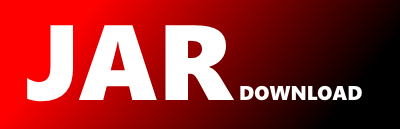
org.fxmisc.wellbehaved.event.template.InputMapTemplate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of richtextfx Show documentation
Show all versions of richtextfx Show documentation
FX-Text-Area for formatted text and other special effects.
package org.fxmisc.wellbehaved.event.template;
import java.util.Arrays;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Function;
import java.util.function.Predicate;
import javafx.event.Event;
import javafx.event.EventType;
import javafx.scene.Node;
import org.fxmisc.wellbehaved.event.EventPattern;
import org.fxmisc.wellbehaved.event.InputHandler;
import org.fxmisc.wellbehaved.event.InputHandler.Result;
import org.fxmisc.wellbehaved.event.InputMap;
import org.fxmisc.wellbehaved.event.Nodes;
public abstract class InputMapTemplate {
@FunctionalInterface
public static interface HandlerTemplateConsumer {
void accept(
EventType extends F> t,
InputHandlerTemplate h);
static HandlerTemplateConsumer from(
InputMap.HandlerConsumer hc, S target) {
return new HandlerTemplateConsumer() {
@Override
public void accept(
EventType extends F> t, InputHandlerTemplate h) {
hc.accept(t, evt -> h.process(target, evt));
}
};
}
}
private InputHandlerTemplateMap inputHandlerTemplates = null;
public final void forEachEventType(HandlerTemplateConsumer f) {
if(inputHandlerTemplates == null) {
inputHandlerTemplates = getInputHandlerTemplateMap();
}
inputHandlerTemplates.forEach(f);
}
public final InputMapTemplate orElse(InputMapTemplate that) {
return sequence(this, that);
}
public final InputMap instantiate(S target) {
return new InputMapTemplateInstance<>(this, target);
}
protected abstract InputHandlerTemplateMap getInputHandlerTemplateMap();
static InputMapTemplate upCast(InputMapTemplate imt) {
@SuppressWarnings("unchecked")
InputMapTemplate res = (InputMapTemplate) imt;
return res;
}
@SafeVarargs
public static InputMapTemplate sequence(InputMapTemplate... templates) {
return new TemplateChain<>(templates);
}
public static InputMapTemplate process(
EventPattern super T, ? extends U> eventPattern,
BiFunction super S, ? super U, InputHandler.Result> action) {
return new PatternActionTemplate<>(eventPattern, action);
}
public static InputMapTemplate process(
EventType extends T> eventType,
BiFunction super S, ? super T, InputHandler.Result> action) {
return process(EventPattern.eventType(eventType), action);
}
public InputMapTemplate ifConsumed(BiConsumer super S, ? super E> postConsumption) {
return new InputMapTemplate() {
@Override
protected InputHandlerTemplateMap getInputHandlerTemplateMap() {
return InputMapTemplate.this.getInputHandlerTemplateMap().map(iht -> {
return (s, evt) -> {
Result res = iht.process(s, evt);
if (res == Result.CONSUME) {
postConsumption.accept(s, evt);
}
return res;
};
});
}
};
}
public static InputMapTemplate consume(
EventPattern super T, ? extends U> eventPattern,
BiConsumer super S, ? super U> action) {
return process(eventPattern, (s, u) -> {
action.accept(s, u);
return Result.CONSUME;
});
}
public static InputMapTemplate consume(
EventType extends T> eventType,
BiConsumer super S, ? super T> action) {
return consume(EventPattern.eventType(eventType), action);
}
public static InputMapTemplate consume(
EventPattern super T, ? extends U> eventPattern) {
return process(eventPattern, (s, u) -> Result.CONSUME);
}
public static InputMapTemplate consume(
EventType extends T> eventType) {
return consume(EventPattern.eventType(eventType));
}
public static InputMapTemplate consumeWhen(
EventPattern super T, ? extends U> eventPattern,
Predicate super S> condition,
BiConsumer super S, ? super U> action) {
return process(eventPattern, (s, u) -> {
if(condition.test(s)) {
action.accept(s, u);
return Result.CONSUME;
} else {
return Result.PROCEED;
}
});
}
public static InputMapTemplate consumeWhen(
EventType extends T> eventType,
Predicate super S> condition,
BiConsumer super S, ? super T> action) {
return consumeWhen(EventPattern.eventType(eventType), condition, action);
}
public static InputMapTemplate consumeUnless(
EventPattern super T, ? extends U> eventPattern,
Predicate super S> condition,
BiConsumer super S, ? super U> action) {
return consumeWhen(eventPattern, condition.negate(), action);
}
public static InputMapTemplate consumeUnless(
EventType extends T> eventType,
Predicate super S> condition,
BiConsumer super S, ? super T> action) {
return consumeUnless(EventPattern.eventType(eventType), condition, action);
}
public static InputMapTemplate ignore(
EventPattern super T, ? extends U> eventPattern) {
return new PatternActionTemplate<>(eventPattern, PatternActionTemplate.CONST_IGNORE);
}
public static InputMapTemplate ignore(
EventType extends T> eventType) {
return ignore(EventPattern.eventType(eventType));
}
public static InputMapTemplate when(
Predicate super S> condition, InputMapTemplate imt) {
return new InputMapTemplate() {
@Override
protected InputHandlerTemplateMap getInputHandlerTemplateMap() {
return imt.getInputHandlerTemplateMap().map(
h -> (s, evt) -> condition.test(s) ? h.process(s, evt) : Result.PROCEED);
}
};
}
public static InputMapTemplate unless(
Predicate super S> condition, InputMapTemplate imt) {
return when(condition.negate(), imt);
}
public static InputMapTemplate lift(
InputMapTemplate imt,
Function super S, ? extends T> f) {
return new InputMapTemplate() {
@Override
protected InputHandlerTemplateMap getInputHandlerTemplateMap() {
return imt.getInputHandlerTemplateMap().map(
h -> (s, evt) -> h.process(f.apply(s), evt));
}
};
}
public static void installOverride(InputMapTemplate imt, S node) {
Nodes.addInputMap(node, imt.instantiate(node));
}
public static void installOverride(InputMapTemplate imt, S target, Function super S, ? extends N> getNode) {
Nodes.addInputMap(getNode.apply(target), imt.instantiate(target));
}
public static void installFallback(InputMapTemplate imt, S node) {
Nodes.addFallbackInputMap(node, imt.instantiate(node));
}
public static void installFallback(InputMapTemplate imt, S target, Function super S, ? extends N> getNode) {
Nodes.addFallbackInputMap(getNode.apply(target), imt.instantiate(target));
}
public static void uninstall(InputMapTemplate imt, S node) {
Nodes.removeInputMap(node, imt.instantiate(node));
}
public static void uninstall(InputMapTemplate imt, S target, Function super S, ? extends N> getNode) {
Nodes.removeInputMap(getNode.apply(target), imt.instantiate(target));
}
}
class PatternActionTemplate extends InputMapTemplate {
static final BiFunction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy