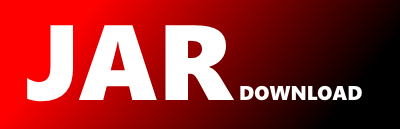
com.sourceclear.plugins.Config Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of srcclr-maven-plugin Show documentation
Show all versions of srcclr-maven-plugin Show documentation
The SRC:CLR Maven Plugin analyzes the dependencies of your project, both immediate and transitive, to
see if you are including any known security vulnerabilities through third-party packages in your
project.
/*
* © Copyright - SourceClear Inc
*/
package com.sourceclear.plugins;
import com.sourceclear.util.config.FailureLevel;
import org.apache.maven.plugin.logging.Log;
import org.apache.maven.settings.Settings;
import java.io.File;
import java.net.URI;
import java.util.Optional;
/**
* A single place to store all configuration parameters and pass it around.
*/
public class Config {
///////////////////////////// Class Attributes \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
public static class Builder {
private boolean useOnlyMavenParams = false;
private String projectName;
private Long userProjectID;
private boolean upload;
private FailureLevel failureThreshold;
private String apiToken;
private URI apiURL;
private boolean licenseProvided = false;
private File pathToTop;
private Log logger;
private Settings settings;
private File headlessOutputFile;
private boolean verbose;
private String pluginVersion;
private String workspaceSlug;
public Builder withUseOnlyMavenParams(boolean useOnlyMavenParams) {
this.useOnlyMavenParams = useOnlyMavenParams;
return this;
}
public Builder withProjectName(String projectName) {
this.projectName = projectName;
return this;
}
public Builder withUserProjectID(Long userProjectID) {
this.userProjectID = userProjectID;
return this;
}
public Builder withUpload(boolean upload) {
this.upload = upload;
return this;
}
public Builder withFailureThreshold(FailureLevel failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
public Builder withApiToken(String apiToken) {
this.apiToken = apiToken;
return this;
}
public Builder withApiURL(URI apiURL) {
this.apiURL = apiURL;
return this;
}
public Builder withLicenseProvided(boolean licenseProvided) {
this.licenseProvided = licenseProvided;
return this;
}
public Builder withPathToTop(File pathToTop) {
this.pathToTop = pathToTop;
return this;
}
public Builder withLogger(Log logger) {
this.logger = logger;
return this;
}
public Builder withSettings(Settings settings) {
this.settings = settings;
return this;
}
public Builder withHeadlessOutputFile(File headlessOutputFile) {
this.headlessOutputFile = headlessOutputFile;
return this;
}
public Builder withVerbose(boolean verbose) {
this.verbose = verbose;
return this;
}
public Builder withPluginVersion(String version) {
this.pluginVersion = version;
return this;
}
public Builder withWorkspaceSlug(String workspaceSlug) {
this.workspaceSlug = workspaceSlug;
return this;
}
public Config build() {
return new Config(this);
}
}
////////////////////////////// Class Methods \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
//////////////////////////////// Attributes \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
private final boolean useOnlyMavenParams;
private final String projectName;
private final Long userProjectID;
private final boolean upload;
private final FailureLevel failureThreshold;
private final String apiToken;
private final URI apiURL;
private final boolean licenseProvided;
private final File pathToTop;
private final Log logger;
private final Settings settings;
private final File headlessOutputFile;
private final boolean verbose;
private final String pluginVersion;
private final String workspaceSlug;
/////////////////////////////// Constructors \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
public Config(Builder builder) {
this.useOnlyMavenParams = builder.useOnlyMavenParams;
this.projectName = builder.projectName;
this.userProjectID = builder.userProjectID;
this.upload = builder.upload;
this.failureThreshold = builder.failureThreshold;
this.apiToken = builder.apiToken;
this.apiURL = builder.apiURL;
this.licenseProvided = builder.licenseProvided;
this.pathToTop = builder.pathToTop;
this.logger = builder.logger;
this.settings = builder.settings;
this.headlessOutputFile = builder.headlessOutputFile;
this.verbose = builder.verbose;
this.pluginVersion = builder.pluginVersion;
this.workspaceSlug = builder.workspaceSlug;
}
////////////////////////////////// Methods \\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\
public boolean useOnlyMavenParams() {
return useOnlyMavenParams;
}
public String getProjectName() {
return projectName;
}
public Long getUserProjectID() {
return userProjectID;
}
public boolean getUpload() {
return upload;
}
public FailureLevel getFailureThreshold() {
return failureThreshold;
}
public String getApiToken() {
return apiToken;
}
public URI getApiURL() {
return apiURL;
}
public boolean licenseProvided() {
return licenseProvided;
}
public File getPathToTop() {
return pathToTop;
}
public Log getLogger() {
return logger;
}
public Settings getSettings() {
return settings;
}
public Optional getHeadlessOutputFile() {
return Optional.ofNullable(headlessOutputFile);
}
public boolean verbose() {
return verbose;
}
public String getPluginVersion() {
return pluginVersion;
}
public Optional getWorkspaceSlug() {
return Optional.ofNullable(workspaceSlug);
}
//------------------------ Implements:
//------------------------ Overrides:
//---------------------------- Abstract Methods -----------------------------
//---------------------------- Utility Methods ------------------------------
public static Builder builder() {
return new Builder();
}
//---------------------------- Property Methods -----------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy