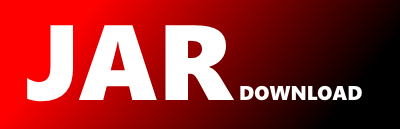
com.spotify.styx.api.TestServiceAccountUsageAuthorizationResponseBuilder Maven / Gradle / Ivy
package com.spotify.styx.api;
import io.norberg.automatter.AutoMatter;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
@AutoMatter.Generated
public final class TestServiceAccountUsageAuthorizationResponseBuilder {
private boolean authorized;
private boolean blacklisted;
private Optional message;
private String serviceAccount;
private String principal;
public TestServiceAccountUsageAuthorizationResponseBuilder() {
this.message = Optional.empty();
}
private TestServiceAccountUsageAuthorizationResponseBuilder(
TestServiceAccountUsageAuthorizationResponse v) {
this.authorized = v.authorized();
this.blacklisted = v.blacklisted();
this.message = v.message();
this.serviceAccount = v.serviceAccount();
this.principal = v.principal();
}
private TestServiceAccountUsageAuthorizationResponseBuilder(
TestServiceAccountUsageAuthorizationResponseBuilder v) {
this.authorized = v.authorized();
this.blacklisted = v.blacklisted();
this.message = v.message();
this.serviceAccount = v.serviceAccount();
this.principal = v.principal();
}
public boolean authorized() {
return authorized;
}
public TestServiceAccountUsageAuthorizationResponseBuilder authorized(boolean authorized) {
this.authorized = authorized;
return this;
}
public boolean blacklisted() {
return blacklisted;
}
public TestServiceAccountUsageAuthorizationResponseBuilder blacklisted(boolean blacklisted) {
this.blacklisted = blacklisted;
return this;
}
public Optional message() {
return message;
}
public TestServiceAccountUsageAuthorizationResponseBuilder message(String message) {
return message(Optional.ofNullable(message));
}
@SuppressWarnings("unchecked")
public TestServiceAccountUsageAuthorizationResponseBuilder message(
Optional extends String> message) {
if (message == null) {
throw new NullPointerException("message");
}
this.message = (Optional)message;
return this;
}
public String serviceAccount() {
return serviceAccount;
}
public TestServiceAccountUsageAuthorizationResponseBuilder serviceAccount(String serviceAccount) {
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
this.serviceAccount = serviceAccount;
return this;
}
public String principal() {
return principal;
}
public TestServiceAccountUsageAuthorizationResponseBuilder principal(String principal) {
if (principal == null) {
throw new NullPointerException("principal");
}
this.principal = principal;
return this;
}
public TestServiceAccountUsageAuthorizationResponse build() {
return new Value(authorized, blacklisted, message, serviceAccount, principal);
}
public static TestServiceAccountUsageAuthorizationResponseBuilder from(
TestServiceAccountUsageAuthorizationResponse v) {
return new TestServiceAccountUsageAuthorizationResponseBuilder(v);
}
public static TestServiceAccountUsageAuthorizationResponseBuilder from(
TestServiceAccountUsageAuthorizationResponseBuilder v) {
return new TestServiceAccountUsageAuthorizationResponseBuilder(v);
}
@AutoMatter.Generated
private static final class Value implements TestServiceAccountUsageAuthorizationResponse {
private final boolean authorized;
private final boolean blacklisted;
private final Optional message;
private final String serviceAccount;
private final String principal;
private Value(@AutoMatter.Field("authorized") boolean authorized,
@AutoMatter.Field("blacklisted") boolean blacklisted,
@AutoMatter.Field("message") Optional message,
@AutoMatter.Field("serviceAccount") String serviceAccount,
@AutoMatter.Field("principal") String principal) {
if (message == null) {
throw new NullPointerException("message");
}
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
if (principal == null) {
throw new NullPointerException("principal");
}
this.authorized = authorized;
this.blacklisted = blacklisted;
this.message = message;
this.serviceAccount = serviceAccount;
this.principal = principal;
}
@AutoMatter.Field
@Override
public boolean authorized() {
return authorized;
}
@AutoMatter.Field
@Override
public boolean blacklisted() {
return blacklisted;
}
@AutoMatter.Field
@Override
public Optional message() {
return message;
}
@AutoMatter.Field
@Override
public String serviceAccount() {
return serviceAccount;
}
@AutoMatter.Field
@Override
public String principal() {
return principal;
}
public TestServiceAccountUsageAuthorizationResponseBuilder builder() {
return new TestServiceAccountUsageAuthorizationResponseBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof TestServiceAccountUsageAuthorizationResponse)) {
return false;
}
final TestServiceAccountUsageAuthorizationResponse that = (TestServiceAccountUsageAuthorizationResponse) o;
if (authorized != that.authorized()) {
return false;
}
if (blacklisted != that.blacklisted()) {
return false;
}
if (message != null ? !message.equals(that.message()) : that.message() != null) {
return false;
}
if (serviceAccount != null ? !serviceAccount.equals(that.serviceAccount()) : that.serviceAccount() != null) {
return false;
}
if (principal != null ? !principal.equals(that.principal()) : that.principal() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + (this.authorized ? 1231 : 1237);
result = 31 * result + (this.blacklisted ? 1231 : 1237);
result = 31 * result + (this.message != null ? this.message.hashCode() : 0);
result = 31 * result + (this.serviceAccount != null ? this.serviceAccount.hashCode() : 0);
result = 31 * result + (this.principal != null ? this.principal.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "TestServiceAccountUsageAuthorizationResponse{" +
"authorized=" + authorized +
", blacklisted=" + blacklisted +
", message=" + message +
", serviceAccount=" + serviceAccount +
", principal=" + principal +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy