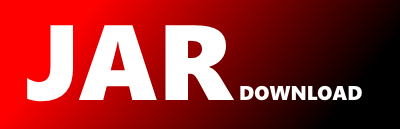
com.squareup.square.models.InventoryAdjustmentGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of square Show documentation
Show all versions of square Show documentation
Java client library for the Square API
package com.squareup.square.models;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonGetter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Objects;
/**
* This is a model class for InventoryAdjustmentGroup type.
*/
public class InventoryAdjustmentGroup {
private final String id;
private final String rootAdjustmentId;
private final String fromState;
private final String toState;
/**
* Initialization constructor.
* @param id String value for id.
* @param rootAdjustmentId String value for rootAdjustmentId.
* @param fromState String value for fromState.
* @param toState String value for toState.
*/
@JsonCreator
public InventoryAdjustmentGroup(
@JsonProperty("id") String id,
@JsonProperty("root_adjustment_id") String rootAdjustmentId,
@JsonProperty("from_state") String fromState,
@JsonProperty("to_state") String toState) {
this.id = id;
this.rootAdjustmentId = rootAdjustmentId;
this.fromState = fromState;
this.toState = toState;
}
/**
* Getter for Id.
* A unique ID generated by Square for the `InventoryAdjustmentGroup`.
* @return Returns the String
*/
@JsonGetter("id")
@JsonInclude(JsonInclude.Include.NON_NULL)
public String getId() {
return id;
}
/**
* Getter for RootAdjustmentId.
* The inventory adjustment of the composed variation.
* @return Returns the String
*/
@JsonGetter("root_adjustment_id")
@JsonInclude(JsonInclude.Include.NON_NULL)
public String getRootAdjustmentId() {
return rootAdjustmentId;
}
/**
* Getter for FromState.
* Indicates the state of a tracked item quantity in the lifecycle of goods.
* @return Returns the String
*/
@JsonGetter("from_state")
@JsonInclude(JsonInclude.Include.NON_NULL)
public String getFromState() {
return fromState;
}
/**
* Getter for ToState.
* Indicates the state of a tracked item quantity in the lifecycle of goods.
* @return Returns the String
*/
@JsonGetter("to_state")
@JsonInclude(JsonInclude.Include.NON_NULL)
public String getToState() {
return toState;
}
@Override
public int hashCode() {
return Objects.hash(id, rootAdjustmentId, fromState, toState);
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof InventoryAdjustmentGroup)) {
return false;
}
InventoryAdjustmentGroup other = (InventoryAdjustmentGroup) obj;
return Objects.equals(id, other.id)
&& Objects.equals(rootAdjustmentId, other.rootAdjustmentId)
&& Objects.equals(fromState, other.fromState)
&& Objects.equals(toState, other.toState);
}
/**
* Converts this InventoryAdjustmentGroup into string format.
* @return String representation of this class
*/
@Override
public String toString() {
return "InventoryAdjustmentGroup [" + "id=" + id + ", rootAdjustmentId=" + rootAdjustmentId
+ ", fromState=" + fromState + ", toState=" + toState + "]";
}
/**
* Builds a new {@link InventoryAdjustmentGroup.Builder} object.
* Creates the instance with the state of the current model.
* @return a new {@link InventoryAdjustmentGroup.Builder} object
*/
public Builder toBuilder() {
Builder builder = new Builder()
.id(getId())
.rootAdjustmentId(getRootAdjustmentId())
.fromState(getFromState())
.toState(getToState());
return builder;
}
/**
* Class to build instances of {@link InventoryAdjustmentGroup}.
*/
public static class Builder {
private String id;
private String rootAdjustmentId;
private String fromState;
private String toState;
/**
* Setter for id.
* @param id String value for id.
* @return Builder
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* Setter for rootAdjustmentId.
* @param rootAdjustmentId String value for rootAdjustmentId.
* @return Builder
*/
public Builder rootAdjustmentId(String rootAdjustmentId) {
this.rootAdjustmentId = rootAdjustmentId;
return this;
}
/**
* Setter for fromState.
* @param fromState String value for fromState.
* @return Builder
*/
public Builder fromState(String fromState) {
this.fromState = fromState;
return this;
}
/**
* Setter for toState.
* @param toState String value for toState.
* @return Builder
*/
public Builder toState(String toState) {
this.toState = toState;
return this;
}
/**
* Builds a new {@link InventoryAdjustmentGroup} object using the set fields.
* @return {@link InventoryAdjustmentGroup}
*/
public InventoryAdjustmentGroup build() {
return new InventoryAdjustmentGroup(id, rootAdjustmentId, fromState, toState);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy