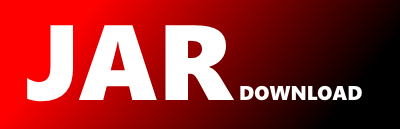
org.fxmisc.wellbehaved.event.Nodes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of richtextfx Show documentation
Show all versions of richtextfx Show documentation
FX-Text-Area for formatted text and other special effects.
package org.fxmisc.wellbehaved.event;
import java.util.AbstractMap.SimpleEntry;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import javafx.collections.MapChangeListener;
import javafx.event.Event;
import javafx.event.EventHandler;
import javafx.event.EventType;
import javafx.scene.Node;
import org.fxmisc.wellbehaved.event.InputMap.HandlerConsumer;
public class Nodes {
private static final String P_INPUTMAP = "org.fxmisc.wellbehaved.event.inputmap";
private static final String P_HANDLERS = "org.fxmisc.wellbehaved.event.handlers";
public static void addInputMap(Node node, InputMap> im) {
init(node);
setInputMap(node, InputMap.sequence(im, getInputMap(node)));
}
public static void addFallbackInputMap(Node node, InputMap> im) {
init(node);
setInputMap(node, InputMap.sequence(getInputMap(node), im));
}
public static void removeInputMap(Node node, InputMap> im) {
setInputMap(node, getInputMap(node).without(im));
}
static InputMap> getInputMap(Node node) {
init(node);
return (InputMap>) node.getProperties().get(P_INPUTMAP);
}
private static void setInputMap(Node node, InputMap> im) {
node.getProperties().put(P_INPUTMAP, im);
}
private static void init(Node node) {
if(node.getProperties().get(P_INPUTMAP) == null) {
node.getProperties().put(P_INPUTMAP, InputMap.empty());
node.getProperties().put(P_HANDLERS, new ArrayList>());
MapChangeListener
© 2015 - 2025 Weber Informatics LLC | Privacy Policy