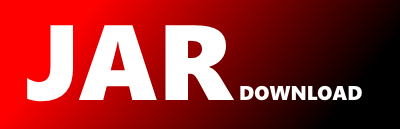
org.tensorflow.metadata.v0.SparseFeature Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow_metadata/proto/v0/schema.proto
// Protobuf Java Version: 3.25.4
package org.tensorflow.metadata.v0;
/**
*
* A sparse feature represents a sparse tensor that is encoded with a
* combination of raw features, namely index features and a value feature. Each
* index feature defines a list of indices in a different dimension.
*
*
* Protobuf type {@code tensorflow.metadata.v0.SparseFeature}
*/
public final class SparseFeature extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.SparseFeature)
SparseFeatureOrBuilder {
private static final long serialVersionUID = 0L;
// Use SparseFeature.newBuilder() to construct.
private SparseFeature(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SparseFeature() {
name_ = "";
lifecycleStage_ = 0;
indexFeature_ = java.util.Collections.emptyList();
type_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SparseFeature();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.SparseFeature.class, org.tensorflow.metadata.v0.SparseFeature.Builder.class);
}
public interface IndexFeatureOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.metadata.v0.SparseFeature.IndexFeature)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code tensorflow.metadata.v0.SparseFeature.IndexFeature}
*/
public static final class IndexFeature extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.SparseFeature.IndexFeature)
IndexFeatureOrBuilder {
private static final long serialVersionUID = 0L;
// Use IndexFeature.newBuilder() to construct.
private IndexFeature(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IndexFeature() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new IndexFeature();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_IndexFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_IndexFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.SparseFeature.IndexFeature.class, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.SparseFeature.IndexFeature)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.SparseFeature.IndexFeature other = (org.tensorflow.metadata.v0.SparseFeature.IndexFeature) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.SparseFeature.IndexFeature prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.metadata.v0.SparseFeature.IndexFeature}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.SparseFeature.IndexFeature)
org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_IndexFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_IndexFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.SparseFeature.IndexFeature.class, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.SparseFeature.IndexFeature.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_IndexFeature_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.SparseFeature.IndexFeature.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature build() {
org.tensorflow.metadata.v0.SparseFeature.IndexFeature result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature buildPartial() {
org.tensorflow.metadata.v0.SparseFeature.IndexFeature result = new org.tensorflow.metadata.v0.SparseFeature.IndexFeature(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tensorflow.metadata.v0.SparseFeature.IndexFeature result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.SparseFeature.IndexFeature) {
return mergeFrom((org.tensorflow.metadata.v0.SparseFeature.IndexFeature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.SparseFeature.IndexFeature other) {
if (other == org.tensorflow.metadata.v0.SparseFeature.IndexFeature.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Name of the index-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.SparseFeature.IndexFeature)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.SparseFeature.IndexFeature)
private static final org.tensorflow.metadata.v0.SparseFeature.IndexFeature DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.SparseFeature.IndexFeature();
}
public static org.tensorflow.metadata.v0.SparseFeature.IndexFeature getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IndexFeature parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ValueFeatureOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.metadata.v0.SparseFeature.ValueFeature)
com.google.protobuf.MessageOrBuilder {
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
boolean hasName();
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
* Protobuf type {@code tensorflow.metadata.v0.SparseFeature.ValueFeature}
*/
public static final class ValueFeature extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.metadata.v0.SparseFeature.ValueFeature)
ValueFeatureOrBuilder {
private static final long serialVersionUID = 0L;
// Use ValueFeature.newBuilder() to construct.
private ValueFeature(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ValueFeature() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ValueFeature();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_ValueFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_ValueFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.SparseFeature.ValueFeature.class, org.tensorflow.metadata.v0.SparseFeature.ValueFeature.Builder.class);
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.SparseFeature.ValueFeature)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.SparseFeature.ValueFeature other = (org.tensorflow.metadata.v0.SparseFeature.ValueFeature) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.SparseFeature.ValueFeature prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.metadata.v0.SparseFeature.ValueFeature}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.SparseFeature.ValueFeature)
org.tensorflow.metadata.v0.SparseFeature.ValueFeatureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_ValueFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_ValueFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.SparseFeature.ValueFeature.class, org.tensorflow.metadata.v0.SparseFeature.ValueFeature.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.SparseFeature.ValueFeature.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_ValueFeature_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.ValueFeature getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.SparseFeature.ValueFeature.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.ValueFeature build() {
org.tensorflow.metadata.v0.SparseFeature.ValueFeature result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.ValueFeature buildPartial() {
org.tensorflow.metadata.v0.SparseFeature.ValueFeature result = new org.tensorflow.metadata.v0.SparseFeature.ValueFeature(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.tensorflow.metadata.v0.SparseFeature.ValueFeature result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.SparseFeature.ValueFeature) {
return mergeFrom((org.tensorflow.metadata.v0.SparseFeature.ValueFeature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.SparseFeature.ValueFeature other) {
if (other == org.tensorflow.metadata.v0.SparseFeature.ValueFeature.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Name of the value-feature. This should be a reference to an existing
* feature in the schema.
*
*
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.SparseFeature.ValueFeature)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.SparseFeature.ValueFeature)
private static final org.tensorflow.metadata.v0.SparseFeature.ValueFeature DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.SparseFeature.ValueFeature();
}
public static org.tensorflow.metadata.v0.SparseFeature.ValueFeature getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ValueFeature parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.ValueFeature getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DEPRECATED_FIELD_NUMBER = 2;
private boolean deprecated_ = false;
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=409
* @return Whether the deprecated field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasDeprecated() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=409
* @return The deprecated.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getDeprecated() {
return deprecated_;
}
public static final int LIFECYCLE_STAGE_FIELD_NUMBER = 7;
private int lifecycleStage_ = 0;
/**
*
* The lifecycle_stage determines where a feature is expected to be used,
* and therefore how important issues with it are.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 7;
* @return Whether the lifecycleStage field is set.
*/
@java.lang.Override public boolean hasLifecycleStage() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The lifecycle_stage determines where a feature is expected to be used,
* and therefore how important issues with it are.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 7;
* @return The lifecycleStage.
*/
@java.lang.Override public org.tensorflow.metadata.v0.LifecycleStage getLifecycleStage() {
org.tensorflow.metadata.v0.LifecycleStage result = org.tensorflow.metadata.v0.LifecycleStage.forNumber(lifecycleStage_);
return result == null ? org.tensorflow.metadata.v0.LifecycleStage.UNKNOWN_STAGE : result;
}
public static final int PRESENCE_FIELD_NUMBER = 4;
private org.tensorflow.metadata.v0.FeaturePresence presence_;
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.presence is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=421
* @return Whether the presence field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasPresence() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.presence is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=421
* @return The presence.
*/
@java.lang.Override
@java.lang.Deprecated public org.tensorflow.metadata.v0.FeaturePresence getPresence() {
return presence_ == null ? org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance() : presence_;
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
@java.lang.Override
@java.lang.Deprecated public org.tensorflow.metadata.v0.FeaturePresenceOrBuilder getPresenceOrBuilder() {
return presence_ == null ? org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance() : presence_;
}
public static final int DENSE_SHAPE_FIELD_NUMBER = 5;
private org.tensorflow.metadata.v0.FixedShape denseShape_;
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
* @return Whether the denseShape field is set.
*/
@java.lang.Override
public boolean hasDenseShape() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
* @return The denseShape.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FixedShape getDenseShape() {
return denseShape_ == null ? org.tensorflow.metadata.v0.FixedShape.getDefaultInstance() : denseShape_;
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.FixedShapeOrBuilder getDenseShapeOrBuilder() {
return denseShape_ == null ? org.tensorflow.metadata.v0.FixedShape.getDefaultInstance() : denseShape_;
}
public static final int INDEX_FEATURE_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List indexFeature_;
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
@java.lang.Override
public java.util.List getIndexFeatureList() {
return indexFeature_;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
@java.lang.Override
public java.util.List extends org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder>
getIndexFeatureOrBuilderList() {
return indexFeature_;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
@java.lang.Override
public int getIndexFeatureCount() {
return indexFeature_.size();
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature getIndexFeature(int index) {
return indexFeature_.get(index);
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder getIndexFeatureOrBuilder(
int index) {
return indexFeature_.get(index);
}
public static final int IS_SORTED_FIELD_NUMBER = 8;
private boolean isSorted_ = false;
/**
*
* If true then the index values are already sorted lexicographically.
*
*
* optional bool is_sorted = 8;
* @return Whether the isSorted field is set.
*/
@java.lang.Override
public boolean hasIsSorted() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* If true then the index values are already sorted lexicographically.
*
*
* optional bool is_sorted = 8;
* @return The isSorted.
*/
@java.lang.Override
public boolean getIsSorted() {
return isSorted_;
}
public static final int VALUE_FEATURE_FIELD_NUMBER = 9;
private org.tensorflow.metadata.v0.SparseFeature.ValueFeature valueFeature_;
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
* @return Whether the valueFeature field is set.
*/
@java.lang.Override
public boolean hasValueFeature() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
* @return The valueFeature.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.ValueFeature getValueFeature() {
return valueFeature_ == null ? org.tensorflow.metadata.v0.SparseFeature.ValueFeature.getDefaultInstance() : valueFeature_;
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature.ValueFeatureOrBuilder getValueFeatureOrBuilder() {
return valueFeature_ == null ? org.tensorflow.metadata.v0.SparseFeature.ValueFeature.getDefaultInstance() : valueFeature_;
}
public static final int TYPE_FIELD_NUMBER = 10;
private int type_ = 0;
/**
*
* Type of value feature.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 10 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.type is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=448
* @return Whether the type field is set.
*/
@java.lang.Override @java.lang.Deprecated public boolean hasType() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Type of value feature.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 10 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.type is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=448
* @return The type.
*/
@java.lang.Override @java.lang.Deprecated public org.tensorflow.metadata.v0.FeatureType getType() {
org.tensorflow.metadata.v0.FeatureType result = org.tensorflow.metadata.v0.FeatureType.forNumber(type_);
return result == null ? org.tensorflow.metadata.v0.FeatureType.TYPE_UNKNOWN : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(2, deprecated_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(4, getPresence());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(5, getDenseShape());
}
for (int i = 0; i < indexFeature_.size(); i++) {
output.writeMessage(6, indexFeature_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeEnum(7, lifecycleStage_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeBool(8, isSorted_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(9, getValueFeature());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeEnum(10, type_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, deprecated_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getPresence());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getDenseShape());
}
for (int i = 0; i < indexFeature_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, indexFeature_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, lifecycleStage_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(8, isSorted_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getValueFeature());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, type_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.metadata.v0.SparseFeature)) {
return super.equals(obj);
}
org.tensorflow.metadata.v0.SparseFeature other = (org.tensorflow.metadata.v0.SparseFeature) obj;
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasDeprecated() != other.hasDeprecated()) return false;
if (hasDeprecated()) {
if (getDeprecated()
!= other.getDeprecated()) return false;
}
if (hasLifecycleStage() != other.hasLifecycleStage()) return false;
if (hasLifecycleStage()) {
if (lifecycleStage_ != other.lifecycleStage_) return false;
}
if (hasPresence() != other.hasPresence()) return false;
if (hasPresence()) {
if (!getPresence()
.equals(other.getPresence())) return false;
}
if (hasDenseShape() != other.hasDenseShape()) return false;
if (hasDenseShape()) {
if (!getDenseShape()
.equals(other.getDenseShape())) return false;
}
if (!getIndexFeatureList()
.equals(other.getIndexFeatureList())) return false;
if (hasIsSorted() != other.hasIsSorted()) return false;
if (hasIsSorted()) {
if (getIsSorted()
!= other.getIsSorted()) return false;
}
if (hasValueFeature() != other.hasValueFeature()) return false;
if (hasValueFeature()) {
if (!getValueFeature()
.equals(other.getValueFeature())) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (type_ != other.type_) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasDeprecated()) {
hash = (37 * hash) + DEPRECATED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getDeprecated());
}
if (hasLifecycleStage()) {
hash = (37 * hash) + LIFECYCLE_STAGE_FIELD_NUMBER;
hash = (53 * hash) + lifecycleStage_;
}
if (hasPresence()) {
hash = (37 * hash) + PRESENCE_FIELD_NUMBER;
hash = (53 * hash) + getPresence().hashCode();
}
if (hasDenseShape()) {
hash = (37 * hash) + DENSE_SHAPE_FIELD_NUMBER;
hash = (53 * hash) + getDenseShape().hashCode();
}
if (getIndexFeatureCount() > 0) {
hash = (37 * hash) + INDEX_FEATURE_FIELD_NUMBER;
hash = (53 * hash) + getIndexFeatureList().hashCode();
}
if (hasIsSorted()) {
hash = (37 * hash) + IS_SORTED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsSorted());
}
if (hasValueFeature()) {
hash = (37 * hash) + VALUE_FEATURE_FIELD_NUMBER;
hash = (53 * hash) + getValueFeature().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.metadata.v0.SparseFeature parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.metadata.v0.SparseFeature prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A sparse feature represents a sparse tensor that is encoded with a
* combination of raw features, namely index features and a value feature. Each
* index feature defines a list of indices in a different dimension.
*
*
* Protobuf type {@code tensorflow.metadata.v0.SparseFeature}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.metadata.v0.SparseFeature)
org.tensorflow.metadata.v0.SparseFeatureOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.metadata.v0.SparseFeature.class, org.tensorflow.metadata.v0.SparseFeature.Builder.class);
}
// Construct using org.tensorflow.metadata.v0.SparseFeature.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getPresenceFieldBuilder();
getDenseShapeFieldBuilder();
getIndexFeatureFieldBuilder();
getValueFeatureFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
deprecated_ = false;
lifecycleStage_ = 0;
presence_ = null;
if (presenceBuilder_ != null) {
presenceBuilder_.dispose();
presenceBuilder_ = null;
}
denseShape_ = null;
if (denseShapeBuilder_ != null) {
denseShapeBuilder_.dispose();
denseShapeBuilder_ = null;
}
if (indexFeatureBuilder_ == null) {
indexFeature_ = java.util.Collections.emptyList();
} else {
indexFeature_ = null;
indexFeatureBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
isSorted_ = false;
valueFeature_ = null;
if (valueFeatureBuilder_ != null) {
valueFeatureBuilder_.dispose();
valueFeatureBuilder_ = null;
}
type_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.metadata.v0.SchemaOuterClass.internal_static_tensorflow_metadata_v0_SparseFeature_descriptor;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature getDefaultInstanceForType() {
return org.tensorflow.metadata.v0.SparseFeature.getDefaultInstance();
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature build() {
org.tensorflow.metadata.v0.SparseFeature result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature buildPartial() {
org.tensorflow.metadata.v0.SparseFeature result = new org.tensorflow.metadata.v0.SparseFeature(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.tensorflow.metadata.v0.SparseFeature result) {
if (indexFeatureBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
indexFeature_ = java.util.Collections.unmodifiableList(indexFeature_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.indexFeature_ = indexFeature_;
} else {
result.indexFeature_ = indexFeatureBuilder_.build();
}
}
private void buildPartial0(org.tensorflow.metadata.v0.SparseFeature result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.deprecated_ = deprecated_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.lifecycleStage_ = lifecycleStage_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.presence_ = presenceBuilder_ == null
? presence_
: presenceBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.denseShape_ = denseShapeBuilder_ == null
? denseShape_
: denseShapeBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.isSorted_ = isSorted_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.valueFeature_ = valueFeatureBuilder_ == null
? valueFeature_
: valueFeatureBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00000080;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.metadata.v0.SparseFeature) {
return mergeFrom((org.tensorflow.metadata.v0.SparseFeature)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.metadata.v0.SparseFeature other) {
if (other == org.tensorflow.metadata.v0.SparseFeature.getDefaultInstance()) return this;
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasDeprecated()) {
setDeprecated(other.getDeprecated());
}
if (other.hasLifecycleStage()) {
setLifecycleStage(other.getLifecycleStage());
}
if (other.hasPresence()) {
mergePresence(other.getPresence());
}
if (other.hasDenseShape()) {
mergeDenseShape(other.getDenseShape());
}
if (indexFeatureBuilder_ == null) {
if (!other.indexFeature_.isEmpty()) {
if (indexFeature_.isEmpty()) {
indexFeature_ = other.indexFeature_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureIndexFeatureIsMutable();
indexFeature_.addAll(other.indexFeature_);
}
onChanged();
}
} else {
if (!other.indexFeature_.isEmpty()) {
if (indexFeatureBuilder_.isEmpty()) {
indexFeatureBuilder_.dispose();
indexFeatureBuilder_ = null;
indexFeature_ = other.indexFeature_;
bitField0_ = (bitField0_ & ~0x00000020);
indexFeatureBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getIndexFeatureFieldBuilder() : null;
} else {
indexFeatureBuilder_.addAllMessages(other.indexFeature_);
}
}
}
if (other.hasIsSorted()) {
setIsSorted(other.getIsSorted());
}
if (other.hasValueFeature()) {
mergeValueFeature(other.getValueFeature());
}
if (other.hasType()) {
setType(other.getType());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
name_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16: {
deprecated_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 16
case 34: {
input.readMessage(
getPresenceFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
input.readMessage(
getDenseShapeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
org.tensorflow.metadata.v0.SparseFeature.IndexFeature m =
input.readMessage(
org.tensorflow.metadata.v0.SparseFeature.IndexFeature.PARSER,
extensionRegistry);
if (indexFeatureBuilder_ == null) {
ensureIndexFeatureIsMutable();
indexFeature_.add(m);
} else {
indexFeatureBuilder_.addMessage(m);
}
break;
} // case 50
case 56: {
int tmpRaw = input.readEnum();
org.tensorflow.metadata.v0.LifecycleStage tmpValue =
org.tensorflow.metadata.v0.LifecycleStage.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(7, tmpRaw);
} else {
lifecycleStage_ = tmpRaw;
bitField0_ |= 0x00000004;
}
break;
} // case 56
case 64: {
isSorted_ = input.readBool();
bitField0_ |= 0x00000040;
break;
} // case 64
case 74: {
input.readMessage(
getValueFeatureFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 74
case 80: {
int tmpRaw = input.readEnum();
org.tensorflow.metadata.v0.FeatureType tmpValue =
org.tensorflow.metadata.v0.FeatureType.forNumber(tmpRaw);
if (tmpValue == null) {
mergeUnknownVarintField(10, tmpRaw);
} else {
type_ = tmpRaw;
bitField0_ |= 0x00000100;
}
break;
} // case 80
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Name for the sparse feature. This should not clash with other features in
* the same schema.
*
*
* optional string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private boolean deprecated_ ;
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=409
* @return Whether the deprecated field is set.
*/
@java.lang.Override
@java.lang.Deprecated public boolean hasDeprecated() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=409
* @return The deprecated.
*/
@java.lang.Override
@java.lang.Deprecated public boolean getDeprecated() {
return deprecated_;
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=409
* @param value The deprecated to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setDeprecated(boolean value) {
deprecated_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* This field is no longer supported. Instead, use:
* lifecycle_stage: DEPRECATED
* TODO(b/111450258): remove this.
*
*
* optional bool deprecated = 2 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.deprecated is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=409
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearDeprecated() {
bitField0_ = (bitField0_ & ~0x00000002);
deprecated_ = false;
onChanged();
return this;
}
private int lifecycleStage_ = 0;
/**
*
* The lifecycle_stage determines where a feature is expected to be used,
* and therefore how important issues with it are.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 7;
* @return Whether the lifecycleStage field is set.
*/
@java.lang.Override public boolean hasLifecycleStage() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The lifecycle_stage determines where a feature is expected to be used,
* and therefore how important issues with it are.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 7;
* @return The lifecycleStage.
*/
@java.lang.Override
public org.tensorflow.metadata.v0.LifecycleStage getLifecycleStage() {
org.tensorflow.metadata.v0.LifecycleStage result = org.tensorflow.metadata.v0.LifecycleStage.forNumber(lifecycleStage_);
return result == null ? org.tensorflow.metadata.v0.LifecycleStage.UNKNOWN_STAGE : result;
}
/**
*
* The lifecycle_stage determines where a feature is expected to be used,
* and therefore how important issues with it are.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 7;
* @param value The lifecycleStage to set.
* @return This builder for chaining.
*/
public Builder setLifecycleStage(org.tensorflow.metadata.v0.LifecycleStage value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
lifecycleStage_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The lifecycle_stage determines where a feature is expected to be used,
* and therefore how important issues with it are.
*
*
* optional .tensorflow.metadata.v0.LifecycleStage lifecycle_stage = 7;
* @return This builder for chaining.
*/
public Builder clearLifecycleStage() {
bitField0_ = (bitField0_ & ~0x00000004);
lifecycleStage_ = 0;
onChanged();
return this;
}
private org.tensorflow.metadata.v0.FeaturePresence presence_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresence, org.tensorflow.metadata.v0.FeaturePresence.Builder, org.tensorflow.metadata.v0.FeaturePresenceOrBuilder> presenceBuilder_;
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.presence is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=421
* @return Whether the presence field is set.
*/
@java.lang.Deprecated public boolean hasPresence() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.presence is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=421
* @return The presence.
*/
@java.lang.Deprecated public org.tensorflow.metadata.v0.FeaturePresence getPresence() {
if (presenceBuilder_ == null) {
return presence_ == null ? org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance() : presence_;
} else {
return presenceBuilder_.getMessage();
}
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder setPresence(org.tensorflow.metadata.v0.FeaturePresence value) {
if (presenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
presence_ = value;
} else {
presenceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder setPresence(
org.tensorflow.metadata.v0.FeaturePresence.Builder builderForValue) {
if (presenceBuilder_ == null) {
presence_ = builderForValue.build();
} else {
presenceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder mergePresence(org.tensorflow.metadata.v0.FeaturePresence value) {
if (presenceBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
presence_ != null &&
presence_ != org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance()) {
getPresenceBuilder().mergeFrom(value);
} else {
presence_ = value;
}
} else {
presenceBuilder_.mergeFrom(value);
}
if (presence_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearPresence() {
bitField0_ = (bitField0_ & ~0x00000008);
presence_ = null;
if (presenceBuilder_ != null) {
presenceBuilder_.dispose();
presenceBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
@java.lang.Deprecated public org.tensorflow.metadata.v0.FeaturePresence.Builder getPresenceBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getPresenceFieldBuilder().getBuilder();
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
@java.lang.Deprecated public org.tensorflow.metadata.v0.FeaturePresenceOrBuilder getPresenceOrBuilder() {
if (presenceBuilder_ != null) {
return presenceBuilder_.getMessageOrBuilder();
} else {
return presence_ == null ?
org.tensorflow.metadata.v0.FeaturePresence.getDefaultInstance() : presence_;
}
}
/**
*
* Constraints on the presence of this feature in examples.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeaturePresence presence = 4 [deprecated = true];
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresence, org.tensorflow.metadata.v0.FeaturePresence.Builder, org.tensorflow.metadata.v0.FeaturePresenceOrBuilder>
getPresenceFieldBuilder() {
if (presenceBuilder_ == null) {
presenceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FeaturePresence, org.tensorflow.metadata.v0.FeaturePresence.Builder, org.tensorflow.metadata.v0.FeaturePresenceOrBuilder>(
getPresence(),
getParentForChildren(),
isClean());
presence_ = null;
}
return presenceBuilder_;
}
private org.tensorflow.metadata.v0.FixedShape denseShape_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FixedShape, org.tensorflow.metadata.v0.FixedShape.Builder, org.tensorflow.metadata.v0.FixedShapeOrBuilder> denseShapeBuilder_;
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
* @return Whether the denseShape field is set.
*/
public boolean hasDenseShape() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
* @return The denseShape.
*/
public org.tensorflow.metadata.v0.FixedShape getDenseShape() {
if (denseShapeBuilder_ == null) {
return denseShape_ == null ? org.tensorflow.metadata.v0.FixedShape.getDefaultInstance() : denseShape_;
} else {
return denseShapeBuilder_.getMessage();
}
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
public Builder setDenseShape(org.tensorflow.metadata.v0.FixedShape value) {
if (denseShapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
denseShape_ = value;
} else {
denseShapeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
public Builder setDenseShape(
org.tensorflow.metadata.v0.FixedShape.Builder builderForValue) {
if (denseShapeBuilder_ == null) {
denseShape_ = builderForValue.build();
} else {
denseShapeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
public Builder mergeDenseShape(org.tensorflow.metadata.v0.FixedShape value) {
if (denseShapeBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
denseShape_ != null &&
denseShape_ != org.tensorflow.metadata.v0.FixedShape.getDefaultInstance()) {
getDenseShapeBuilder().mergeFrom(value);
} else {
denseShape_ = value;
}
} else {
denseShapeBuilder_.mergeFrom(value);
}
if (denseShape_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
public Builder clearDenseShape() {
bitField0_ = (bitField0_ & ~0x00000010);
denseShape_ = null;
if (denseShapeBuilder_ != null) {
denseShapeBuilder_.dispose();
denseShapeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
public org.tensorflow.metadata.v0.FixedShape.Builder getDenseShapeBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getDenseShapeFieldBuilder().getBuilder();
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
public org.tensorflow.metadata.v0.FixedShapeOrBuilder getDenseShapeOrBuilder() {
if (denseShapeBuilder_ != null) {
return denseShapeBuilder_.getMessageOrBuilder();
} else {
return denseShape_ == null ?
org.tensorflow.metadata.v0.FixedShape.getDefaultInstance() : denseShape_;
}
}
/**
*
* Shape of the sparse tensor that this SparseFeature represents.
* Currently not supported.
* TODO(b/109669962): Consider deriving this from the referred features.
*
*
* optional .tensorflow.metadata.v0.FixedShape dense_shape = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FixedShape, org.tensorflow.metadata.v0.FixedShape.Builder, org.tensorflow.metadata.v0.FixedShapeOrBuilder>
getDenseShapeFieldBuilder() {
if (denseShapeBuilder_ == null) {
denseShapeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.FixedShape, org.tensorflow.metadata.v0.FixedShape.Builder, org.tensorflow.metadata.v0.FixedShapeOrBuilder>(
getDenseShape(),
getParentForChildren(),
isClean());
denseShape_ = null;
}
return denseShapeBuilder_;
}
private java.util.List indexFeature_ =
java.util.Collections.emptyList();
private void ensureIndexFeatureIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
indexFeature_ = new java.util.ArrayList(indexFeature_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.SparseFeature.IndexFeature, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder, org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder> indexFeatureBuilder_;
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public java.util.List getIndexFeatureList() {
if (indexFeatureBuilder_ == null) {
return java.util.Collections.unmodifiableList(indexFeature_);
} else {
return indexFeatureBuilder_.getMessageList();
}
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public int getIndexFeatureCount() {
if (indexFeatureBuilder_ == null) {
return indexFeature_.size();
} else {
return indexFeatureBuilder_.getCount();
}
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature getIndexFeature(int index) {
if (indexFeatureBuilder_ == null) {
return indexFeature_.get(index);
} else {
return indexFeatureBuilder_.getMessage(index);
}
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder setIndexFeature(
int index, org.tensorflow.metadata.v0.SparseFeature.IndexFeature value) {
if (indexFeatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIndexFeatureIsMutable();
indexFeature_.set(index, value);
onChanged();
} else {
indexFeatureBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder setIndexFeature(
int index, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder builderForValue) {
if (indexFeatureBuilder_ == null) {
ensureIndexFeatureIsMutable();
indexFeature_.set(index, builderForValue.build());
onChanged();
} else {
indexFeatureBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder addIndexFeature(org.tensorflow.metadata.v0.SparseFeature.IndexFeature value) {
if (indexFeatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIndexFeatureIsMutable();
indexFeature_.add(value);
onChanged();
} else {
indexFeatureBuilder_.addMessage(value);
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder addIndexFeature(
int index, org.tensorflow.metadata.v0.SparseFeature.IndexFeature value) {
if (indexFeatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIndexFeatureIsMutable();
indexFeature_.add(index, value);
onChanged();
} else {
indexFeatureBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder addIndexFeature(
org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder builderForValue) {
if (indexFeatureBuilder_ == null) {
ensureIndexFeatureIsMutable();
indexFeature_.add(builderForValue.build());
onChanged();
} else {
indexFeatureBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder addIndexFeature(
int index, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder builderForValue) {
if (indexFeatureBuilder_ == null) {
ensureIndexFeatureIsMutable();
indexFeature_.add(index, builderForValue.build());
onChanged();
} else {
indexFeatureBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder addAllIndexFeature(
java.lang.Iterable extends org.tensorflow.metadata.v0.SparseFeature.IndexFeature> values) {
if (indexFeatureBuilder_ == null) {
ensureIndexFeatureIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, indexFeature_);
onChanged();
} else {
indexFeatureBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder clearIndexFeature() {
if (indexFeatureBuilder_ == null) {
indexFeature_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
indexFeatureBuilder_.clear();
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public Builder removeIndexFeature(int index) {
if (indexFeatureBuilder_ == null) {
ensureIndexFeatureIsMutable();
indexFeature_.remove(index);
onChanged();
} else {
indexFeatureBuilder_.remove(index);
}
return this;
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder getIndexFeatureBuilder(
int index) {
return getIndexFeatureFieldBuilder().getBuilder(index);
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder getIndexFeatureOrBuilder(
int index) {
if (indexFeatureBuilder_ == null) {
return indexFeature_.get(index); } else {
return indexFeatureBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public java.util.List extends org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder>
getIndexFeatureOrBuilderList() {
if (indexFeatureBuilder_ != null) {
return indexFeatureBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(indexFeature_);
}
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder addIndexFeatureBuilder() {
return getIndexFeatureFieldBuilder().addBuilder(
org.tensorflow.metadata.v0.SparseFeature.IndexFeature.getDefaultInstance());
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder addIndexFeatureBuilder(
int index) {
return getIndexFeatureFieldBuilder().addBuilder(
index, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.getDefaultInstance());
}
/**
*
* Features that represent indexes. Should be integers >= 0.
*
*
* repeated .tensorflow.metadata.v0.SparseFeature.IndexFeature index_feature = 6;
*/
public java.util.List
getIndexFeatureBuilderList() {
return getIndexFeatureFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.SparseFeature.IndexFeature, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder, org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder>
getIndexFeatureFieldBuilder() {
if (indexFeatureBuilder_ == null) {
indexFeatureBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.metadata.v0.SparseFeature.IndexFeature, org.tensorflow.metadata.v0.SparseFeature.IndexFeature.Builder, org.tensorflow.metadata.v0.SparseFeature.IndexFeatureOrBuilder>(
indexFeature_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
indexFeature_ = null;
}
return indexFeatureBuilder_;
}
private boolean isSorted_ ;
/**
*
* If true then the index values are already sorted lexicographically.
*
*
* optional bool is_sorted = 8;
* @return Whether the isSorted field is set.
*/
@java.lang.Override
public boolean hasIsSorted() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* If true then the index values are already sorted lexicographically.
*
*
* optional bool is_sorted = 8;
* @return The isSorted.
*/
@java.lang.Override
public boolean getIsSorted() {
return isSorted_;
}
/**
*
* If true then the index values are already sorted lexicographically.
*
*
* optional bool is_sorted = 8;
* @param value The isSorted to set.
* @return This builder for chaining.
*/
public Builder setIsSorted(boolean value) {
isSorted_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* If true then the index values are already sorted lexicographically.
*
*
* optional bool is_sorted = 8;
* @return This builder for chaining.
*/
public Builder clearIsSorted() {
bitField0_ = (bitField0_ & ~0x00000040);
isSorted_ = false;
onChanged();
return this;
}
private org.tensorflow.metadata.v0.SparseFeature.ValueFeature valueFeature_;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.SparseFeature.ValueFeature, org.tensorflow.metadata.v0.SparseFeature.ValueFeature.Builder, org.tensorflow.metadata.v0.SparseFeature.ValueFeatureOrBuilder> valueFeatureBuilder_;
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
* @return Whether the valueFeature field is set.
*/
public boolean hasValueFeature() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
* @return The valueFeature.
*/
public org.tensorflow.metadata.v0.SparseFeature.ValueFeature getValueFeature() {
if (valueFeatureBuilder_ == null) {
return valueFeature_ == null ? org.tensorflow.metadata.v0.SparseFeature.ValueFeature.getDefaultInstance() : valueFeature_;
} else {
return valueFeatureBuilder_.getMessage();
}
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
public Builder setValueFeature(org.tensorflow.metadata.v0.SparseFeature.ValueFeature value) {
if (valueFeatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
valueFeature_ = value;
} else {
valueFeatureBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
public Builder setValueFeature(
org.tensorflow.metadata.v0.SparseFeature.ValueFeature.Builder builderForValue) {
if (valueFeatureBuilder_ == null) {
valueFeature_ = builderForValue.build();
} else {
valueFeatureBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
public Builder mergeValueFeature(org.tensorflow.metadata.v0.SparseFeature.ValueFeature value) {
if (valueFeatureBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
valueFeature_ != null &&
valueFeature_ != org.tensorflow.metadata.v0.SparseFeature.ValueFeature.getDefaultInstance()) {
getValueFeatureBuilder().mergeFrom(value);
} else {
valueFeature_ = value;
}
} else {
valueFeatureBuilder_.mergeFrom(value);
}
if (valueFeature_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
public Builder clearValueFeature() {
bitField0_ = (bitField0_ & ~0x00000080);
valueFeature_ = null;
if (valueFeatureBuilder_ != null) {
valueFeatureBuilder_.dispose();
valueFeatureBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
public org.tensorflow.metadata.v0.SparseFeature.ValueFeature.Builder getValueFeatureBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getValueFeatureFieldBuilder().getBuilder();
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
public org.tensorflow.metadata.v0.SparseFeature.ValueFeatureOrBuilder getValueFeatureOrBuilder() {
if (valueFeatureBuilder_ != null) {
return valueFeatureBuilder_.getMessageOrBuilder();
} else {
return valueFeature_ == null ?
org.tensorflow.metadata.v0.SparseFeature.ValueFeature.getDefaultInstance() : valueFeature_;
}
}
/**
*
* required
*
*
* optional .tensorflow.metadata.v0.SparseFeature.ValueFeature value_feature = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.SparseFeature.ValueFeature, org.tensorflow.metadata.v0.SparseFeature.ValueFeature.Builder, org.tensorflow.metadata.v0.SparseFeature.ValueFeatureOrBuilder>
getValueFeatureFieldBuilder() {
if (valueFeatureBuilder_ == null) {
valueFeatureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.metadata.v0.SparseFeature.ValueFeature, org.tensorflow.metadata.v0.SparseFeature.ValueFeature.Builder, org.tensorflow.metadata.v0.SparseFeature.ValueFeatureOrBuilder>(
getValueFeature(),
getParentForChildren(),
isClean());
valueFeature_ = null;
}
return valueFeatureBuilder_;
}
private int type_ = 0;
/**
*
* Type of value feature.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 10 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.type is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=448
* @return Whether the type field is set.
*/
@java.lang.Override @java.lang.Deprecated public boolean hasType() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* Type of value feature.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 10 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.type is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=448
* @return The type.
*/
@java.lang.Override
@java.lang.Deprecated public org.tensorflow.metadata.v0.FeatureType getType() {
org.tensorflow.metadata.v0.FeatureType result = org.tensorflow.metadata.v0.FeatureType.forNumber(type_);
return result == null ? org.tensorflow.metadata.v0.FeatureType.TYPE_UNKNOWN : result;
}
/**
*
* Type of value feature.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 10 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.type is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=448
* @param value The type to set.
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder setType(org.tensorflow.metadata.v0.FeatureType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
type_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Type of value feature.
* Deprecated, this is inferred by the referred features.
*
*
* optional .tensorflow.metadata.v0.FeatureType type = 10 [deprecated = true];
* @deprecated tensorflow.metadata.v0.SparseFeature.type is deprecated.
* See tensorflow_metadata/proto/v0/schema.proto;l=448
* @return This builder for chaining.
*/
@java.lang.Deprecated public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000100);
type_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.metadata.v0.SparseFeature)
}
// @@protoc_insertion_point(class_scope:tensorflow.metadata.v0.SparseFeature)
private static final org.tensorflow.metadata.v0.SparseFeature DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.metadata.v0.SparseFeature();
}
public static org.tensorflow.metadata.v0.SparseFeature getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SparseFeature parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.tensorflow.metadata.v0.SparseFeature getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy