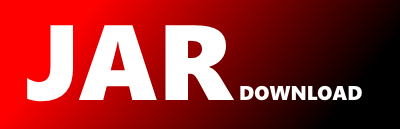
com.sta.cts.HXMLHandler Maven / Gradle / Ivy
package com.sta.cts;
import java.util.Vector;
import java.util.Hashtable;
import java.io.IOException;
import org.xml.sax.XMLReader;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
import org.xml.sax.helpers.XMLReaderFactory;
import com.sta.mlogger.MLogger;
/**
* Name: HXMLHandler
* Description:
* Klasse zur Realisierung der HXML-Transformation.
*
* Copyright: Copyright (c) 2001-2004, 2012, 2014, 2016, 2017, 2019, 2021
* Company: >StA-Soft<
* @author StA
* @version 1.0
*/
public class HXMLHandler extends DefaultHandler
{
/**
* Namen.
*/
private Vector myVNames;
/**
* Attribute.
*/
private Vector myVAttrs;
/**
* Spalte.
*/
private int sp;
/**
* Aktuell.
*/
private int cur;
/**
* XML-Generator.
*/
private XMLGenerator xg;
//===========================================================================
/**
* Attribute in Hash-Tabelle ?berf?hren.
* @param attrs Attribute
* @return die erstellte Hash-Tabelle
*/
private Hashtable convAttr2HT(Attributes attrs)
{
Hashtable ht = new Hashtable();
int s = attrs.getLength();
for (int i = 0; i < s; i++)
{
String n = attrs.getQName(i);
String v = attrs.getValue(i);
ht.put(n, v);
}
return ht;
}
/**
* Hash-Tabelle mit Attributen vergleichen.
* @param ht Hash-Table
* @param attrs Attribute
* @return Vergleichsergebnis
*/
private boolean compareHT2Attr(Hashtable ht, Attributes attrs)
{
int s1 = attrs.getLength();
int s2 = ht.size();
if (s1 != s2)
{
return false;
}
for (int i = 0; i < s1; i++)
{
String n = attrs.getQName(i);
String v1 = attrs.getValue(i);
String v2 = (String) ht.get(n);
if ((v1 != null) & (v2 == null))
{
return false;
}
if ((v1 == null) & (v2 != null))
{
return false;
}
if ((v1 != null) & (v2 != null))
{
if (!v1.equals(v2))
{
return false;
}
}
}
return true;
}
/**
* Flush.
* @throws SAXException im Fehlerfall
*/
private void flush() throws SAXException
{
try
{
int i = sp - 1;
while (i >= cur)
{
xg.closeTag((String) myVNames.elementAt(i));
myVNames.remove(i);
myVAttrs.remove(i);
i--;
}
sp = cur;
}
catch (IOException e)
{
throw new SAXException(e.getMessage());
}
}
/**
* Tag mit Attributen ?ffnen.
* @param pName Tag-Name
* @param pAttrs Attribute
* @throws SAXException im Fehlerfall
*/
private void openTagWithAttr(String pName, Attributes pAttrs) throws SAXException
{
try
{
xg.openTag(pName);
int cnt = pAttrs.getLength();
for (int i = 0; i < cnt; i++)
{
String n = pAttrs.getQName(i);
String v = pAttrs.getValue(i);
xg.putAttr(n, v);
}
myVNames.add(sp, pName);
myVAttrs.add(sp, convAttr2HT(pAttrs));
sp++;
cur++;
}
catch (IOException e)
{
throw new SAXException(e.getMessage());
}
}
@Override
public void startDocument() throws SAXException
{
myVNames = new Vector();
myVAttrs = new Vector();
sp = 0;
cur = 0;
}
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException
{
if (cur < sp)
{
String n = (String) myVNames.elementAt(cur);
Hashtable ht = (Hashtable) myVAttrs.elementAt(cur);
if ((n.equals(localName)) & compareHT2Attr(ht, attributes)) // && ?
{
cur++;
}
else
{
flush();
openTagWithAttr(localName, attributes);
}
}
else
{
openTagWithAttr(localName, attributes);
}
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException
{
// Optimierung m?glich?
String s = new String(ch, start, length);
s = s.trim();
if (s.length() != 0)
{
try
{
flush();
xg.putContent(s);
}
catch (IOException e)
{
throw new SAXException(e.getMessage());
}
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException
{
if (cur <= 0)
{
MLogger.err("Error: cur <= 0");
throw new SAXException("Error: cur <= 0");
}
cur--;
if (!(myVNames.elementAt(cur)).equals(localName))
{
MLogger.err("Error: Invalid Name");
throw new SAXException("Error: Invalid Name");
}
}
@Override
public void endDocument() throws SAXException
{
cur = 0;
flush();
}
/**
* Main-Methode.
* @param pSrcFileName Quelldateiname
* @param pDstFileName Zieldateiname
*/
public static void main(String pSrcFileName, String pDstFileName)
{
try
{
XMLReader parser = XMLReaderFactory.createXMLReader("org.apache.xerces.parsers.SAXParser");
HXMLHandler aHXMLHandlerInstance = new HXMLHandler();
aHXMLHandlerInstance.xg = new XMLGenerator();
aHXMLHandlerInstance.xg.createXML(pDstFileName);
parser.setContentHandler(aHXMLHandlerInstance);
parser.parse(pSrcFileName);
aHXMLHandlerInstance.xg.closeXML();
}
catch (IOException ioe)
{
MLogger.err("", ioe);
}
catch (SAXException saxe)
{
MLogger.err("", saxe);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy