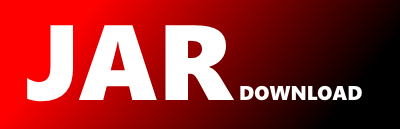
commonMain.com.squareup.wire.internal.Internal.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wire-runtime-metadata Show documentation
Show all versions of wire-runtime-metadata Show documentation
Multiplatform runtime library to support generated code
The newest version!
/*
* Copyright 2019 Square Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
@file:JvmName("Internal")
@file:JvmMultifileClass
package com.squareup.wire.internal
import com.squareup.wire.ProtoAdapter
import kotlin.jvm.JvmMultifileClass
import kotlin.jvm.JvmName
// Methods for generated code use only. Not subject to public API rules.
fun newMutableList(): MutableList = MutableOnWriteList(emptyList())
fun newMutableMap(): MutableMap = LinkedHashMap()
@Deprecated(
message = "Please regenerate code using wire-compiler version 3.0.0 or higher.",
replaceWith = ReplaceWith("com.squareup.internal.Internal.copyOf(list)")
)
fun copyOf(name: String, list: List?): MutableList = copyOf(list!!)
fun copyOf(list: List): MutableList {
return if (list === emptyList() || list is ImmutableList<*>) {
MutableOnWriteList(list)
} else {
ArrayList(list)
}
}
@Deprecated(
message = "Please regenerate code using wire-compiler version 3.0.0 or higher.",
replaceWith = ReplaceWith("com.squareup.internal.Internal.copyOf(map)")
)
fun copyOf(name: String, map: Map?): MutableMap = copyOf(map!!)
fun copyOf(map: Map): MutableMap = LinkedHashMap(map)
fun immutableCopyOf(name: String, list: List): List {
var list = list
if (list is MutableOnWriteList<*>) {
list = (list as MutableOnWriteList).mutableList
}
if (list === emptyList() || list is ImmutableList<*>) {
return list
}
val result = ImmutableList(list)
// Check after the list has been copied to defend against races.
require(null !in result) { "$name.contains(null)" }
return result as List
}
fun immutableCopyOf(name: String, map: Map): Map {
if (map.isEmpty()) {
return emptyMap()
}
val result = LinkedHashMap(map)
// Check after the map has been copied to defend against races.
require(null !in (result.keys as Collection)) { "$name.containsKey(null)" }
require(null !in (result.values as Collection)) { "$name.containsValue(null)" }
return result.toUnmodifiableMap()
}
/** Confirms the values of [map] are structs and returns an immutable copy. */
fun immutableCopyOfMapWithStructValues(name: String, map: Map): Map {
val copy = mutableMapOf()
for ((k, v) in map) {
require(k != null) { "$name.containsKey(null)" }
copy[k] = immutableCopyOfStruct(name, v)
}
return copy.toUnmodifiableMap() as Map
}
/** Confirms [value] is a struct and returns an immutable copy. */
fun immutableCopyOfStruct(name: String, value: T): T {
return when (value) {
null -> value
is Boolean -> value
is Double -> value
is String -> value
is List<*> -> {
val copy = mutableListOf()
for (element in value) {
copy += immutableCopyOfStruct(name, element)
}
copy.toUnmodifiableList() as T
}
is Map<*, *> -> {
val copy = mutableMapOf()
for ((k, v) in value) {
copy[immutableCopyOfStruct(name, k)] = immutableCopyOfStruct(name, v)
}
copy.toUnmodifiableMap() as T
}
else -> {
throw IllegalArgumentException("struct value $name must be a JSON type " +
"(null, Boolean, Double, String, List, or Map) but was ${value.typeName}: $value")
}
}
}
private val Any.typeName
get() = this::class
@JvmName("-redactElements") // Hide from Java
fun List.redactElements(adapter: ProtoAdapter): List = map(adapter::redact)
@JvmName("-redactElements") // Hide from Java
fun Map.redactElements(adapter: ProtoAdapter): Map {
return mapValues { (_, value) -> adapter.redact(value) }
}
fun equals(a: Any?, b: Any?): Boolean = a === b || (a != null && a == b)
/**
* Create an exception for missing required fields.
*
* @param args Alternating field value and field name pairs.
*/
fun missingRequiredFields(vararg args: Any?): IllegalStateException {
var plural = ""
val fields = buildString {
for (i in 0 until args.size step 2) {
if (args[i] == null) {
if (isNotEmpty()) {
plural = "s" // Found more than one missing field
}
append("\n ")
append(args[i + 1])
}
}
}
throw IllegalStateException("Required field$plural not set:$fields")
}
/** Throw [NullPointerException] if any of `list`'s items is null. */
fun checkElementsNotNull(list: List<*>) {
for (i in 0 until list.size) {
if (list[i] == null) {
throw NullPointerException("Element at index $i is null")
}
}
}
/** Throw [NullPointerException] if any of`map`'s keys or values is null. */
fun checkElementsNotNull(map: Map<*, *>) {
for ((key, value) in map) {
if (key == null) {
throw NullPointerException("map.containsKey(null)")
}
if (value == null) {
throw NullPointerException("Value for key $key is null")
}
}
}
/** Returns the number of non-null values in `a, b`. */
fun countNonNull(a: Any?, b: Any?): Int = (if (a != null) 1 else 0) + (if (b != null) 1 else 0)
/** Returns the number of non-null values in `a, b, c`. */
fun countNonNull(a: Any?, b: Any?, c: Any?): Int {
return (if (a != null) 1 else 0) + (if (b != null) 1 else 0) + (if (c != null) 1 else 0)
}
/** Returns the number of non-null values in `a, b, c, d, rest`. */
fun countNonNull(a: Any?, b: Any?, c: Any?, d: Any?, vararg rest: Any?): Int {
var result = 0
if (a != null) result++
if (b != null) result++
if (c != null) result++
if (d != null) result++
for (o in rest) {
if (o != null) result++
}
return result
}
private const val ESCAPED_CHARS = ",[]{}\\"
/** Return a string where `,[]{}\` are escaped with a `\`. */
fun sanitize(value: String): String {
return buildString(value.length) {
value.forEach { char ->
if (char in ESCAPED_CHARS) append('\\')
append(char)
}
}
}
/** Return a string where `,[]{}\` are escaped with a `\`. */
fun sanitize(values: List): String {
return values.joinToString(prefix = "[", postfix = "]", transform = ::sanitize)
}
fun boxedOneOfClassName(oneOfName: String): String {
return oneOfName.capitalize()
}
/**
* Maps [oneOfName] and [fieldName] to the companion object key representing a boxed oneof field.
*/
fun boxedOneOfKeyFieldName(oneOfName: String, fieldName: String): String {
return (oneOfName + "_" + fieldName).toUpperCase()
}
/** Maps [oneOfName] to the companion object field of type `Set` containing the eligible keys. */
fun boxedOneOfKeysFieldName(oneOfName: String): String {
return "${oneOfName}_keys".toUpperCase()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy