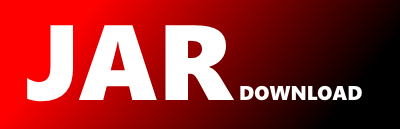
com.sta.cts.hssf.FontDesc Maven / Gradle / Ivy
package com.sta.cts.hssf;
import org.apache.poi.hssf.usermodel.HSSFFont;
/**
* Name: FontDesc
* Description:
* HSSF-Font-Descriptor, used to minimize the count of fonts.
*
* Copyright: Copyright (c) 2003, 2016, 2017, 2019, 2021
* Company: >StA-Soft<
* @author StA
* @version 1.0
*/
public class FontDesc
{
/**
* Bold-Weight.
*/
private short myBoldweight = HSSFFont.BOLDWEIGHT_NORMAL;
/**
* Color.
*/
private short myColor = HSSFFont.AUTOMATIC;
/**
* Font-Height.
*/
private short myFontHeightInPoints = 10;
/**
* Font-Name.
*/
private String myFontName = "Arial";
/**
* Italic.
*/
private boolean myItalic = false;
/**
* Strike-Out.
*/
private boolean myStrikeout = false;
/**
* Type-Offset.
*/
private short myTypeOffset = HSSFFont.SS_NONE;
/**
* Unterline.
*/
private short myUnderline = HSSFFont.U_NONE;
//===========================================================================
/**
* Standard-Constructor.
*/
public FontDesc()
{
}
/**
* Constructor mit Font.
* @param font Font
*/
public FontDesc(HSSFFont font)
{
setBoldweight(font.getBoldweight());
setColor(font.getColor());
setFontHeightInPoints(font.getFontHeightInPoints());
setFontName(font.getFontName());
setItalic(font.getItalic());
setStrikeout(font.getStrikeout());
setTypeOffset(font.getTypeOffset());
setUnderline(font.getUnderline());
}
//===========================================================================
@Override
public boolean equals(Object other)
{
if (this == other)
{
return true;
}
if (other == null)
{
return false;
}
if (other.getClass() != getClass())
{
return false;
}
FontDesc fd = (FontDesc) other;
if (myBoldweight != fd.myBoldweight)
{
return false;
}
if (myColor != fd.myColor)
{
return false;
}
if (myFontHeightInPoints != fd.myFontHeightInPoints)
{
return false;
}
if ((myFontName == null) && (fd.myFontName != null))
{
return false;
}
if ((myFontName != null) && (!myFontName.equals(fd.myFontName)))
{
return false;
}
if (myItalic != fd.myItalic)
{
return false;
}
if (myStrikeout != fd.myStrikeout)
{
return false;
}
if (myTypeOffset != fd.myTypeOffset)
{
return false;
}
if (myUnderline != fd.myUnderline)
{
return false;
}
return true;
}
@Override
public int hashCode()
{
int hc = 17; // super.hashCode();
int mult = 59; // eine Primzahl
hc = hc * mult + myBoldweight;
hc = hc * mult + myColor;
hc = hc * mult + myFontHeightInPoints;
hc = hc * mult + (myFontName == null ? 0 : myFontName.hashCode());
hc = hc * mult + (myItalic ? 1 : 0);
hc = hc * mult + (myStrikeout ? 1 : 0);
hc = hc * mult + myTypeOffset;
hc = hc * mult + myUnderline;
return hc;
}
//---------------------------------------------------------------------------
/**
* Bold-Weight vorgeben.
* @param pBoldweight Bold-Weight
*/
public void setBoldweight(short pBoldweight)
{
myBoldweight = pBoldweight;
}
/**
* Color vorgeben.
* @param pColor Color
*/
public void setColor(short pColor)
{
myColor = pColor;
}
/**
* Font-Height vorgeben.
* @param pFontHeightInPoints Font-Height
*/
public void setFontHeightInPoints(short pFontHeightInPoints)
{
myFontHeightInPoints = pFontHeightInPoints;
}
/**
* Font-Name vorgeben.
* @param pFontName Font-Name
*/
public void setFontName(String pFontName)
{
myFontName = pFontName;
}
/**
* Italic vorgeben.
* @param pItalic Italic
*/
public void setItalic(boolean pItalic)
{
myItalic = pItalic;
}
/**
* Strike-Out vorgeben.
* @param pStrikeout Strike-Out
*/
public void setStrikeout(boolean pStrikeout)
{
myStrikeout = pStrikeout;
}
/**
* Type-Offset vorgeben.
* @param pTypeOffset Type-Offset
*/
public void setTypeOffset(short pTypeOffset)
{
myTypeOffset = pTypeOffset;
}
// public void setUnderline(byte pUnderline)
/**
* Underline vorgeben.
* @param pUnderline Underline
*/
public void setUnderline(short pUnderline)
{
myUnderline = pUnderline;
}
//---------------------------------------------------------------------------
/**
* Font ?bernehmen.
* @param font Font
*/
public void fillFont(HSSFFont font)
{
font.setBoldweight(myBoldweight);
font.setColor(myColor);
font.setFontHeightInPoints(myFontHeightInPoints);
font.setFontName(myFontName);
font.setItalic(myItalic);
font.setStrikeout(myStrikeout);
font.setTypeOffset(myTypeOffset);
font.setUnderline((byte) myUnderline);
}
@Override
public String toString()
{
return "Boldweight: " + myBoldweight + " " +
"Color: " + myColor + " " +
"FontHeightInPoints: " + myFontHeightInPoints + " " +
"FontName: " + myFontName + " " +
"Italic: " + myItalic + " " +
"Strikeout: " + myStrikeout + " " +
"TypeOffset: " + myTypeOffset + " " +
"Underline: " + myUnderline;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy