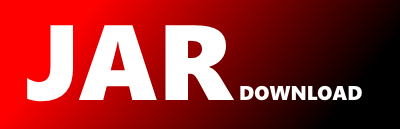
com.spotify.styx.model.DockerExecConfBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import java.util.List;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
@AutoMatter.Generated
public final class DockerExecConfBuilder {
private Optional dockerImage;
private Optional> dockerArgs;
private boolean dockerTerminationLogging;
public DockerExecConfBuilder() {
this.dockerImage = Optional.empty();
this.dockerArgs = Optional.empty();
}
private DockerExecConfBuilder(DockerExecConf v) {
this.dockerImage = v.dockerImage();
this.dockerArgs = v.dockerArgs();
this.dockerTerminationLogging = v.dockerTerminationLogging();
}
private DockerExecConfBuilder(DockerExecConfBuilder v) {
this.dockerImage = v.dockerImage();
this.dockerArgs = v.dockerArgs();
this.dockerTerminationLogging = v.dockerTerminationLogging();
}
public Optional dockerImage() {
return dockerImage;
}
public DockerExecConfBuilder dockerImage(String dockerImage) {
return dockerImage(Optional.ofNullable(dockerImage));
}
@SuppressWarnings("unchecked")
public DockerExecConfBuilder dockerImage(Optional extends String> dockerImage) {
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
this.dockerImage = (Optional)dockerImage;
return this;
}
public Optional> dockerArgs() {
return dockerArgs;
}
public DockerExecConfBuilder dockerArgs(List dockerArgs) {
return dockerArgs(Optional.ofNullable(dockerArgs));
}
@SuppressWarnings("unchecked")
public DockerExecConfBuilder dockerArgs(Optional extends List> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
this.dockerArgs = (Optional>)dockerArgs;
return this;
}
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
public DockerExecConfBuilder dockerTerminationLogging(boolean dockerTerminationLogging) {
this.dockerTerminationLogging = dockerTerminationLogging;
return this;
}
public DockerExecConf build() {
return new Value(dockerImage, dockerArgs, dockerTerminationLogging);
}
public static DockerExecConfBuilder from(DockerExecConf v) {
return new DockerExecConfBuilder(v);
}
public static DockerExecConfBuilder from(DockerExecConfBuilder v) {
return new DockerExecConfBuilder(v);
}
@AutoMatter.Generated
private static final class Value implements DockerExecConf {
private final Optional dockerImage;
private final Optional> dockerArgs;
private final boolean dockerTerminationLogging;
private Value(@AutoMatter.Field("dockerImage") Optional dockerImage,
@AutoMatter.Field("dockerArgs") Optional> dockerArgs,
@AutoMatter.Field("dockerTerminationLogging") boolean dockerTerminationLogging) {
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
this.dockerImage = dockerImage;
this.dockerArgs = dockerArgs;
this.dockerTerminationLogging = dockerTerminationLogging;
}
@AutoMatter.Field
@Override
public Optional dockerImage() {
return dockerImage;
}
@AutoMatter.Field
@Override
public Optional> dockerArgs() {
return dockerArgs;
}
@AutoMatter.Field
@Override
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
public DockerExecConfBuilder builder() {
return new DockerExecConfBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof DockerExecConf)) {
return false;
}
final DockerExecConf that = (DockerExecConf) o;
if (dockerImage != null ? !dockerImage.equals(that.dockerImage()) : that.dockerImage() != null) {
return false;
}
if (dockerArgs != null ? !dockerArgs.equals(that.dockerArgs()) : that.dockerArgs() != null) {
return false;
}
if (dockerTerminationLogging != that.dockerTerminationLogging()) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + (this.dockerImage != null ? this.dockerImage.hashCode() : 0);
result = 31 * result + (this.dockerArgs != null ? this.dockerArgs.hashCode() : 0);
result = 31 * result + (this.dockerTerminationLogging ? 1231 : 1237);
return result;
}
@Override
public String toString() {
return "DockerExecConf{" +
"dockerImage=" + dockerImage +
", dockerArgs=" + dockerArgs +
", dockerTerminationLogging=" + dockerTerminationLogging +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy