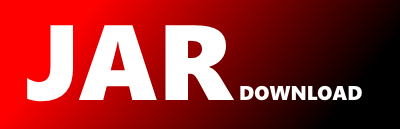
com.spotify.styx.model.ExecutionDescriptionBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class ExecutionDescriptionBuilder {
private String dockerImage;
private List dockerArgs;
private boolean dockerTerminationLogging;
private Optional secret;
private Optional serviceAccount;
private Optional commitSha;
public ExecutionDescriptionBuilder() {
this.secret = Optional.empty();
this.serviceAccount = Optional.empty();
this.commitSha = Optional.empty();
}
private ExecutionDescriptionBuilder(ExecutionDescription v) {
this.dockerImage = v.dockerImage();
List extends String> _dockerArgs = v.dockerArgs();
this.dockerArgs = (_dockerArgs == null) ? null : new ArrayList(_dockerArgs);
this.dockerTerminationLogging = v.dockerTerminationLogging();
this.secret = v.secret();
this.serviceAccount = v.serviceAccount();
this.commitSha = v.commitSha();
}
private ExecutionDescriptionBuilder(ExecutionDescriptionBuilder v) {
this.dockerImage = v.dockerImage;
this.dockerArgs = (v.dockerArgs == null) ? null : new ArrayList(v.dockerArgs);
this.dockerTerminationLogging = v.dockerTerminationLogging;
this.secret = v.secret;
this.serviceAccount = v.serviceAccount;
this.commitSha = v.commitSha;
}
public String dockerImage() {
return dockerImage;
}
public ExecutionDescriptionBuilder dockerImage(String dockerImage) {
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
this.dockerImage = dockerImage;
return this;
}
public List dockerArgs() {
if (this.dockerArgs == null) {
this.dockerArgs = new ArrayList();
}
return dockerArgs;
}
public ExecutionDescriptionBuilder dockerArgs(List extends String> dockerArgs) {
return dockerArgs((Collection extends String>) dockerArgs);
}
public ExecutionDescriptionBuilder dockerArgs(Collection extends String> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
for (String item : dockerArgs) {
if (item == null) {
throw new NullPointerException("dockerArgs: null item");
}
}
this.dockerArgs = new ArrayList(dockerArgs);
return this;
}
public ExecutionDescriptionBuilder dockerArgs(Iterable extends String> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
if (dockerArgs instanceof Collection) {
return dockerArgs((Collection extends String>) dockerArgs);
}
return dockerArgs(dockerArgs.iterator());
}
public ExecutionDescriptionBuilder dockerArgs(Iterator extends String> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
this.dockerArgs = new ArrayList();
while (dockerArgs.hasNext()) {
String item = dockerArgs.next();
if (item == null) {
throw new NullPointerException("dockerArgs: null item");
}
this.dockerArgs.add(item);
}
return this;
}
@SafeVarargs
public final ExecutionDescriptionBuilder dockerArgs(String... dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
return dockerArgs(Arrays.asList(dockerArgs));
}
public ExecutionDescriptionBuilder addDockerArg(String dockerArg) {
if (dockerArg == null) {
throw new NullPointerException("dockerArg");
}
if (this.dockerArgs == null) {
this.dockerArgs = new ArrayList();
}
dockerArgs.add(dockerArg);
return this;
}
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
public ExecutionDescriptionBuilder dockerTerminationLogging(boolean dockerTerminationLogging) {
this.dockerTerminationLogging = dockerTerminationLogging;
return this;
}
public Optional secret() {
return secret;
}
public ExecutionDescriptionBuilder secret(WorkflowConfiguration.Secret secret) {
return secret(Optional.ofNullable(secret));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder secret(Optional extends WorkflowConfiguration.Secret> secret) {
if (secret == null) {
throw new NullPointerException("secret");
}
this.secret = (Optional)secret;
return this;
}
public Optional serviceAccount() {
return serviceAccount;
}
public ExecutionDescriptionBuilder serviceAccount(String serviceAccount) {
return serviceAccount(Optional.ofNullable(serviceAccount));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder serviceAccount(Optional extends String> serviceAccount) {
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
this.serviceAccount = (Optional)serviceAccount;
return this;
}
public Optional commitSha() {
return commitSha;
}
public ExecutionDescriptionBuilder commitSha(String commitSha) {
return commitSha(Optional.ofNullable(commitSha));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder commitSha(Optional extends String> commitSha) {
if (commitSha == null) {
throw new NullPointerException("commitSha");
}
this.commitSha = (Optional)commitSha;
return this;
}
public ExecutionDescription build() {
List _dockerArgs = (dockerArgs != null) ? Collections.unmodifiableList(new ArrayList(dockerArgs)) : Collections.emptyList();
return new Value(dockerImage, _dockerArgs, dockerTerminationLogging, secret, serviceAccount, commitSha);
}
public static ExecutionDescriptionBuilder from(ExecutionDescription v) {
return new ExecutionDescriptionBuilder(v);
}
public static ExecutionDescriptionBuilder from(ExecutionDescriptionBuilder v) {
return new ExecutionDescriptionBuilder(v);
}
private static final class Value implements ExecutionDescription {
private final String dockerImage;
private final List dockerArgs;
private final boolean dockerTerminationLogging;
private final Optional secret;
private final Optional serviceAccount;
private final Optional commitSha;
private Value(@AutoMatter.Field("dockerImage") String dockerImage, @AutoMatter.Field("dockerArgs") List dockerArgs, @AutoMatter.Field("dockerTerminationLogging") boolean dockerTerminationLogging, @AutoMatter.Field("secret") Optional secret, @AutoMatter.Field("serviceAccount") Optional serviceAccount, @AutoMatter.Field("commitSha") Optional commitSha) {
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
if (secret == null) {
throw new NullPointerException("secret");
}
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
if (commitSha == null) {
throw new NullPointerException("commitSha");
}
this.dockerImage = dockerImage;
this.dockerArgs = (dockerArgs != null) ? dockerArgs : Collections.emptyList();
this.dockerTerminationLogging = dockerTerminationLogging;
this.secret = secret;
this.serviceAccount = serviceAccount;
this.commitSha = commitSha;
}
@AutoMatter.Field
@Override
public String dockerImage() {
return dockerImage;
}
@AutoMatter.Field
@Override
public List dockerArgs() {
return dockerArgs;
}
@AutoMatter.Field
@Override
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
@AutoMatter.Field
@Override
public Optional secret() {
return secret;
}
@AutoMatter.Field
@Override
public Optional serviceAccount() {
return serviceAccount;
}
@AutoMatter.Field
@Override
public Optional commitSha() {
return commitSha;
}
public ExecutionDescriptionBuilder builder() {
return new ExecutionDescriptionBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ExecutionDescription)) {
return false;
}
final ExecutionDescription that = (ExecutionDescription) o;
if (dockerImage != null ? !dockerImage.equals(that.dockerImage()) : that.dockerImage() != null) {
return false;
}
if (dockerArgs != null ? !dockerArgs.equals(that.dockerArgs()) : that.dockerArgs() != null) {
return false;
}
if (dockerTerminationLogging != that.dockerTerminationLogging()) {
return false;
}
if (secret != null ? !secret.equals(that.secret()) : that.secret() != null) {
return false;
}
if (serviceAccount != null ? !serviceAccount.equals(that.serviceAccount()) : that.serviceAccount() != null) {
return false;
}
if (commitSha != null ? !commitSha.equals(that.commitSha()) : that.commitSha() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (dockerImage != null ? dockerImage.hashCode() : 0);
result = 31 * result + (dockerArgs != null ? dockerArgs.hashCode() : 0);
result = 31 * result + (dockerTerminationLogging ? 1231 : 1237);
result = 31 * result + (secret != null ? secret.hashCode() : 0);
result = 31 * result + (serviceAccount != null ? serviceAccount.hashCode() : 0);
result = 31 * result + (commitSha != null ? commitSha.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "ExecutionDescription{" +
"dockerImage=" + dockerImage +
", dockerArgs=" + dockerArgs +
", dockerTerminationLogging=" + dockerTerminationLogging +
", secret=" + secret +
", serviceAccount=" + serviceAccount +
", commitSha=" + commitSha +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy