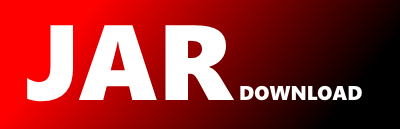
com.spotify.styx.model.WorkflowConfigurationBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import java.time.Duration;
import java.time.Instant;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
@AutoMatter.Generated
public final class WorkflowConfigurationBuilder {
private String id;
private Schedule schedule;
private Optional offset;
private Optional commitSha;
private Optional dockerImage;
private Optional> dockerArgs;
private boolean dockerTerminationLogging;
private Optional serviceAccount;
private List resources;
private Map env;
private Optional runningTimeout;
private Optional retryCondition;
private Optional flyteExecConf;
private Optional deploymentSource;
private Optional deploymentTime;
public WorkflowConfigurationBuilder() {
this.offset = Optional.empty();
this.commitSha = Optional.empty();
this.dockerImage = Optional.empty();
this.dockerArgs = Optional.empty();
this.serviceAccount = Optional.empty();
this.runningTimeout = Optional.empty();
this.retryCondition = Optional.empty();
this.flyteExecConf = Optional.empty();
this.deploymentSource = Optional.empty();
this.deploymentTime = Optional.empty();
}
private WorkflowConfigurationBuilder(WorkflowConfiguration v) {
this.id = v.id();
this.schedule = v.schedule();
this.offset = v.offset();
this.commitSha = v.commitSha();
this.dockerImage = v.dockerImage();
this.dockerArgs = v.dockerArgs();
this.dockerTerminationLogging = v.dockerTerminationLogging();
this.serviceAccount = v.serviceAccount();
List _resources = v.resources();
this.resources = (_resources == null) ? null : new ArrayList(_resources);
Map _env = v.env();
this.env = (_env == null) ? null : new HashMap(_env);
this.runningTimeout = v.runningTimeout();
this.retryCondition = v.retryCondition();
this.flyteExecConf = v.flyteExecConf();
this.deploymentSource = v.deploymentSource();
this.deploymentTime = v.deploymentTime();
}
private WorkflowConfigurationBuilder(WorkflowConfigurationBuilder v) {
this.id = v.id();
this.schedule = v.schedule();
this.offset = v.offset();
this.commitSha = v.commitSha();
this.dockerImage = v.dockerImage();
this.dockerArgs = v.dockerArgs();
this.dockerTerminationLogging = v.dockerTerminationLogging();
this.serviceAccount = v.serviceAccount();
this.resources = new ArrayList(v.resources());
this.env = new HashMap(v.env());
this.runningTimeout = v.runningTimeout();
this.retryCondition = v.retryCondition();
this.flyteExecConf = v.flyteExecConf();
this.deploymentSource = v.deploymentSource();
this.deploymentTime = v.deploymentTime();
}
public String id() {
return id;
}
public WorkflowConfigurationBuilder id(String id) {
if (id == null) {
throw new NullPointerException("id");
}
this.id = id;
return this;
}
public Schedule schedule() {
return schedule;
}
public WorkflowConfigurationBuilder schedule(Schedule schedule) {
if (schedule == null) {
throw new NullPointerException("schedule");
}
this.schedule = schedule;
return this;
}
public Optional offset() {
return offset;
}
public WorkflowConfigurationBuilder offset(String offset) {
return offset(Optional.ofNullable(offset));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder offset(Optional extends String> offset) {
if (offset == null) {
throw new NullPointerException("offset");
}
this.offset = (Optional)offset;
return this;
}
public Optional commitSha() {
return commitSha;
}
public WorkflowConfigurationBuilder commitSha(String commitSha) {
return commitSha(Optional.ofNullable(commitSha));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder commitSha(Optional extends String> commitSha) {
if (commitSha == null) {
throw new NullPointerException("commitSha");
}
this.commitSha = (Optional)commitSha;
return this;
}
public Optional dockerImage() {
return dockerImage;
}
public WorkflowConfigurationBuilder dockerImage(String dockerImage) {
return dockerImage(Optional.ofNullable(dockerImage));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder dockerImage(Optional extends String> dockerImage) {
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
this.dockerImage = (Optional)dockerImage;
return this;
}
public Optional> dockerArgs() {
return dockerArgs;
}
public WorkflowConfigurationBuilder dockerArgs(List dockerArgs) {
return dockerArgs(Optional.ofNullable(dockerArgs));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder dockerArgs(Optional extends List> dockerArgs) {
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
this.dockerArgs = (Optional>)dockerArgs;
return this;
}
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
public WorkflowConfigurationBuilder dockerTerminationLogging(boolean dockerTerminationLogging) {
this.dockerTerminationLogging = dockerTerminationLogging;
return this;
}
public Optional serviceAccount() {
return serviceAccount;
}
public WorkflowConfigurationBuilder serviceAccount(String serviceAccount) {
return serviceAccount(Optional.ofNullable(serviceAccount));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder serviceAccount(Optional extends String> serviceAccount) {
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
this.serviceAccount = (Optional)serviceAccount;
return this;
}
public List resources() {
if (this.resources == null) {
this.resources = new ArrayList();
}
return resources;
}
public WorkflowConfigurationBuilder resources(List extends String> resources) {
return resources((Collection extends String>) resources);
}
public WorkflowConfigurationBuilder resources(Collection extends String> resources) {
if (resources == null) {
throw new NullPointerException("resources");
}
for (String item : resources) {
if (item == null) {
throw new NullPointerException("resources: null item");
}
}
this.resources = new ArrayList(resources);
return this;
}
public WorkflowConfigurationBuilder resources(Iterable extends String> resources) {
if (resources == null) {
throw new NullPointerException("resources");
}
if (resources instanceof Collection) {
return resources((Collection extends String>) resources);
}
return resources(resources.iterator());
}
public WorkflowConfigurationBuilder resources(Iterator extends String> resources) {
if (resources == null) {
throw new NullPointerException("resources");
}
this.resources = new ArrayList();
while (resources.hasNext()) {
String item = resources.next();
if (item == null) {
throw new NullPointerException("resources: null item");
}
this.resources.add(item);
}
return this;
}
@SafeVarargs
@SuppressWarnings("varargs")
public final WorkflowConfigurationBuilder resources(String... resources) {
if (resources == null) {
throw new NullPointerException("resources");
}
return resources(Arrays.asList(resources));
}
public WorkflowConfigurationBuilder addResource(String resource) {
if (resource == null) {
throw new NullPointerException("resource");
}
if (this.resources == null) {
this.resources = new ArrayList();
}
resources.add(resource);
return this;
}
public Map env() {
if (this.env == null) {
this.env = new HashMap();
}
return env;
}
public WorkflowConfigurationBuilder env(Map extends String, ? extends String> env) {
if (env == null) {
throw new NullPointerException("env");
}
for (Map.Entry extends String, ? extends String> entry : env.entrySet()) {
if (entry.getKey() == null) {
throw new NullPointerException("env: null key");
}
if (entry.getValue() == null) {
throw new NullPointerException("env: null value");
}
}
this.env = new HashMap(env);
return this;
}
public WorkflowConfigurationBuilder env(String k1, String v1) {
if (k1 == null) {
throw new NullPointerException("env: k1");
}
if (v1 == null) {
throw new NullPointerException("env: v1");
}
env = new HashMap();
env.put(k1, v1);
return this;
}
public WorkflowConfigurationBuilder env(String k1, String v1, String k2, String v2) {
env(k1, v1);
if (k2 == null) {
throw new NullPointerException("env: k2");
}
if (v2 == null) {
throw new NullPointerException("env: v2");
}
env.put(k2, v2);
return this;
}
public WorkflowConfigurationBuilder env(String k1, String v1, String k2, String v2, String k3,
String v3) {
env(k1, v1, k2, v2);
if (k3 == null) {
throw new NullPointerException("env: k3");
}
if (v3 == null) {
throw new NullPointerException("env: v3");
}
env.put(k3, v3);
return this;
}
public WorkflowConfigurationBuilder env(String k1, String v1, String k2, String v2, String k3,
String v3, String k4, String v4) {
env(k1, v1, k2, v2, k3, v3);
if (k4 == null) {
throw new NullPointerException("env: k4");
}
if (v4 == null) {
throw new NullPointerException("env: v4");
}
env.put(k4, v4);
return this;
}
public WorkflowConfigurationBuilder env(String k1, String v1, String k2, String v2, String k3,
String v3, String k4, String v4, String k5, String v5) {
env(k1, v1, k2, v2, k3, v3, k4, v4);
if (k5 == null) {
throw new NullPointerException("env: k5");
}
if (v5 == null) {
throw new NullPointerException("env: v5");
}
env.put(k5, v5);
return this;
}
public Optional runningTimeout() {
return runningTimeout;
}
public WorkflowConfigurationBuilder runningTimeout(Duration runningTimeout) {
return runningTimeout(Optional.ofNullable(runningTimeout));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder runningTimeout(Optional extends Duration> runningTimeout) {
if (runningTimeout == null) {
throw new NullPointerException("runningTimeout");
}
this.runningTimeout = (Optional)runningTimeout;
return this;
}
public Optional retryCondition() {
return retryCondition;
}
public WorkflowConfigurationBuilder retryCondition(String retryCondition) {
return retryCondition(Optional.ofNullable(retryCondition));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder retryCondition(Optional extends String> retryCondition) {
if (retryCondition == null) {
throw new NullPointerException("retryCondition");
}
this.retryCondition = (Optional)retryCondition;
return this;
}
public Optional flyteExecConf() {
return flyteExecConf;
}
public WorkflowConfigurationBuilder flyteExecConf(FlyteExecConf flyteExecConf) {
return flyteExecConf(Optional.ofNullable(flyteExecConf));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder flyteExecConf(
Optional extends FlyteExecConf> flyteExecConf) {
if (flyteExecConf == null) {
throw new NullPointerException("flyteExecConf");
}
this.flyteExecConf = (Optional)flyteExecConf;
return this;
}
public Optional deploymentSource() {
return deploymentSource;
}
public WorkflowConfigurationBuilder deploymentSource(DeploymentSource deploymentSource) {
return deploymentSource(Optional.ofNullable(deploymentSource));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder deploymentSource(
Optional extends DeploymentSource> deploymentSource) {
if (deploymentSource == null) {
throw new NullPointerException("deploymentSource");
}
this.deploymentSource = (Optional)deploymentSource;
return this;
}
public Optional deploymentTime() {
return deploymentTime;
}
public WorkflowConfigurationBuilder deploymentTime(Instant deploymentTime) {
return deploymentTime(Optional.ofNullable(deploymentTime));
}
@SuppressWarnings("unchecked")
public WorkflowConfigurationBuilder deploymentTime(Optional extends Instant> deploymentTime) {
if (deploymentTime == null) {
throw new NullPointerException("deploymentTime");
}
this.deploymentTime = (Optional)deploymentTime;
return this;
}
public WorkflowConfiguration build() {
List _resources = (resources != null) ? Collections.unmodifiableList(new ArrayList(resources)) : Collections.emptyList();
Map _env = (env != null) ? Collections.unmodifiableMap(new HashMap(env)) : Collections.emptyMap();
return new Value(id, schedule, offset, commitSha, dockerImage, dockerArgs, dockerTerminationLogging, serviceAccount, _resources, _env, runningTimeout, retryCondition, flyteExecConf, deploymentSource, deploymentTime);
}
public static WorkflowConfigurationBuilder from(WorkflowConfiguration v) {
return new WorkflowConfigurationBuilder(v);
}
public static WorkflowConfigurationBuilder from(WorkflowConfigurationBuilder v) {
return new WorkflowConfigurationBuilder(v);
}
@AutoMatter.Generated
private static final class Value implements WorkflowConfiguration {
private final String id;
private final Schedule schedule;
private final Optional offset;
private final Optional commitSha;
private final Optional dockerImage;
private final Optional> dockerArgs;
private final boolean dockerTerminationLogging;
private final Optional serviceAccount;
private final List resources;
private final Map env;
private final Optional runningTimeout;
private final Optional retryCondition;
private final Optional flyteExecConf;
private final Optional deploymentSource;
private final Optional deploymentTime;
private Value(@AutoMatter.Field("id") String id,
@AutoMatter.Field("schedule") Schedule schedule,
@AutoMatter.Field("offset") Optional offset,
@AutoMatter.Field("commitSha") Optional commitSha,
@AutoMatter.Field("dockerImage") Optional dockerImage,
@AutoMatter.Field("dockerArgs") Optional> dockerArgs,
@AutoMatter.Field("dockerTerminationLogging") boolean dockerTerminationLogging,
@AutoMatter.Field("serviceAccount") Optional serviceAccount,
@AutoMatter.Field("resources") List resources,
@AutoMatter.Field("env") Map env,
@AutoMatter.Field("runningTimeout") Optional runningTimeout,
@AutoMatter.Field("retryCondition") Optional retryCondition,
@AutoMatter.Field("flyteExecConf") Optional flyteExecConf,
@AutoMatter.Field("deploymentSource") Optional deploymentSource,
@AutoMatter.Field("deploymentTime") Optional deploymentTime) {
if (id == null) {
throw new NullPointerException("id");
}
if (schedule == null) {
throw new NullPointerException("schedule");
}
if (offset == null) {
throw new NullPointerException("offset");
}
if (commitSha == null) {
throw new NullPointerException("commitSha");
}
if (dockerImage == null) {
throw new NullPointerException("dockerImage");
}
if (dockerArgs == null) {
throw new NullPointerException("dockerArgs");
}
if (serviceAccount == null) {
throw new NullPointerException("serviceAccount");
}
if (runningTimeout == null) {
throw new NullPointerException("runningTimeout");
}
if (retryCondition == null) {
throw new NullPointerException("retryCondition");
}
if (flyteExecConf == null) {
throw new NullPointerException("flyteExecConf");
}
if (deploymentSource == null) {
throw new NullPointerException("deploymentSource");
}
if (deploymentTime == null) {
throw new NullPointerException("deploymentTime");
}
this.id = id;
this.schedule = schedule;
this.offset = offset;
this.commitSha = commitSha;
this.dockerImage = dockerImage;
this.dockerArgs = dockerArgs;
this.dockerTerminationLogging = dockerTerminationLogging;
this.serviceAccount = serviceAccount;
this.resources = (resources != null) ? resources : Collections.emptyList();
this.env = (env != null) ? env : Collections.emptyMap();
this.runningTimeout = runningTimeout;
this.retryCondition = retryCondition;
this.flyteExecConf = flyteExecConf;
this.deploymentSource = deploymentSource;
this.deploymentTime = deploymentTime;
}
@AutoMatter.Field
@Override
public String id() {
return id;
}
@AutoMatter.Field
@Override
public Schedule schedule() {
return schedule;
}
@AutoMatter.Field
@Override
public Optional offset() {
return offset;
}
@AutoMatter.Field
@Override
public Optional commitSha() {
return commitSha;
}
@AutoMatter.Field
@Override
public Optional dockerImage() {
return dockerImage;
}
@AutoMatter.Field
@Override
public Optional> dockerArgs() {
return dockerArgs;
}
@AutoMatter.Field
@Override
public boolean dockerTerminationLogging() {
return dockerTerminationLogging;
}
@AutoMatter.Field
@Override
public Optional serviceAccount() {
return serviceAccount;
}
@AutoMatter.Field
@Override
public List resources() {
return resources;
}
@AutoMatter.Field
@Override
public Map env() {
return env;
}
@AutoMatter.Field
@Override
public Optional runningTimeout() {
return runningTimeout;
}
@AutoMatter.Field
@Override
public Optional retryCondition() {
return retryCondition;
}
@AutoMatter.Field
@Override
public Optional flyteExecConf() {
return flyteExecConf;
}
@AutoMatter.Field
@Override
public Optional deploymentSource() {
return deploymentSource;
}
@AutoMatter.Field
@Override
public Optional deploymentTime() {
return deploymentTime;
}
public WorkflowConfigurationBuilder builder() {
return new WorkflowConfigurationBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof WorkflowConfiguration)) {
return false;
}
final WorkflowConfiguration that = (WorkflowConfiguration) o;
if (id != null ? !id.equals(that.id()) : that.id() != null) {
return false;
}
if (schedule != null ? !schedule.equals(that.schedule()) : that.schedule() != null) {
return false;
}
if (offset != null ? !offset.equals(that.offset()) : that.offset() != null) {
return false;
}
if (commitSha != null ? !commitSha.equals(that.commitSha()) : that.commitSha() != null) {
return false;
}
if (dockerImage != null ? !dockerImage.equals(that.dockerImage()) : that.dockerImage() != null) {
return false;
}
if (dockerArgs != null ? !dockerArgs.equals(that.dockerArgs()) : that.dockerArgs() != null) {
return false;
}
if (dockerTerminationLogging != that.dockerTerminationLogging()) {
return false;
}
if (serviceAccount != null ? !serviceAccount.equals(that.serviceAccount()) : that.serviceAccount() != null) {
return false;
}
if (resources != null ? !resources.equals(that.resources()) : that.resources() != null) {
return false;
}
if (env != null ? !env.equals(that.env()) : that.env() != null) {
return false;
}
if (runningTimeout != null ? !runningTimeout.equals(that.runningTimeout()) : that.runningTimeout() != null) {
return false;
}
if (retryCondition != null ? !retryCondition.equals(that.retryCondition()) : that.retryCondition() != null) {
return false;
}
if (flyteExecConf != null ? !flyteExecConf.equals(that.flyteExecConf()) : that.flyteExecConf() != null) {
return false;
}
if (deploymentSource != null ? !deploymentSource.equals(that.deploymentSource()) : that.deploymentSource() != null) {
return false;
}
if (deploymentTime != null ? !deploymentTime.equals(that.deploymentTime()) : that.deploymentTime() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
result = 31 * result + (this.id != null ? this.id.hashCode() : 0);
result = 31 * result + (this.schedule != null ? this.schedule.hashCode() : 0);
result = 31 * result + (this.offset != null ? this.offset.hashCode() : 0);
result = 31 * result + (this.commitSha != null ? this.commitSha.hashCode() : 0);
result = 31 * result + (this.dockerImage != null ? this.dockerImage.hashCode() : 0);
result = 31 * result + (this.dockerArgs != null ? this.dockerArgs.hashCode() : 0);
result = 31 * result + (this.dockerTerminationLogging ? 1231 : 1237);
result = 31 * result + (this.serviceAccount != null ? this.serviceAccount.hashCode() : 0);
result = 31 * result + (this.resources != null ? this.resources.hashCode() : 0);
result = 31 * result + (this.env != null ? this.env.hashCode() : 0);
result = 31 * result + (this.runningTimeout != null ? this.runningTimeout.hashCode() : 0);
result = 31 * result + (this.retryCondition != null ? this.retryCondition.hashCode() : 0);
result = 31 * result + (this.flyteExecConf != null ? this.flyteExecConf.hashCode() : 0);
result = 31 * result + (this.deploymentSource != null ? this.deploymentSource.hashCode() : 0);
result = 31 * result + (this.deploymentTime != null ? this.deploymentTime.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "WorkflowConfiguration{" +
"id=" + id +
", schedule=" + schedule +
", offset=" + offset +
", commitSha=" + commitSha +
", dockerImage=" + dockerImage +
", dockerArgs=" + dockerArgs +
", dockerTerminationLogging=" + dockerTerminationLogging +
", serviceAccount=" + serviceAccount +
", resources=" + resources +
", env=" + env +
", runningTimeout=" + runningTimeout +
", retryCondition=" + retryCondition +
", flyteExecConf=" + flyteExecConf +
", deploymentSource=" + deploymentSource +
", deploymentTime=" + deploymentTime +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy