
public org.tensorflow.metadata.v0.URLDomain.Builder getUrlDomainBuilder() {
return getUrlDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.URLDomainOrBuilder getUrlDomainOrBuilder() {
if ((domainInfoCase_ == 27) && (urlDomainBuilder_ != null)) {
return urlDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 27) {
return (org.tensorflow.metadata.v0.URLDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.URLDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.URLDomain url_domain = 27;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.URLDomain, org.tensorflow.metadata.v0.URLDomain.Builder, org.tensorflow.metadata.v0.URLDomainOrBuilder>
getUrlDomainFieldBuilder() {
if (urlDomainBuilder_ == null) {
if (!(domainInfoCase_ == 27)) {
domainInfo_ = org.tensorflow.metadata.v0.URLDomain.getDefaultInstance();
}
urlDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.URLDomain, org.tensorflow.metadata.v0.URLDomain.Builder, org.tensorflow.metadata.v0.URLDomainOrBuilder>(
(org.tensorflow.metadata.v0.URLDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 27;
onChanged();
return urlDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.TimeDomain, org.tensorflow.metadata.v0.TimeDomain.Builder, org.tensorflow.metadata.v0.TimeDomainOrBuilder> timeDomainBuilder_;
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
* @return Whether the timeDomain field is set.
*/
@java.lang.Override
public boolean hasTimeDomain() {
return domainInfoCase_ == 28;
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
* @return The timeDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeDomain getTimeDomain() {
if (timeDomainBuilder_ == null) {
if (domainInfoCase_ == 28) {
return (org.tensorflow.metadata.v0.TimeDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 28) {
return timeDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.TimeDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
public Builder setTimeDomain(org.tensorflow.metadata.v0.TimeDomain value) {
if (timeDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
timeDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 28;
return this;
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
public Builder setTimeDomain(
org.tensorflow.metadata.v0.TimeDomain.Builder builderForValue) {
if (timeDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
timeDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 28;
return this;
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
public Builder mergeTimeDomain(org.tensorflow.metadata.v0.TimeDomain value) {
if (timeDomainBuilder_ == null) {
if (domainInfoCase_ == 28 &&
domainInfo_ != org.tensorflow.metadata.v0.TimeDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.TimeDomain.newBuilder((org.tensorflow.metadata.v0.TimeDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 28) {
timeDomainBuilder_.mergeFrom(value);
} else {
timeDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 28;
return this;
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
public Builder clearTimeDomain() {
if (timeDomainBuilder_ == null) {
if (domainInfoCase_ == 28) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 28) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
timeDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
public org.tensorflow.metadata.v0.TimeDomain.Builder getTimeDomainBuilder() {
return getTimeDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeDomainOrBuilder getTimeDomainOrBuilder() {
if ((domainInfoCase_ == 28) && (timeDomainBuilder_ != null)) {
return timeDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 28) {
return (org.tensorflow.metadata.v0.TimeDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.TimeDomain time_domain = 28;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.TimeDomain, org.tensorflow.metadata.v0.TimeDomain.Builder, org.tensorflow.metadata.v0.TimeDomainOrBuilder>
getTimeDomainFieldBuilder() {
if (timeDomainBuilder_ == null) {
if (!(domainInfoCase_ == 28)) {
domainInfo_ = org.tensorflow.metadata.v0.TimeDomain.getDefaultInstance();
}
timeDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.TimeDomain, org.tensorflow.metadata.v0.TimeDomain.Builder, org.tensorflow.metadata.v0.TimeDomainOrBuilder>(
(org.tensorflow.metadata.v0.TimeDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 28;
onChanged();
return timeDomainBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.TimeOfDayDomain, org.tensorflow.metadata.v0.TimeOfDayDomain.Builder, org.tensorflow.metadata.v0.TimeOfDayDomainOrBuilder> timeOfDayDomainBuilder_;
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
* @return Whether the timeOfDayDomain field is set.
*/
@java.lang.Override
public boolean hasTimeOfDayDomain() {
return domainInfoCase_ == 30;
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
* @return The timeOfDayDomain.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeOfDayDomain getTimeOfDayDomain() {
if (timeOfDayDomainBuilder_ == null) {
if (domainInfoCase_ == 30) {
return (org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeOfDayDomain.getDefaultInstance();
} else {
if (domainInfoCase_ == 30) {
return timeOfDayDomainBuilder_.getMessage();
}
return org.tensorflow.metadata.v0.TimeOfDayDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
public Builder setTimeOfDayDomain(org.tensorflow.metadata.v0.TimeOfDayDomain value) {
if (timeOfDayDomainBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
domainInfo_ = value;
onChanged();
} else {
timeOfDayDomainBuilder_.setMessage(value);
}
domainInfoCase_ = 30;
return this;
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
public Builder setTimeOfDayDomain(
org.tensorflow.metadata.v0.TimeOfDayDomain.Builder builderForValue) {
if (timeOfDayDomainBuilder_ == null) {
domainInfo_ = builderForValue.build();
onChanged();
} else {
timeOfDayDomainBuilder_.setMessage(builderForValue.build());
}
domainInfoCase_ = 30;
return this;
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
public Builder mergeTimeOfDayDomain(org.tensorflow.metadata.v0.TimeOfDayDomain value) {
if (timeOfDayDomainBuilder_ == null) {
if (domainInfoCase_ == 30 &&
domainInfo_ != org.tensorflow.metadata.v0.TimeOfDayDomain.getDefaultInstance()) {
domainInfo_ = org.tensorflow.metadata.v0.TimeOfDayDomain.newBuilder((org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_)
.mergeFrom(value).buildPartial();
} else {
domainInfo_ = value;
}
onChanged();
} else {
if (domainInfoCase_ == 30) {
timeOfDayDomainBuilder_.mergeFrom(value);
} else {
timeOfDayDomainBuilder_.setMessage(value);
}
}
domainInfoCase_ = 30;
return this;
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
public Builder clearTimeOfDayDomain() {
if (timeOfDayDomainBuilder_ == null) {
if (domainInfoCase_ == 30) {
domainInfoCase_ = 0;
domainInfo_ = null;
onChanged();
}
} else {
if (domainInfoCase_ == 30) {
domainInfoCase_ = 0;
domainInfo_ = null;
}
timeOfDayDomainBuilder_.clear();
}
return this;
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
public org.tensorflow.metadata.v0.TimeOfDayDomain.Builder getTimeOfDayDomainBuilder() {
return getTimeOfDayDomainFieldBuilder().getBuilder();
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.TimeOfDayDomainOrBuilder getTimeOfDayDomainOrBuilder() {
if ((domainInfoCase_ == 30) && (timeOfDayDomainBuilder_ != null)) {
return timeOfDayDomainBuilder_.getMessageOrBuilder();
} else {
if (domainInfoCase_ == 30) {
return (org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_;
}
return org.tensorflow.metadata.v0.TimeOfDayDomain.getDefaultInstance();
}
}
/**
* .tensorflow.metadata.v0.TimeOfDayDomain time_of_day_domain = 30;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.TimeOfDayDomain, org.tensorflow.metadata.v0.TimeOfDayDomain.Builder, org.tensorflow.metadata.v0.TimeOfDayDomainOrBuilder>
getTimeOfDayDomainFieldBuilder() {
if (timeOfDayDomainBuilder_ == null) {
if (!(domainInfoCase_ == 30)) {
domainInfo_ = org.tensorflow.metadata.v0.TimeOfDayDomain.getDefaultInstance();
}
timeOfDayDomainBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.TimeOfDayDomain, org.tensorflow.metadata.v0.TimeOfDayDomain.Builder, org.tensorflow.metadata.v0.TimeOfDayDomainOrBuilder>(
(org.tensorflow.metadata.v0.TimeOfDayDomain) domainInfo_,
getParentForChildren(),
isClean());
domainInfo_ = null;
}
domainInfoCase_ = 30;
onChanged();
return timeOfDayDomainBuilder_;
}
private org.tensorflow.metadata.v0.DistributionConstraints distributionConstraints_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.DistributionConstraints, org.tensorflow.metadata.v0.DistributionConstraints.Builder, org.tensorflow.metadata.v0.DistributionConstraintsOrBuilder> distributionConstraintsBuilder_;
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
* @return Whether the distributionConstraints field is set.
*/
public boolean hasDistributionConstraints() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
* @return The distributionConstraints.
*/
public org.tensorflow.metadata.v0.DistributionConstraints getDistributionConstraints() {
if (distributionConstraintsBuilder_ == null) {
return distributionConstraints_ == null ? org.tensorflow.metadata.v0.DistributionConstraints.getDefaultInstance() : distributionConstraints_;
} else {
return distributionConstraintsBuilder_.getMessage();
}
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
public Builder setDistributionConstraints(org.tensorflow.metadata.v0.DistributionConstraints value) {
if (distributionConstraintsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
distributionConstraints_ = value;
} else {
distributionConstraintsBuilder_.setMessage(value);
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
public Builder setDistributionConstraints(
org.tensorflow.metadata.v0.DistributionConstraints.Builder builderForValue) {
if (distributionConstraintsBuilder_ == null) {
distributionConstraints_ = builderForValue.build();
} else {
distributionConstraintsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
public Builder mergeDistributionConstraints(org.tensorflow.metadata.v0.DistributionConstraints value) {
if (distributionConstraintsBuilder_ == null) {
if (((bitField0_ & 0x00100000) != 0) &&
distributionConstraints_ != null &&
distributionConstraints_ != org.tensorflow.metadata.v0.DistributionConstraints.getDefaultInstance()) {
getDistributionConstraintsBuilder().mergeFrom(value);
} else {
distributionConstraints_ = value;
}
} else {
distributionConstraintsBuilder_.mergeFrom(value);
}
if (distributionConstraints_ != null) {
bitField0_ |= 0x00100000;
onChanged();
}
return this;
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
public Builder clearDistributionConstraints() {
bitField0_ = (bitField0_ & ~0x00100000);
distributionConstraints_ = null;
if (distributionConstraintsBuilder_ != null) {
distributionConstraintsBuilder_.dispose();
distributionConstraintsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
public org.tensorflow.metadata.v0.DistributionConstraints.Builder getDistributionConstraintsBuilder() {
bitField0_ |= 0x00100000;
onChanged();
return getDistributionConstraintsFieldBuilder().getBuilder();
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
public org.tensorflow.metadata.v0.DistributionConstraintsOrBuilder getDistributionConstraintsOrBuilder() {
if (distributionConstraintsBuilder_ != null) {
return distributionConstraintsBuilder_.getMessageOrBuilder();
} else {
return distributionConstraints_ == null ?
org.tensorflow.metadata.v0.DistributionConstraints.getDefaultInstance() : distributionConstraints_;
}
}
/**
*
* Constraints on the distribution of the feature values.
* Only supported for StringDomains.
*
*
* optional .tensorflow.metadata.v0.DistributionConstraints distribution_constraints = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.DistributionConstraints, org.tensorflow.metadata.v0.DistributionConstraints.Builder, org.tensorflow.metadata.v0.DistributionConstraintsOrBuilder>
getDistributionConstraintsFieldBuilder() {
if (distributionConstraintsBuilder_ == null) {
distributionConstraintsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.DistributionConstraints, org.tensorflow.metadata.v0.DistributionConstraints.Builder, org.tensorflow.metadata.v0.DistributionConstraintsOrBuilder>(
getDistributionConstraints(),
getParentForChildren(),
isClean());
distributionConstraints_ = null;
}
return distributionConstraintsBuilder_;
}
private org.tensorflow.metadata.v0.Annotation annotation_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Annotation, org.tensorflow.metadata.v0.Annotation.Builder, org.tensorflow.metadata.v0.AnnotationOrBuilder> annotationBuilder_;
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
* @return Whether the annotation field is set.
*/
public boolean hasAnnotation() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
* @return The annotation.
*/
public org.tensorflow.metadata.v0.Annotation getAnnotation() {
if (annotationBuilder_ == null) {
return annotation_ == null ? org.tensorflow.metadata.v0.Annotation.getDefaultInstance() : annotation_;
} else {
return annotationBuilder_.getMessage();
}
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
public Builder setAnnotation(org.tensorflow.metadata.v0.Annotation value) {
if (annotationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
annotation_ = value;
} else {
annotationBuilder_.setMessage(value);
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
public Builder setAnnotation(
org.tensorflow.metadata.v0.Annotation.Builder builderForValue) {
if (annotationBuilder_ == null) {
annotation_ = builderForValue.build();
} else {
annotationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
public Builder mergeAnnotation(org.tensorflow.metadata.v0.Annotation value) {
if (annotationBuilder_ == null) {
if (((bitField0_ & 0x00200000) != 0) &&
annotation_ != null &&
annotation_ != org.tensorflow.metadata.v0.Annotation.getDefaultInstance()) {
getAnnotationBuilder().mergeFrom(value);
} else {
annotation_ = value;
}
} else {
annotationBuilder_.mergeFrom(value);
}
if (annotation_ != null) {
bitField0_ |= 0x00200000;
onChanged();
}
return this;
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
public Builder clearAnnotation() {
bitField0_ = (bitField0_ & ~0x00200000);
annotation_ = null;
if (annotationBuilder_ != null) {
annotationBuilder_.dispose();
annotationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
public org.tensorflow.metadata.v0.Annotation.Builder getAnnotationBuilder() {
bitField0_ |= 0x00200000;
onChanged();
return getAnnotationFieldBuilder().getBuilder();
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
public org.tensorflow.metadata.v0.AnnotationOrBuilder getAnnotationOrBuilder() {
if (annotationBuilder_ != null) {
return annotationBuilder_.getMessageOrBuilder();
} else {
return annotation_ == null ?
org.tensorflow.metadata.v0.Annotation.getDefaultInstance() : annotation_;
}
}
/**
*
* Additional information about the feature for documentation purpose.
*
*
* optional .tensorflow.metadata.v0.Annotation annotation = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Annotation, org.tensorflow.metadata.v0.Annotation.Builder, org.tensorflow.metadata.v0.AnnotationOrBuilder>
getAnnotationFieldBuilder() {
if (annotationBuilder_ == null) {
annotationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.Annotation, org.tensorflow.metadata.v0.Annotation.Builder, org.tensorflow.metadata.v0.AnnotationOrBuilder>(
getAnnotation(),
getParentForChildren(),
isClean());
annotation_ = null;
}
return annotationBuilder_;
}
private org.tensorflow.metadata.v0.FeatureComparator skewComparator_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeatureComparator, org.tensorflow.metadata.v0.FeatureComparator.Builder, org.tensorflow.metadata.v0.FeatureComparatorOrBuilder> skewComparatorBuilder_;
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
* @return Whether the skewComparator field is set.
*/
public boolean hasSkewComparator() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
* @return The skewComparator.
*/
public org.tensorflow.metadata.v0.FeatureComparator getSkewComparator() {
if (skewComparatorBuilder_ == null) {
return skewComparator_ == null ? org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : skewComparator_;
} else {
return skewComparatorBuilder_.getMessage();
}
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
public Builder setSkewComparator(org.tensorflow.metadata.v0.FeatureComparator value) {
if (skewComparatorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
skewComparator_ = value;
} else {
skewComparatorBuilder_.setMessage(value);
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
public Builder setSkewComparator(
org.tensorflow.metadata.v0.FeatureComparator.Builder builderForValue) {
if (skewComparatorBuilder_ == null) {
skewComparator_ = builderForValue.build();
} else {
skewComparatorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
public Builder mergeSkewComparator(org.tensorflow.metadata.v0.FeatureComparator value) {
if (skewComparatorBuilder_ == null) {
if (((bitField0_ & 0x00400000) != 0) &&
skewComparator_ != null &&
skewComparator_ != org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance()) {
getSkewComparatorBuilder().mergeFrom(value);
} else {
skewComparator_ = value;
}
} else {
skewComparatorBuilder_.mergeFrom(value);
}
if (skewComparator_ != null) {
bitField0_ |= 0x00400000;
onChanged();
}
return this;
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
public Builder clearSkewComparator() {
bitField0_ = (bitField0_ & ~0x00400000);
skewComparator_ = null;
if (skewComparatorBuilder_ != null) {
skewComparatorBuilder_.dispose();
skewComparatorBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
public org.tensorflow.metadata.v0.FeatureComparator.Builder getSkewComparatorBuilder() {
bitField0_ |= 0x00400000;
onChanged();
return getSkewComparatorFieldBuilder().getBuilder();
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
public org.tensorflow.metadata.v0.FeatureComparatorOrBuilder getSkewComparatorOrBuilder() {
if (skewComparatorBuilder_ != null) {
return skewComparatorBuilder_.getMessageOrBuilder();
} else {
return skewComparator_ == null ?
org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : skewComparator_;
}
}
/**
*
* Tests comparing the distribution to the associated serving data.
*
*
* optional .tensorflow.metadata.v0.FeatureComparator skew_comparator = 18;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeatureComparator, org.tensorflow.metadata.v0.FeatureComparator.Builder, org.tensorflow.metadata.v0.FeatureComparatorOrBuilder>
getSkewComparatorFieldBuilder() {
if (skewComparatorBuilder_ == null) {
skewComparatorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeatureComparator, org.tensorflow.metadata.v0.FeatureComparator.Builder, org.tensorflow.metadata.v0.FeatureComparatorOrBuilder>(
getSkewComparator(),
getParentForChildren(),
isClean());
skewComparator_ = null;
}
return skewComparatorBuilder_;
}
private org.tensorflow.metadata.v0.FeatureComparator driftComparator_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeatureComparator, org.tensorflow.metadata.v0.FeatureComparator.Builder, org.tensorflow.metadata.v0.FeatureComparatorOrBuilder> driftComparatorBuilder_;
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
* @return Whether the driftComparator field is set.
*/
public boolean hasDriftComparator() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
* @return The driftComparator.
*/
public org.tensorflow.metadata.v0.FeatureComparator getDriftComparator() {
if (driftComparatorBuilder_ == null) {
return driftComparator_ == null ? org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : driftComparator_;
} else {
return driftComparatorBuilder_.getMessage();
}
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
public Builder setDriftComparator(org.tensorflow.metadata.v0.FeatureComparator value) {
if (driftComparatorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
driftComparator_ = value;
} else {
driftComparatorBuilder_.setMessage(value);
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
public Builder setDriftComparator(
org.tensorflow.metadata.v0.FeatureComparator.Builder builderForValue) {
if (driftComparatorBuilder_ == null) {
driftComparator_ = builderForValue.build();
} else {
driftComparatorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
public Builder mergeDriftComparator(org.tensorflow.metadata.v0.FeatureComparator value) {
if (driftComparatorBuilder_ == null) {
if (((bitField0_ & 0x00800000) != 0) &&
driftComparator_ != null &&
driftComparator_ != org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance()) {
getDriftComparatorBuilder().mergeFrom(value);
} else {
driftComparator_ = value;
}
} else {
driftComparatorBuilder_.mergeFrom(value);
}
if (driftComparator_ != null) {
bitField0_ |= 0x00800000;
onChanged();
}
return this;
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
public Builder clearDriftComparator() {
bitField0_ = (bitField0_ & ~0x00800000);
driftComparator_ = null;
if (driftComparatorBuilder_ != null) {
driftComparatorBuilder_.dispose();
driftComparatorBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
public org.tensorflow.metadata.v0.FeatureComparator.Builder getDriftComparatorBuilder() {
bitField0_ |= 0x00800000;
onChanged();
return getDriftComparatorFieldBuilder().getBuilder();
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
public org.tensorflow.metadata.v0.FeatureComparatorOrBuilder getDriftComparatorOrBuilder() {
if (driftComparatorBuilder_ != null) {
return driftComparatorBuilder_.getMessageOrBuilder();
} else {
return driftComparator_ == null ?
org.tensorflow.metadata.v0.FeatureComparator.getDefaultInstance() : driftComparator_;
}
}
/**
*
* Tests comparing the distribution between two consecutive spans (e.g. days).
*
*
* optional .tensorflow.metadata.v0.FeatureComparator drift_comparator = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeatureComparator, org.tensorflow.metadata.v0.FeatureComparator.Builder, org.tensorflow.metadata.v0.FeatureComparatorOrBuilder>
getDriftComparatorFieldBuilder() {
if (driftComparatorBuilder_ == null) {
driftComparatorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeatureComparator, org.tensorflow.metadata.v0.FeatureComparator.Builder, org.tensorflow.metadata.v0.FeatureComparatorOrBuilder>(
getDriftComparator(),
getParentForChildren(),
isClean());
driftComparator_ = null;
}
return driftComparatorBuilder_;
}
private com.google.protobuf.LazyStringArrayList inEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureInEnvironmentIsMutable() {
if (!inEnvironment_.isModifiable()) {
inEnvironment_ = new com.google.protobuf.LazyStringArrayList(inEnvironment_);
}
bitField0_ |= 0x01000000;
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @return A list containing the inEnvironment.
*/
public com.google.protobuf.ProtocolStringList
getInEnvironmentList() {
inEnvironment_.makeImmutable();
return inEnvironment_;
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @return The count of inEnvironment.
*/
public int getInEnvironmentCount() {
return inEnvironment_.size();
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param index The index of the element to return.
* @return The inEnvironment at the given index.
*/
public java.lang.String getInEnvironment(int index) {
return inEnvironment_.get(index);
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param index The index of the value to return.
* @return The bytes of the inEnvironment at the given index.
*/
public com.google.protobuf.ByteString
getInEnvironmentBytes(int index) {
return inEnvironment_.getByteString(index);
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param index The index to set the value at.
* @param value The inEnvironment to set.
* @return This builder for chaining.
*/
public Builder setInEnvironment(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureInEnvironmentIsMutable();
inEnvironment_.set(index, value);
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param value The inEnvironment to add.
* @return This builder for chaining.
*/
public Builder addInEnvironment(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureInEnvironmentIsMutable();
inEnvironment_.add(value);
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param values The inEnvironment to add.
* @return This builder for chaining.
*/
public Builder addAllInEnvironment(
java.lang.Iterable values) {
ensureInEnvironmentIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, inEnvironment_);
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @return This builder for chaining.
*/
public Builder clearInEnvironment() {
inEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x01000000);;
onChanged();
return this;
}
/**
*
* List of environments this feature is present in.
* Should be disjoint from not_in_environment.
* This feature is in environment "foo" if:
* ("foo" is in in_environment or default_environment) AND
* "foo" is not in not_in_environment.
* See Schema::default_environment.
*
*
* repeated string in_environment = 20;
* @param value The bytes of the inEnvironment to add.
* @return This builder for chaining.
*/
public Builder addInEnvironmentBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureInEnvironmentIsMutable();
inEnvironment_.add(value);
bitField0_ |= 0x01000000;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList notInEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureNotInEnvironmentIsMutable() {
if (!notInEnvironment_.isModifiable()) {
notInEnvironment_ = new com.google.protobuf.LazyStringArrayList(notInEnvironment_);
}
bitField0_ |= 0x02000000;
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @return A list containing the notInEnvironment.
*/
public com.google.protobuf.ProtocolStringList
getNotInEnvironmentList() {
notInEnvironment_.makeImmutable();
return notInEnvironment_;
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @return The count of notInEnvironment.
*/
public int getNotInEnvironmentCount() {
return notInEnvironment_.size();
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param index The index of the element to return.
* @return The notInEnvironment at the given index.
*/
public java.lang.String getNotInEnvironment(int index) {
return notInEnvironment_.get(index);
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param index The index of the value to return.
* @return The bytes of the notInEnvironment at the given index.
*/
public com.google.protobuf.ByteString
getNotInEnvironmentBytes(int index) {
return notInEnvironment_.getByteString(index);
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param index The index to set the value at.
* @param value The notInEnvironment to set.
* @return This builder for chaining.
*/
public Builder setNotInEnvironment(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureNotInEnvironmentIsMutable();
notInEnvironment_.set(index, value);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param value The notInEnvironment to add.
* @return This builder for chaining.
*/
public Builder addNotInEnvironment(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureNotInEnvironmentIsMutable();
notInEnvironment_.add(value);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param values The notInEnvironment to add.
* @return This builder for chaining.
*/
public Builder addAllNotInEnvironment(
java.lang.Iterable values) {
ensureNotInEnvironmentIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, notInEnvironment_);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @return This builder for chaining.
*/
public Builder clearNotInEnvironment() {
notInEnvironment_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x02000000);;
onChanged();
return this;
}
/**
*
* List of environments this feature is not present in.
* Should be disjoint from of in_environment.
* See Schema::default_environment and in_environment.
*
*
* repeated string not_in_environment = 19;
* @param value The bytes of the notInEnvironment to add.
* @return This builder for chaining.
*/
public Builder addNotInEnvironmentBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
ensureNotInEnvironmentIsMutable();
notInEnvironment_.add(value);
bitField0_ |= 0x02000000;
onChanged();
return this;
}
private int lifecycleStage_ = 0;
/**
*
* The lifecycle stage of a feature. It can also apply to its descendants.
* i.e., if a struct is DEPRECATED, its children are implicitly deprecated.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 22;
* @return Whether the lifecycleStage field is set.
*/
@java.lang.Override public boolean hasLifecycleStage() {
return ((bitField0_ & 0x04000000) != 0);
}
/**
*
* The lifecycle stage of a feature. It can also apply to its descendants.
* i.e., if a struct is DEPRECATED, its children are implicitly deprecated.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 22;
* @return The lifecycleStage.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.LifecycleStage getLifecycleStage() {
org.tensorflow.metadata.v0.LifecycleStage result = org.tensorflow.metadata.v0.LifecycleStage.forNumber(lifecycleStage_);
return result == null ? org.tensorflow.metadata.v0.LifecycleStage.UNKNOWN_STAGE : result;
}
/**
*
* The lifecycle stage of a feature. It can also apply to its descendants.
* i.e., if a struct is DEPRECATED, its children are implicitly deprecated.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 22;
* @param value The lifecycleStage to set.
* @return This builder for chaining.
*/
public Builder setLifecycleStage(org.tensorflow.metadata.v0.LifecycleStage value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x04000000;
lifecycleStage_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The lifecycle stage of a feature. It can also apply to its descendants.
* i.e., if a struct is DEPRECATED, its children are implicitly deprecated.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 22;
* @return This builder for chaining.
*/
public Builder clearLifecycleStage() {
bitField0_ = (bitField0_ & ~0x04000000);
lifecycleStage_ = 0;
onChanged();
return this;
}
private org.tensorflow.metadata.v0.UniqueConstraints uniqueConstraints_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.UniqueConstraints, org.tensorflow.metadata.v0.UniqueConstraints.Builder, org.tensorflow.metadata.v0.UniqueConstraintsOrBuilder> uniqueConstraintsBuilder_;
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
* @return Whether the uniqueConstraints field is set.
*/
public boolean hasUniqueConstraints() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
* @return The uniqueConstraints.
*/
public org.tensorflow.metadata.v0.UniqueConstraints getUniqueConstraints() {
if (uniqueConstraintsBuilder_ == null) {
return uniqueConstraints_ == null ? org.tensorflow.metadata.v0.UniqueConstraints.getDefaultInstance() : uniqueConstraints_;
} else {
return uniqueConstraintsBuilder_.getMessage();
}
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
public Builder setUniqueConstraints(org.tensorflow.metadata.v0.UniqueConstraints value) {
if (uniqueConstraintsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
uniqueConstraints_ = value;
} else {
uniqueConstraintsBuilder_.setMessage(value);
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
public Builder setUniqueConstraints(
org.tensorflow.metadata.v0.UniqueConstraints.Builder builderForValue) {
if (uniqueConstraintsBuilder_ == null) {
uniqueConstraints_ = builderForValue.build();
} else {
uniqueConstraintsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
public Builder mergeUniqueConstraints(org.tensorflow.metadata.v0.UniqueConstraints value) {
if (uniqueConstraintsBuilder_ == null) {
if (((bitField0_ & 0x08000000) != 0) &&
uniqueConstraints_ != null &&
uniqueConstraints_ != org.tensorflow.metadata.v0.UniqueConstraints.getDefaultInstance()) {
getUniqueConstraintsBuilder().mergeFrom(value);
} else {
uniqueConstraints_ = value;
}
} else {
uniqueConstraintsBuilder_.mergeFrom(value);
}
if (uniqueConstraints_ != null) {
bitField0_ |= 0x08000000;
onChanged();
}
return this;
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
public Builder clearUniqueConstraints() {
bitField0_ = (bitField0_ & ~0x08000000);
uniqueConstraints_ = null;
if (uniqueConstraintsBuilder_ != null) {
uniqueConstraintsBuilder_.dispose();
uniqueConstraintsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
public org.tensorflow.metadata.v0.UniqueConstraints.Builder getUniqueConstraintsBuilder() {
bitField0_ |= 0x08000000;
onChanged();
return getUniqueConstraintsFieldBuilder().getBuilder();
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
public org.tensorflow.metadata.v0.UniqueConstraintsOrBuilder getUniqueConstraintsOrBuilder() {
if (uniqueConstraintsBuilder_ != null) {
return uniqueConstraintsBuilder_.getMessageOrBuilder();
} else {
return uniqueConstraints_ == null ?
org.tensorflow.metadata.v0.UniqueConstraints.getDefaultInstance() : uniqueConstraints_;
}
}
/**
*
* Constraints on the number of unique values for a given feature.
* This is supported for string and categorical features only.
*
*
* optional .tensorflow.metadata.v0.UniqueConstraints unique_constraints = 31;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.UniqueConstraints, org.tensorflow.metadata.v0.UniqueConstraints.Builder, org.tensorflow.metadata.v0.UniqueConstraintsOrBuilder>
getUniqueConstraintsFieldBuilder() {
if (uniqueConstraintsBuilder_ == null) {
uniqueConstraintsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.UniqueConstraints, org.tensorflow.metadata.v0.UniqueConstraints.Builder, org.tensorflow.metadata.v0.UniqueConstraintsOrBuilder>(
getUniqueConstraints(),
getParentForChildren(),
isClean());
uniqueConstraints_ = null;
}
return uniqueConstraintsBuilder_;
}
private org.tensorflow.metadata.v0.DerivedFeatureSource validationDerivedSource_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.DerivedFeatureSource, org.tensorflow.metadata.v0.DerivedFeatureSource.Builder, org.tensorflow.metadata.v0.DerivedFeatureSourceOrBuilder> validationDerivedSourceBuilder_;
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
* @return Whether the validationDerivedSource field is set.
*/
public boolean hasValidationDerivedSource() {
return ((bitField0_ & 0x10000000) != 0);
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
* @return The validationDerivedSource.
*/
public org.tensorflow.metadata.v0.DerivedFeatureSource getValidationDerivedSource() {
if (validationDerivedSourceBuilder_ == null) {
return validationDerivedSource_ == null ? org.tensorflow.metadata.v0.DerivedFeatureSource.getDefaultInstance() : validationDerivedSource_;
} else {
return validationDerivedSourceBuilder_.getMessage();
}
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
public Builder setValidationDerivedSource(org.tensorflow.metadata.v0.DerivedFeatureSource value) {
if (validationDerivedSourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
validationDerivedSource_ = value;
} else {
validationDerivedSourceBuilder_.setMessage(value);
}
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
public Builder setValidationDerivedSource(
org.tensorflow.metadata.v0.DerivedFeatureSource.Builder builderForValue) {
if (validationDerivedSourceBuilder_ == null) {
validationDerivedSource_ = builderForValue.build();
} else {
validationDerivedSourceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
public Builder mergeValidationDerivedSource(org.tensorflow.metadata.v0.DerivedFeatureSource value) {
if (validationDerivedSourceBuilder_ == null) {
if (((bitField0_ & 0x10000000) != 0) &&
validationDerivedSource_ != null &&
validationDerivedSource_ != org.tensorflow.metadata.v0.DerivedFeatureSource.getDefaultInstance()) {
getValidationDerivedSourceBuilder().mergeFrom(value);
} else {
validationDerivedSource_ = value;
}
} else {
validationDerivedSourceBuilder_.mergeFrom(value);
}
if (validationDerivedSource_ != null) {
bitField0_ |= 0x10000000;
onChanged();
}
return this;
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
public Builder clearValidationDerivedSource() {
bitField0_ = (bitField0_ & ~0x10000000);
validationDerivedSource_ = null;
if (validationDerivedSourceBuilder_ != null) {
validationDerivedSourceBuilder_.dispose();
validationDerivedSourceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
public org.tensorflow.metadata.v0.DerivedFeatureSource.Builder getValidationDerivedSourceBuilder() {
bitField0_ |= 0x10000000;
onChanged();
return getValidationDerivedSourceFieldBuilder().getBuilder();
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
public org.tensorflow.metadata.v0.DerivedFeatureSourceOrBuilder getValidationDerivedSourceOrBuilder() {
if (validationDerivedSourceBuilder_ != null) {
return validationDerivedSourceBuilder_.getMessageOrBuilder();
} else {
return validationDerivedSource_ == null ?
org.tensorflow.metadata.v0.DerivedFeatureSource.getDefaultInstance() : validationDerivedSource_;
}
}
/**
*
* If set, indicates that that this feature is derived, and stores metadata
* about its source. If this field is set, this feature should have a
* disabled stage (PLANNED, ALPHA, DEPRECATED, DISABLED, DEBUG_ONLY), or
* lifecycle_stage VALIDATION_DERIVED.
* Experimental and subject to change.
*
*
* optional .tensorflow.metadata.v0.DerivedFeatureSource validation_derived_source = 34;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.DerivedFeatureSource, org.tensorflow.metadata.v0.DerivedFeatureSource.Builder, org.tensorflow.metadata.v0.DerivedFeatureSourceOrBuilder>
getValidationDerivedSourceFieldBuilder() {
if (validationDerivedSourceBuilder_ == null) {
validationDerivedSourceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.DerivedFeatureSource, org.tensorflow.metadata.v0.DerivedFeatureSource.Builder, org.tensorflow.metadata.v0.DerivedFeatureSourceOrBuilder>(
getValidationDerivedSource(),
getParentForChildren(),
isClean());
validationDerivedSource_ = null;
}
return validationDerivedSourceBuilder_;
}
private org.tensorflow.metadata.v0.SequenceMetadata sequenceMetadata_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.SequenceMetadata, org.tensorflow.metadata.v0.SequenceMetadata.Builder, org.tensorflow.metadata.v0.SequenceMetadataOrBuilder> sequenceMetadataBuilder_;
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
* @return Whether the sequenceMetadata field is set.
*/
public boolean hasSequenceMetadata() {
return ((bitField0_ & 0x20000000) != 0);
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
* @return The sequenceMetadata.
*/
public org.tensorflow.metadata.v0.SequenceMetadata getSequenceMetadata() {
if (sequenceMetadataBuilder_ == null) {
return sequenceMetadata_ == null ? org.tensorflow.metadata.v0.SequenceMetadata.getDefaultInstance() : sequenceMetadata_;
} else {
return sequenceMetadataBuilder_.getMessage();
}
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
public Builder setSequenceMetadata(org.tensorflow.metadata.v0.SequenceMetadata value) {
if (sequenceMetadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
sequenceMetadata_ = value;
} else {
sequenceMetadataBuilder_.setMessage(value);
}
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
public Builder setSequenceMetadata(
org.tensorflow.metadata.v0.SequenceMetadata.Builder builderForValue) {
if (sequenceMetadataBuilder_ == null) {
sequenceMetadata_ = builderForValue.build();
} else {
sequenceMetadataBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
public Builder mergeSequenceMetadata(org.tensorflow.metadata.v0.SequenceMetadata value) {
if (sequenceMetadataBuilder_ == null) {
if (((bitField0_ & 0x20000000) != 0) &&
sequenceMetadata_ != null &&
sequenceMetadata_ != org.tensorflow.metadata.v0.SequenceMetadata.getDefaultInstance()) {
getSequenceMetadataBuilder().mergeFrom(value);
} else {
sequenceMetadata_ = value;
}
} else {
sequenceMetadataBuilder_.mergeFrom(value);
}
if (sequenceMetadata_ != null) {
bitField0_ |= 0x20000000;
onChanged();
}
return this;
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
public Builder clearSequenceMetadata() {
bitField0_ = (bitField0_ & ~0x20000000);
sequenceMetadata_ = null;
if (sequenceMetadataBuilder_ != null) {
sequenceMetadataBuilder_.dispose();
sequenceMetadataBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
public org.tensorflow.metadata.v0.SequenceMetadata.Builder getSequenceMetadataBuilder() {
bitField0_ |= 0x20000000;
onChanged();
return getSequenceMetadataFieldBuilder().getBuilder();
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
public org.tensorflow.metadata.v0.SequenceMetadataOrBuilder getSequenceMetadataOrBuilder() {
if (sequenceMetadataBuilder_ != null) {
return sequenceMetadataBuilder_.getMessageOrBuilder();
} else {
return sequenceMetadata_ == null ?
org.tensorflow.metadata.v0.SequenceMetadata.getDefaultInstance() : sequenceMetadata_;
}
}
/**
*
* This field specifies if this feature could be treated as a sequence
* feature which has meaningful element order.
*
*
* optional .tensorflow.metadata.v0.SequenceMetadata sequence_metadata = 35;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.SequenceMetadata, org.tensorflow.metadata.v0.SequenceMetadata.Builder, org.tensorflow.metadata.v0.SequenceMetadataOrBuilder>
getSequenceMetadataFieldBuilder() {
if (sequenceMetadataBuilder_ == null) {
sequenceMetadataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.SequenceMetadata, org.tensorflow.metadata.v0.SequenceMetadata.Builder, org.tensorflow.metadata.v0.SequenceMetadataOrBuilder>(
getSequenceMetadata(),
getParentForChildren(),
isClean());
sequenceMetadata_ = null;
}
return sequenceMetadataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.Feature)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.Feature)
private static final org.tensorflow.metadata.v0.Feature DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.Feature();
}
public static org.tensorflow.metadata.v0.Feature getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Feature parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.Feature getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy