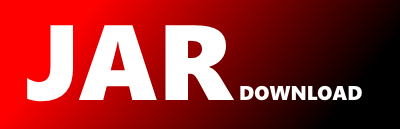
com.spotify.styx.model.BackfillBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import java.time.Instant;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
@AutoMatter.Generated
public final class BackfillBuilder {
private String id;
private Instant start;
private Instant end;
private WorkflowId workflowId;
private int concurrency;
private Optional description;
private Instant nextTrigger;
private Schedule schedule;
private boolean allTriggered;
private boolean halted;
private boolean reverse;
private Optional triggerParameters;
private Optional created;
private Optional lastModified;
public BackfillBuilder() {
this.description = Optional.empty();
this.triggerParameters = Optional.empty();
this.created = Optional.empty();
this.lastModified = Optional.empty();
}
private BackfillBuilder(Backfill v) {
this.id = v.id();
this.start = v.start();
this.end = v.end();
this.workflowId = v.workflowId();
this.concurrency = v.concurrency();
this.description = v.description();
this.nextTrigger = v.nextTrigger();
this.schedule = v.schedule();
this.allTriggered = v.allTriggered();
this.halted = v.halted();
this.reverse = v.reverse();
this.triggerParameters = v.triggerParameters();
this.created = v.created();
this.lastModified = v.lastModified();
}
private BackfillBuilder(BackfillBuilder v) {
this.id = v.id();
this.start = v.start();
this.end = v.end();
this.workflowId = v.workflowId();
this.concurrency = v.concurrency();
this.description = v.description();
this.nextTrigger = v.nextTrigger();
this.schedule = v.schedule();
this.allTriggered = v.allTriggered();
this.halted = v.halted();
this.reverse = v.reverse();
this.triggerParameters = v.triggerParameters();
this.created = v.created();
this.lastModified = v.lastModified();
}
public String id() {
return id;
}
public BackfillBuilder id(String id) {
if (id == null) {
throw new NullPointerException("id");
}
this.id = id;
return this;
}
public Instant start() {
return start;
}
public BackfillBuilder start(Instant start) {
if (start == null) {
throw new NullPointerException("start");
}
this.start = start;
return this;
}
public Instant end() {
return end;
}
public BackfillBuilder end(Instant end) {
if (end == null) {
throw new NullPointerException("end");
}
this.end = end;
return this;
}
public WorkflowId workflowId() {
return workflowId;
}
public BackfillBuilder workflowId(WorkflowId workflowId) {
if (workflowId == null) {
throw new NullPointerException("workflowId");
}
this.workflowId = workflowId;
return this;
}
public int concurrency() {
return concurrency;
}
public BackfillBuilder concurrency(int concurrency) {
this.concurrency = concurrency;
return this;
}
public Optional description() {
return description;
}
public BackfillBuilder description(String description) {
return description(Optional.ofNullable(description));
}
@SuppressWarnings("unchecked")
public BackfillBuilder description(Optional extends String> description) {
if (description == null) {
throw new NullPointerException("description");
}
this.description = (Optional)description;
return this;
}
public Instant nextTrigger() {
return nextTrigger;
}
public BackfillBuilder nextTrigger(Instant nextTrigger) {
if (nextTrigger == null) {
throw new NullPointerException("nextTrigger");
}
this.nextTrigger = nextTrigger;
return this;
}
public Schedule schedule() {
return schedule;
}
public BackfillBuilder schedule(Schedule schedule) {
if (schedule == null) {
throw new NullPointerException("schedule");
}
this.schedule = schedule;
return this;
}
public boolean allTriggered() {
return allTriggered;
}
public BackfillBuilder allTriggered(boolean allTriggered) {
this.allTriggered = allTriggered;
return this;
}
public boolean halted() {
return halted;
}
public BackfillBuilder halted(boolean halted) {
this.halted = halted;
return this;
}
public boolean reverse() {
return reverse;
}
public BackfillBuilder reverse(boolean reverse) {
this.reverse = reverse;
return this;
}
public Optional triggerParameters() {
return triggerParameters;
}
public BackfillBuilder triggerParameters(TriggerParameters triggerParameters) {
return triggerParameters(Optional.ofNullable(triggerParameters));
}
@SuppressWarnings("unchecked")
public BackfillBuilder triggerParameters(
Optional extends TriggerParameters> triggerParameters) {
if (triggerParameters == null) {
throw new NullPointerException("triggerParameters");
}
this.triggerParameters = (Optional)triggerParameters;
return this;
}
public Optional created() {
return created;
}
public BackfillBuilder created(Instant created) {
return created(Optional.ofNullable(created));
}
@SuppressWarnings("unchecked")
public BackfillBuilder created(Optional extends Instant> created) {
if (created == null) {
throw new NullPointerException("created");
}
this.created = (Optional)created;
return this;
}
public Optional lastModified() {
return lastModified;
}
public BackfillBuilder lastModified(Instant lastModified) {
return lastModified(Optional.ofNullable(lastModified));
}
@SuppressWarnings("unchecked")
public BackfillBuilder lastModified(Optional extends Instant> lastModified) {
if (lastModified == null) {
throw new NullPointerException("lastModified");
}
this.lastModified = (Optional)lastModified;
return this;
}
public BackfillBuilder builder() {
return new BackfillBuilder(this);
}
public Backfill build() {
return new Value(id, start, end, workflowId, concurrency, description, nextTrigger, schedule, allTriggered, halted, reverse, triggerParameters, created, lastModified);
}
public static BackfillBuilder from(Backfill v) {
return new BackfillBuilder(v);
}
public static BackfillBuilder from(BackfillBuilder v) {
return new BackfillBuilder(v);
}
@AutoMatter.Generated
private static final class Value implements Backfill {
private final String id;
private final Instant start;
private final Instant end;
private final WorkflowId workflowId;
private final int concurrency;
private final Optional description;
private final Instant nextTrigger;
private final Schedule schedule;
private final boolean allTriggered;
private final boolean halted;
private final boolean reverse;
private final Optional triggerParameters;
private final Optional created;
private final Optional lastModified;
private Value(@AutoMatter.Field("id") String id, @AutoMatter.Field("start") Instant start,
@AutoMatter.Field("end") Instant end, @AutoMatter.Field("workflowId") WorkflowId workflowId,
@AutoMatter.Field("concurrency") int concurrency,
@AutoMatter.Field("description") Optional description,
@AutoMatter.Field("nextTrigger") Instant nextTrigger,
@AutoMatter.Field("schedule") Schedule schedule,
@AutoMatter.Field("allTriggered") boolean allTriggered,
@AutoMatter.Field("halted") boolean halted, @AutoMatter.Field("reverse") boolean reverse,
@AutoMatter.Field("triggerParameters") Optional triggerParameters,
@AutoMatter.Field("created") Optional created,
@AutoMatter.Field("lastModified") Optional lastModified) {
if (id == null) {
throw new NullPointerException("id");
}
if (start == null) {
throw new NullPointerException("start");
}
if (end == null) {
throw new NullPointerException("end");
}
if (workflowId == null) {
throw new NullPointerException("workflowId");
}
if (description == null) {
throw new NullPointerException("description");
}
if (nextTrigger == null) {
throw new NullPointerException("nextTrigger");
}
if (schedule == null) {
throw new NullPointerException("schedule");
}
if (triggerParameters == null) {
throw new NullPointerException("triggerParameters");
}
if (created == null) {
throw new NullPointerException("created");
}
if (lastModified == null) {
throw new NullPointerException("lastModified");
}
this.id = id;
this.start = start;
this.end = end;
this.workflowId = workflowId;
this.concurrency = concurrency;
this.description = description;
this.nextTrigger = nextTrigger;
this.schedule = schedule;
this.allTriggered = allTriggered;
this.halted = halted;
this.reverse = reverse;
this.triggerParameters = triggerParameters;
this.created = created;
this.lastModified = lastModified;
}
@AutoMatter.Field
@Override
public String id() {
return id;
}
@AutoMatter.Field
@Override
public Instant start() {
return start;
}
@AutoMatter.Field
@Override
public Instant end() {
return end;
}
@AutoMatter.Field
@Override
public WorkflowId workflowId() {
return workflowId;
}
@AutoMatter.Field
@Override
public int concurrency() {
return concurrency;
}
@AutoMatter.Field
@Override
public Optional description() {
return description;
}
@AutoMatter.Field
@Override
public Instant nextTrigger() {
return nextTrigger;
}
@AutoMatter.Field
@Override
public Schedule schedule() {
return schedule;
}
@AutoMatter.Field
@Override
public boolean allTriggered() {
return allTriggered;
}
@AutoMatter.Field
@Override
public boolean halted() {
return halted;
}
@AutoMatter.Field
@Override
public boolean reverse() {
return reverse;
}
@AutoMatter.Field
@Override
public Optional triggerParameters() {
return triggerParameters;
}
@AutoMatter.Field
@Override
public Optional created() {
return created;
}
@AutoMatter.Field
@Override
public Optional lastModified() {
return lastModified;
}
@Override
public BackfillBuilder builder() {
return new BackfillBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Backfill)) {
return false;
}
final Backfill that = (Backfill) o;
if (id != null ? !id.equals(that.id()) : that.id() != null) {
return false;
}
if (start != null ? !start.equals(that.start()) : that.start() != null) {
return false;
}
if (end != null ? !end.equals(that.end()) : that.end() != null) {
return false;
}
if (workflowId != null ? !workflowId.equals(that.workflowId()) : that.workflowId() != null) {
return false;
}
if (concurrency != that.concurrency()) {
return false;
}
if (description != null ? !description.equals(that.description()) : that.description() != null) {
return false;
}
if (nextTrigger != null ? !nextTrigger.equals(that.nextTrigger()) : that.nextTrigger() != null) {
return false;
}
if (schedule != null ? !schedule.equals(that.schedule()) : that.schedule() != null) {
return false;
}
if (allTriggered != that.allTriggered()) {
return false;
}
if (halted != that.halted()) {
return false;
}
if (reverse != that.reverse()) {
return false;
}
if (triggerParameters != null ? !triggerParameters.equals(that.triggerParameters()) : that.triggerParameters() != null) {
return false;
}
if (created != null ? !created.equals(that.created()) : that.created() != null) {
return false;
}
if (lastModified != null ? !lastModified.equals(that.lastModified()) : that.lastModified() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + (this.id != null ? this.id.hashCode() : 0);
result = 31 * result + (this.start != null ? this.start.hashCode() : 0);
result = 31 * result + (this.end != null ? this.end.hashCode() : 0);
result = 31 * result + (this.workflowId != null ? this.workflowId.hashCode() : 0);
result = 31 * result + this.concurrency;
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.nextTrigger != null ? this.nextTrigger.hashCode() : 0);
result = 31 * result + (this.schedule != null ? this.schedule.hashCode() : 0);
result = 31 * result + (this.allTriggered ? 1231 : 1237);
result = 31 * result + (this.halted ? 1231 : 1237);
result = 31 * result + (this.reverse ? 1231 : 1237);
result = 31 * result + (this.triggerParameters != null ? this.triggerParameters.hashCode() : 0);
result = 31 * result + (this.created != null ? this.created.hashCode() : 0);
result = 31 * result + (this.lastModified != null ? this.lastModified.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "Backfill{" +
"id=" + id +
", start=" + start +
", end=" + end +
", workflowId=" + workflowId +
", concurrency=" + concurrency +
", description=" + description +
", nextTrigger=" + nextTrigger +
", schedule=" + schedule +
", allTriggered=" + allTriggered +
", halted=" + halted +
", reverse=" + reverse +
", triggerParameters=" + triggerParameters +
", created=" + created +
", lastModified=" + lastModified +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy