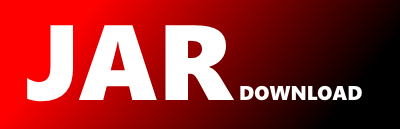
com.squareup.square.api.DefaultMerchantsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of square Show documentation
Show all versions of square Show documentation
Java client library for the Square API
package com.squareup.square.api;
import com.squareup.square.ApiHelper;
import com.squareup.square.Server;
import com.squareup.square.exceptions.ApiException;
import com.squareup.square.http.client.HttpContext;
import com.squareup.square.http.request.HttpMethod;
import com.squareup.square.models.ListMerchantsResponse;
import com.squareup.square.models.RetrieveMerchantResponse;
import io.apimatic.core.ApiCall;
import io.apimatic.core.GlobalConfiguration;
import java.io.IOException;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionException;
/**
* This class lists all the endpoints of the groups.
*/
public final class DefaultMerchantsApi extends BaseApi implements MerchantsApi {
/**
* Initializes the controller.
* @param globalConfig Configurations added in client.
*/
public DefaultMerchantsApi(GlobalConfiguration globalConfig) {
super(globalConfig);
}
/**
* Provides details about the merchant associated with a given access token. The access token
* used to connect your application to a Square seller is associated with a single merchant.
* That means that `ListMerchants` returns a list with a single `Merchant` object. You can
* specify your personal access token to get your own merchant information or specify an OAuth
* token to get the information for the merchant that granted your application access. If you
* know the merchant ID, you can also use the [RetrieveMerchant]($e/Merchants/RetrieveMerchant)
* endpoint to retrieve the merchant information.
* @param cursor Optional parameter: The cursor generated by the previous response.
* @return Returns the ListMerchantsResponse response from the API call
* @throws ApiException Represents error response from the server.
* @throws IOException Signals that an I/O exception of some sort has occurred.
*/
public ListMerchantsResponse listMerchants(
final Integer cursor) throws ApiException, IOException {
return prepareListMerchantsRequest(cursor).execute();
}
/**
* Provides details about the merchant associated with a given access token. The access token
* used to connect your application to a Square seller is associated with a single merchant.
* That means that `ListMerchants` returns a list with a single `Merchant` object. You can
* specify your personal access token to get your own merchant information or specify an OAuth
* token to get the information for the merchant that granted your application access. If you
* know the merchant ID, you can also use the [RetrieveMerchant]($e/Merchants/RetrieveMerchant)
* endpoint to retrieve the merchant information.
* @param cursor Optional parameter: The cursor generated by the previous response.
* @return Returns the ListMerchantsResponse response from the API call
*/
public CompletableFuture listMerchantsAsync(
final Integer cursor) {
try {
return prepareListMerchantsRequest(cursor).executeAsync();
} catch (Exception e) {
throw new CompletionException(e);
}
}
/**
* Builds the ApiCall object for listMerchants.
*/
private ApiCall prepareListMerchantsRequest(
final Integer cursor) throws IOException {
return new ApiCall.Builder()
.globalConfig(getGlobalConfiguration())
.requestBuilder(requestBuilder -> requestBuilder
.server(Server.ENUM_DEFAULT.value())
.path("/v2/merchants")
.queryParam(param -> param.key("cursor")
.value(cursor).isRequired(false))
.headerParam(param -> param.key("accept").value("application/json"))
.authenticationKey(BaseApi.AUTHENTICATION_KEY)
.httpMethod(HttpMethod.GET))
.responseHandler(responseHandler -> responseHandler
.deserializer(
response -> ApiHelper.deserialize(response, ListMerchantsResponse.class))
.nullify404(false)
.contextInitializer((context, result) ->
result.toBuilder().httpContext((HttpContext)context).build())
.globalErrorCase(GLOBAL_ERROR_CASES))
.build();
}
/**
* Retrieves the `Merchant` object for the given `merchant_id`.
* @param merchantId Required parameter: The ID of the merchant to retrieve. If the string
* "me" is supplied as the ID, then retrieve the merchant that is currently accessible
* to this call.
* @return Returns the RetrieveMerchantResponse response from the API call
* @throws ApiException Represents error response from the server.
* @throws IOException Signals that an I/O exception of some sort has occurred.
*/
public RetrieveMerchantResponse retrieveMerchant(
final String merchantId) throws ApiException, IOException {
return prepareRetrieveMerchantRequest(merchantId).execute();
}
/**
* Retrieves the `Merchant` object for the given `merchant_id`.
* @param merchantId Required parameter: The ID of the merchant to retrieve. If the string
* "me" is supplied as the ID, then retrieve the merchant that is currently accessible
* to this call.
* @return Returns the RetrieveMerchantResponse response from the API call
*/
public CompletableFuture retrieveMerchantAsync(
final String merchantId) {
try {
return prepareRetrieveMerchantRequest(merchantId).executeAsync();
} catch (Exception e) {
throw new CompletionException(e);
}
}
/**
* Builds the ApiCall object for retrieveMerchant.
*/
private ApiCall prepareRetrieveMerchantRequest(
final String merchantId) throws IOException {
return new ApiCall.Builder()
.globalConfig(getGlobalConfiguration())
.requestBuilder(requestBuilder -> requestBuilder
.server(Server.ENUM_DEFAULT.value())
.path("/v2/merchants/{merchant_id}")
.templateParam(param -> param.key("merchant_id").value(merchantId)
.shouldEncode(true))
.headerParam(param -> param.key("accept").value("application/json"))
.authenticationKey(BaseApi.AUTHENTICATION_KEY)
.httpMethod(HttpMethod.GET))
.responseHandler(responseHandler -> responseHandler
.deserializer(
response -> ApiHelper.deserialize(response, RetrieveMerchantResponse.class))
.nullify404(false)
.contextInitializer((context, result) ->
result.toBuilder().httpContext((HttpContext)context).build())
.globalErrorCase(GLOBAL_ERROR_CASES))
.build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy