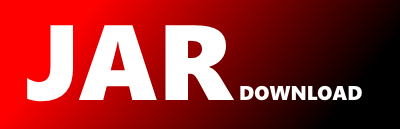
com.squarespace.template.ReprEmitter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of template-core Show documentation
Show all versions of template-core Show documentation
Squarespace template compiler
/**
* Copyright (c) 2014 SQUARESPACE, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.squarespace.template;
import java.util.List;
import com.squarespace.template.Instructions.AlternatesWithInst;
import com.squarespace.template.Instructions.BindVarInst;
import com.squarespace.template.Instructions.CommentInst;
import com.squarespace.template.Instructions.CtxVarInst;
import com.squarespace.template.Instructions.EndInst;
import com.squarespace.template.Instructions.EvalInst;
import com.squarespace.template.Instructions.IfInst;
import com.squarespace.template.Instructions.IfPredicateInst;
import com.squarespace.template.Instructions.IncludeInst;
import com.squarespace.template.Instructions.InjectInst;
import com.squarespace.template.Instructions.LiteralInst;
import com.squarespace.template.Instructions.MacroInst;
import com.squarespace.template.Instructions.MetaInst;
import com.squarespace.template.Instructions.PredicateInst;
import com.squarespace.template.Instructions.RepeatedInst;
import com.squarespace.template.Instructions.RootInst;
import com.squarespace.template.Instructions.SectionInst;
import com.squarespace.template.Instructions.TextInst;
import com.squarespace.template.Instructions.VariableInst;
/**
* Given an instruction, (recursively) emits the canonical representation.
*/
public class ReprEmitter {
private ReprEmitter() {
}
public static String get(Instruction inst, boolean recurse) {
StringBuilder buf = new StringBuilder();
inst.repr(buf, recurse);
return buf.toString();
}
public static String get(Arguments args, boolean includeDelimiter) {
StringBuilder buf = new StringBuilder();
emit(args, includeDelimiter, buf);
return buf.toString();
}
public static String get(Variables variables) {
StringBuilder buf = new StringBuilder();
emitVariables(variables, buf);
return buf.toString();
}
public static String get(Object[] names) {
StringBuilder buf = new StringBuilder();
emitNames(names, buf);
return buf.toString();
}
private static void emitPreprocess(Instruction inst, StringBuilder buf) {
if (inst.inPreprocessScope()) {
buf.append('^');
}
}
public static void emit(AlternatesWithInst inst, StringBuilder buf, boolean recurse) {
buf.append('{');
emitPreprocess(inst, buf);
buf.append(".alternates with}");
if (recurse) {
emitBlock(inst, buf, recurse);
}
}
public static void emit(CommentInst inst, StringBuilder buf) {
boolean preproc = inst.inPreprocessScope() && !inst.isMultiLine();
buf.append('{');
if (preproc) {
buf.append('^');
}
buf.append('#');
if (inst.isMultiLine()) {
buf.append('#');
}
StringView view = inst.getView();
buf.append(view.data(), view.start(), view.end());
if (inst.isMultiLine()) {
buf.append("##");
}
buf.append('}');
}
public static void emit(EndInst inst, StringBuilder buf) {
buf.append('{');
emitPreprocess(inst, buf);
buf.append(".end}");
}
public static void emit(EvalInst inst, StringBuilder buf) {
buf.append('{');
emitPreprocess(inst, buf);
buf.append(".eval ");
buf.append(inst.body());
buf.append('}');
}
public static void emit(Arguments args, StringBuilder buf) {
emit(args, true, buf);
}
public static void emit(Arguments args, boolean includeDelimiter, StringBuilder buf) {
if (args.isEmpty()) {
return;
}
char delimiter = args.getDelimiter();
List argList = args.getArgs();
for (int i = 0, size = argList.size(); i < size; i++) {
// In some cases we want to get the arguments' representation without the prefixed
// delimiter. When rendering the template representation we want the prefix.
if (includeDelimiter || i > 0) {
buf.append(delimiter);
}
buf.append(argList.get(i));
}
}
public static void emit(IfInst inst, StringBuilder buf, boolean recurse) {
buf.append('{');
emitPreprocess(inst, buf);
buf.append(".if ");
emitIfExpression(inst, buf);
buf.append('}');
if (recurse) {
emitBlock(inst, buf, recurse);
}
}
public static void emitIfExpression(IfInst inst, StringBuilder buf) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy