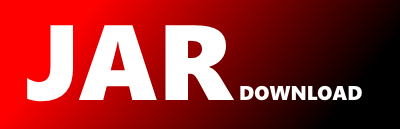
com.sta.cts.hssf.HSSF Maven / Gradle / Ivy
package com.sta.cts.hssf;
import org.xml.sax.SAXException;
import org.apache.poi.hssf.usermodel.HSSFFont;
import com.sta.mlogger.MLogger;
/**
* Name: HSSF
* Description:
* HSSF-Tools.
*
* Copyright: Copyright (c) 2003, 2014, 2016, 2017, 2019
* Company: >StA-Soft<
* @author StA
* @version 1.0
*/
public class HSSF
{
/**
* Tag f?r Workbook.
*/
public static final String TAG_WORKBOOK = "workbook";
/**
* Tag f?r Sheet.
*/
public static final String TAG_SHEET = "sheet";
/**
* Tag f?r Spalte.
*/
public static final String TAG_COLUMN = "column";
/**
* Tag f?r Zeile.
*/
public static final String TAG_ROW = "row";
/**
* Tag f?r Zelle.
*/
public static final String TAG_CELL = "cell";
// --- sheet ---
/**
* Attribut f?r Sheet-Name.
*/
public static final String ATTR_NAME = "name";
/**
* Attribut f?r Sheet-Alternative-Expression.
*/
public static final String ATTR_ALTERNATIVE_EXPRESSION = "alternative-expression";
/**
* Attribut f?r Sheet-Alternative-Formula.
*/
public static final String ATTR_ALTERNATIVE_FORMULA = "alternative-formula";
/**
* Attribut f?r Sheet-Auto-Breaks.
*/
public static final String ATTR_AUTO_BREAKS = "auto-breaks";
/**
* Attribut f?r Sheet-Default-Column-Width.
*/
public static final String ATTR_DEFAULT_COLUMN_WIDTH = "default-column-width";
/**
* Attribut f?r Sheet-Default-Row-Height.
*/
public static final String ATTR_DEFAULT_ROW_HEIGHT = "default-row-height";
/**
* Attribut f?r Sheet-Dialog.
*/
public static final String ATTR_DIALOG = "dialog";
/**
* Attribut f?r Sheet-Display-Guts.
*/
public static final String ATTR_DISPLAY_GUTS = "display-guts";
/**
* Attribut f?r Sheet-Fit-To-Page.
*/
public static final String ATTR_FIT_TO_PAGE = "fit-to-page";
/**
* Attribut f?r Sheet-Grids-Printed.
*/
public static final String ATTR_GRIDS_PRINTED = "grids-printed";
/**
* Attribut f?r Sheet-Row-Sums-Below.
*/
public static final String ATTR_ROW_SUMS_BELOW = "row-sums-below";
/**
* Attribut f?r Sheet-Row-Sums-Right.
*/
public static final String ATTR_ROW_SUMS_RIGHT = "row-sums-right";
/**
* Attribut f?r Sheet-Vertically-Center.
*/
public static final String ATTR_VERTICALLY_CENTER = "vertically-center";
/**
* Attribut f?r Sheet-Empty-Rows.
*/
public static final String ATTR_EMPTY_ROWS = "empty-rows";
// --- column ---
/**
* Attribut f?r Column-Width.
*/
public static final String ATTR_COLUMN_WIDTH = "column-width";
// --- row ---
/**
* Attribut f?r Row-Height.
*/
public static final String ATTR_ROW_HEIGHT = "row-height";
/**
* Attribut f?r Row-Empty-Cells.
*/
public static final String ATTR_EMPTY_CELLS = "empty-cells";
// --- cell ---
/**
* Attribut f?r Cell-Font-Name.
*/
public static final String ATTR_FONT_NAME = "font-name";
/**
* Attribut f?r Cell-Font-Size.
*/
public static final String ATTR_FONT_SIZE = "font-size";
/**
* Attribut f?r Cell-Color.
*/
public static final String ATTR_COLOR = "color";
/**
* Attribut f?r Cell-Color-Index.
*/
public static final String ATTR_COLOR_INDEX = "colorindex";
/**
* Attribut f?r Cell-Font-Weight.
*/
public static final String ATTR_FONT_WEIGHT = "font-weight";
/**
* Attribut f?r Cell-Font-Style.
*/
public static final String ATTR_FONT_STYLE = "font-style";
/**
* Attribut f?r Cell-Text-Deco.
*/
public static final String ATTR_TEXT_DECO = "text-deco";
/**
* Attribut f?r Cell-Underline.
*/
public static final String ATTR_UNDERLINE = "underline";
/**
* Attribut f?r Cell-Type-Offset.
*/
public static final String ATTR_TYPE_OFFSET = "typeoffset";
/**
* Attribut f?r Cell-Rotation.
*/
public static final String ATTR_ROTATION = "rotation";
/**
* Attribut f?r Cell-Indent.
*/
public static final String ATTR_INDENT = "indent";
/**
* Attribut f?r Cell-Fill-Background-Color.
*/
public static final String ATTR_FILL_BACKGROUND_COLOR = "fillbackgroundcolor";
/**
* Attribut f?r Cell-Fill-Foreground-Color.
*/
public static final String ATTR_FILL_FOREGROUND_COLOR = "fillforegroundcolor";
/**
* Attribut f?r Cell-Fill-Pattern.
*/
public static final String ATTR_FILL_PATTERN = "fillpattern";
/**
* Attribut f?r Cell-BG-Color.
*/
public static final String ATTR_BG_COLOR = "bgcolor";
/**
* Attribut f?r Cell-Border-Bottom.
*/
public static final String ATTR_BORDER_BOTTOM = "borderbottom";
/**
* Attribut f?r Cell-Border-Left.
*/
public static final String ATTR_BORDER_LEFT = "borderleft";
/**
* Attribut f?r Cell-Border-Right.
*/
public static final String ATTR_BORDER_RIGHT = "borderright";
/**
* Attribut f?r Cell-Border-Top.
*/
public static final String ATTR_BORDER_TOP = "bordertop";
/**
* Attribut f?r Cell-Border-Bottom-Color.
*/
public static final String ATTR_BORDER_BOTTOM_COLOR = "borderbottomcolor";
/**
* Attribut f?r Cell-Border-Left-Color.
*/
public static final String ATTR_BORDER_LEFT_COLOR = "borderleftcolor";
/**
* Attribut f?r Cell-Border-Right-Color.
*/
public static final String ATTR_BORDER_RIGHT_COLOR = "borderrightcolor";
/**
* Attribut f?r Cell-Border-Top-Color.
*/
public static final String ATTR_BORDER_TOP_COLOR = "bordertopcolor";
/**
* Attribut f?r Cell-Align.
*/
public static final String ATTR_ALIGN = "align";
/**
* Attribut f?r Cell-V-Align.
*/
public static final String ATTR_V_ALIGN = "valign";
/**
* Attribut f?r Cell-Wrap.
*/
public static final String ATTR_WRAP = "wrap";
/**
* Attribut f?r Cell-Locked.
*/
public static final String ATTR_LOCKED = "locked";
/**
* Attribut f?r Cell-Hidden.
*/
public static final String ATTR_HIDDEN = "hidden";
/**
* Attribut f?r Cell-Type.
*/
public static final String ATTR_CELL_TYPE = "cell-type";
/**
* Attribut f?r Cell-Row-Span.
*/
public static final String ATTR_ROW_SPAN = "row-span";
/**
* Attribut f?r Cell-Span.
*/
public static final String ATTR_CELL_SPAN = "cell-span";
// --- standard values (sheet) ---
/**
* Attribut f?r Sheet-Standard-Wert-Alternative-Expression.
*/
public static final boolean STD_ALTERNATIVE_EXPRESSION = true;
/**
* Attribut f?r Sheet-Standard-Wert-Alternative-Formula.
*/
public static final boolean STD_ALTERNATIVE_FORMULA = true;
/**
* Attribut f?r Sheet-Standard-Wert-Auto-Breaks.
*/
public static final boolean STD_AUTO_BREAKS = false;
/**
* Attribut f?r Sheet-Standard-Wert-Default-Column-Width.
*/
public static final int STD_DEFAULT_COLUMN_WIDTH = 8;
/**
* Attribut f?r Sheet-Standard-Wert-Default-Row-Height.
*/
public static final float STD_DEFAULT_ROW_HEIGHT = 12;
/**
* Attribut f?r Sheet-Standard-Wert-Row-Height.
*/
public static final float STD_ROW_HEIGHT = 12;
/**
* Attribut f?r Sheet-Standard-Wert-Dialog.
*/
public static final boolean STD_DIALOG = false;
/**
* Attribut f?r Sheet-Standard-Wert-Display-Guts.
*/
public static final boolean STD_DISPLAY_GUTS = false;
/**
* Attribut f?r Sheet-Standard-Wert-Fit-To-Page.
*/
public static final boolean STD_FIT_TO_PAGE = true;
/**
* Attribut f?r Sheet-Standard-Wert-Row-Sums-Below.
*/
public static final boolean STD_ROW_SUMS_BELOW = false;
/**
* Attribut f?r Sheet-Standard-Wert-Row-Sums-Right.
*/
public static final boolean STD_ROW_SUMS_RIGHT = false;
/**
* Attribut f?r Sheet-Standard-Wert-Vertically-Center.
*/
public static final boolean STD_VERTICALLY_CENTER = false;
// --- standard values (cell) ---
/**
* Attribut f?r Cell-Standard-Wert-Font-Name.
*/
public static final String STD_FONT_NAME = "Arial";
/**
* Attribut f?r Cell-Standard-Wert-Font-Size.
*/
public static final int STD_FONT_SIZE = 10;
/**
* Attribut f?r Cell-Standard-Wert-Unterline.
*/
public static final short STD_UNDERLINE = 0;
/**
* Attribut f?r Cell-Standard-Wert-Type-Offset.
*/
public static final short STD_TYPE_OFFSET = 0;
/**
* Attribut f?r Cell-Standard-Wert-Rotation.
*/
public static final short STD_ROTATION = 0;
/**
* Attribut f?r Cell-Standard-Wert-Indent.
*/
public static final short STD_INDENT = 0;
/**
* Attribut f?r Cell-Standard-Wert-Wrap.
*/
public static final boolean STD_WRAP = false;
/**
* Attribut f?r Cell-Standard-Wert-Hidden.
*/
public static final boolean STD_HIDDEN = false;
/**
* Attribut f?r Cell-Standard-Wert-Locked.
*/
public static final boolean STD_LOCKED = true;
//---------------------------------------------------------------------------
// --- color component index into colors array ---
/**
* Index f?r Rot.
*/
public static final int RED = 1;
/**
* Index f?r Gr?n.
*/
public static final int GREEN = 2;
/**
* Index f?r Blau.
*/
public static final int BLUE = 3;
// --- colors array ---
/**
* Feld mit Farbwerten.
*/
public static final short[][] COLORS = {
/*
{ 0, 0, 0, 0 },
{ 1, 255, 255, 255 },
{ 2, 255, 0, 0 },
{ 3, 0, 255, 0 },
{ 4, 0, 0, 255 },
{ 5, 255, 255, 0 },
{ 6, 255, 0, 255 },
{ 7, 0, 255, 255 },
*/
{8, 0, 0, 0}, // !
{9, 255, 255, 255}, // !
{10, 255, 0, 0}, // !
{11, 0, 255, 0}, // !
{12, 0, 0, 255}, // !
{13, 255, 255, 0}, // !
{14, 255, 0, 255}, // !
{15, 0, 255, 255}, // !
{16, 128, 0, 0}, // !
{17, 0, 128, 0}, // !
{18, 0, 0, 128}, // !
{19, 128, 128, 0}, // !
{20, 128, 0, 128}, // !
{21, 0, 128, 128}, // !
{22, 192, 192, 192}, // !
{23, 128, 128, 128}, // !
/*
{ 24, 153, 153, 255 },
{ 25, 153, 51, 102 },
{ 26, 255, 255, 204 },
{ 27, 204, 255, 255 },
{ 28, 102, 102, 102 },
{ 29, 255, 128, 128 },
{ 30, 0, 102, 204 },
{ 31, 204, 204, 255 },
{ 32, 0, 0, 128 },
{ 33, 255, 0, 255 },
{ 34, 255, 255, 0 },
{ 35, 0, 255, 255 },
{ 36, 128, 0, 128 },
{ 37, 128, 0, 0 },
{ 38, 0, 128, 128 },
{ 39, 0, 255, 255 },
*/
{40, 0, 204, 255}, // !
{41, 204, 255, 255}, // !
{42, 204, 255, 204}, // !
{43, 255, 255, 153}, // !
{44, 153, 204, 255}, // !
{45, 255, 153, 204}, // !
{46, 204, 153, 255}, // !
{47, 255, 204, 153}, // !
{48, 51, 102, 255}, // !
{49, 51, 204, 204}, // !
{50, 153, 204, 0}, // !
{51, 255, 204, 0}, // !
{52, 255, 153, 0}, // !
{53, 255, 102, 0}, // !
{54, 102, 102, 153}, // !
{55, 150, 150, 150}, // !
{56, 0, 51, 102}, // !
{57, 51, 153, 102}, // !
{58, 0, 51, 0}, // !
{59, 51, 51, 0}, // !
{60, 153, 51, 0}, // !
{61, 153, 51, 102}, // !
{62, 51, 51, 153}, // !
{63, 51, 51, 51} // !
/*
{ HSSFFont.BLACK, 0, 0, 0 },
{ HSSFFont.BLUE, 0, 0, 255 },
{ HSSFFont.GREEN, 0, 255, 0 },
{ HSSFFont.RED, 255, 0, 0 },
{ HSSFFont.YELLOW, 255, 255, 0 },
{ HSSFFont.WHITE, 255, 255, 255 }
*/
};
/**
* Alignments.
*/
public static final String[] ALIGNMENTS = { "general", "left", "center", "right", "fill", "justify", "centerselection" };
/**
* Verticals.
*/
public static final String[] VERTICALS = { "top", "center", "bottom", "justify" };
/**
* Borders.
*/
public static final String[] BORDERS = { "none", "thin", "medium", "dashed",
"dotted", "thick", "double", "hair",
"mediumdashed", "dashdot", "mediumhashdot", "dashdotdot",
"mediumdashdotdot", "slanteddashdot"
};
/**
* Booleans.
*/
public static final String[] BOOLEANS = { "no", "yes", "0", "1", "off", "on" };
/**
* Zelltypen.
*/
public static final String[] CELL_TYPES = { "numeric", "string", "formula", "blank", "boolean", "error" };
/**
* Font-Weights.
*/
public static final String[] FONT_WEIGHTS = { "normal", "bold" };
/**
* Font-Weight-Werte.
*/
public static final short[] FONT_WEIGHT_VALUES = { HSSFFont.BOLDWEIGHT_NORMAL, HSSFFont.BOLDWEIGHT_BOLD };
/**
* Font-Styles.
*/
public static final String[] FONT_STYLES = { "normal", "italic" };
/**
* Text-Decos.
*/
public static final String[] TEXT_DECOS = { "normal", "line-through" };
/**
* Unterlines.
*/
public static final String[] UNDERLINES = { "normal", "single", "double", "single-accounting", "double-accounting" };
/**
* Underline-Werte.
*/
public static final short[] UNDERLINE_VALUES = { HSSFFont.U_NONE, HSSFFont.U_SINGLE, HSSFFont.U_DOUBLE, HSSFFont.U_SINGLE_ACCOUNTING, HSSFFont.U_DOUBLE_ACCOUNTING };
/**
* Type-Offsets.
*/
public static final String[] TYPE_OFFSETS = { "normal", "super", "sub" };
/**
* Fill-Patterns.
*/
public static final String[] FILL_PATTERNS = {
"no-fill",
"solid-foreground",
"fine-dots",
"alt-bars",
"sparse-dots",
"thick-horizontal-bands",
"thick-vertical-bands",
"thick-backward-diags",
"thick-forward-diags",
"large-spots",
"bricks",
"thin-horizontal-bands",
"thin-vertical-bands",
"thin-backward-diags",
"thin-forward-diags",
"squares",
"diamonds"
};
//===========================================================================
/**
* Ausgabe mit MLogger.deb.
* @param s Text
*/
public static void println(String s)
{
MLogger.deb(s);
}
/**
* Ausgabe mit MLogger.err.
* @param s Text
* @throws SAXException wird immer geworfen
*/
public static void err(String s) throws SAXException
{
MLogger.err("Error: " + s);
throw new SAXException(s);
}
//===========================================================================
/**
* Dummy-Constructor.
*/
protected HSSF()
{
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy