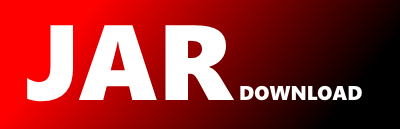
com.squareup.sqldelight.Query.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of runtime-common Show documentation
Show all versions of runtime-common Show documentation
Common runtime library to support generated code
The newest version!
/*
* Copyright (C) 2018 Square, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.squareup.sqldelight
import com.squareup.sqldelight.db.SqlPreparedStatement
import com.squareup.sqldelight.db.SqlResultSet
import com.squareup.sqldelight.db.use
import com.squareup.sqldelight.internal.QueryList
/**
* A listenable, typed query generated by SQLDelight.
*
* @param RowType the type that this query can map it's result set to.
*/
open class Query(
private val statement: SqlPreparedStatement,
private val queries: QueryList,
private val mapper: (SqlResultSet) -> RowType
) {
private val listeners = mutableSetOf()
/**
* Notify listeners that their current result set is staled.
*
* Called internally by SQLDelight when it detects a possible staling of the result set. Emits
* some false positives but never misses a true positive.
*/
fun notifyResultSetChanged() {
synchronized(listeners) {
listeners.forEach(Listener::queryResultsChanged)
}
}
/**
* Register a listener to be notified of future changes in the result set.
*/
fun addListener(listener: Listener) {
synchronized(listeners) {
if (listeners.isEmpty()) queries.addQuery(this)
listeners.add(listener)
}
}
fun removeListener(listener: Listener) {
synchronized(listeners) {
listeners.remove(listener)
if (listeners.isEmpty()) queries.removeQuery(this)
}
}
/**
* Execute [statement] as a query.
*/
fun execute() = statement.executeQuery()
/**
* Execute [statement] and return the result set as a list of [RowType].
*/
fun executeAsList(): List {
val result = mutableListOf()
execute().use {
while (it.next()) result.add(mapper(it))
}
return result
}
/**
* Execute [statement] and return the only row of the result set as a non null [RowType].
*
* @throws NullPointerException if when executed this query has no rows in its result set.
* @throws IllegalStateException if when executed this query has multiple rows in its result set.
*/
fun executeAsOne(): RowType {
return executeAsOneOrNull()
?: throw NullPointerException("ResultSet returned null for $statement")
}
/**
* Execute [statement] and return the first row of the result set as a non null [RowType] or null
* if the result set has no rows.
*
* @throws IllegalStateException if when executed this query has multiple rows in its result set.
*/
fun executeAsOneOrNull(): RowType? {
execute().use {
if (!it.next()) return null
val item = mapper(it)
if (it.next()) {
throw IllegalStateException("ResultSet returned more than 1 row for $statement")
}
return item
}
}
interface Listener {
fun queryResultsChanged()
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy