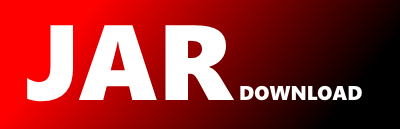
com.spotify.styx.model.SecretBuilder Maven / Gradle / Ivy
package com.spotify.styx.model;
import io.norberg.automatter.AutoMatter;
import javax.annotation.processing.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class SecretBuilder {
private String name;
private String mountPath;
public SecretBuilder() {
}
private SecretBuilder(WorkflowConfiguration.Secret v) {
this.name = v.name();
this.mountPath = v.mountPath();
}
private SecretBuilder(SecretBuilder v) {
this.name = v.name;
this.mountPath = v.mountPath;
}
public String name() {
return name;
}
public SecretBuilder name(String name) {
if (name == null) {
throw new NullPointerException("name");
}
this.name = name;
return this;
}
public String mountPath() {
return mountPath;
}
public SecretBuilder mountPath(String mountPath) {
if (mountPath == null) {
throw new NullPointerException("mountPath");
}
this.mountPath = mountPath;
return this;
}
public WorkflowConfiguration.Secret build() {
return new Value(name, mountPath);
}
public static SecretBuilder from(WorkflowConfiguration.Secret v) {
return new SecretBuilder(v);
}
public static SecretBuilder from(SecretBuilder v) {
return new SecretBuilder(v);
}
private static final class Value implements WorkflowConfiguration.Secret {
private final String name;
private final String mountPath;
private Value(@AutoMatter.Field("name") String name,
@AutoMatter.Field("mountPath") String mountPath) {
if (name == null) {
throw new NullPointerException("name");
}
if (mountPath == null) {
throw new NullPointerException("mountPath");
}
this.name = name;
this.mountPath = mountPath;
}
@AutoMatter.Field
@Override
public String name() {
return name;
}
@AutoMatter.Field
@Override
public String mountPath() {
return mountPath;
}
public SecretBuilder builder() {
return new SecretBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof WorkflowConfiguration.Secret)) {
return false;
}
final WorkflowConfiguration.Secret that = (WorkflowConfiguration.Secret) o;
if (name != null ? !name.equals(that.name()) : that.name() != null) {
return false;
}
if (mountPath != null ? !mountPath.equals(that.mountPath()) : that.mountPath() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
result = 31 * result + (this.mountPath != null ? this.mountPath.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "WorkflowConfiguration.Secret{" +
"name=" + name +
", mountPath=" + mountPath +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy