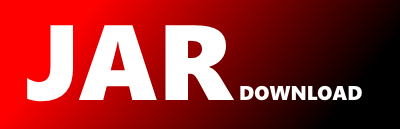
org.reactfx.collection.ProperLiveList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of richtextfx Show documentation
Show all versions of richtextfx Show documentation
FX-Text-Area for formatted text and other special effects.
package org.reactfx.collection;
import java.util.Collections;
import java.util.List;
import org.reactfx.ObservableBase;
import org.reactfx.ProperObservable;
import org.reactfx.util.Lists;
import org.reactfx.util.NotificationAccumulator;
/**
* Trait to be mixed into {@link ObservableBase} to obtain default
* implementation of some {@link LiveList} methods and get additional
* helper methods for implementations of proper {@linkplain LiveList}.
*/
public interface ProperLiveList
extends LiveList, ProperObservable, QuasiListChange extends E>> {
@Override
default NotificationAccumulator, QuasiListChange extends E>, ?> defaultNotificationAccumulator() {
return NotificationAccumulator.listNotifications();
}
default void fireModification(QuasiListModification extends E> mod) {
notifyObservers(mod.asListChange());
}
static QuasiListModification elemReplacement(int index, E replaced) {
return new QuasiListModificationImpl(
index, Collections.singletonList(replaced), 1);
}
default void fireElemReplacement(int index, E replaced) {
fireModification(elemReplacement(index, replaced));
}
default QuasiListModification contentReplacement(List removed) {
return new QuasiListModificationImpl(0, removed, size());
}
default void fireContentReplacement(List removed) {
fireModification(contentReplacement(removed));
}
static QuasiListModification elemInsertion(int index) {
return rangeInsertion(index, 1);
}
default void fireElemInsertion(int index) {
fireModification(elemInsertion(index));
}
static QuasiListModification rangeInsertion(int index, int size) {
return new QuasiListModificationImpl(
index, Collections.emptyList(), size);
}
default void fireRangeInsertion(int index, int size) {
fireModification(rangeInsertion(index, size));
}
static QuasiListModification elemRemoval(int index, E removed) {
return new QuasiListModificationImpl(
index, Collections.singletonList(removed), 0);
}
default void fireElemRemoval(int index, E removed) {
fireModification(elemRemoval(index, removed));
}
static QuasiListModification rangeRemoval(int index, List removed) {
return new QuasiListModificationImpl(index, removed, 0);
}
default void fireRemoveRange(int index, List removed) {
fireModification(rangeRemoval(index, removed));
}
@Override
default int defaultHashCode() {
return Lists.hashCode(this);
}
@Override
default boolean defaultEquals(Object o) {
return Lists.equals(this, o);
}
@Override
default String defaultToString() {
return Lists.toString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy