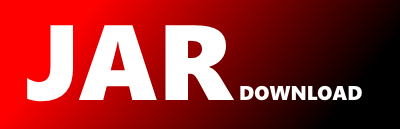
org.fxmisc.wellbehaved.event.EventPattern Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of richtextfx Show documentation
Show all versions of richtextfx Show documentation
FX-Text-Area for formatted text and other special effects.
package org.fxmisc.wellbehaved.event;
import static javafx.scene.input.KeyEvent.*;
import static javafx.scene.input.MouseEvent.*;
import java.util.Collections;
import java.util.HashSet;
import java.util.Optional;
import java.util.Set;
import java.util.function.Predicate;
import javafx.event.Event;
import javafx.event.EventType;
import javafx.scene.input.KeyCharacterCombination;
import javafx.scene.input.KeyCode;
import javafx.scene.input.KeyCodeCombination;
import javafx.scene.input.KeyCombination;
import javafx.scene.input.KeyEvent;
import javafx.scene.input.MouseButton;
import javafx.scene.input.MouseEvent;
public interface EventPattern {
Optional extends U> match(T event);
Set> getEventTypes();
default EventPattern andThen(EventPattern super U, V> next) {
return new EventPattern() {
@Override
public Optional extends V> match(T event) {
return EventPattern.this.match(event).flatMap(next::match);
}
@Override
public Set> getEventTypes() {
return next.getEventTypes();
}
};
}
default EventPattern onlyIf(Predicate super U> condition) {
return new EventPattern() {
@Override
public Optional match(T event) {
return EventPattern.this.match(event).map(u -> condition.test(u) ? u : null);
}
@Override
public Set> getEventTypes() {
return EventPattern.this.getEventTypes();
}
};
}
default EventPattern unless(Predicate super U> condition) {
return onlyIf(condition.negate());
}
@SafeVarargs
static EventPattern anyOf(EventPattern... events) {
return new EventPattern() {
@Override
public Optional extends U> match(T event) {
for (EventPattern evt : events) {
Optional extends U> match = evt.match(event);
if(match.isPresent()) {
return match;
}
}
return Optional.empty();
}
@Override
public Set> getEventTypes() {
HashSet> ret = new HashSet<>();
for (EventPattern evt : events) {
ret.addAll(evt.getEventTypes());
}
return ret;
}
};
}
static EventPattern eventType(EventType extends T> eventType) {
return new EventPattern() {
@Override
public Optional match(Event event) {
EventType extends Event> actualType = event.getEventType();
do {
if(actualType.equals(eventType)) {
@SuppressWarnings("unchecked")
T res = (T) event;
return Optional.of(res);
}
actualType = actualType.getSuperType();
} while(actualType != null);
return Optional.empty();
}
@Override
public Set> getEventTypes() {
return Collections.singleton(eventType);
}
};
}
static EventPattern keyPressed() {
return eventType(KeyEvent.KEY_PRESSED);
}
static EventPattern keyPressed(KeyCombination combination) {
return keyPressed().onlyIf(combination::match);
}
static EventPattern keyPressed(KeyCode code, KeyCombination.Modifier... modifiers) {
return keyPressed(new KeyCodeCombination(code, modifiers));
}
static EventPattern keyPressed(Predicate keyTest, KeyCombination.Modifier... modifiers) {
return keyPressed(new KeyCodePatternCombination(keyTest, modifiers));
}
static EventPattern keyPressed(String character, KeyCombination.Modifier... modifiers) {
return keyPressed(new KeyCharacterCombination(character, modifiers));
}
static EventPattern keyReleased() {
return eventType(KEY_RELEASED);
}
static EventPattern keyReleased(KeyCombination combination) {
return keyReleased().onlyIf(combination::match);
}
static EventPattern keyReleased(KeyCode code, KeyCombination.Modifier... modifiers) {
return keyReleased(new KeyCodeCombination(code, modifiers));
}
static EventPattern keyReleased(Predicate keyTest, KeyCombination.Modifier... modifiers) {
return keyReleased(new KeyCodePatternCombination(keyTest, modifiers));
}
static EventPattern keyReleased(String character, KeyCombination.Modifier... modifiers) {
return keyReleased(new KeyCharacterCombination(character, modifiers));
}
static EventPattern keyTyped() {
return eventType(KEY_TYPED);
}
static EventPattern keyTyped(Predicate charTest, KeyCombination.Modifier... modifiers) {
KeyTypedCombination combination = new KeyTypedCombination(charTest, modifiers);
return keyTyped().onlyIf(combination::match);
}
static EventPattern keyTyped(String character, KeyCombination.Modifier... modifiers) {
return keyTyped(character::equals, modifiers);
}
static EventPattern mouseClicked() {
return eventType(MOUSE_CLICKED);
}
static EventPattern mouseClicked(MouseButton button) {
return mouseClicked().onlyIf(e -> e.getButton() == button);
}
static EventPattern mousePressed() {
return eventType(MOUSE_PRESSED);
}
static EventPattern mousePressed(MouseButton button) {
return mousePressed().onlyIf(e -> e.getButton() == button);
}
static EventPattern mouseReleased() {
return eventType(MOUSE_RELEASED);
}
static EventPattern mouseReleased(MouseButton button) {
return mouseReleased().onlyIf(e -> e.getButton() == button);
}
static EventPattern mouseMoved() {
return eventType(MOUSE_MOVED);
}
static EventPattern mouseDragged() {
return eventType(MOUSE_DRAGGED);
}
static EventPattern dragDetected() {
return eventType(DRAG_DETECTED);
}
static EventPattern mouseEntered() {
return eventType(MOUSE_ENTERED);
}
static EventPattern mouseEnteredTarget() {
return eventType(MOUSE_ENTERED_TARGET);
}
static EventPattern mouseExited() {
return eventType(MOUSE_EXITED);
}
static EventPattern mouseExitedTarget() {
return eventType(MOUSE_EXITED_TARGET);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy