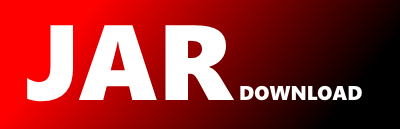
com.squarespace.template.CodeStats Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of template-core Show documentation
Show all versions of template-core Show documentation
Squarespace template compiler
/**
* Copyright (c) 2014 SQUARESPACE, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.squarespace.template;
import java.util.HashMap;
import java.util.Map;
import com.squarespace.template.Instructions.IfPredicateInst;
import com.squarespace.template.Instructions.PredicateInst;
import com.squarespace.template.Instructions.VariableInst;
/**
* Examines sequences of instructions and gathers some statistics.
*/
public class CodeStats implements CodeSink {
private Counter instructionCounter;
private Counter formatterCounter;
private Counter predicateCounter;
private int totalInstructions;
public CodeStats() {
this.instructionCounter = new Counter<>();
this.formatterCounter = new Counter<>();
this.predicateCounter = new Counter<>();
}
@Override
public void accept(Instruction instruction) throws CodeSyntaxException {
totalInstructions++;
InstructionType type = instruction.getType();
instructionCounter.increment(type);
switch (type) {
case PREDICATE:
case OR_PREDICATE:
Predicate predicate = ((PredicateInst)instruction).getPredicate();
if (predicate != null) {
predicateCounter.increment(predicate.identifier());
}
break;
case IF:
if (instruction instanceof IfPredicateInst) {
predicateCounter.increment(((IfPredicateInst)instruction).getPredicate().identifier());
}
break;
case VARIABLE:
for (FormatterCall formatter : ((VariableInst)instruction).getFormatters()) {
formatterCounter.increment(formatter.getFormatter().identifier());
}
break;
default:
break;
}
}
@Override
public void complete() {
}
public int getTotalInstructions() {
return totalInstructions;
}
public Map getInstructionCounts() {
return instructionCounter.getMap();
}
public Map getFormatterCounts() {
return formatterCounter.getMap();
}
public Map getPredicateCounts() {
return predicateCounter.getMap();
}
private static class Counter {
private Map map = new HashMap<>();
public void increment(K key) {
Integer val = map.get(key);
if (val == null) {
val = 0;
}
map.put(key, val + 1);
}
public Map getMap() {
return map;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy