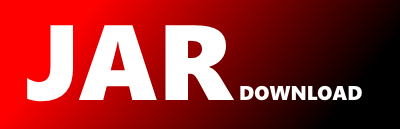
com.spotinst.sdkjava.model.ApiLaunchSpec Maven / Gradle / Ivy
package com.spotinst.sdkjava.model;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.spotinst.sdkjava.client.rest.IPartialUpdateEntity;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* Created by aharontwizer on 8/26/15.
*/
@JsonIgnoreProperties(ignoreUnknown = true)
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonFilter("PartialUpdateEntityFilter")
class ApiLaunchSpec implements IPartialUpdateEntity {
//region Members
@JsonIgnore
private Set isSet;
private String healthCheckType;
private Integer healthCheckGracePeriod;
private List securityGroupIds;
private Boolean monitoring;
private Boolean ebsOptimized;
private String imageId;
private ApiIamRole iamRole;
private String keyPair;
private Integer healthCheckUnhealthyDurationBeforeReplacement;
private String userData;
private List blockDeviceMappings;
private List networkInterfaces;
private List tags;
private ApiGroupResourceTagSpecification resourceTagSpecification;
private ApiLoadBalancersConfig loadBalancersConfig;
private ApiItf itf;
//endregion
//region Constructor
public ApiLaunchSpec() {
isSet = new HashSet<>();
}
//endregion
//region Getters & Setters
public Set getIsSet() {
return isSet;
}
public void setIsSet(Set isSet) {
this.isSet = isSet;
}
public ApiGroupResourceTagSpecification getResourceTagSpecification() {
return resourceTagSpecification;
}
public void setResourceTagSpecification(ApiGroupResourceTagSpecification resourceTagSpecification) {
isSet.add("resourceTagSpecification");
this.resourceTagSpecification = resourceTagSpecification;
}
public String getHealthCheckType() {
return healthCheckType;
}
public void setHealthCheckType(String healthCheckType) {
isSet.add("healthCheckType");
this.healthCheckType = healthCheckType;
}
public Integer getHealthCheckGracePeriod() {
return healthCheckGracePeriod;
}
public void setHealthCheckGracePeriod(Integer healthCheckGracePeriod) {
isSet.add("healthCheckGracePeriod");
this.healthCheckGracePeriod = healthCheckGracePeriod;
}
public List getSecurityGroupIds() {
return securityGroupIds;
}
public void setSecurityGroupIds(List securityGroupIds) {
isSet.add("securityGroupIds");
this.securityGroupIds = securityGroupIds;
}
public Boolean getMonitoring() {
return monitoring;
}
public void setMonitoring(Boolean monitoring) {
isSet.add("monitoring");
this.monitoring = monitoring;
}
public Boolean getEbsOptimized() {
return ebsOptimized;
}
public void setEbsOptimized(Boolean ebsOptimized) {
isSet.add("ebsOptimized");
this.ebsOptimized = ebsOptimized;
}
public String getImageId() {
return imageId;
}
public void setImageId(String imageId) {
isSet.add("imageId");
this.imageId = imageId;
}
public ApiIamRole getIamRole() {
return iamRole;
}
public void setIamRole(ApiIamRole iamRole) {
isSet.add("iamRole");
this.iamRole = iamRole;
}
public String getKeyPair() {
return keyPair;
}
public void setKeyPair(String keyPair) {
isSet.add("keyPair");
this.keyPair = keyPair;
}
public String getUserData() {
return userData;
}
public void setUserData(String userData) {
isSet.add("userData");
this.userData = userData;
}
public Integer getHealthCheckUnhealthyDurationBeforeReplacement() {
return healthCheckUnhealthyDurationBeforeReplacement;
}
public void setHealthCheckUnhealthyDurationBeforeReplacement(Integer healthCheckUnhealthyDurationBeforeReplacement) {
isSet.add("healthCheckUnhealthyDurationBeforeReplacement");
this.healthCheckUnhealthyDurationBeforeReplacement = healthCheckUnhealthyDurationBeforeReplacement;
}
public List getBlockDeviceMappings() {
return blockDeviceMappings;
}
public void setBlockDeviceMappings(List blockDeviceMappings) {
isSet.add("blockDeviceMappings");
this.blockDeviceMappings = blockDeviceMappings;
}
public List getNetworkInterfaces() {
return networkInterfaces;
}
public void setNetworkInterfaces(List networkInterfaces) {
isSet.add("networkInterfaces");
this.networkInterfaces = networkInterfaces;
}
public List getTags() {
return tags;
}
public void setTags(List tags) {
isSet.add("tags");
this.tags = tags;
}
public ApiLoadBalancersConfig getLoadBalancersConfig() {
return loadBalancersConfig;
}
public void setLoadBalancersConfig(ApiLoadBalancersConfig loadBalancersConfig) {
isSet.add("loadBalancersConfig");
this.loadBalancersConfig = loadBalancersConfig;
}
public ApiItf getItf() {
return itf;
}
public void setItf(ApiItf itf) {
isSet.add("itf");
this.itf = itf;
}
//endregion
//region isSet methods
// Is healthCheckType Set boolean method
@JsonIgnore
public boolean isHealthCheckTypeSet() {
return isSet.contains("healthCheckType");
}
// Is resourceTagSpecification Set boolean method
@JsonIgnore
public boolean isResourceTagSpecificationSet() {
return isSet.contains("resourceTagSpecification");
}
// Is healthCheckGracePeriod Set boolean method
@JsonIgnore
public boolean isHealthCheckGracePeriodSet() {
return isSet.contains("healthCheckGracePeriod");
}
// Is securityGroupIds Set boolean method
@JsonIgnore
public boolean isSecurityGroupIdsSet() {
return isSet.contains("securityGroupIds");
}
// Is monitoring Set boolean method
@JsonIgnore
public boolean isMonitoringSet() {
return isSet.contains("monitoring");
}
// Is ebsOptimized Set boolean method
@JsonIgnore
public boolean isEbsOptimizedSet() {
return isSet.contains("ebsOptimized");
}
// Is imageId Set boolean method
@JsonIgnore
public boolean isImageIdSet() {
return isSet.contains("imageId");
}
// Is iamRole Set boolean method
@JsonIgnore
public boolean isIamRoleSet() {
return isSet.contains("iamRole");
}
// Is keyPair Set boolean method
@JsonIgnore
public boolean isKeyPairSet() {
return isSet.contains("keyPair");
}
// Is userData Set boolean method
@JsonIgnore
public boolean isUserDataSet() {
return isSet.contains("userData");
}
// Is blockDeviceMappings Set boolean method
@JsonIgnore
public boolean isBlockDeviceMappingsSet() {
return isSet.contains("blockDeviceMappings");
}
// Is networkInterfaces Set boolean method
@JsonIgnore
public boolean isNetworkInterfacesSet() {
return isSet.contains("networkInterfaces");
}
// Is tags Set boolean method
@JsonIgnore
public boolean isTagsSet() {
return isSet.contains("tags");
}
// Is loadBalancersConfig Set boolean method
@JsonIgnore
public boolean isLoadBalancersConfigSet() {
return isSet.contains("loadBalancersConfig");
}
// Is healthCheckUnhealthyDurationBeforeReplacement Set boolean method
@JsonIgnore
public boolean isHealthCheckUnhealthyDurationBeforeReplacementSet() {
return isSet.contains("healthCheckUnhealthyDurationBeforeReplacement");
}
// Is itf Set boolean method
@JsonIgnore
public boolean isItfSet() { return isSet.contains("itf"); }
//endregion
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy