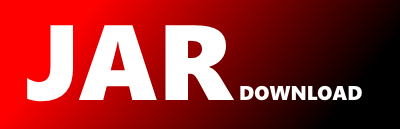
com.sta.cts.Lines2Hierarchy Maven / Gradle / Ivy
package com.sta.cts;
import java.util.Vector;
import java.util.Hashtable;
import java.util.Enumeration;
import com.sta.mlogger.MLogger;
/**
* Name: Lines2Hierarchy
* Description: Klasse zur Hierarchisierung einer Zeilenstruktur.
*
* Copyright: Copyright (c) 2002-2004, 2012, 2014, 2016-2019
* Company: >StA-Soft<
* @author StA
* @version 1.0
*/
public class Lines2Hierarchy
{
/**
* Standard-Root-Tag-Name.
*/
private static final String ROOT = "Root";
/**
* Standard-Line-Tag-Name.
*/
private static final String LINE = "Line";
/**
* Attribute.
*/
private Vector myAttributes = new Vector();
/**
* Hierarchie-Tag-Namen.
*/
private Vector myHTagNames = new Vector();
/**
* Tag-Namen.
*/
private Vector myTagNames = new Vector();
/**
* Werte.
*/
private Vector myValues = new Vector();
/**
* Root-Tag-Name.
*/
private String myRootTagName = null;
/**
* Hierarchie-Count.
*/
private int myHCount = 0;
/**
* Zustand.
*/
private int myState = 0;
/**
* Hierarchie-Inhalt lesen.
* @param xs XML-Scanner
* @throws Exception im Fehlerfall
*/
private void readHierarchyCont(XMLScanner xs) throws Exception
{
XMLTag tag = xs.getLeafStart(null);
if (tag != null)
{
String aTagName = tag.getName();
myTagNames.add(myHCount, aTagName);
Enumeration names = tag.getAttrNames();
Hashtable attrs = new Hashtable();
if (names != null)
{
while (names.hasMoreElements())
{
String attrName = names.nextElement();
String attrValue = tag.getAttr(attrName);
if (attrName.equals("htagname"))
{
myHTagNames.add(myHCount, attrValue);
}
else
{
attrs.put(attrName, attrValue);
}
}
}
myAttributes.add(myHCount, attrs);
myHCount++;
readHierarchyCont(xs);
tag = xs.getLeafEnd(null);
if (tag == null)
{
throw new Exception("Missing root end tag (" + aTagName + ").");
}
if (!tag.getName().equals(aTagName))
{
throw new Exception("Invalid root end tag (" + tag.getName() + ").");
}
}
}
/**
* Hierarchie-Beschreibung lesen.
* @param pDscFileName Beschreibungsdateiname
* @throws Exception im Fehlerfall
*/
private void readHierarchyDesc(String pDscFileName) throws Exception
{
myAttributes.clear();
myHTagNames.clear();
myTagNames.clear();
myRootTagName = null;
myHCount = 0;
XMLScanner xs = new XMLScanner();
xs.init(pDscFileName);
xs.initH(null);
XMLTag tag = xs.getLeafStart(null);
if (tag != null)
{
myRootTagName = tag.getName();
readHierarchyCont(xs);
tag = xs.getLeafEnd(null);
if (tag == null)
{
throw new Exception("Missing root end tag (" + myRootTagName + ").");
}
if (!tag.getName().equals(myRootTagName))
{
throw new Exception("Invalid root end tag (" + tag.getName() + ").");
}
}
xs.done();
}
/**
* Daten einer Zeile lesen.
* @param xs XML-Scanner
* @return XML-Leaf-Hash-Tabelle
* @throws Exception im Fehlerfall
*/
private LeafHashtable readLine(XMLScanner xs) throws Exception
{
LeafHashtable lht = null;
XMLTag tag = xs.getLeafStart(LINE);
if (tag != null)
{
lht = xs.getLeafs();
tag = xs.getLeafEnd(LINE);
if (tag == null)
{
throw new Exception("Missing line end tag (" + ROOT + ")");
}
}
return lht;
}
/**
* Lines2Hierarchy starten.
* @param pSrcFileName Quelldateiname
* @param pDstFileName Zieldateiname
* @throws Exception im Fehlerfall
*/
private void runLines2Hierarchy(String pSrcFileName, String pDstFileName) throws Exception
{
myValues.clear();
for (int i = 0; i < myHCount; i++)
{
myValues.add(null);
}
myState = -1;
XMLScanner xs = new XMLScanner();
XMLTag tag;
xs.init(pSrcFileName);
xs.initH(null);
try
{
tag = xs.getLeafStart(ROOT);
if (tag != null)
{
XMLGenerator xg = new XMLGenerator();
xg.createXML(pDstFileName);
try
{
xg.openTag(myRootTagName);
LeafHashtable line = null;
do
{
// Zeile einlesen ...
line = readLine(xs);
if (line != null)
{
// zentraler Vergleichs-Alg.
boolean curChange = false;
boolean change = false;
int i = 0;
while ((i < myHCount) && (!change || curChange))
{
curChange = false;
String locHTagName = (String) myHTagNames.get(i);
String locHTagValue = (String) line.get(locHTagName);
String value = (String) myValues.get(i);
if ((locHTagValue != null) && (!locHTagValue.equals("")))
{
if ((value == null) || (!locHTagValue.equals(value))) // Wechsel !!!
{
curChange = true;
change = true;
// schlie?e alle ev. noch offenen Tags:
for (int j = myState; j >= i; j--)
{
xg.closeTag((String) myTagNames.get(j));
}
// neuen Wert merken
myValues.set(i, locHTagValue);
// folgende Werte l?schen
for (int j = i + 1; j < myHCount; j++)
{
myValues.set(j, null);
}
// neuer Status
myState = i;
// neues Tag ?ffnen und Attribute kopieren
xg.openTag((String) myTagNames.get(i));
Hashtable attrs = (Hashtable) myAttributes.get(i);
Enumeration e = attrs.keys();
while (e.hasMoreElements())
{
String attrName = (String) e.nextElement();
String attrValue = (String) attrs.get(attrName);
String newValue = (String) line.get(attrValue);
if ((newValue != null) && (newValue.length() != 0))
{
xg.putAttr(attrName, newValue);
}
}
}
}
i++;
}
}
}
while (line != null);
// Rest schreiben ...
// schlie?e alle ev. noch offenen Tags:
for (int j = myState; j >= 0; j--)
{
xg.closeTag((String) myTagNames.get(j));
}
xg.closeTag(myRootTagName);
}
finally
{
xg.closeXML();
}
}
tag = xs.getLeafEnd(ROOT);
if (tag == null)
{
throw new Exception("Missing root end tag (" + ROOT + ")");
}
}
finally
{
xs.done();
}
}
/**
* Zentrale Run-Methode.
* @param pSrcFileName Quelldateiname
* @param pDscFileName Beschreibungsdateiname
* @param pDstFileName Zieldateiname
* @throws Exception im Fehlerfall
*/
private void run(String pSrcFileName, String pDscFileName, String pDstFileName) throws Exception
{
readHierarchyDesc(pDscFileName);
runLines2Hierarchy(pSrcFileName, pDstFileName);
}
/**
* Alternative Main-Methode.
* @param pSrcFileName Quelldateiname
* @param pDscFileName Beschreibungsdateiname
* @param pDstFileName Zieldateiname
* @throws Exception im Fehlerfall
*/
public static void main(String pSrcFileName, String pDscFileName, String pDstFileName) throws Exception
{
Lines2Hierarchy l2h = new Lines2Hierarchy();
l2h.run(pSrcFileName, pDscFileName, pDstFileName);
}
/**
* Main-Methode zu Testzwecken.
* @param args Argumente
*/
public static void main(String[] args)
{
try
{
main("", "d:/alfred2/generator2/gen/source/xml/db_pdm_erwin_hierarchy.xml", "");
// main(args[0], args[1], args[2]);
}
catch (Exception ex)
{
MLogger.err("", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy