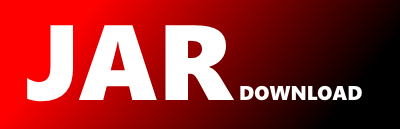
com.spotify.styx.model.BackfillInputBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import java.time.Instant;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
@AutoMatter.Generated
public final class BackfillInputBuilder {
private Instant start;
private Instant end;
private String component;
private String workflow;
private int concurrency;
private boolean reverse;
private Optional description;
private Optional triggerParameters;
public BackfillInputBuilder() {
this.description = Optional.empty();
this.triggerParameters = Optional.empty();
}
private BackfillInputBuilder(BackfillInput v) {
this.start = v.start();
this.end = v.end();
this.component = v.component();
this.workflow = v.workflow();
this.concurrency = v.concurrency();
this.reverse = v.reverse();
this.description = v.description();
this.triggerParameters = v.triggerParameters();
}
private BackfillInputBuilder(BackfillInputBuilder v) {
this.start = v.start();
this.end = v.end();
this.component = v.component();
this.workflow = v.workflow();
this.concurrency = v.concurrency();
this.reverse = v.reverse();
this.description = v.description();
this.triggerParameters = v.triggerParameters();
}
public Instant start() {
return start;
}
public BackfillInputBuilder start(Instant start) {
if (start == null) {
throw new NullPointerException("start");
}
this.start = start;
return this;
}
public Instant end() {
return end;
}
public BackfillInputBuilder end(Instant end) {
if (end == null) {
throw new NullPointerException("end");
}
this.end = end;
return this;
}
public String component() {
return component;
}
public BackfillInputBuilder component(String component) {
if (component == null) {
throw new NullPointerException("component");
}
this.component = component;
return this;
}
public String workflow() {
return workflow;
}
public BackfillInputBuilder workflow(String workflow) {
if (workflow == null) {
throw new NullPointerException("workflow");
}
this.workflow = workflow;
return this;
}
public int concurrency() {
return concurrency;
}
public BackfillInputBuilder concurrency(int concurrency) {
this.concurrency = concurrency;
return this;
}
public boolean reverse() {
return reverse;
}
public BackfillInputBuilder reverse(boolean reverse) {
this.reverse = reverse;
return this;
}
public Optional description() {
return description;
}
public BackfillInputBuilder description(String description) {
return description(Optional.ofNullable(description));
}
@SuppressWarnings("unchecked")
public BackfillInputBuilder description(Optional extends String> description) {
if (description == null) {
throw new NullPointerException("description");
}
this.description = (Optional)description;
return this;
}
public Optional triggerParameters() {
return triggerParameters;
}
public BackfillInputBuilder triggerParameters(TriggerParameters triggerParameters) {
return triggerParameters(Optional.ofNullable(triggerParameters));
}
@SuppressWarnings("unchecked")
public BackfillInputBuilder triggerParameters(
Optional extends TriggerParameters> triggerParameters) {
if (triggerParameters == null) {
throw new NullPointerException("triggerParameters");
}
this.triggerParameters = (Optional)triggerParameters;
return this;
}
public BackfillInputBuilder builder() {
return new BackfillInputBuilder(this);
}
public BackfillInput build() {
return new Value(start, end, component, workflow, concurrency, reverse, description, triggerParameters);
}
public static BackfillInputBuilder from(BackfillInput v) {
return new BackfillInputBuilder(v);
}
public static BackfillInputBuilder from(BackfillInputBuilder v) {
return new BackfillInputBuilder(v);
}
@AutoMatter.Generated
private static final class Value implements BackfillInput {
private final Instant start;
private final Instant end;
private final String component;
private final String workflow;
private final int concurrency;
private final boolean reverse;
private final Optional description;
private final Optional triggerParameters;
private Value(@AutoMatter.Field("start") Instant start, @AutoMatter.Field("end") Instant end,
@AutoMatter.Field("component") String component,
@AutoMatter.Field("workflow") String workflow,
@AutoMatter.Field("concurrency") int concurrency,
@AutoMatter.Field("reverse") boolean reverse,
@AutoMatter.Field("description") Optional description,
@AutoMatter.Field("triggerParameters") Optional triggerParameters) {
if (start == null) {
throw new NullPointerException("start");
}
if (end == null) {
throw new NullPointerException("end");
}
if (component == null) {
throw new NullPointerException("component");
}
if (workflow == null) {
throw new NullPointerException("workflow");
}
if (description == null) {
throw new NullPointerException("description");
}
if (triggerParameters == null) {
throw new NullPointerException("triggerParameters");
}
this.start = start;
this.end = end;
this.component = component;
this.workflow = workflow;
this.concurrency = concurrency;
this.reverse = reverse;
this.description = description;
this.triggerParameters = triggerParameters;
}
@AutoMatter.Field
@Override
public Instant start() {
return start;
}
@AutoMatter.Field
@Override
public Instant end() {
return end;
}
@AutoMatter.Field
@Override
public String component() {
return component;
}
@AutoMatter.Field
@Override
public String workflow() {
return workflow;
}
@AutoMatter.Field
@Override
public int concurrency() {
return concurrency;
}
@AutoMatter.Field
@Override
public boolean reverse() {
return reverse;
}
@AutoMatter.Field
@Override
public Optional description() {
return description;
}
@AutoMatter.Field
@Override
public Optional triggerParameters() {
return triggerParameters;
}
@Override
public BackfillInputBuilder builder() {
return new BackfillInputBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof BackfillInput)) {
return false;
}
final BackfillInput that = (BackfillInput) o;
if (start != null ? !start.equals(that.start()) : that.start() != null) {
return false;
}
if (end != null ? !end.equals(that.end()) : that.end() != null) {
return false;
}
if (component != null ? !component.equals(that.component()) : that.component() != null) {
return false;
}
if (workflow != null ? !workflow.equals(that.workflow()) : that.workflow() != null) {
return false;
}
if (concurrency != that.concurrency()) {
return false;
}
if (reverse != that.reverse()) {
return false;
}
if (description != null ? !description.equals(that.description()) : that.description() != null) {
return false;
}
if (triggerParameters != null ? !triggerParameters.equals(that.triggerParameters()) : that.triggerParameters() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + (this.start != null ? this.start.hashCode() : 0);
result = 31 * result + (this.end != null ? this.end.hashCode() : 0);
result = 31 * result + (this.component != null ? this.component.hashCode() : 0);
result = 31 * result + (this.workflow != null ? this.workflow.hashCode() : 0);
result = 31 * result + this.concurrency;
result = 31 * result + (this.reverse ? 1231 : 1237);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.triggerParameters != null ? this.triggerParameters.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "BackfillInput{" +
"start=" + start +
", end=" + end +
", component=" + component +
", workflow=" + workflow +
", concurrency=" + concurrency +
", reverse=" + reverse +
", description=" + description +
", triggerParameters=" + triggerParameters +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy