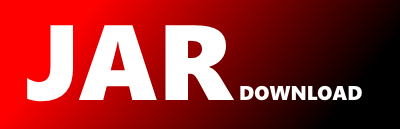
com.spotinst.sdkjava.model.SpotinstMrScalerAwsRepo Maven / Gradle / Ivy
package com.spotinst.sdkjava.model;
import com.spotinst.sdkjava.exception.ExceptionHelper;
import com.spotinst.sdkjava.exception.SpotinstHttpException;
import com.spotinst.sdkjava.model.api.mrScaler.aws.ApiMrScalerAws;
import com.spotinst.sdkjava.model.bl.mrScaler.aws.BlMrScalerAws;
import java.util.ArrayList;
import java.util.List;
public class SpotinstMrScalerAwsRepo implements ISpotinstMrScalerAwsRepo {
@Override
public RepoGenericResponse create(ApiMrScalerAws mrScalerToCreate, String authToken, String account) {
RepoGenericResponse retVal;
try {
BlMrScalerAws blMrScalerToCreate = MrScalerAwsConverter.toBl(mrScalerToCreate);
BlMrScalerAws blCreatedMrScaler = SpotinstMrScalerAwsService.createMrScaler(blMrScalerToCreate, authToken, account);
ApiMrScalerAws createdMrScaler = MrScalerAwsConverter.toApi(blCreatedMrScaler);
retVal = new RepoGenericResponse<>(createdMrScaler);
} catch (SpotinstHttpException ex) {
retVal = ExceptionHelper.handleHttpException(ex);
}
return retVal;
}
@Override
public RepoGenericResponse update(String clusterId, ApiMrScalerAws mrScalerToUpdate, String authToken, String account) {
RepoGenericResponse retVal;
try{
BlMrScalerAws blMrScalerToUpdate = MrScalerAwsConverter.toBl(mrScalerToUpdate);
Boolean success = SpotinstMrScalerAwsService.updateMrScaler(clusterId, blMrScalerToUpdate, authToken, account);
retVal = new RepoGenericResponse<>(success);
} catch (SpotinstHttpException ex) {
retVal = ExceptionHelper.handleHttpException(ex);
}
return retVal;
}
@Override
public RepoGenericResponse delete(String identifier, String authToken, String account) {
RepoGenericResponse retVal;
try {
Boolean updated = SpotinstMrScalerAwsService.deleteMrScaler(identifier, authToken, account);
retVal = new RepoGenericResponse<>(updated);
} catch (SpotinstHttpException e) {
retVal = ExceptionHelper.handleHttpException(e);
}
return retVal;
}
@Override
public RepoGenericResponse get(String mrScalerId, String authToken,String account) {
RepoGenericResponse retVal;
try {
BlMrScalerAws blMrScaler = SpotinstMrScalerAwsService.getMrScaler(mrScalerId, authToken, account);
ApiMrScalerAws apiMrScalerAws = MrScalerAwsConverter.toApi(blMrScaler);
retVal = new RepoGenericResponse<>(apiMrScalerAws);
} catch (SpotinstHttpException e) {
retVal = ExceptionHelper.handleHttpException(e);
}
return retVal;
}
@Override
public RepoGenericResponse> getAll(Void filter, String authToken,String account){
RepoGenericResponse> retVal;
try{
List blMrScalerList = SpotinstMrScalerAwsService.getAllMrScaler(authToken, account);
List convertedList = new ArrayList<>();
for(BlMrScalerAws singleMrScaler: blMrScalerList){
ApiMrScalerAws temp = MrScalerAwsConverter.toApi(singleMrScaler);
convertedList.add(temp);
}
retVal = new RepoGenericResponse<>(convertedList);
} catch (SpotinstHttpException e) {
retVal = ExceptionHelper.handleHttpException(e);
}
return retVal;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy