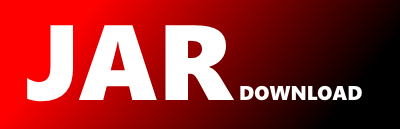
com.sta.cts.hssf.HSSFHandlerXML2XLS Maven / Gradle / Ivy
package com.sta.cts.hssf;
import java.lang.reflect.Method;
import java.io.IOException;
import java.io.FileOutputStream;
import java.util.Hashtable;
import org.xml.sax.XMLReader;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
import org.xml.sax.helpers.XMLReaderFactory;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFFont;
import org.apache.poi.hssf.util.Region;
import com.sta.mlogger.MLogger;
import com.sta.cts.UniTypeConv;
/**
* Name: HSSFHandlerXML2XLS
* Description: Dient dazu eine XML-Datei in eine XLS-Datei zu konvertieren.
*
* Copyright: Copyright (c) 2003, 2016, 2017, 2019
* Company: >StA-Soft<
* @author StA
* @version 1.0
*/
public class HSSFHandlerXML2XLS extends DefaultHandler
{
/**
* Output-Stream.
*/
private FileOutputStream out;
/**
* Status.
*/
private int state = 0;
/**
* Text.
*/
private String text = null;
/**
* HSSF-Workbook.
*/
private HSSFWorkbook wb = null;
/**
* HSSF-Sheet.
*/
private HSSFSheet sheet = null;
/**
* HSSF-Row.
*/
private HSSFRow myRow = null;
/**
* HSSF-Cell.
*/
private HSSFCell myCell = null;
/**
* Font-Beschreibungen.
*/
private Hashtable myFontDescs = null;
/**
* Cell-Style-Beschreibungen.
*/
private Hashtable myCellStyleDescs = null;
/**
* Spaltennummer (f?r Spaltenbreite in "sheet").
*/
private short myColNr;
/**
* Zeilennummer.
*/
private short myRowNr;
/**
* Zellennummer.
*/
private short myCellNr;
/**
* Zeilen-Span.
*/
private int myRowSpan;
/**
* Zellen-Span.
*/
private int myCellSpan;
/**
* Hash-Tabelle f?r Workbook.
*/
private Hashtable htWorkbook = new Hashtable();
/**
* Hash-Tabelle f?r Sheet.
*/
private Hashtable htSheet;
/**
* Hash-Tabelle f?r Zeile.
*/
private Hashtable htRow;
/**
* Hash-Tabelle f?r Zelle.
*/
private Hashtable htCell;
//-------------------------------------------------------------------------
/**
* Attribute in Hash-Tabelle ?bertragen (?berschreibend hinzuf?gen).
* @param ph Hash-Tabelle oder null
* @param a Attribute
* @return Hash-Tabelle
*/
private Hashtable attr2ht(Hashtable ph, Attributes a)
{
int s;
int i;
String n;
String v;
Hashtable h;
if (ph == null)
{
h = new Hashtable();
}
else
{
h = (Hashtable) ph.clone();
}
s = a.getLength();
for (i = 0; i < s; i++)
{
n = a.getQName(i);
v = a.getValue(i);
h.put(n, v);
}
return h;
}
//-------------------------------------------------------------------------
/**
* Es wird gepr?ft, ob in einer Hash-Tabelle (ht) unter einem Schl?ssel (key)
* ein Wert abgelegt ist. Falls nein, wird ein Standardwert (std)
* zur?ckgeliefert. Falls ja, wird der Wert in einem String-Feld (sa) gesucht.
* Wurde der Wert gefunden, wird der Index in das Feld zur?ckgegeben, falls
* nicht, wird eine Exception mit einer Nachricht (msg) samt dem ermittelten
* Wert ausgel?st.
* @param ht die Hash-Tabelle, in der unter key ein Wert gesucht wird
* @param sa das String-Feld, in dem der Wert gesucht wird
* @param key der Schl?ssel f?r ht
* @param std der Standardwert, falls unter key kein Wert in ht vorhanden
* @param msg die Fehlermeldung, wird nur im Fehlerfall benutzt
* @return Index in das String-Feld
* @throws SAXException im Fehlerfall
*/
private short convKey(Hashtable ht, String[] sa, String key, short std, String msg) throws SAXException
{
String v = (String) ht.get(key);
if (v != null)
{
for (short i = 0; i < sa.length; i++)
{
if (v.equals(sa[i]))
{
return i;
}
}
HSSF.err(msg + " (" + v + ").");
}
return std;
}
// std = Standard-Index!
/**
* Schl?ssel konvertieren.
* @param ht Hash-Tabelle
* @param sa das String-Feld, in dem der Wert gesucht wird
* @param va Werte-Feld
* @param key der Schl?ssel f?r ht
* @param std der Standardwert, falls unter key kein Wert in ht vorhanden
* @param msg die Fehlermeldung, wird nur im Fehlerfall benutzt
* @return Index in das String-Feld
* @throws SAXException im Fehlerfall
*/
private short convKey(Hashtable ht, String[] sa, short[] va, String key, short std, String msg) throws SAXException
{
return va[convKey(ht, sa, key, std, msg)];
}
/**
* Farbe konvertieren.
* @param s Farbe
* @return konvertierte Farbe
*/
private short convColor(String s)
{
int color = UniTypeConv.convString2Int(s).intValue();
int r = (color >> 16) & 255;
int g = (color >> 8) & 255;
int b = (color) & 255;
int curIndex = 0;
int curDelta = 0xffffff;
for (int i = 0; i < HSSF.COLORS.length; i++)
{
short[] c = HSSF.COLORS[i];
int d = Math.abs(c[HSSF.RED] - r) + Math.abs(c[HSSF.GREEN] - g) + Math.abs(c[HSSF.BLUE] - b);
if (d <= curDelta)
{
curDelta = d;
curIndex = i;
}
}
return HSSF.COLORS[curIndex][0];
}
//---------------------------------------------------------------------------
/**
* Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param pParamType Parameter-Typ
* @param pParamValue Parameter-Wert
* @throws SAXException im Fehlerfall
*/
private void setOption(Object obj, String pMethodName, Class pParamType, Object pParamValue) throws SAXException
{
try
{
Class c = obj.getClass();
Class[] ca = {pParamType};
Method m = c.getMethod("set" + pMethodName, ca);
Object[] pa = {pParamValue};
m.invoke(obj, pa);
}
catch (Exception e)
{
throw new SAXException(e.getMessage());
}
}
/**
* Boolean-Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @throws SAXException im Fehlerfall
*/
private void setOptionBoolean(Object obj, String pMethodName, Hashtable ht, String pOptionName) throws SAXException
{
short v = convKey(ht, HSSF.BOOLEANS, pOptionName, (short) -1, "Invalid value for @" + pOptionName);
if (v != -1)
{
setOption(obj, pMethodName, Boolean.TYPE, new Boolean(v != 0));
}
}
/**
* Short-Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @throws SAXException im Fehlerfall
*/
private void setOptionShort(Object obj, String pMethodName, Hashtable ht, String pOptionName) throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
setOption(obj, pMethodName, Short.TYPE, new Short(UniTypeConv.convString2Int(s).shortValue()));
}
}
/**
* Integer-Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @throws SAXException im Fehlerfall
*/
private void setOptionInteger(Object obj, String pMethodName, Hashtable ht, String pOptionName) throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
setOption(obj, pMethodName, Integer.TYPE, new Integer(UniTypeConv.convString2Int(s).intValue()));
}
}
/**
* Float-Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @throws SAXException im Fehlerfall
*/
private void setOptionFloat(Object obj, String pMethodName, Hashtable ht, String pOptionName) throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
setOption(obj, pMethodName, Float.TYPE, new Float(UniTypeConv.convString2Float(s).floatValue()));
}
}
/**
* Double-Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @throws SAXException im Fehlerfall
*/
private void setOptionDouble(Object obj, String pMethodName, Hashtable ht, String pOptionName) throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
setOption(obj, pMethodName, Double.TYPE, new Double(UniTypeConv.convString2Double(s).doubleValue()));
}
}
/**
* String-Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @throws SAXException im Fehlerfall
*/
private void setOptionString(Object obj, String pMethodName, Hashtable ht, String pOptionName) throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
setOption(obj, pMethodName, String.class, s);
}
}
/**
* Farb-Option setzen.
* @param obj Objekt
* @param pMethodName Name der Setter-Methode
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @throws SAXException im Fehlerfall
*/
private void setOptionColor(Object obj, String pMethodName, Hashtable ht, String pOptionName) throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
setOption(obj, pMethodName, Short.TYPE, new Short(convColor(s)));
}
}
//---------------------------------------------------------------------------
/**
* Wert einer Short-Option ermitteln.
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @param std Standard-Wert
* @return Wert
*/
private short getOptionShort(Hashtable ht, String pOptionName, short std) // throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
return UniTypeConv.convString2Int(s).shortValue();
}
return std;
}
/**
* Wert einer Integer-Option ermitteln.
* @param ht Hash-Tabelle
* @param pOptionName Optionsname
* @param std Standard-Wert
* @return Wert
*/
private int getOptionInteger(Hashtable ht, String pOptionName, int std) // throws SAXException
{
String s = (String) ht.get(pOptionName);
if (s != null)
{
return UniTypeConv.convString2Int(s).intValue();
}
return std;
}
//---------------------------------------------------------------------------
/**
* Font-Weight konvertieren.
* @param fd Font-Desc
* @throws SAXException im Fehlerfall
*/
private void convFontWeight(FontDesc fd) throws SAXException
{
String s = (String) htCell.get(HSSF.ATTR_FONT_WEIGHT);
if (s != null)
{
fd.setBoldweight(convKey(htCell, HSSF.FONT_WEIGHTS, HSSF.FONT_WEIGHT_VALUES, HSSF.ATTR_FONT_WEIGHT, (short) 0, "Invalid font weight"));
}
}
/**
* Font-Style konvertieren.
* @param fd Font-Desc
* @throws SAXException im Fehlerfall
*/
private void convFontStyle(FontDesc fd) throws SAXException
{
String s = (String) htCell.get(HSSF.ATTR_FONT_STYLE);
if (s != null)
{
fd.setItalic(convKey(htCell, HSSF.FONT_STYLES, HSSF.ATTR_FONT_STYLE, (short) 0, "Invalid font style") != 0);
}
}
/**
* Text-Deco konvertieren.
* @param fd Font-Desc
* @throws SAXException im Fehlerfall
*/
private void convTextDeco(FontDesc fd) throws SAXException
{
String s = (String) htCell.get(HSSF.ATTR_TEXT_DECO);
if (s != null)
{
fd.setStrikeout(convKey(htCell, HSSF.TEXT_DECOS, HSSF.ATTR_TEXT_DECO, (short) 0, "Invalid text decoration") != 0);
}
}
/**
* Underline konvertieren.
* @param fd Font-Desc
* @throws SAXException im Fehlerfall
*/
private void convUnderline(FontDesc fd) throws SAXException
{
String s = (String) htCell.get(HSSF.ATTR_UNDERLINE);
if (s != null)
{
fd.setUnderline(convKey(htCell, HSSF.UNDERLINES, HSSF.UNDERLINE_VALUES, HSSF.ATTR_UNDERLINE, (short) 0, "Invalid underline"));
}
}
/**
* Type-Offset konvertieren.
* @param fd Font-Desc
* @throws SAXException im Fehlerfall
*/
private void convTypeOffset(FontDesc fd) throws SAXException
{
String s = (String) htCell.get(HSSF.ATTR_TYPE_OFFSET);
if (s != null)
{
fd.setTypeOffset(convKey(htCell, HSSF.TYPE_OFFSETS, HSSF.ATTR_TYPE_OFFSET, (short) 0, "Invalid type offset"));
}
}
/**
* Border konvertieren.
* @param key Schl?ssel
* @return Border
* @throws SAXException im Fehlerfall
*/
private short convBorder(String key) throws SAXException
{
return convKey(htCell, HSSF.BORDERS, /* "border" + */ key, HSSFCellStyle.BORDER_NONE, "Invalid border");
}
/**
* Fill-Pattern konvertieren.
* @param csd Cell-Style-Desc
* @throws SAXException im Fehlerfall
*/
private void convFillPattern(CellStyleDesc csd) throws SAXException
{
String s = (String) htCell.get(HSSF.ATTR_FILL_PATTERN);
if (s != null)
{
csd.setFillPattern(convKey(htCell, HSSF.FILL_PATTERNS, HSSF.ATTR_FILL_PATTERN, (short) 0, "Invalid fill pattern"));
}
}
//-------------------------------------------------------------------------
/**
* Row-Span ermitteln.
* @param ht Hash-Tabelle
* @return Row-Span
* @throws SAXException im Fehlerfall
*/
private int getRowSpan(Hashtable ht) throws SAXException
{
int rowspan = getOptionInteger(ht, HSSF.ATTR_ROW_SPAN, 1);
if (rowspan < 1)
{
HSSF.err("Invalid rowspan value (" + rowspan + ").");
}
return rowspan;
}
/**
* Cell-Span ermitteln.
* @param ht Hash-Tabelle
* @return Cell-Span
* @throws SAXException im Fehlerfall
*/
private int getCellSpan(Hashtable ht) throws SAXException
{
int cellspan = getOptionInteger(ht, HSSF.ATTR_CELL_SPAN, 1);
if (cellspan < 1)
{
HSSF.err("Invalid cellspan value (" + cellspan + ").");
}
return cellspan;
}
/**
* Merged-Regions kopieren.
*/
private void copyMergedRegions()
{
try
{
int cnt = sheet.getNumMergedRegions();
for (int i = 0; i < cnt; i++)
{
Region region = sheet.getMergedRegionAt(i);
int za = region.getRowFrom();
int ze = region.getRowTo();
int sa = region.getColumnFrom();
int se = region.getColumnTo();
HSSFCellStyle cs = null;
for (int z = za; z <= ze; z++)
{
HSSFRow row = sheet.getRow((short) z);
if (row == null)
{
row = sheet.createRow((short) z);
}
for (int s = sa; s <= se; s++)
{
HSSFCell cell = row.getCell((short) s);
if (cell == null)
{
cell = row.createCell((short) s);
}
if ((z == za) && (s == sa))
{
cs = cell.getCellStyle();
}
else
{
cell.setCellStyle(cs);
}
}
}
}
}
catch (Exception e)
{
}
}
//-------------------------------------------------------------------------
@Override
public void startDocument() throws SAXException
{
HSSF.println("start...");
// htWorkbook.put("", "");
}
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException
{
switch (state)
{
case 0:
{
if (localName.equals(HSSF.TAG_WORKBOOK))
{
HSSF.println(" create workbook...");
wb = new HSSFWorkbook();
myFontDescs = new Hashtable();
myCellStyleDescs = new Hashtable();
state = 1;
break;
}
}
case 1:
{
if (localName.equals(HSSF.TAG_SHEET))
{
htSheet = attr2ht(htWorkbook, attributes);
String name = (String) htSheet.get(HSSF.ATTR_NAME);
if (name == null)
{
HSSF.err("Missing @name (sheet)");
}
HSSF.println(" create sheet (" + name + ")...");
sheet = wb.createSheet(name);
setOptionBoolean(sheet, "AlternativeExpression", htSheet, HSSF.ATTR_ALTERNATIVE_EXPRESSION);
setOptionBoolean(sheet, "AlternativeFormula", htSheet, HSSF.ATTR_ALTERNATIVE_FORMULA);
setOptionBoolean(sheet, "Autobreaks", htSheet, HSSF.ATTR_AUTO_BREAKS);
setOptionShort(sheet, "DefaultColumnWidth", htSheet, HSSF.ATTR_DEFAULT_COLUMN_WIDTH);
setOptionFloat(sheet, "DefaultRowHeightInPoints", htSheet, HSSF.ATTR_DEFAULT_ROW_HEIGHT);
setOptionBoolean(sheet, "Dialog", htSheet, HSSF.ATTR_DIALOG);
setOptionBoolean(sheet, "DisplayGuts", htSheet, HSSF.ATTR_DISPLAY_GUTS);
setOptionBoolean(sheet, "FitToPage", htSheet, HSSF.ATTR_FIT_TO_PAGE);
setOptionBoolean(sheet, "GridsPrinted", htSheet, HSSF.ATTR_GRIDS_PRINTED);
setOptionBoolean(sheet, "RowSumsBelow", htSheet, HSSF.ATTR_ROW_SUMS_BELOW);
setOptionBoolean(sheet, "RowSumsRight", htSheet, HSSF.ATTR_ROW_SUMS_RIGHT);
setOptionBoolean(sheet, "VerticallyCenter", htSheet, HSSF.ATTR_VERTICALLY_CENTER);
myRowNr = getOptionShort(htSheet, HSSF.ATTR_EMPTY_ROWS, (short) 0);
myColNr = 0;
state = 2;
break;
}
}
case 2:
{
if (localName.equals(HSSF.TAG_COLUMN))
{
Hashtable htCol = attr2ht(htSheet, attributes);
String s;
s = (String) htCol.get(HSSF.ATTR_COLUMN_WIDTH);
if (s != null)
{
sheet.setColumnWidth(myColNr, (short) (UniTypeConv.convString2Int(s).intValue() * 256));
}
myColNr++;
state = 20;
break;
}
if (localName.equals(HSSF.TAG_ROW))
{
htRow = attr2ht(htSheet, attributes);
// HSSF.println(" create row...");
String s;
// rowspan
myRowSpan = getRowSpan(htRow);
// the row itself
myRow = sheet.getRow(myRowNr);
if (myRow == null)
{
myRow = sheet.createRow(myRowNr);
}
setOptionFloat(myRow, "HeightInPoints", htRow, HSSF.ATTR_ROW_HEIGHT);
myRowNr++;
myCellNr = getOptionShort(htRow, HSSF.ATTR_EMPTY_CELLS, (short) 0);
state = 3;
break;
}
}
case 3:
{
if (localName.equals(HSSF.TAG_CELL))
{
htCell = attr2ht(htRow, attributes);
// HSSF.println(" create cell...");
CellStyleDesc csd = new CellStyleDesc();
// font: size and (forground) color
FontDesc fd = new FontDesc();
String s;
setOptionString(fd, "FontName", htCell, HSSF.ATTR_FONT_NAME);
setOptionShort(fd, "FontHeightInPoints", htCell, HSSF.ATTR_FONT_SIZE);
setOptionColor(fd, "Color", htCell, HSSF.ATTR_COLOR);
setOptionShort(fd, "Color", htCell, HSSF.ATTR_COLOR_INDEX);
convFontWeight(fd);
convFontStyle(fd);
convTextDeco(fd);
convUnderline(fd);
convTypeOffset(fd);
// setFont...
HSSFFont fx = (HSSFFont) myFontDescs.get(fd);
if (fx == null)
{
fx = wb.createFont();
fd.fillFont(fx);
myFontDescs.put(fd, fx);
}
csd.setFont(fx);
// rotation
setOptionShort(csd, "Rotation", htCell, HSSF.ATTR_ROTATION);
// indent
setOptionShort(csd, "Indention", htCell, HSSF.ATTR_INDENT);
// fill background color
setOptionColor(csd, "FillBackgroundColor", htCell, HSSF.ATTR_FILL_BACKGROUND_COLOR);
setOptionColor(csd, "FillForegroundColor", htCell, HSSF.ATTR_FILL_FOREGROUND_COLOR);
convFillPattern(csd);
// background color
s = (String) htCell.get(HSSF.ATTR_BG_COLOR);
if (s != null)
{
setOptionColor(csd, "FillForegroundColor", htCell, HSSF.ATTR_BG_COLOR);
csd.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND);
}
// borders
csd.setBorderBottom(convBorder(HSSF.ATTR_BORDER_BOTTOM));
csd.setBorderLeft(convBorder(HSSF.ATTR_BORDER_LEFT));
csd.setBorderRight(convBorder(HSSF.ATTR_BORDER_RIGHT));
csd.setBorderTop(convBorder(HSSF.ATTR_BORDER_TOP));
setOptionColor(csd, "BottomBorderColor", htCell, HSSF.ATTR_BORDER_BOTTOM_COLOR);
setOptionColor(csd, "LeftBorderColor", htCell, HSSF.ATTR_BORDER_LEFT_COLOR);
setOptionColor(csd, "RightBorderColor", htCell, HSSF.ATTR_BORDER_RIGHT_COLOR);
setOptionColor(csd, "TopBorderColor", htCell, HSSF.ATTR_BORDER_TOP_COLOR);
// alignments
csd.setAlignment(convKey(htCell, HSSF.ALIGNMENTS, HSSF.ATTR_ALIGN, HSSFCellStyle.ALIGN_GENERAL, "Invalid alignment"));
csd.setVerticalAlignment(convKey(htCell, HSSF.VERTICALS, HSSF.ATTR_V_ALIGN, HSSFCellStyle.VERTICAL_TOP, "Invalid vertical alignment"));
setOptionBoolean(csd, "WrapText", htCell, HSSF.ATTR_WRAP);
// hidden, locked
setOptionBoolean(csd, "Locked", htCell, HSSF.ATTR_LOCKED);
setOptionBoolean(csd, "Hidden", htCell, HSSF.ATTR_HIDDEN);
// the cell itself
myCell = myRow.createCell(myCellNr);
// cell type (f?r sp?tere Erweiterungen, sobald Formeln unterst?tzt werden)
/*
s = (String) htCell.get("cell-type");
if (s != null)
{
cell.setCellType(convKey(celltypes, "myCell-type", (short) 1, "Invalid myCell type"));
}
*/
// cell style
HSSFCellStyle csx = (HSSFCellStyle) myCellStyleDescs.get(csd);
if (csx == null)
{
csx = wb.createCellStyle();
csd.fillCellStyle(csx);
myCellStyleDescs.put(csd, csx);
}
myCell.setCellStyle(csx);
myCellNr++;
// cellspan
myCellSpan = getCellSpan(htCell);
int rowspan = getRowSpan(htCell); // kann auch an der Zelle angegeben werden
if ((myCellSpan > 1) || (rowspan > 1))
{
sheet.addMergedRegion(new Region(myRowNr - 1, (short) (myCellNr - 1), myRowNr + rowspan - 2, (short) (myCellNr + myCellSpan - 2)));
}
text = "";
state = 4;
break;
}
}
default:
{
HSSF.err("Error: Invalid state (" + state + ") or invalid element (<" + localName + ">).");
}
}
}
@Override
public void characters(char[] ch, int start, int length) throws SAXException
{
String s = new String(ch, start, length);
switch (state)
{
case 4:
{
text = text + s;
break;
}
default:
{
if (!s.trim().equals(""))
{
HSSF.err("Error: Invalid state (" + state + ") or invalid text position (" + s + ").");
}
}
}
}
@Override
public void endElement(String uri, String localName, String qName) throws SAXException
{
switch (state)
{
case 4:
{
if (localName.equals(HSSF.TAG_CELL))
{
String s = text.trim();
String ct = (String) htCell.get(HSSF.ATTR_CELL_TYPE);
if (ct != null)
{
if (ct.equalsIgnoreCase("String"))
{
myCell.setCellValue(s);
}
else if (ct.equalsIgnoreCase("Int") || ct.equalsIgnoreCase("Integer"))
{
myCell.setCellValue(UniTypeConv.convString2Int(s).intValue());
}
else if (ct.equalsIgnoreCase("Real") || ct.equalsIgnoreCase("Double") || ct.equalsIgnoreCase("Numeric"))
{
myCell.setCellValue(UniTypeConv.convString2Double(s).doubleValue());
}
else if (ct.equalsIgnoreCase("Date"))
{
myCell.setCellValue(UniTypeConv.convString2Date(s, null));
}
else if (ct.equalsIgnoreCase("Time"))
{
myCell.setCellValue(UniTypeConv.convString2Time(s, null));
}
else if (ct.equalsIgnoreCase("DateTime"))
{
myCell.setCellValue(UniTypeConv.convString2DateTime(s, null));
}
else if (ct.equalsIgnoreCase("Boolean") || s.equalsIgnoreCase("Bool"))
{
myCell.setCellValue(UniTypeConv.convString2Bool(s).booleanValue());
}
else
{
myCell.setCellValue(s);
}
}
else
{
myCell.setCellValue(s);
}
// HSSF.println(" text = " + tt);
// HSSF.println(" close cell.");
// cellspan
// int rowspan = getRowSpan(htCell);
// if ((cellspan > 1) || (rowspan > 1))
if (myCellSpan > 1)
{
// F?r ?berspannte Zellen wird normalerweise kein createCell(...) ben?tigt.
// Bei Einsatz von addMergedRegion schadet es aber auch nicht.
/*
HSSFCellStyle cs = cell.getCellStyle();
for (int i = 1; i < cellspan; i++)
{
cell = row.createCell((short) (myCellNr + i - 1));
cell.setCellStyle(cs);
}
for (int j = 1; j < rowspan; j++)
{
HSSFRow row = sheet.getRow(myRowNr + j - 1);
if (row == null)
{
row = sheet.createRow((short) (myRowNr + j - 1));
}
for (int i = 0; i < cellspan; i++)
{
cell = row.createCell((short) (myCellNr + i - 1));
cell.setCellStyle(cs);
}
}
*/
myCellNr += myCellSpan - 1;
}
text = null;
state = 3;
break;
}
}
case 3:
{
if (localName.equals(HSSF.TAG_ROW))
{
// HSSF.println(" close row.");
// rowspan
if (myRowSpan > 1)
{
// F?r ?berspannte Zeilen wird kein createRow(...) ben?tigt.
myRowNr += myRowSpan - 1;
}
state = 2;
break;
}
}
case 20:
{
if (localName.equals(HSSF.TAG_COLUMN))
{
state = 2;
break;
}
}
case 2:
{
if (localName.equals(HSSF.TAG_SHEET))
{
HSSF.println(" close sheet.");
// copy merged regions
copyMergedRegions();
state = 1;
break;
}
}
case 1:
{
if (localName.equals(HSSF.TAG_WORKBOOK))
{
HSSF.println(" close workbook.");
HSSF.println("Fonts = " + wb.getNumberOfFonts());
HSSF.println("CellStyles = " + wb.getNumCellStyles());
state = 0;
break;
}
}
default:
{
HSSF.err("Error: Invalid state (" + state + ") or invalid element (" + localName + ">).");
}
}
}
@Override
public void endDocument() throws SAXException
{
try
{
wb.write(out);
}
catch (IOException e)
{
HSSF.err(e.getMessage());
}
HSSF.println("end...");
}
//===========================================================================
/**
* Zentraler Einstiegspunkt.
* @param pSrcFileName Quelldateiname
* @param pDstFileName Zieldateiname
*/
public static void main(String pSrcFileName, String pDstFileName)
{
try
{
XMLReader parser = XMLReaderFactory.createXMLReader("org.apache.xerces.parsers.SAXParser");
HSSFHandlerXML2XLS aHSSFHandlerInstance = new HSSFHandlerXML2XLS();
aHSSFHandlerInstance.out = new FileOutputStream(pDstFileName);
parser.setContentHandler(aHSSFHandlerInstance);
parser.parse(pSrcFileName);
aHSSFHandlerInstance.out.close();
}
catch (IOException ioe)
{
MLogger.err("", ioe);
}
catch (SAXException saxe)
{
MLogger.err("", saxe);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy