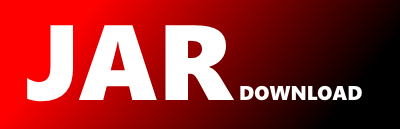
com.spotify.styx.model.Event Maven / Gradle / Ivy
package com.spotify.styx.model;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Generated;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import com.spotify.styx.state.Message;
import com.spotify.styx.state.Trigger;
@Generated("com.github.sviperll.adt4j.GenerateValueClassForVisitorProcessor")
@ParametersAreNonnullByDefault
public class Event {
private final EventAcceptor acceptor;
@SuppressWarnings({
"unchecked",
"rawtypes"
})
private final static EventFactory FACTORY = new EventFactory();
private Event(EventAcceptor acceptor) {
this.acceptor = acceptor;
}
@SuppressWarnings({
"null"
})
protected Event(
@Nonnull
Event implementation) {
if (implementation == null) {
throw new NullPointerException("Argument shouldn't be null: 'implementation' argument in class constructor invocation: com.spotify.styx.model.Event");
}
this.acceptor = implementation.acceptor;
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event created(
@Nonnull
WorkflowInstance workflowInstance,
@Nonnull
String executionId,
@Nonnull
String dockerImage) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'created' in class com.spotify.styx.model.Event");
}
if (executionId == null) {
throw new NullPointerException("Argument shouldn't be null: 'executionId' argument in static method invocation: 'created' in class com.spotify.styx.model.Event");
}
if (dockerImage == null) {
throw new NullPointerException("Argument shouldn't be null: 'dockerImage' argument in static method invocation: 'created' in class com.spotify.styx.model.Event");
}
return new Event(new CreatedCaseEventAcceptor(workflowInstance, executionId, dockerImage));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event dequeue(
@Nonnull
WorkflowInstance workflowInstance,
@Nonnull
Set resourceIds) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'dequeue' in class com.spotify.styx.model.Event");
}
if (resourceIds == null) {
throw new NullPointerException("Argument shouldn't be null: 'resourceIds' argument in static method invocation: 'dequeue' in class com.spotify.styx.model.Event");
}
return new Event(new DequeueCaseEventAcceptor(workflowInstance, resourceIds));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event halt(
@Nonnull
WorkflowInstance workflowInstance) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'halt' in class com.spotify.styx.model.Event");
}
return new Event(new HaltCaseEventAcceptor(workflowInstance));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event info(
@Nonnull
WorkflowInstance workflowInstance,
@Nonnull
Message message) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'info' in class com.spotify.styx.model.Event");
}
if (message == null) {
throw new NullPointerException("Argument shouldn't be null: 'message' argument in static method invocation: 'info' in class com.spotify.styx.model.Event");
}
return new Event(new InfoCaseEventAcceptor(workflowInstance, message));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event retry(
@Nonnull
WorkflowInstance workflowInstance) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'retry' in class com.spotify.styx.model.Event");
}
return new Event(new RetryCaseEventAcceptor(workflowInstance));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event retryAfter(
@Nonnull
WorkflowInstance workflowInstance, long delayMillis) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'retryAfter' in class com.spotify.styx.model.Event");
}
return new Event(new RetryAfterCaseEventAcceptor(workflowInstance, delayMillis));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event runError(
@Nonnull
WorkflowInstance workflowInstance,
@Nonnull
String message) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'runError' in class com.spotify.styx.model.Event");
}
if (message == null) {
throw new NullPointerException("Argument shouldn't be null: 'message' argument in static method invocation: 'runError' in class com.spotify.styx.model.Event");
}
return new Event(new RunErrorCaseEventAcceptor(workflowInstance, message));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event started(
@Nonnull
WorkflowInstance workflowInstance) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'started' in class com.spotify.styx.model.Event");
}
return new Event(new StartedCaseEventAcceptor(workflowInstance));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event stop(
@Nonnull
WorkflowInstance workflowInstance) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'stop' in class com.spotify.styx.model.Event");
}
return new Event(new StopCaseEventAcceptor(workflowInstance));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event submit(
@Nonnull
WorkflowInstance workflowInstance,
@Nonnull
ExecutionDescription executionDescription,
@Nullable
String executionId) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'submit' in class com.spotify.styx.model.Event");
}
if (executionDescription == null) {
throw new NullPointerException("Argument shouldn't be null: 'executionDescription' argument in static method invocation: 'submit' in class com.spotify.styx.model.Event");
}
return new Event(new SubmitCaseEventAcceptor(workflowInstance, executionDescription, executionId));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event submitted(
@Nonnull
WorkflowInstance workflowInstance,
@Nullable
String executionId,
@Nullable
String runnerId) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'submitted' in class com.spotify.styx.model.Event");
}
return new Event(new SubmittedCaseEventAcceptor(workflowInstance, executionId, runnerId));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event success(
@Nonnull
WorkflowInstance workflowInstance) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'success' in class com.spotify.styx.model.Event");
}
return new Event(new SuccessCaseEventAcceptor(workflowInstance));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event terminate(
@Nonnull
WorkflowInstance workflowInstance,
@Nonnull
Optional exitCode) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'terminate' in class com.spotify.styx.model.Event");
}
if (exitCode == null) {
throw new NullPointerException("Argument shouldn't be null: 'exitCode' argument in static method invocation: 'terminate' in class com.spotify.styx.model.Event");
}
return new Event(new TerminateCaseEventAcceptor(workflowInstance, exitCode));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event timeTrigger(
@Nonnull
WorkflowInstance workflowInstance) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'timeTrigger' in class com.spotify.styx.model.Event");
}
return new Event(new TimeTriggerCaseEventAcceptor(workflowInstance));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event timeout(
@Nonnull
WorkflowInstance workflowInstance) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'timeout' in class com.spotify.styx.model.Event");
}
return new Event(new TimeoutCaseEventAcceptor(workflowInstance));
}
@Nonnull
@SuppressWarnings({
"null"
})
public static Event triggerExecution(
@Nonnull
WorkflowInstance workflowInstance,
@Nonnull
Trigger trigger,
@Nonnull
TriggerParameters parameters) {
if (workflowInstance == null) {
throw new NullPointerException("Argument shouldn't be null: 'workflowInstance' argument in static method invocation: 'triggerExecution' in class com.spotify.styx.model.Event");
}
if (trigger == null) {
throw new NullPointerException("Argument shouldn't be null: 'trigger' argument in static method invocation: 'triggerExecution' in class com.spotify.styx.model.Event");
}
if (parameters == null) {
throw new NullPointerException("Argument shouldn't be null: 'parameters' argument in static method invocation: 'triggerExecution' in class com.spotify.styx.model.Event");
}
return new Event(new TriggerExecutionCaseEventAcceptor(workflowInstance, trigger, parameters));
}
public final R accept(EventVisitor visitor) {
return acceptor.accept(visitor);
}
@Nonnull
public final WorkflowInstance workflowInstance() {
return this.acceptor.workflowInstance();
}
@Override
public final boolean equals(Object thatObject) {
if (this == thatObject) {
return true;
} else {
if (!(thatObject instanceof Event)) {
return false;
} else {
Event that = ((Event) thatObject);
return this.acceptor.eventEquals(that.acceptor);
}
}
}
@Override
public final int hashCode() {
return this.acceptor.eventHashCode();
}
@Override
@Nonnull
public final String toString() {
return this.acceptor.toString();
}
@Nonnull
@SuppressWarnings("unchecked")
public static EventVisitor factory() {
return FACTORY;
}
private static class CreatedCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final String executionId;
private final String dockerImage;
CreatedCaseEventAcceptor(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
this.workflowInstance = workflowInstance;
this.executionId = executionId;
this.dockerImage = dockerImage;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.created(this.workflowInstance, this.executionId, this.dockerImage);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsCreated(this.workflowInstance, this.executionId, this.dockerImage);
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
if (!executionId.equals(this.executionId)) {
return false;
}
return dockerImage.equals(this.dockerImage);
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 1;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+ this.executionId.hashCode());
result = ((result* 37)+ this.dockerImage.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Created{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("executionId = ");
result.append(this.executionId);
result.append(", ");
result.append("dockerImage = ");
result.append(this.dockerImage);
result.append("}");
return result.toString();
}
}
private static class DequeueCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final Set resourceIds;
DequeueCaseEventAcceptor(WorkflowInstance workflowInstance, Set resourceIds) {
this.workflowInstance = workflowInstance;
this.resourceIds = resourceIds;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.dequeue(this.workflowInstance, this.resourceIds);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsDequeue(this.workflowInstance, this.resourceIds);
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
return resourceIds.equals(this.resourceIds);
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 2;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+ this.resourceIds.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Dequeue{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("resourceIds = ");
result.append(this.resourceIds);
result.append("}");
return result.toString();
}
}
private interface EventAcceptor {
public R accept(EventVisitor visitor);
@Nonnull
public WorkflowInstance workflowInstance();
public boolean eventEquals(EventAcceptor thatAcceptor);
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage);
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds);
public boolean eventEqualsHalt(WorkflowInstance workflowInstance);
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message);
public boolean eventEqualsRetry(WorkflowInstance workflowInstance);
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis);
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message);
public boolean eventEqualsStarted(WorkflowInstance workflowInstance);
public boolean eventEqualsStop(WorkflowInstance workflowInstance);
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId);
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId);
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance);
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode);
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance);
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance);
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters);
public int eventHashCode();
}
private static class EventFactory
implements EventVisitor
{
@Nonnull
@Override
public Event created(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return Event.created(workflowInstance, executionId, dockerImage);
}
@Nonnull
@Override
public Event dequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return Event.dequeue(workflowInstance, resourceIds);
}
@Nonnull
@Override
public Event halt(WorkflowInstance workflowInstance) {
return Event.halt(workflowInstance);
}
@Nonnull
@Override
public Event info(WorkflowInstance workflowInstance, Message message) {
return Event.info(workflowInstance, message);
}
@Nonnull
@Override
public Event retry(WorkflowInstance workflowInstance) {
return Event.retry(workflowInstance);
}
@Nonnull
@Override
public Event retryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return Event.retryAfter(workflowInstance, delayMillis);
}
@Nonnull
@Override
public Event runError(WorkflowInstance workflowInstance, String message) {
return Event.runError(workflowInstance, message);
}
@Nonnull
@Override
public Event started(WorkflowInstance workflowInstance) {
return Event.started(workflowInstance);
}
@Nonnull
@Override
public Event stop(WorkflowInstance workflowInstance) {
return Event.stop(workflowInstance);
}
@Nonnull
@Override
public Event submit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return Event.submit(workflowInstance, executionDescription, executionId);
}
@Nonnull
@Override
public Event submitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return Event.submitted(workflowInstance, executionId, runnerId);
}
@Nonnull
@Override
public Event success(WorkflowInstance workflowInstance) {
return Event.success(workflowInstance);
}
@Nonnull
@Override
public Event terminate(WorkflowInstance workflowInstance, Optional exitCode) {
return Event.terminate(workflowInstance, exitCode);
}
@Nonnull
@Override
public Event timeTrigger(WorkflowInstance workflowInstance) {
return Event.timeTrigger(workflowInstance);
}
@Nonnull
@Override
public Event timeout(WorkflowInstance workflowInstance) {
return Event.timeout(workflowInstance);
}
@Nonnull
@Override
public Event triggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return Event.triggerExecution(workflowInstance, trigger, parameters);
}
}
private static class HaltCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
HaltCaseEventAcceptor(WorkflowInstance workflowInstance) {
this.workflowInstance = workflowInstance;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.halt(this.workflowInstance);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsHalt(this.workflowInstance);
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return workflowInstance.equals(this.workflowInstance);
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 3;
result = ((result* 37)+ this.workflowInstance.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Halt{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append("}");
return result.toString();
}
}
private static class InfoCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final Message message;
InfoCaseEventAcceptor(WorkflowInstance workflowInstance, Message message) {
this.workflowInstance = workflowInstance;
this.message = message;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.info(this.workflowInstance, this.message);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsInfo(this.workflowInstance, this.message);
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
return message.equals(this.message);
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 4;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+ this.message.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Info{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("message = ");
result.append(this.message);
result.append("}");
return result.toString();
}
}
private static class RetryAfterCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final long delayMillis;
RetryAfterCaseEventAcceptor(WorkflowInstance workflowInstance, long delayMillis) {
this.workflowInstance = workflowInstance;
this.delayMillis = delayMillis;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.retryAfter(this.workflowInstance, this.delayMillis);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsRetryAfter(this.workflowInstance, this.delayMillis);
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
return (delayMillis == this.delayMillis);
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 6;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+((int)(this.delayMillis^(this.delayMillis >>> 32))));
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.RetryAfter{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("delayMillis = ");
result.append(this.delayMillis);
result.append("}");
return result.toString();
}
}
private static class RetryCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
RetryCaseEventAcceptor(WorkflowInstance workflowInstance) {
this.workflowInstance = workflowInstance;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.retry(this.workflowInstance);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsRetry(this.workflowInstance);
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return workflowInstance.equals(this.workflowInstance);
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 5;
result = ((result* 37)+ this.workflowInstance.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Retry{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append("}");
return result.toString();
}
}
private static class RunErrorCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final String message;
RunErrorCaseEventAcceptor(WorkflowInstance workflowInstance, String message) {
this.workflowInstance = workflowInstance;
this.message = message;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.runError(this.workflowInstance, this.message);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsRunError(this.workflowInstance, this.message);
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
return message.equals(this.message);
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 7;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+ this.message.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.RunError{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("message = ");
result.append(this.message);
result.append("}");
return result.toString();
}
}
private static class StartedCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
StartedCaseEventAcceptor(WorkflowInstance workflowInstance) {
this.workflowInstance = workflowInstance;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.started(this.workflowInstance);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsStarted(this.workflowInstance);
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return workflowInstance.equals(this.workflowInstance);
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 8;
result = ((result* 37)+ this.workflowInstance.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Started{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append("}");
return result.toString();
}
}
private static class StopCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
StopCaseEventAcceptor(WorkflowInstance workflowInstance) {
this.workflowInstance = workflowInstance;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.stop(this.workflowInstance);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsStop(this.workflowInstance);
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return workflowInstance.equals(this.workflowInstance);
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 9;
result = ((result* 37)+ this.workflowInstance.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Stop{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append("}");
return result.toString();
}
}
private static class SubmitCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final ExecutionDescription executionDescription;
private final String executionId;
SubmitCaseEventAcceptor(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
this.workflowInstance = workflowInstance;
this.executionDescription = executionDescription;
this.executionId = executionId;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.submit(this.workflowInstance, this.executionDescription, this.executionId);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsSubmit(this.workflowInstance, this.executionDescription, this.executionId);
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
if (!executionDescription.equals(this.executionDescription)) {
return false;
}
if (executionId == null) {
return (this.executionId == null);
} else {
return executionId.equals(this.executionId);
}
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 10;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+ this.executionDescription.hashCode());
if (this.executionId == null) {
result = ((result* 37)+ 0);
} else {
result = ((result* 37)+ this.executionId.hashCode());
}
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Submit{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("executionDescription = ");
result.append(this.executionDescription);
result.append(", ");
result.append("executionId = ");
result.append(this.executionId);
result.append("}");
return result.toString();
}
}
private static class SubmittedCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final String executionId;
private final String runnerId;
SubmittedCaseEventAcceptor(WorkflowInstance workflowInstance, String executionId, String runnerId) {
this.workflowInstance = workflowInstance;
this.executionId = executionId;
this.runnerId = runnerId;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.submitted(this.workflowInstance, this.executionId, this.runnerId);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsSubmitted(this.workflowInstance, this.executionId, this.runnerId);
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
if (executionId == null) {
if (this.executionId!= null) {
return false;
}
} else {
if (!executionId.equals(this.executionId)) {
return false;
}
}
if (runnerId == null) {
return (this.runnerId == null);
} else {
return runnerId.equals(this.runnerId);
}
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 11;
result = ((result* 37)+ this.workflowInstance.hashCode());
if (this.executionId == null) {
result = ((result* 37)+ 0);
} else {
result = ((result* 37)+ this.executionId.hashCode());
}
if (this.runnerId == null) {
result = ((result* 37)+ 0);
} else {
result = ((result* 37)+ this.runnerId.hashCode());
}
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Submitted{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("executionId = ");
result.append(this.executionId);
result.append(", ");
result.append("runnerId = ");
result.append(this.runnerId);
result.append("}");
return result.toString();
}
}
private static class SuccessCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
SuccessCaseEventAcceptor(WorkflowInstance workflowInstance) {
this.workflowInstance = workflowInstance;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.success(this.workflowInstance);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsSuccess(this.workflowInstance);
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return workflowInstance.equals(this.workflowInstance);
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 12;
result = ((result* 37)+ this.workflowInstance.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Success{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append("}");
return result.toString();
}
}
private static class TerminateCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final Optional exitCode;
TerminateCaseEventAcceptor(WorkflowInstance workflowInstance, Optional exitCode) {
this.workflowInstance = workflowInstance;
this.exitCode = exitCode;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.terminate(this.workflowInstance, this.exitCode);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsTerminate(this.workflowInstance, this.exitCode);
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
return exitCode.equals(this.exitCode);
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 13;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+ this.exitCode.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Terminate{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("exitCode = ");
result.append(this.exitCode);
result.append("}");
return result.toString();
}
}
private static class TimeoutCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
TimeoutCaseEventAcceptor(WorkflowInstance workflowInstance) {
this.workflowInstance = workflowInstance;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.timeout(this.workflowInstance);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsTimeout(this.workflowInstance);
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return workflowInstance.equals(this.workflowInstance);
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 15;
result = ((result* 37)+ this.workflowInstance.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.Timeout{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append("}");
return result.toString();
}
}
private static class TimeTriggerCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
TimeTriggerCaseEventAcceptor(WorkflowInstance workflowInstance) {
this.workflowInstance = workflowInstance;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.timeTrigger(this.workflowInstance);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsTimeTrigger(this.workflowInstance);
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return workflowInstance.equals(this.workflowInstance);
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
return false;
}
@Override
public final int eventHashCode() {
int result = 14;
result = ((result* 37)+ this.workflowInstance.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.TimeTrigger{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append("}");
return result.toString();
}
}
private static class TriggerExecutionCaseEventAcceptor
implements EventAcceptor
{
private final WorkflowInstance workflowInstance;
private final Trigger trigger;
private final TriggerParameters parameters;
TriggerExecutionCaseEventAcceptor(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
this.workflowInstance = workflowInstance;
this.trigger = trigger;
this.parameters = parameters;
}
@Override
public R accept(EventVisitor visitor) {
return visitor.triggerExecution(this.workflowInstance, this.trigger, this.parameters);
}
@Override
@Nonnull
public final WorkflowInstance workflowInstance() {
return workflowInstance;
}
@Override
public boolean eventEqualsCreated(WorkflowInstance workflowInstance, String executionId, String dockerImage) {
return false;
}
@Override
public boolean eventEqualsDequeue(WorkflowInstance workflowInstance, Set resourceIds) {
return false;
}
@Override
public boolean eventEqualsHalt(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsInfo(WorkflowInstance workflowInstance, Message message) {
return false;
}
@Override
public boolean eventEqualsRetry(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsRetryAfter(WorkflowInstance workflowInstance, long delayMillis) {
return false;
}
@Override
public boolean eventEqualsRunError(WorkflowInstance workflowInstance, String message) {
return false;
}
@Override
public boolean eventEqualsStarted(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsStop(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsSubmit(WorkflowInstance workflowInstance, ExecutionDescription executionDescription, String executionId) {
return false;
}
@Override
public boolean eventEqualsSubmitted(WorkflowInstance workflowInstance, String executionId, String runnerId) {
return false;
}
@Override
public boolean eventEqualsSuccess(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTerminate(WorkflowInstance workflowInstance, Optional exitCode) {
return false;
}
@Override
public boolean eventEqualsTimeTrigger(WorkflowInstance workflowInstance) {
return false;
}
@Override
public boolean eventEqualsTimeout(WorkflowInstance workflowInstance) {
return false;
}
@Override
public final boolean eventEquals(EventAcceptor thatAcceptor) {
return thatAcceptor.eventEqualsTriggerExecution(this.workflowInstance, this.trigger, this.parameters);
}
@Override
public boolean eventEqualsTriggerExecution(WorkflowInstance workflowInstance, Trigger trigger, TriggerParameters parameters) {
if (!workflowInstance.equals(this.workflowInstance)) {
return false;
}
if (!trigger.equals(this.trigger)) {
return false;
}
return parameters.equals(this.parameters);
}
@Override
public final int eventHashCode() {
int result = 16;
result = ((result* 37)+ this.workflowInstance.hashCode());
result = ((result* 37)+ this.trigger.hashCode());
result = ((result* 37)+ this.parameters.hashCode());
return result;
}
@Override
@Nonnull
public final String toString() {
StringBuilder result = new StringBuilder();
result.append("Event.TriggerExecution{");
result.append("workflowInstance = ");
result.append(this.workflowInstance);
result.append(", ");
result.append("trigger = ");
result.append(this.trigger);
result.append(", ");
result.append("parameters = ");
result.append(this.parameters);
result.append("}");
return result.toString();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy