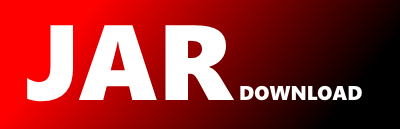
com.spotify.styx.model.FlyteIdentifierBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
@AutoMatter.Generated
public final class FlyteIdentifierBuilder {
private String resourceType;
private String project;
private String domain;
private String name;
private String version;
public FlyteIdentifierBuilder() {
}
private FlyteIdentifierBuilder(FlyteIdentifier v) {
this.resourceType = v.resourceType();
this.project = v.project();
this.domain = v.domain();
this.name = v.name();
this.version = v.version();
}
private FlyteIdentifierBuilder(FlyteIdentifierBuilder v) {
this.resourceType = v.resourceType();
this.project = v.project();
this.domain = v.domain();
this.name = v.name();
this.version = v.version();
}
public String resourceType() {
return resourceType;
}
public FlyteIdentifierBuilder resourceType(String resourceType) {
if (resourceType == null) {
throw new NullPointerException("resourceType");
}
this.resourceType = resourceType;
return this;
}
public String project() {
return project;
}
public FlyteIdentifierBuilder project(String project) {
if (project == null) {
throw new NullPointerException("project");
}
this.project = project;
return this;
}
public String domain() {
return domain;
}
public FlyteIdentifierBuilder domain(String domain) {
if (domain == null) {
throw new NullPointerException("domain");
}
this.domain = domain;
return this;
}
public String name() {
return name;
}
public FlyteIdentifierBuilder name(String name) {
if (name == null) {
throw new NullPointerException("name");
}
this.name = name;
return this;
}
public String version() {
return version;
}
public FlyteIdentifierBuilder version(String version) {
if (version == null) {
throw new NullPointerException("version");
}
this.version = version;
return this;
}
public FlyteIdentifier build() {
return new Value(resourceType, project, domain, name, version);
}
public static FlyteIdentifierBuilder from(FlyteIdentifier v) {
return new FlyteIdentifierBuilder(v);
}
public static FlyteIdentifierBuilder from(FlyteIdentifierBuilder v) {
return new FlyteIdentifierBuilder(v);
}
@AutoMatter.Generated
private static final class Value implements FlyteIdentifier {
private final String resourceType;
private final String project;
private final String domain;
private final String name;
private final String version;
private Value(@AutoMatter.Field("resourceType") String resourceType,
@AutoMatter.Field("project") String project, @AutoMatter.Field("domain") String domain,
@AutoMatter.Field("name") String name, @AutoMatter.Field("version") String version) {
if (resourceType == null) {
throw new NullPointerException("resourceType");
}
if (project == null) {
throw new NullPointerException("project");
}
if (domain == null) {
throw new NullPointerException("domain");
}
if (name == null) {
throw new NullPointerException("name");
}
if (version == null) {
throw new NullPointerException("version");
}
this.resourceType = resourceType;
this.project = project;
this.domain = domain;
this.name = name;
this.version = version;
}
@AutoMatter.Field
@Override
public String resourceType() {
return resourceType;
}
@AutoMatter.Field
@Override
public String project() {
return project;
}
@AutoMatter.Field
@Override
public String domain() {
return domain;
}
@AutoMatter.Field
@Override
public String name() {
return name;
}
@AutoMatter.Field
@Override
public String version() {
return version;
}
public FlyteIdentifierBuilder builder() {
return new FlyteIdentifierBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof FlyteIdentifier)) {
return false;
}
final FlyteIdentifier that = (FlyteIdentifier) o;
if (resourceType != null ? !resourceType.equals(that.resourceType()) : that.resourceType() != null) {
return false;
}
if (project != null ? !project.equals(that.project()) : that.project() != null) {
return false;
}
if (domain != null ? !domain.equals(that.domain()) : that.domain() != null) {
return false;
}
if (name != null ? !name.equals(that.name()) : that.name() != null) {
return false;
}
if (version != null ? !version.equals(that.version()) : that.version() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + (this.resourceType != null ? this.resourceType.hashCode() : 0);
result = 31 * result + (this.project != null ? this.project.hashCode() : 0);
result = 31 * result + (this.domain != null ? this.domain.hashCode() : 0);
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
result = 31 * result + (this.version != null ? this.version.hashCode() : 0);
return result;
}
@Override
public String toString() {
return urn();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy