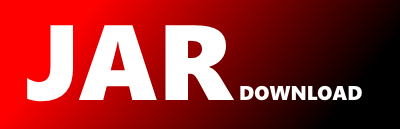
com.sta.cts.hssf.CellStyleDesc Maven / Gradle / Ivy
package com.sta.cts.hssf;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFFont;
/**
* Name: CellStyleDesc
* Description:
* HSSF-Cell-Style-Descriptor, used to minimize the count of cell styles.
*
* Copyright: Copyright (c) 2003, 2004, 2016, 2017, 2019, 2021
* Company: >StA-Soft<
* @author StA
* @version 1.0
*/
public class CellStyleDesc
{
/**
* Alignment.
*/
private short myAlignment = HSSFCellStyle.ALIGN_GENERAL;
/**
* Border-Bottom.
*/
private short myBorderBottom = HSSFCellStyle.BORDER_NONE;
/**
* Border-Left.
*/
private short myBorderLeft = HSSFCellStyle.BORDER_NONE;
/**
* Border-Right.
*/
private short myBorderRight = HSSFCellStyle.BORDER_NONE;
/**
* Border-Top.
*/
private short myBorderTop = HSSFCellStyle.BORDER_NONE;
/**
* Border-Bottom-Color.
*/
private short myBottomBorderColor = HSSFCellStyle.AUTOMATIC;
/**
* Border-Left-Color.
*/
private short myLeftBorderColor = HSSFCellStyle.AUTOMATIC;
/**
* Border-Right-Color.
*/
private short myRightBorderColor = HSSFCellStyle.AUTOMATIC;
/**
* Border-Top-Color.
*/
private short myTopBorderColor = HSSFCellStyle.AUTOMATIC;
/* *
* Data-Format.
*/
// private short myDataFormat = .;
/**
* Fill-Background-Color.
*/
private short myFillBackgroundColor = HSSFCellStyle.AUTOMATIC;
/**
* Fill-Foreground-Color.
*/
private short myFillForegroundColor = HSSFCellStyle.AUTOMATIC;
/**
* Fill-Pattern.
*/
private short myFillPattern = HSSFCellStyle.NO_FILL;
/**
* Font.
*/
private HSSFFont myFont = null; // ???
/**
* Hidden.
*/
private boolean myHidden = false;
/**
* Indention.
*/
private short myIndention = 0;
/**
* Locked.
*/
private boolean myLocked = false;
/**
* Rotation.
*/
private short myRotation = 0;
/**
* Vertical-Alignment.
*/
private short myVerticalAlignment = HSSFCellStyle.VERTICAL_TOP;
/**
* Wrap-Text.
*/
private boolean myWrapText = false;
//===========================================================================
/**
* Standard-Constructor.
*/
public CellStyleDesc()
{
}
/**
* Constructor mit Cell-Style.
* @param cs Cell-Style
*/
public CellStyleDesc(HSSFCellStyle cs)
{
myAlignment = cs.getAlignment();
myBorderBottom = cs.getBorderBottom();
myBorderLeft = cs.getBorderLeft();
myBorderRight = cs.getBorderRight();
myBorderTop = cs.getBorderTop();
myBottomBorderColor = cs.getBottomBorderColor();
myLeftBorderColor = cs.getLeftBorderColor();
myRightBorderColor = cs.getRightBorderColor();
myTopBorderColor = cs.getTopBorderColor();
// myDataFormat = cs.getDataFormat();
myFillBackgroundColor = cs.getFillBackgroundColor();
myFillForegroundColor = cs.getFillForegroundColor();
myFillPattern = cs.getFillPattern();
myFont = null; // cs.getFontIndex(); ???
myHidden = cs.getHidden();
myIndention = cs.getIndention();
myLocked = cs.getLocked();
myRotation = cs.getRotation();
myVerticalAlignment = cs.getVerticalAlignment();
myWrapText = cs.getWrapText();
}
//===========================================================================
@Override
public boolean equals(Object other)
{
if (this == other)
{
return true;
}
if (other == null)
{
return false;
}
if (other.getClass() != getClass())
{
return false;
}
CellStyleDesc csd = (CellStyleDesc) other;
if (myAlignment != csd.myAlignment)
{
return false;
}
if (myBorderBottom != csd.myBorderBottom)
{
return false;
}
if (myBorderLeft != csd.myBorderLeft)
{
return false;
}
if (myBorderRight != csd.myBorderRight)
{
return false;
}
if (myBorderTop != csd.myBorderTop)
{
return false;
}
if (myBottomBorderColor != csd.myBottomBorderColor)
{
return false;
}
if (myLeftBorderColor != csd.myLeftBorderColor)
{
return false;
}
if (myRightBorderColor != csd.myRightBorderColor)
{
return false;
}
if (myTopBorderColor != csd.myTopBorderColor)
{
return false;
}
// if (myDataFormat != csd.myDataFormat)
// {
// return false;
// }
if (myFillBackgroundColor != csd.myFillBackgroundColor)
{
return false;
}
if (myFillForegroundColor != csd.myFillForegroundColor)
{
return false;
}
if (myFillPattern != csd.myFillPattern)
{
return false;
}
// if (myFont != csd.myFont) // ???
// {
// return false;
// }
if ((myFont == null) && (csd.myFont != null))
{
return false;
}
if ((myFont != null) && (csd.myFont == null))
{
return false;
}
if ((myFont != null) && (csd.myFont != null) && (myFont.getIndex() != (csd.myFont.getIndex())))
{
return false;
}
if (myHidden != csd.myHidden)
{
return false;
}
if (myIndention != csd.myIndention)
{
return false;
}
if (myLocked != csd.myLocked)
{
return false;
}
if (myRotation != csd.myRotation)
{
return false;
}
if (myVerticalAlignment != csd.myVerticalAlignment)
{
return false;
}
if (myWrapText != csd.myWrapText)
{
return false;
}
return true;
}
@Override
public int hashCode()
{
int hc = 17; // super.hashCode();
int mult = 59; // eine Primzahl
hc = hc * mult + myAlignment;
hc = hc * mult + myBorderBottom;
hc = hc * mult + myBorderLeft;
hc = hc * mult + myBorderRight;
hc = hc * mult + myBorderTop;
hc = hc * mult + myBottomBorderColor;
hc = hc * mult + myLeftBorderColor;
hc = hc * mult + myRightBorderColor;
hc = hc * mult + myTopBorderColor;
// hc = hc * mult + myDataFormat;
hc = hc * mult + myFillBackgroundColor;
hc = hc * mult + myFillForegroundColor;
hc = hc * mult + myFillPattern;
hc = hc * mult + (myFont == null ? 0 : 1); // ???
hc = hc * mult + (myHidden ? 1 : 0);
hc = hc * mult + myIndention;
hc = hc * mult + (myLocked ? 1 : 0);
hc = hc * mult + myRotation;
hc = hc * mult + myVerticalAlignment;
hc = hc * mult + (myWrapText ? 1 : 0);
return hc;
}
//---------------------------------------------------------------------------
/**
* Alignment vorgeben.
* @param pAlignment Alignment
*/
public void setAlignment(short pAlignment)
{
myAlignment = pAlignment;
}
/**
* Border-Bottom vorgeben.
* @param pBorderBottom Border-Bottom
*/
public void setBorderBottom(short pBorderBottom)
{
myBorderBottom = pBorderBottom;
}
/**
* Border-Left vorgeben.
* @param pBorderLeft Border-Left
*/
public void setBorderLeft(short pBorderLeft)
{
myBorderLeft = pBorderLeft;
}
/**
* Border-Right vorgeben.
* @param pBorderRight Border-Right
*/
public void setBorderRight(short pBorderRight)
{
myBorderRight = pBorderRight;
}
/**
* Border-Top vorgeben.
* @param pBorderTop Border-Top
*/
public void setBorderTop(short pBorderTop)
{
myBorderTop = pBorderTop;
}
/**
* Border-Bottom-Color vorgeben.
* @param pBottomBorderColor Border-Bottom-Color
*/
public void setBottomBorderColor(short pBottomBorderColor)
{
myBottomBorderColor = pBottomBorderColor;
}
/**
* Border-Left-Color vorgeben.
* @param pLeftBorderColor Border-Left-Color
*/
public void setLeftBorderColor(short pLeftBorderColor)
{
myLeftBorderColor = pLeftBorderColor;
}
/**
* Border-Right-Color vorgeben.
* @param pRightBorderColor Border-Right-Color
*/
public void setRightBorderColor(short pRightBorderColor)
{
myRightBorderColor = pRightBorderColor;
}
/**
* Border-Top-Color vorgeben.
* @param pTopBorderColor Border-Top-Color
*/
public void setTopBorderColor(short pTopBorderColor)
{
myTopBorderColor = pTopBorderColor;
}
/* *
* Data-Format vorgeben.
* @param pDataFormat Data-Format
*/
// public void setDataFormat(short pDataFormat)
// {
// myDataFormat = pDataFormat;
// }
/**
* Fill-Background-Color vorgeben.
* @param pFillBackgroundColor Fill-Background-Color
*/
public void setFillBackgroundColor(short pFillBackgroundColor)
{
myFillBackgroundColor = pFillBackgroundColor;
}
/**
* Fill-Foreground-Color vorgeben.
* @param pFillForegroundColor Fill-Foreground-Color
*/
public void setFillForegroundColor(short pFillForegroundColor)
{
myFillForegroundColor = pFillForegroundColor;
}
/**
* Fill-Pattern vorgeben.
* @param pFillPattern Fill-Pattern
*/
public void setFillPattern(short pFillPattern)
{
myFillPattern = pFillPattern;
}
/**
* Font vorgeben.
* @param pFont Font
*/
public void setFont(HSSFFont pFont)
{
myFont = pFont;
}
/**
* Hidden vorgeben.
* @param pHidden Hidden
*/
public void setHidden(boolean pHidden)
{
myHidden = pHidden;
}
/**
* Indention vorgeben.
* @param pIndention Indention
*/
public void setIndention(short pIndention)
{
myIndention = pIndention;
}
/**
* Locked vorgeben.
* @param pLocked Locked
*/
public void setLocked(boolean pLocked)
{
myLocked = pLocked;
}
/**
* Rotation vorgeben.
* @param pRotation Rotation
*/
public void setRotation(short pRotation)
{
myRotation = pRotation;
}
/**
* Vertical-Alignment vorgeben.
* @param pVerticalAlignment Vertical-Alignment
*/
public void setVerticalAlignment(short pVerticalAlignment)
{
myVerticalAlignment = pVerticalAlignment;
}
/**
* Wrap-Text vorgeben.
* @param pWrapText Wrap-Text
*/
public void setWrapText(boolean pWrapText)
{
myWrapText = pWrapText;
}
//---------------------------------------------------------------------------
/**
* Cell-Style ?bertragen, falls nicht Standard-Fall.
* @param cs Cell-Style
*/
public void fillCellStyle(HSSFCellStyle cs)
{
if (myAlignment != HSSFCellStyle.ALIGN_GENERAL)
{
cs.setAlignment(myAlignment);
}
if (myBorderBottom != HSSFCellStyle.BORDER_NONE)
{
cs.setBorderBottom(myBorderBottom);
}
if (myBorderLeft != HSSFCellStyle.BORDER_NONE)
{
cs.setBorderLeft(myBorderLeft);
}
if (myBorderRight != HSSFCellStyle.BORDER_NONE)
{
cs.setBorderRight(myBorderRight);
}
if (myBorderTop != HSSFCellStyle.BORDER_NONE)
{
cs.setBorderTop(myBorderTop);
}
if (myBottomBorderColor != HSSFCellStyle.AUTOMATIC)
{
cs.setBottomBorderColor(myBottomBorderColor);
}
if (myLeftBorderColor != HSSFCellStyle.AUTOMATIC)
{
cs.setLeftBorderColor(myLeftBorderColor);
}
if (myRightBorderColor != HSSFCellStyle.AUTOMATIC)
{
cs.setRightBorderColor(myRightBorderColor);
}
if (myTopBorderColor != HSSFCellStyle.AUTOMATIC)
{
cs.setTopBorderColor(myTopBorderColor);
}
// if (myDataFormat != .)
// {
// cs.setDataFormat(myDataFormat);
// }
if (myFillBackgroundColor != HSSFCellStyle.AUTOMATIC)
{
cs.setFillBackgroundColor(myFillBackgroundColor);
}
if (myFillForegroundColor != HSSFCellStyle.AUTOMATIC)
{
cs.setFillForegroundColor(myFillForegroundColor);
}
if (myFillPattern != HSSFCellStyle.NO_FILL)
{
cs.setFillPattern(myFillPattern);
}
if (myFont != null)
{
cs.setFont(myFont);
}
if (myHidden)
{
cs.setHidden(myHidden);
}
if (myIndention != 0)
{
cs.setIndention(myIndention);
}
if (myLocked)
{
cs.setLocked(myLocked);
}
if (myRotation != 0)
{
cs.setRotation(myRotation);
}
// !!!
cs.setVerticalAlignment(myVerticalAlignment);
if (myWrapText)
{
cs.setWrapText(myWrapText);
}
}
@Override
public String toString()
{
return "Alignment: " + myAlignment + " " +
"BorderBottom: " + myBorderBottom + " " +
"BorderLeft: " + myBorderLeft + " " +
"BorderRight: " + myBorderRight + " " +
"BorderTop: " + myBorderTop + " " +
"BottomBorderColor: " + myBottomBorderColor + " " +
"LeftBorderColor: " + myLeftBorderColor + " " +
"RightBorderColor: " + myRightBorderColor + " " +
"TopBorderColor: " + myTopBorderColor + " " +
// "DataFormat: " + myDataFormat + " " +
"FillBackgroundColor: " + myFillBackgroundColor + " " +
"FillForegroundColor: " + myFillForegroundColor + " " +
"FillPattern: " + myFillPattern + " " +
"Font: " + myFont + " " +
"Hidden: " + myHidden + " " +
"Indention: " + myIndention + " " +
"Locked: " + myLocked + " " +
"Rotation: " + myRotation + " " +
"VerticalAlignment: " + myVerticalAlignment + " " +
"WrapText: " + myWrapText;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy