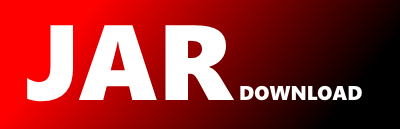
org.reactfx.collection.QuasiListModification Maven / Gradle / Ivy
package org.reactfx.collection;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import javafx.collections.ListChangeListener.Change;
import javafx.collections.ObservableList;
public interface QuasiListModification extends ListModificationLike {
static QuasiListModification create(
int position,
List extends E> removed,
int addedSize) {
return new QuasiListModificationImpl<>(position, removed, addedSize);
}
static QuasiListModification fromCurrentStateOf(
Change ch) {
List list = ch.getList();
int from = ch.getFrom();
int addedSize = ch.getTo() - from; // use (to - from), because
// ch.getAddedSize() is 0 on permutation
List removed;
if(ch.wasPermutated()) {
removed = new ArrayList<>(addedSize);
for(int i = 0; i < addedSize; ++i) {
int pi = ch.getPermutation(from + i);
removed.add(list.get(pi));
}
} else {
removed = ch.getRemoved();
}
return new QuasiListModificationImpl<>(from, removed, addedSize);
}
static ListModification instantiate(
QuasiListModification extends E> template,
ObservableList list) {
return new ListModificationImpl<>(
template.getFrom(),
template.getRemoved(),
template.getAddedSize(),
list);
}
static MaterializedListModification materialize(
QuasiListModification extends E> template,
ObservableList list) {
return MaterializedListModification.create(
template.getFrom(),
template.getRemoved(),
new ArrayList<>(list.subList(template.getFrom(), template.getTo())));
}
default ListModification instantiate(ObservableList list) {
return instantiate(this, list);
}
default MaterializedListModification materialize(ObservableList list) {
return materialize(this, list);
}
default QuasiListChange asListChange() {
return () -> Collections.singletonList(this);
}
}
class QuasiListModificationImpl implements QuasiListModification {
private final int position;
private final List extends E> removed;
private final int addedSize;
QuasiListModificationImpl(
int position,
List extends E> removed,
int addedSize) {
this.position = position;
this.removed = Collections.unmodifiableList(removed);
this.addedSize = addedSize;
}
@Override
public int getFrom() {
return position;
}
@Override
public int getAddedSize() {
return addedSize;
}
@Override
public List extends E> getRemoved() {
return removed;
}
@Override
public String toString() {
return "[modification at: " + getFrom() +
", removed: " + getRemoved() +
", added size: " + getAddedSize() + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy