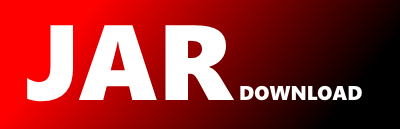
com.spotify.styx.model.data.AutoValue_Trigger Maven / Gradle / Ivy
package com.spotify.styx.model.data;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.Instant;
import java.util.List;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Trigger extends Trigger {
private final String triggerId;
private final Instant timestamp;
private final boolean complete;
private final List executions;
AutoValue_Trigger(
String triggerId,
Instant timestamp,
boolean complete,
List executions) {
if (triggerId == null) {
throw new NullPointerException("Null triggerId");
}
this.triggerId = triggerId;
if (timestamp == null) {
throw new NullPointerException("Null timestamp");
}
this.timestamp = timestamp;
this.complete = complete;
if (executions == null) {
throw new NullPointerException("Null executions");
}
this.executions = executions;
}
@JsonProperty
@Override
public String triggerId() {
return triggerId;
}
@JsonProperty
@Override
public Instant timestamp() {
return timestamp;
}
@JsonProperty
@Override
public boolean complete() {
return complete;
}
@JsonProperty
@Override
public List executions() {
return executions;
}
@Override
public String toString() {
return "Trigger{"
+ "triggerId=" + triggerId + ", "
+ "timestamp=" + timestamp + ", "
+ "complete=" + complete + ", "
+ "executions=" + executions
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Trigger) {
Trigger that = (Trigger) o;
return (this.triggerId.equals(that.triggerId()))
&& (this.timestamp.equals(that.timestamp()))
&& (this.complete == that.complete())
&& (this.executions.equals(that.executions()));
}
return false;
}
@Override
public int hashCode() {
int h = 1;
h *= 1000003;
h ^= this.triggerId.hashCode();
h *= 1000003;
h ^= this.timestamp.hashCode();
h *= 1000003;
h ^= this.complete ? 1231 : 1237;
h *= 1000003;
h ^= this.executions.hashCode();
return h;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy