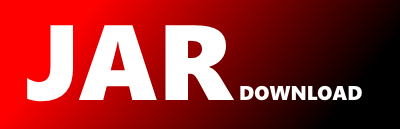
com.sqlapp.gradle.plugins.pojo.DataSourcePojo.groovy Maven / Gradle / Ivy
/*
* Copyright (C) 2007-2017 Tatsuo Satoh
*
* This file is part of sqlapp-gradle-plugin.
*
* sqlapp-gradle-plugin is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* sqlapp-gradle-plugin is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with sqlapp-gradle-plugin. If not, see .
*/
package com.sqlapp.gradle.plugins.pojo
import com.sqlapp.util.CommonUtils
import java.io.File
import java.util.List;
import org.gradle.api.DefaultTask
import org.gradle.api.Plugin
import org.gradle.api.Project
import org.gradle.api.tasks.Input
import org.gradle.api.tasks.Optional;
import org.gradle.api.tasks.TaskAction;
class DataSourcePojo implements Cloneable{
Project project;
public DataSourcePojo(Project project) {
this.project=project;
}
/**
* JDBC Driver Class Name
*/
@Input
@Optional
String driverClassName;
/**
* JDBC URL
*/
@Input
@Optional
String url;
/**
* JDBC User Name
*/
@Input
@Optional
String username;
/**
* JDBC Password
*/
@Input
@Optional
String password;
/**
* プールの起動時に作成されるコネクションの初期サイズ
*/
@Input
@Optional
int initialSize = 0;
/**
* 最大接続数
*/
@Input
@Optional
int maxActive = 8;
/**
* プール内のコネクションが不足したときの最大待ち時間
*/
@Input
@Optional
int maxWait = -1;
/**
* プールに保持しておく最大のコネクション数
*/
@Input
@Optional
int maxIdle = 0;
/**
* プールに保持する最小のコネクション数
*/
@Input
@Optional
int minIdle = 0;
/**
* コネクションが有効かどうかを検証するためのSQL
*/
@Input
@Optional
volatile String validationQuery;
/**
* コネクションをプールから取り出すときに検証するかどうか
*/
@Input
@Optional
boolean testOnBorrow;
/**
* コネクションをプールに返すときに検証するかどうか
*/
@Input
@Optional
boolean testOnReturn;
/**
* クローズ漏れとなったコネクションを回収するかどうか
*/
@Input
@Optional
boolean removeAbandoned = false;
/**
* コネクションが最後に使用されてから回収対象となるまでの時間(秒)
*/
@Input
@Optional
int removeAbandonedTimeout = 60 * 10;
/**
* デフォルトトランザクション分離レベル
*/
@Input
@Optional
int defaultTransactionIsolation = -1;
/**
* デフォルトオートコミット
*/
@Input
@Optional
boolean defaultAutoCommit = false;
/**
* プール内のアイドル接続を一定時間毎に監視するスレッドを開始させます。間隔をミリ秒単位で指定します。
*/
@Input
@Optional
int timeBetweenEvictionRunsMillis = -1;
/**
* 監視処理時、アイドル接続の有効性を確認します。
*/
@Input
@Optional
boolean testWhileIdle = false;
/**
* 監視処理時、アイドル接続の生存期間をチェックします。
*/
@Input
@Optional
int minEvictableIdleTimeMillis = 1000 * 60 * 30;
/**
* 1回の監視処理でチェックするアイドル接続数の最大値を指定します。
*/
@Input
@Optional
int numTestsPerEvictionRun = 3;
/**
* JDBCインターセプタ
*/
@Input
@Optional
String jdbcInterceptors = null;
void driverClassName(String driverClassName){
this.driverClassName=driverClassName;
}
void url(String url){
this.url=url;
}
void username(String username){
this.username=username;
}
void password(String password){
this.password=password;
}
void defaultTransactionIsolation(String value){
if ("NONE".equalsIgnoreCase(value)||"TRANSACTION_NONE".equalsIgnoreCase(value)){
this.defaultTransactionIsolation=java.sql.Connection.TRANSACTION_NONE;
}else if ("READ_COMMITTED".equalsIgnoreCase(value)||"TRANSACTION_READ_COMMITTED".equalsIgnoreCase(value)){
this.defaultTransactionIsolation=java.sql.Connection.TRANSACTION_READ_COMMITTED;
}else if ("READ_UNCOMMITTED".equalsIgnoreCase(value)||"TRANSACTION_READ_UNCOMMITTED".equalsIgnoreCase(value)){
this.defaultTransactionIsolation=java.sql.Connection.TRANSACTION_READ_UNCOMMITTED;
}else if ("REPEATABLE_READ".equalsIgnoreCase(value)||"TRANSACTION_REPEATABLE_READ".equalsIgnoreCase(value)){
this.defaultTransactionIsolation=java.sql.Connection.TRANSACTION_REPEATABLE_READ;
}else if ("SERIALIZABLE".equalsIgnoreCase(value)||"TRANSACTION_SERIALIZABLE".equalsIgnoreCase(value)){
this.defaultTransactionIsolation=java.sql.Connection.TRANSACTION_SERIALIZABLE;
}
}
void setDefaultTransactionIsolation(String value){
this.defaultTransactionIsolation(value);
}
@Override
DataSourcePojo clone(){
return super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy