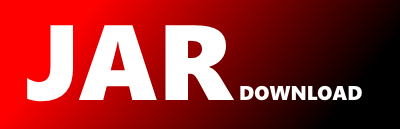
com.squarespace.cldrengine.api.UnitFormatOptions Maven / Gradle / Ivy
The newest version!
package com.squarespace.cldrengine.api;
import lombok.Generated;
import lombok.EqualsAndHashCode;
@Generated
@EqualsAndHashCode(callSuper = true)
public class UnitFormatOptions extends NumberFormatOptions {
public final Option divisor = Option.option();
public final Option style = Option.option();
public final Option length = Option.option();
public UnitFormatOptions() {
}
public UnitFormatOptions(UnitFormatOptions arg) {
super(arg);
this.divisor.set(arg.divisor);
this.style.set(arg.style);
this.length.set(arg.length);
}
public UnitFormatOptions divisor(Integer arg) {
this.divisor.set(arg);
return this;
}
public UnitFormatOptions divisor(Option arg) {
this.divisor.set(arg);
return this;
}
public UnitFormatOptions style(UnitFormatStyleType arg) {
this.style.set(arg);
return this;
}
public UnitFormatOptions style(Option arg) {
this.style.set(arg);
return this;
}
public UnitFormatOptions length(UnitLength arg) {
this.length.set(arg);
return this;
}
public UnitFormatOptions length(Option arg) {
this.length.set(arg);
return this;
}
public static UnitFormatOptions fromSuper(NumberFormatOptions arg) {
UnitFormatOptions o = UnitFormatOptions.build();
o.group.setIf(arg.group);
o.numberSystem.setIf(arg.numberSystem);
o.trimZeroFractions.setIf(arg.trimZeroFractions);
return o;
}
public UnitFormatOptions group(Boolean arg) {
this.group.set(arg);
return this;
}
public UnitFormatOptions group(Option arg) {
this.group.set(arg);
return this;
}
public UnitFormatOptions numberSystem(String arg) {
this.numberSystem.set(arg);
return this;
}
public UnitFormatOptions numberSystem(Option arg) {
this.numberSystem.set(arg);
return this;
}
public UnitFormatOptions trimZeroFractions(Boolean arg) {
this.trimZeroFractions.set(arg);
return this;
}
public UnitFormatOptions trimZeroFractions(Option arg) {
this.trimZeroFractions.set(arg);
return this;
}
public UnitFormatOptions round(RoundingModeType arg) {
this.round.set(arg);
return this;
}
public UnitFormatOptions round(Option arg) {
this.round.set(arg);
return this;
}
public UnitFormatOptions minimumIntegerDigits(Integer arg) {
this.minimumIntegerDigits.set(arg);
return this;
}
public UnitFormatOptions minimumIntegerDigits(Option arg) {
this.minimumIntegerDigits.set(arg);
return this;
}
public UnitFormatOptions maximumFractionDigits(Integer arg) {
this.maximumFractionDigits.set(arg);
return this;
}
public UnitFormatOptions maximumFractionDigits(Option arg) {
this.maximumFractionDigits.set(arg);
return this;
}
public UnitFormatOptions minimumFractionDigits(Integer arg) {
this.minimumFractionDigits.set(arg);
return this;
}
public UnitFormatOptions minimumFractionDigits(Option arg) {
this.minimumFractionDigits.set(arg);
return this;
}
public UnitFormatOptions maximumSignificantDigits(Integer arg) {
this.maximumSignificantDigits.set(arg);
return this;
}
public UnitFormatOptions maximumSignificantDigits(Option arg) {
this.maximumSignificantDigits.set(arg);
return this;
}
public UnitFormatOptions minimumSignificantDigits(Integer arg) {
this.minimumSignificantDigits.set(arg);
return this;
}
public UnitFormatOptions minimumSignificantDigits(Option arg) {
this.minimumSignificantDigits.set(arg);
return this;
}
public static UnitFormatOptions build() {
return new UnitFormatOptions();
}
public UnitFormatOptions copy() {
return new UnitFormatOptions(this);
}
public UnitFormatOptions mergeIf(UnitFormatOptions ...args) {
UnitFormatOptions o = new UnitFormatOptions(this);
for (UnitFormatOptions arg : args) {
o._mergeIf(arg);
}
return o;
}
protected void _mergeIf(UnitFormatOptions o) {
super._mergeIf(o);
this.divisor.setIf(o.divisor);
this.style.setIf(o.style);
this.length.setIf(o.length);
}
public UnitFormatOptions merge(UnitFormatOptions ...args) {
UnitFormatOptions o = new UnitFormatOptions(this);
for (UnitFormatOptions arg : args) {
o._merge(arg);
}
return o;
}
protected void _merge(UnitFormatOptions o) {
super._merge(o);
this.divisor.set(o.divisor);
this.style.set(o.style);
this.length.set(o.length);
}
@Override
public String toString() {
StringBuilder buf = new StringBuilder("UnitFormatOptions( ");
this._tostring(buf);
return buf.append(')').toString();
}
protected void _tostring(StringBuilder buf) {
super._tostring(buf);
if (divisor.ok()) {
buf.append("divisor=").append(divisor).append(' ');
}
if (style.ok()) {
buf.append("style=").append(style).append(' ');
}
if (length.ok()) {
buf.append("length=").append(length).append(' ');
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy