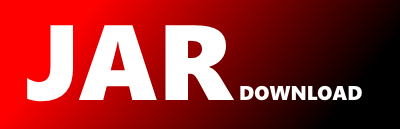
com.spotify.styx.model.ExecutionDescriptionBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import java.time.Duration;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
@AutoMatter.Generated
public final class ExecutionDescriptionBuilder {
private Optional dockerImage;
private List dockerArgs;
private boolean dockerTerminationLogging;
private Optional serviceAccount;
private Optional commitSha;
private Map env;
private Optional runningTimeout;
private Optional retryCondition;
private Optional flyteExecConf;
private Optional flyteExecutionId;
public ExecutionDescriptionBuilder() {
this.dockerImage = Optional.empty();
this.serviceAccount = Optional.empty();
this.commitSha = Optional.empty();
this.runningTimeout = Optional.empty();
this.retryCondition = Optional.empty();
this.flyteExecConf = Optional.empty();
this.flyteExecutionId = Optional.empty();
}
private ExecutionDescriptionBuilder(ExecutionDescription v) {
this.dockerImage = v.dockerImage();
List _dockerArgs = v.dockerArgs();
this.dockerArgs = (_dockerArgs == null) ? null : new ArrayList(_dockerArgs);
this.dockerTerminationLogging = v.dockerTerminationLogging();
this.serviceAccount = v.serviceAccount();
this.commitSha = v.commitSha();
Map _env = v.env();
this.env = (_env == null) ? null : new HashMap(_env);
this.runningTimeout = v.runningTimeout();
this.retryCondition = v.retryCondition();
this.flyteExecConf = v.flyteExecConf();
this.flyteExecutionId = v.flyteExecutionId();
}
private ExecutionDescriptionBuilder(ExecutionDescriptionBuilder v) {
this.dockerImage = v.dockerImage();
this.dockerArgs = new ArrayList(v.dockerArgs());
this.dockerTerminationLogging = v.dockerTerminationLogging();
this.serviceAccount = v.serviceAccount();
this.commitSha = v.commitSha();
this.env = new HashMap(v.env());
this.runningTimeout = v.runningTimeout();
this.retryCondition = v.retryCondition();
this.flyteExecConf = v.flyteExecConf();
this.flyteExecutionId = v.flyteExecutionId();
}
public Optional dockerImage() {
return dockerImage;
}
public ExecutionDescriptionBuilder dockerImage(String dockerImage) {
return dockerImage(Optional.ofNullable(dockerImage));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder dockerImage(Optional extends String> dockerImage) {
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
this.dockerImage = (Optional)dockerImage;
return this;
}
public List dockerArgs() {
if (this.dockerArgs == null) {
this.dockerArgs = new ArrayList();
}
return dockerArgs;
}
public ExecutionDescriptionBuilder dockerArgs(List extends String> dockerArgs) {
return dockerArgs((Collection extends String>) dockerArgs);
}
public ExecutionDescriptionBuilder dockerArgs(Collection extends String> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
for (String item : dockerArgs) {
if (item == null) {
throw new NullPointerException("dockerArgs: null item");
}
}
this.dockerArgs = new ArrayList(dockerArgs);
return this;
}
public ExecutionDescriptionBuilder dockerArgs(Iterable extends String> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
if (dockerArgs instanceof Collection) {
return dockerArgs((Collection extends String>) dockerArgs);
}
return dockerArgs(dockerArgs.iterator());
}
public ExecutionDescriptionBuilder dockerArgs(Iterator extends String> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
this.dockerArgs = new ArrayList();
while (dockerArgs.hasNext()) {
String item = dockerArgs.next();
if (item == null) {
throw new NullPointerException("dockerArgs: null item");
}
this.dockerArgs.add(item);
}
return this;
}
@SafeVarargs
@SuppressWarnings("varargs")
public final ExecutionDescriptionBuilder dockerArgs(String... dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
return dockerArgs(Arrays.asList(dockerArgs));
}
public ExecutionDescriptionBuilder addDockerArg(String dockerArg) {
if (dockerArg == null) {
throw new NullPointerException("dockerArg");
}
if (this.dockerArgs == null) {
this.dockerArgs = new ArrayList();
}
dockerArgs.add(dockerArg);
return this;
}
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
public ExecutionDescriptionBuilder dockerTerminationLogging(boolean dockerTerminationLogging) {
this.dockerTerminationLogging = dockerTerminationLogging;
return this;
}
public Optional serviceAccount() {
return serviceAccount;
}
public ExecutionDescriptionBuilder serviceAccount(String serviceAccount) {
return serviceAccount(Optional.ofNullable(serviceAccount));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder serviceAccount(Optional extends String> serviceAccount) {
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
this.serviceAccount = (Optional)serviceAccount;
return this;
}
public Optional commitSha() {
return commitSha;
}
public ExecutionDescriptionBuilder commitSha(String commitSha) {
return commitSha(Optional.ofNullable(commitSha));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder commitSha(Optional extends String> commitSha) {
if (commitSha == null) {
throw new NullPointerException("commitSha");
}
this.commitSha = (Optional)commitSha;
return this;
}
public Map env() {
if (this.env == null) {
this.env = new HashMap();
}
return env;
}
public ExecutionDescriptionBuilder env(Map extends String, ? extends String> env) {
if (env == null) {
throw new NullPointerException("env");
}
for (Map.Entry extends String, ? extends String> entry : env.entrySet()) {
if (entry.getKey() == null) {
throw new NullPointerException("env: null key");
}
if (entry.getValue() == null) {
throw new NullPointerException("env: null value");
}
}
this.env = new HashMap(env);
return this;
}
public ExecutionDescriptionBuilder env(String k1, String v1) {
if (k1 == null) {
throw new NullPointerException("env: k1");
}
if (v1 == null) {
throw new NullPointerException("env: v1");
}
env = new HashMap();
env.put(k1, v1);
return this;
}
public ExecutionDescriptionBuilder env(String k1, String v1, String k2, String v2) {
env(k1, v1);
if (k2 == null) {
throw new NullPointerException("env: k2");
}
if (v2 == null) {
throw new NullPointerException("env: v2");
}
env.put(k2, v2);
return this;
}
public ExecutionDescriptionBuilder env(String k1, String v1, String k2, String v2, String k3,
String v3) {
env(k1, v1, k2, v2);
if (k3 == null) {
throw new NullPointerException("env: k3");
}
if (v3 == null) {
throw new NullPointerException("env: v3");
}
env.put(k3, v3);
return this;
}
public ExecutionDescriptionBuilder env(String k1, String v1, String k2, String v2, String k3,
String v3, String k4, String v4) {
env(k1, v1, k2, v2, k3, v3);
if (k4 == null) {
throw new NullPointerException("env: k4");
}
if (v4 == null) {
throw new NullPointerException("env: v4");
}
env.put(k4, v4);
return this;
}
public ExecutionDescriptionBuilder env(String k1, String v1, String k2, String v2, String k3,
String v3, String k4, String v4, String k5, String v5) {
env(k1, v1, k2, v2, k3, v3, k4, v4);
if (k5 == null) {
throw new NullPointerException("env: k5");
}
if (v5 == null) {
throw new NullPointerException("env: v5");
}
env.put(k5, v5);
return this;
}
public Optional runningTimeout() {
return runningTimeout;
}
public ExecutionDescriptionBuilder runningTimeout(Duration runningTimeout) {
return runningTimeout(Optional.ofNullable(runningTimeout));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder runningTimeout(Optional extends Duration> runningTimeout) {
if (runningTimeout == null) {
throw new NullPointerException("runningTimeout");
}
this.runningTimeout = (Optional)runningTimeout;
return this;
}
public Optional retryCondition() {
return retryCondition;
}
public ExecutionDescriptionBuilder retryCondition(String retryCondition) {
return retryCondition(Optional.ofNullable(retryCondition));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder retryCondition(Optional extends String> retryCondition) {
if (retryCondition == null) {
throw new NullPointerException("retryCondition");
}
this.retryCondition = (Optional)retryCondition;
return this;
}
public Optional flyteExecConf() {
return flyteExecConf;
}
public ExecutionDescriptionBuilder flyteExecConf(FlyteExecConf flyteExecConf) {
return flyteExecConf(Optional.ofNullable(flyteExecConf));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder flyteExecConf(
Optional extends FlyteExecConf> flyteExecConf) {
if (flyteExecConf == null) {
throw new NullPointerException("flyteExecConf");
}
this.flyteExecConf = (Optional)flyteExecConf;
return this;
}
public Optional flyteExecutionId() {
return flyteExecutionId;
}
public ExecutionDescriptionBuilder flyteExecutionId(String flyteExecutionId) {
return flyteExecutionId(Optional.ofNullable(flyteExecutionId));
}
@SuppressWarnings("unchecked")
public ExecutionDescriptionBuilder flyteExecutionId(Optional extends String> flyteExecutionId) {
if (flyteExecutionId == null) {
throw new NullPointerException("flyteExecutionId");
}
this.flyteExecutionId = (Optional)flyteExecutionId;
return this;
}
public ExecutionDescription build() {
List _dockerArgs = (dockerArgs != null) ? Collections.unmodifiableList(new ArrayList(dockerArgs)) : Collections.emptyList();
Map _env = (env != null) ? Collections.unmodifiableMap(new HashMap(env)) : Collections.emptyMap();
return new Value(dockerImage, _dockerArgs, dockerTerminationLogging, serviceAccount, commitSha, _env, runningTimeout, retryCondition, flyteExecConf, flyteExecutionId);
}
public static ExecutionDescriptionBuilder from(ExecutionDescription v) {
return new ExecutionDescriptionBuilder(v);
}
public static ExecutionDescriptionBuilder from(ExecutionDescriptionBuilder v) {
return new ExecutionDescriptionBuilder(v);
}
@AutoMatter.Generated
private static final class Value implements ExecutionDescription {
private final Optional dockerImage;
private final List dockerArgs;
private final boolean dockerTerminationLogging;
private final Optional serviceAccount;
private final Optional commitSha;
private final Map env;
private final Optional runningTimeout;
private final Optional retryCondition;
private final Optional flyteExecConf;
private final Optional flyteExecutionId;
private Value(@AutoMatter.Field("dockerImage") Optional dockerImage,
@AutoMatter.Field("dockerArgs") List dockerArgs,
@AutoMatter.Field("dockerTerminationLogging") boolean dockerTerminationLogging,
@AutoMatter.Field("serviceAccount") Optional serviceAccount,
@AutoMatter.Field("commitSha") Optional commitSha,
@AutoMatter.Field("env") Map env,
@AutoMatter.Field("runningTimeout") Optional runningTimeout,
@AutoMatter.Field("retryCondition") Optional retryCondition,
@AutoMatter.Field("flyteExecConf") Optional flyteExecConf,
@AutoMatter.Field("flyteExecutionId") Optional flyteExecutionId) {
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
if (commitSha == null) {
throw new NullPointerException("commitSha");
}
if (runningTimeout == null) {
throw new NullPointerException("runningTimeout");
}
if (retryCondition == null) {
throw new NullPointerException("retryCondition");
}
if (flyteExecConf == null) {
throw new NullPointerException("flyteExecConf");
}
if (flyteExecutionId == null) {
throw new NullPointerException("flyteExecutionId");
}
this.dockerImage = dockerImage;
this.dockerArgs = (dockerArgs != null) ? dockerArgs : Collections.emptyList();
this.dockerTerminationLogging = dockerTerminationLogging;
this.serviceAccount = serviceAccount;
this.commitSha = commitSha;
this.env = (env != null) ? env : Collections.emptyMap();
this.runningTimeout = runningTimeout;
this.retryCondition = retryCondition;
this.flyteExecConf = flyteExecConf;
this.flyteExecutionId = flyteExecutionId;
}
@AutoMatter.Field
@Override
public Optional dockerImage() {
return dockerImage;
}
@AutoMatter.Field
@Override
public List dockerArgs() {
return dockerArgs;
}
@AutoMatter.Field
@Override
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
@AutoMatter.Field
@Override
public Optional serviceAccount() {
return serviceAccount;
}
@AutoMatter.Field
@Override
public Optional commitSha() {
return commitSha;
}
@AutoMatter.Field
@Override
public Map env() {
return env;
}
@AutoMatter.Field
@Override
public Optional runningTimeout() {
return runningTimeout;
}
@AutoMatter.Field
@Override
public Optional retryCondition() {
return retryCondition;
}
@AutoMatter.Field
@Override
public Optional flyteExecConf() {
return flyteExecConf;
}
@AutoMatter.Field
@Override
public Optional flyteExecutionId() {
return flyteExecutionId;
}
public ExecutionDescriptionBuilder builder() {
return new ExecutionDescriptionBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ExecutionDescription)) {
return false;
}
final ExecutionDescription that = (ExecutionDescription) o;
if (dockerImage != null ? !dockerImage.equals(that.dockerImage()) : that.dockerImage() != null) {
return false;
}
if (dockerArgs != null ? !dockerArgs.equals(that.dockerArgs()) : that.dockerArgs() != null) {
return false;
}
if (dockerTerminationLogging != that.dockerTerminationLogging()) {
return false;
}
if (serviceAccount != null ? !serviceAccount.equals(that.serviceAccount()) : that.serviceAccount() != null) {
return false;
}
if (commitSha != null ? !commitSha.equals(that.commitSha()) : that.commitSha() != null) {
return false;
}
if (env != null ? !env.equals(that.env()) : that.env() != null) {
return false;
}
if (runningTimeout != null ? !runningTimeout.equals(that.runningTimeout()) : that.runningTimeout() != null) {
return false;
}
if (retryCondition != null ? !retryCondition.equals(that.retryCondition()) : that.retryCondition() != null) {
return false;
}
if (flyteExecConf != null ? !flyteExecConf.equals(that.flyteExecConf()) : that.flyteExecConf() != null) {
return false;
}
if (flyteExecutionId != null ? !flyteExecutionId.equals(that.flyteExecutionId()) : that.flyteExecutionId() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + (this.dockerImage != null ? this.dockerImage.hashCode() : 0);
result = 31 * result + (this.dockerArgs != null ? this.dockerArgs.hashCode() : 0);
result = 31 * result + (this.dockerTerminationLogging ? 1231 : 1237);
result = 31 * result + (this.serviceAccount != null ? this.serviceAccount.hashCode() : 0);
result = 31 * result + (this.commitSha != null ? this.commitSha.hashCode() : 0);
result = 31 * result + (this.env != null ? this.env.hashCode() : 0);
result = 31 * result + (this.runningTimeout != null ? this.runningTimeout.hashCode() : 0);
result = 31 * result + (this.retryCondition != null ? this.retryCondition.hashCode() : 0);
result = 31 * result + (this.flyteExecConf != null ? this.flyteExecConf.hashCode() : 0);
result = 31 * result + (this.flyteExecutionId != null ? this.flyteExecutionId.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "ExecutionDescription{" +
"dockerImage=" + dockerImage +
", dockerArgs=" + dockerArgs +
", dockerTerminationLogging=" + dockerTerminationLogging +
", serviceAccount=" + serviceAccount +
", commitSha=" + commitSha +
", env=" + env +
", runningTimeout=" + runningTimeout +
", retryCondition=" + retryCondition +
", flyteExecConf=" + flyteExecConf +
", flyteExecutionId=" + flyteExecutionId +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy