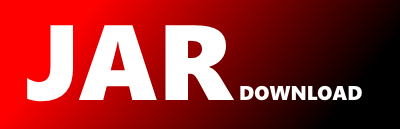
com.stackify.api.common.proto.StackifyProto Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: stackify-agent.proto
package com.stackify.api.common.proto;
public final class StackifyProto {
private StackifyProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface LogGroupOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup)
com.google.protobuf.MessageOrBuilder {
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
java.lang.String getEnvironment();
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
com.google.protobuf.ByteString
getEnvironmentBytes();
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
java.lang.String getServerName();
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
com.google.protobuf.ByteString
getServerNameBytes();
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
java.lang.String getApplicationName();
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
com.google.protobuf.ByteString
getApplicationNameBytes();
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
java.lang.String getApplicationLocation();
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
com.google.protobuf.ByteString
getApplicationLocationBytes();
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
java.lang.String getLogger();
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
com.google.protobuf.ByteString
getLoggerBytes();
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
java.lang.String getPlatform();
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
com.google.protobuf.ByteString
getPlatformBytes();
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
java.util.List
getLogsList();
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log getLogs(int index);
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
int getLogsCount();
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
java.util.List extends com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder>
getLogsOrBuilderList();
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder getLogsOrBuilder(
int index);
/**
* .stackify.LogGroup.Container container = 8;
*/
boolean hasContainer();
/**
* .stackify.LogGroup.Container container = 8;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Container getContainer();
/**
* .stackify.LogGroup.Container container = 8;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.ContainerOrBuilder getContainerOrBuilder();
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
boolean hasKubernetes();
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes getKubernetes();
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.KubernetesOrBuilder getKubernetesOrBuilder();
}
/**
* Protobuf type {@code stackify.LogGroup}
*/
public static final class LogGroup extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup)
LogGroupOrBuilder {
private static final long serialVersionUID = 0L;
// Use LogGroup.newBuilder() to construct.
private LogGroup(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LogGroup() {
environment_ = "";
serverName_ = "";
applicationName_ = "";
applicationLocation_ = "";
logger_ = "";
platform_ = "";
logs_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LogGroup();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LogGroup(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
environment_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
serverName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
applicationName_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
applicationLocation_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
logger_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
platform_ = s;
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
logs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
logs_.add(
input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.parser(), extensionRegistry));
break;
}
case 66: {
com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder subBuilder = null;
if (container_ != null) {
subBuilder = container_.toBuilder();
}
container_ = input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Container.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(container_);
container_ = subBuilder.buildPartial();
}
break;
}
case 74: {
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder subBuilder = null;
if (kubernetes_ != null) {
subBuilder = kubernetes_.toBuilder();
}
kubernetes_ = input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(kubernetes_);
kubernetes_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Builder.class);
}
public interface ContainerOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Container)
com.google.protobuf.MessageOrBuilder {
/**
* string image_id = 1;
*/
java.lang.String getImageId();
/**
* string image_id = 1;
*/
com.google.protobuf.ByteString
getImageIdBytes();
/**
* string image_repository = 2;
*/
java.lang.String getImageRepository();
/**
* string image_repository = 2;
*/
com.google.protobuf.ByteString
getImageRepositoryBytes();
/**
* string image_tag = 3;
*/
java.lang.String getImageTag();
/**
* string image_tag = 3;
*/
com.google.protobuf.ByteString
getImageTagBytes();
/**
* string container_id = 4;
*/
java.lang.String getContainerId();
/**
* string container_id = 4;
*/
com.google.protobuf.ByteString
getContainerIdBytes();
/**
* string container_name = 5;
*/
java.lang.String getContainerName();
/**
* string container_name = 5;
*/
com.google.protobuf.ByteString
getContainerNameBytes();
}
/**
* Protobuf type {@code stackify.LogGroup.Container}
*/
public static final class Container extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Container)
ContainerOrBuilder {
private static final long serialVersionUID = 0L;
// Use Container.newBuilder() to construct.
private Container(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Container() {
imageId_ = "";
imageRepository_ = "";
imageTag_ = "";
containerId_ = "";
containerName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Container();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Container(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
imageId_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
imageRepository_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
imageTag_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
containerId_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
containerName_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Container_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Container_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Container.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder.class);
}
public static final int IMAGE_ID_FIELD_NUMBER = 1;
private volatile java.lang.Object imageId_;
/**
* string image_id = 1;
*/
public java.lang.String getImageId() {
java.lang.Object ref = imageId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageId_ = s;
return s;
}
}
/**
* string image_id = 1;
*/
public com.google.protobuf.ByteString
getImageIdBytes() {
java.lang.Object ref = imageId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IMAGE_REPOSITORY_FIELD_NUMBER = 2;
private volatile java.lang.Object imageRepository_;
/**
* string image_repository = 2;
*/
public java.lang.String getImageRepository() {
java.lang.Object ref = imageRepository_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageRepository_ = s;
return s;
}
}
/**
* string image_repository = 2;
*/
public com.google.protobuf.ByteString
getImageRepositoryBytes() {
java.lang.Object ref = imageRepository_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IMAGE_TAG_FIELD_NUMBER = 3;
private volatile java.lang.Object imageTag_;
/**
* string image_tag = 3;
*/
public java.lang.String getImageTag() {
java.lang.Object ref = imageTag_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageTag_ = s;
return s;
}
}
/**
* string image_tag = 3;
*/
public com.google.protobuf.ByteString
getImageTagBytes() {
java.lang.Object ref = imageTag_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTAINER_ID_FIELD_NUMBER = 4;
private volatile java.lang.Object containerId_;
/**
* string container_id = 4;
*/
public java.lang.String getContainerId() {
java.lang.Object ref = containerId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
containerId_ = s;
return s;
}
}
/**
* string container_id = 4;
*/
public com.google.protobuf.ByteString
getContainerIdBytes() {
java.lang.Object ref = containerId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
containerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTAINER_NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object containerName_;
/**
* string container_name = 5;
*/
public java.lang.String getContainerName() {
java.lang.Object ref = containerName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
containerName_ = s;
return s;
}
}
/**
* string container_name = 5;
*/
public com.google.protobuf.ByteString
getContainerNameBytes() {
java.lang.Object ref = containerName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
containerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getImageIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, imageId_);
}
if (!getImageRepositoryBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, imageRepository_);
}
if (!getImageTagBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, imageTag_);
}
if (!getContainerIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, containerId_);
}
if (!getContainerNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, containerName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getImageIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, imageId_);
}
if (!getImageRepositoryBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, imageRepository_);
}
if (!getImageTagBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, imageTag_);
}
if (!getContainerIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, containerId_);
}
if (!getContainerNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, containerName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Container)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Container other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Container) obj;
if (!getImageId()
.equals(other.getImageId())) return false;
if (!getImageRepository()
.equals(other.getImageRepository())) return false;
if (!getImageTag()
.equals(other.getImageTag())) return false;
if (!getContainerId()
.equals(other.getContainerId())) return false;
if (!getContainerName()
.equals(other.getContainerName())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + IMAGE_ID_FIELD_NUMBER;
hash = (53 * hash) + getImageId().hashCode();
hash = (37 * hash) + IMAGE_REPOSITORY_FIELD_NUMBER;
hash = (53 * hash) + getImageRepository().hashCode();
hash = (37 * hash) + IMAGE_TAG_FIELD_NUMBER;
hash = (53 * hash) + getImageTag().hashCode();
hash = (37 * hash) + CONTAINER_ID_FIELD_NUMBER;
hash = (53 * hash) + getContainerId().hashCode();
hash = (37 * hash) + CONTAINER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getContainerName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Container prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Container}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Container)
com.stackify.api.common.proto.StackifyProto.LogGroup.ContainerOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Container_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Container_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Container.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Container.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
imageId_ = "";
imageRepository_ = "";
imageTag_ = "";
containerId_ = "";
containerName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Container_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Container getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Container.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Container build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Container result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Container buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Container result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Container(this);
result.imageId_ = imageId_;
result.imageRepository_ = imageRepository_;
result.imageTag_ = imageTag_;
result.containerId_ = containerId_;
result.containerName_ = containerName_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Container) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Container)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Container other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Container.getDefaultInstance()) return this;
if (!other.getImageId().isEmpty()) {
imageId_ = other.imageId_;
onChanged();
}
if (!other.getImageRepository().isEmpty()) {
imageRepository_ = other.imageRepository_;
onChanged();
}
if (!other.getImageTag().isEmpty()) {
imageTag_ = other.imageTag_;
onChanged();
}
if (!other.getContainerId().isEmpty()) {
containerId_ = other.containerId_;
onChanged();
}
if (!other.getContainerName().isEmpty()) {
containerName_ = other.containerName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Container parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Container) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object imageId_ = "";
/**
* string image_id = 1;
*/
public java.lang.String getImageId() {
java.lang.Object ref = imageId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string image_id = 1;
*/
public com.google.protobuf.ByteString
getImageIdBytes() {
java.lang.Object ref = imageId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string image_id = 1;
*/
public Builder setImageId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
imageId_ = value;
onChanged();
return this;
}
/**
* string image_id = 1;
*/
public Builder clearImageId() {
imageId_ = getDefaultInstance().getImageId();
onChanged();
return this;
}
/**
* string image_id = 1;
*/
public Builder setImageIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
imageId_ = value;
onChanged();
return this;
}
private java.lang.Object imageRepository_ = "";
/**
* string image_repository = 2;
*/
public java.lang.String getImageRepository() {
java.lang.Object ref = imageRepository_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageRepository_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string image_repository = 2;
*/
public com.google.protobuf.ByteString
getImageRepositoryBytes() {
java.lang.Object ref = imageRepository_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageRepository_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string image_repository = 2;
*/
public Builder setImageRepository(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
imageRepository_ = value;
onChanged();
return this;
}
/**
* string image_repository = 2;
*/
public Builder clearImageRepository() {
imageRepository_ = getDefaultInstance().getImageRepository();
onChanged();
return this;
}
/**
* string image_repository = 2;
*/
public Builder setImageRepositoryBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
imageRepository_ = value;
onChanged();
return this;
}
private java.lang.Object imageTag_ = "";
/**
* string image_tag = 3;
*/
public java.lang.String getImageTag() {
java.lang.Object ref = imageTag_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
imageTag_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string image_tag = 3;
*/
public com.google.protobuf.ByteString
getImageTagBytes() {
java.lang.Object ref = imageTag_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
imageTag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string image_tag = 3;
*/
public Builder setImageTag(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
imageTag_ = value;
onChanged();
return this;
}
/**
* string image_tag = 3;
*/
public Builder clearImageTag() {
imageTag_ = getDefaultInstance().getImageTag();
onChanged();
return this;
}
/**
* string image_tag = 3;
*/
public Builder setImageTagBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
imageTag_ = value;
onChanged();
return this;
}
private java.lang.Object containerId_ = "";
/**
* string container_id = 4;
*/
public java.lang.String getContainerId() {
java.lang.Object ref = containerId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
containerId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string container_id = 4;
*/
public com.google.protobuf.ByteString
getContainerIdBytes() {
java.lang.Object ref = containerId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
containerId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string container_id = 4;
*/
public Builder setContainerId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
containerId_ = value;
onChanged();
return this;
}
/**
* string container_id = 4;
*/
public Builder clearContainerId() {
containerId_ = getDefaultInstance().getContainerId();
onChanged();
return this;
}
/**
* string container_id = 4;
*/
public Builder setContainerIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
containerId_ = value;
onChanged();
return this;
}
private java.lang.Object containerName_ = "";
/**
* string container_name = 5;
*/
public java.lang.String getContainerName() {
java.lang.Object ref = containerName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
containerName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string container_name = 5;
*/
public com.google.protobuf.ByteString
getContainerNameBytes() {
java.lang.Object ref = containerName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
containerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string container_name = 5;
*/
public Builder setContainerName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
containerName_ = value;
onChanged();
return this;
}
/**
* string container_name = 5;
*/
public Builder clearContainerName() {
containerName_ = getDefaultInstance().getContainerName();
onChanged();
return this;
}
/**
* string container_name = 5;
*/
public Builder setContainerNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
containerName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Container)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Container)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Container DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Container();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Container getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Container parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Container(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Container getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KubernetesOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Kubernetes)
com.google.protobuf.MessageOrBuilder {
/**
* string pod_name = 1;
*/
java.lang.String getPodName();
/**
* string pod_name = 1;
*/
com.google.protobuf.ByteString
getPodNameBytes();
/**
* string pod_namespace = 2;
*/
java.lang.String getPodNamespace();
/**
* string pod_namespace = 2;
*/
com.google.protobuf.ByteString
getPodNamespaceBytes();
/**
* string cluster_name = 3;
*/
java.lang.String getClusterName();
/**
* string cluster_name = 3;
*/
com.google.protobuf.ByteString
getClusterNameBytes();
}
/**
* Protobuf type {@code stackify.LogGroup.Kubernetes}
*/
public static final class Kubernetes extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Kubernetes)
KubernetesOrBuilder {
private static final long serialVersionUID = 0L;
// Use Kubernetes.newBuilder() to construct.
private Kubernetes(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Kubernetes() {
podName_ = "";
podNamespace_ = "";
clusterName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Kubernetes();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Kubernetes(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
podName_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
podNamespace_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
clusterName_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Kubernetes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Kubernetes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder.class);
}
public static final int POD_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object podName_;
/**
* string pod_name = 1;
*/
public java.lang.String getPodName() {
java.lang.Object ref = podName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
podName_ = s;
return s;
}
}
/**
* string pod_name = 1;
*/
public com.google.protobuf.ByteString
getPodNameBytes() {
java.lang.Object ref = podName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
podName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POD_NAMESPACE_FIELD_NUMBER = 2;
private volatile java.lang.Object podNamespace_;
/**
* string pod_namespace = 2;
*/
public java.lang.String getPodNamespace() {
java.lang.Object ref = podNamespace_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
podNamespace_ = s;
return s;
}
}
/**
* string pod_namespace = 2;
*/
public com.google.protobuf.ByteString
getPodNamespaceBytes() {
java.lang.Object ref = podNamespace_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
podNamespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLUSTER_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object clusterName_;
/**
* string cluster_name = 3;
*/
public java.lang.String getClusterName() {
java.lang.Object ref = clusterName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clusterName_ = s;
return s;
}
}
/**
* string cluster_name = 3;
*/
public com.google.protobuf.ByteString
getClusterNameBytes() {
java.lang.Object ref = clusterName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clusterName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getPodNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, podName_);
}
if (!getPodNamespaceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, podNamespace_);
}
if (!getClusterNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, clusterName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getPodNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, podName_);
}
if (!getPodNamespaceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, podNamespace_);
}
if (!getClusterNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, clusterName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes) obj;
if (!getPodName()
.equals(other.getPodName())) return false;
if (!getPodNamespace()
.equals(other.getPodNamespace())) return false;
if (!getClusterName()
.equals(other.getClusterName())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + POD_NAME_FIELD_NUMBER;
hash = (53 * hash) + getPodName().hashCode();
hash = (37 * hash) + POD_NAMESPACE_FIELD_NUMBER;
hash = (53 * hash) + getPodNamespace().hashCode();
hash = (37 * hash) + CLUSTER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getClusterName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Kubernetes}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Kubernetes)
com.stackify.api.common.proto.StackifyProto.LogGroup.KubernetesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Kubernetes_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Kubernetes_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
podName_ = "";
podNamespace_ = "";
clusterName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Kubernetes_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes(this);
result.podName_ = podName_;
result.podNamespace_ = podNamespace_;
result.clusterName_ = clusterName_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.getDefaultInstance()) return this;
if (!other.getPodName().isEmpty()) {
podName_ = other.podName_;
onChanged();
}
if (!other.getPodNamespace().isEmpty()) {
podNamespace_ = other.podNamespace_;
onChanged();
}
if (!other.getClusterName().isEmpty()) {
clusterName_ = other.clusterName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object podName_ = "";
/**
* string pod_name = 1;
*/
public java.lang.String getPodName() {
java.lang.Object ref = podName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
podName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string pod_name = 1;
*/
public com.google.protobuf.ByteString
getPodNameBytes() {
java.lang.Object ref = podName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
podName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string pod_name = 1;
*/
public Builder setPodName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
podName_ = value;
onChanged();
return this;
}
/**
* string pod_name = 1;
*/
public Builder clearPodName() {
podName_ = getDefaultInstance().getPodName();
onChanged();
return this;
}
/**
* string pod_name = 1;
*/
public Builder setPodNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
podName_ = value;
onChanged();
return this;
}
private java.lang.Object podNamespace_ = "";
/**
* string pod_namespace = 2;
*/
public java.lang.String getPodNamespace() {
java.lang.Object ref = podNamespace_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
podNamespace_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string pod_namespace = 2;
*/
public com.google.protobuf.ByteString
getPodNamespaceBytes() {
java.lang.Object ref = podNamespace_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
podNamespace_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string pod_namespace = 2;
*/
public Builder setPodNamespace(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
podNamespace_ = value;
onChanged();
return this;
}
/**
* string pod_namespace = 2;
*/
public Builder clearPodNamespace() {
podNamespace_ = getDefaultInstance().getPodNamespace();
onChanged();
return this;
}
/**
* string pod_namespace = 2;
*/
public Builder setPodNamespaceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
podNamespace_ = value;
onChanged();
return this;
}
private java.lang.Object clusterName_ = "";
/**
* string cluster_name = 3;
*/
public java.lang.String getClusterName() {
java.lang.Object ref = clusterName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clusterName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string cluster_name = 3;
*/
public com.google.protobuf.ByteString
getClusterNameBytes() {
java.lang.Object ref = clusterName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
clusterName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string cluster_name = 3;
*/
public Builder setClusterName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clusterName_ = value;
onChanged();
return this;
}
/**
* string cluster_name = 3;
*/
public Builder clearClusterName() {
clusterName_ = getDefaultInstance().getClusterName();
onChanged();
return this;
}
/**
* string cluster_name = 3;
*/
public Builder setClusterNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clusterName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Kubernetes)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Kubernetes)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Kubernetes parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Kubernetes(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface LogOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Log)
com.google.protobuf.MessageOrBuilder {
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
java.lang.String getMessage();
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
java.lang.String getData();
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
com.google.protobuf.ByteString
getDataBytes();
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
java.lang.String getThreadName();
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
com.google.protobuf.ByteString
getThreadNameBytes();
/**
*
* REQUIRED Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 4;
*/
long getDateMillis();
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
java.lang.String getLevel();
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
com.google.protobuf.ByteString
getLevelBytes();
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
java.lang.String getTransactionId();
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
com.google.protobuf.ByteString
getTransactionIdBytes();
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
java.lang.String getSourceMethod();
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
com.google.protobuf.ByteString
getSourceMethodBytes();
/**
*
* Optional. Line number. Example: 57
*
*
* int32 source_line = 8;
*/
int getSourceLine();
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
java.lang.String getId();
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
java.util.List
getTagsList();
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
int getTagsCount();
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
java.lang.String getTags(int index);
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
com.google.protobuf.ByteString
getTagsBytes(int index);
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
boolean hasError();
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error getError();
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.ErrorOrBuilder getErrorOrBuilder();
}
/**
* Protobuf type {@code stackify.LogGroup.Log}
*/
public static final class Log extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Log)
LogOrBuilder {
private static final long serialVersionUID = 0L;
// Use Log.newBuilder() to construct.
private Log(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Log() {
message_ = "";
data_ = "";
threadName_ = "";
level_ = "";
transactionId_ = "";
sourceMethod_ = "";
id_ = "";
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Log();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Log(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
data_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
threadName_ = s;
break;
}
case 32: {
dateMillis_ = input.readInt64();
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
level_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
transactionId_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
sourceMethod_ = s;
break;
}
case 64: {
sourceLine_ = input.readInt32();
break;
}
case 74: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 82: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
tags_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
tags_.add(s);
break;
}
case 90: {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder subBuilder = null;
if (error_ != null) {
subBuilder = error_.toBuilder();
}
error_ = input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(error_);
error_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
tags_ = tags_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder.class);
}
public interface ErrorOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Log.Error)
com.google.protobuf.MessageOrBuilder {
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
boolean hasEnvironmentDetail();
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail getEnvironmentDetail();
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetailOrBuilder getEnvironmentDetailOrBuilder();
/**
*
* Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 2;
*/
long getDateMillis();
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
boolean hasErrorItem();
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getErrorItem();
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder getErrorItemOrBuilder();
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
boolean hasWebRequestDetail();
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail getWebRequestDetail();
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetailOrBuilder getWebRequestDetailOrBuilder();
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
int getServerVariablesCount();
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
boolean containsServerVariables(
java.lang.String key);
/**
* Use {@link #getServerVariablesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getServerVariables();
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
java.util.Map
getServerVariablesMap();
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
java.lang.String getServerVariablesOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
java.lang.String getServerVariablesOrThrow(
java.lang.String key);
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
java.lang.String getCustomerName();
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
com.google.protobuf.ByteString
getCustomerNameBytes();
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
java.lang.String getUsername();
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
com.google.protobuf.ByteString
getUsernameBytes();
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error}
*/
public static final class Error extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Log.Error)
ErrorOrBuilder {
private static final long serialVersionUID = 0L;
// Use Error.newBuilder() to construct.
private Error(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Error() {
customerName_ = "";
username_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Error();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Error(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder subBuilder = null;
if (environmentDetail_ != null) {
subBuilder = environmentDetail_.toBuilder();
}
environmentDetail_ = input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(environmentDetail_);
environmentDetail_ = subBuilder.buildPartial();
}
break;
}
case 16: {
dateMillis_ = input.readInt64();
break;
}
case 26: {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder subBuilder = null;
if (errorItem_ != null) {
subBuilder = errorItem_.toBuilder();
}
errorItem_ = input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(errorItem_);
errorItem_ = subBuilder.buildPartial();
}
break;
}
case 34: {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder subBuilder = null;
if (webRequestDetail_ != null) {
subBuilder = webRequestDetail_.toBuilder();
}
webRequestDetail_ = input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(webRequestDetail_);
webRequestDetail_ = subBuilder.buildPartial();
}
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
serverVariables_ = com.google.protobuf.MapField.newMapField(
ServerVariablesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
serverVariables__ = input.readMessage(
ServerVariablesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
serverVariables_.getMutableMap().put(
serverVariables__.getKey(), serverVariables__.getValue());
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
customerName_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
username_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 5:
return internalGetServerVariables();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder.class);
}
public interface EnvironmentDetailOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Log.Error.EnvironmentDetail)
com.google.protobuf.MessageOrBuilder {
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
java.lang.String getDeviceName();
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
com.google.protobuf.ByteString
getDeviceNameBytes();
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
java.lang.String getApplicationName();
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
com.google.protobuf.ByteString
getApplicationNameBytes();
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
java.lang.String getApplicationLocation();
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
com.google.protobuf.ByteString
getApplicationLocationBytes();
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
java.lang.String getConfiguredApplicationName();
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
com.google.protobuf.ByteString
getConfiguredApplicationNameBytes();
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
java.lang.String getConfiguredEnvironmentName();
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
com.google.protobuf.ByteString
getConfiguredEnvironmentNameBytes();
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.EnvironmentDetail}
*/
public static final class EnvironmentDetail extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Log.Error.EnvironmentDetail)
EnvironmentDetailOrBuilder {
private static final long serialVersionUID = 0L;
// Use EnvironmentDetail.newBuilder() to construct.
private EnvironmentDetail(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EnvironmentDetail() {
deviceName_ = "";
applicationName_ = "";
applicationLocation_ = "";
configuredApplicationName_ = "";
configuredEnvironmentName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new EnvironmentDetail();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EnvironmentDetail(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
deviceName_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
applicationName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
applicationLocation_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
configuredApplicationName_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
configuredEnvironmentName_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder.class);
}
public static final int DEVICE_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object deviceName_;
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
deviceName_ = s;
return s;
}
}
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
public com.google.protobuf.ByteString
getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPLICATION_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object applicationName_;
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
public java.lang.String getApplicationName() {
java.lang.Object ref = applicationName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationName_ = s;
return s;
}
}
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
public com.google.protobuf.ByteString
getApplicationNameBytes() {
java.lang.Object ref = applicationName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPLICATION_LOCATION_FIELD_NUMBER = 3;
private volatile java.lang.Object applicationLocation_;
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
public java.lang.String getApplicationLocation() {
java.lang.Object ref = applicationLocation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationLocation_ = s;
return s;
}
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
public com.google.protobuf.ByteString
getApplicationLocationBytes() {
java.lang.Object ref = applicationLocation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationLocation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONFIGURED_APPLICATION_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object configuredApplicationName_;
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
public java.lang.String getConfiguredApplicationName() {
java.lang.Object ref = configuredApplicationName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configuredApplicationName_ = s;
return s;
}
}
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
public com.google.protobuf.ByteString
getConfiguredApplicationNameBytes() {
java.lang.Object ref = configuredApplicationName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configuredApplicationName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONFIGURED_ENVIRONMENT_NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object configuredEnvironmentName_;
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
public java.lang.String getConfiguredEnvironmentName() {
java.lang.Object ref = configuredEnvironmentName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configuredEnvironmentName_ = s;
return s;
}
}
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
public com.google.protobuf.ByteString
getConfiguredEnvironmentNameBytes() {
java.lang.Object ref = configuredEnvironmentName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configuredEnvironmentName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDeviceNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, deviceName_);
}
if (!getApplicationNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, applicationName_);
}
if (!getApplicationLocationBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, applicationLocation_);
}
if (!getConfiguredApplicationNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, configuredApplicationName_);
}
if (!getConfiguredEnvironmentNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, configuredEnvironmentName_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDeviceNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, deviceName_);
}
if (!getApplicationNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, applicationName_);
}
if (!getApplicationLocationBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, applicationLocation_);
}
if (!getConfiguredApplicationNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, configuredApplicationName_);
}
if (!getConfiguredEnvironmentNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, configuredEnvironmentName_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail) obj;
if (!getDeviceName()
.equals(other.getDeviceName())) return false;
if (!getApplicationName()
.equals(other.getApplicationName())) return false;
if (!getApplicationLocation()
.equals(other.getApplicationLocation())) return false;
if (!getConfiguredApplicationName()
.equals(other.getConfiguredApplicationName())) return false;
if (!getConfiguredEnvironmentName()
.equals(other.getConfiguredEnvironmentName())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DEVICE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getDeviceName().hashCode();
hash = (37 * hash) + APPLICATION_NAME_FIELD_NUMBER;
hash = (53 * hash) + getApplicationName().hashCode();
hash = (37 * hash) + APPLICATION_LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getApplicationLocation().hashCode();
hash = (37 * hash) + CONFIGURED_APPLICATION_NAME_FIELD_NUMBER;
hash = (53 * hash) + getConfiguredApplicationName().hashCode();
hash = (37 * hash) + CONFIGURED_ENVIRONMENT_NAME_FIELD_NUMBER;
hash = (53 * hash) + getConfiguredEnvironmentName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.EnvironmentDetail}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Log.Error.EnvironmentDetail)
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetailOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
deviceName_ = "";
applicationName_ = "";
applicationLocation_ = "";
configuredApplicationName_ = "";
configuredEnvironmentName_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail(this);
result.deviceName_ = deviceName_;
result.applicationName_ = applicationName_;
result.applicationLocation_ = applicationLocation_;
result.configuredApplicationName_ = configuredApplicationName_;
result.configuredEnvironmentName_ = configuredEnvironmentName_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.getDefaultInstance()) return this;
if (!other.getDeviceName().isEmpty()) {
deviceName_ = other.deviceName_;
onChanged();
}
if (!other.getApplicationName().isEmpty()) {
applicationName_ = other.applicationName_;
onChanged();
}
if (!other.getApplicationLocation().isEmpty()) {
applicationLocation_ = other.applicationLocation_;
onChanged();
}
if (!other.getConfiguredApplicationName().isEmpty()) {
configuredApplicationName_ = other.configuredApplicationName_;
onChanged();
}
if (!other.getConfiguredEnvironmentName().isEmpty()) {
configuredEnvironmentName_ = other.configuredEnvironmentName_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object deviceName_ = "";
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
deviceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
public com.google.protobuf.ByteString
getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
public Builder setDeviceName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
deviceName_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
public Builder clearDeviceName() {
deviceName_ = getDefaultInstance().getDeviceName();
onChanged();
return this;
}
/**
*
* REQUIRED. The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string device_name = 1;
*/
public Builder setDeviceNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
deviceName_ = value;
onChanged();
return this;
}
private java.lang.Object applicationName_ = "";
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
public java.lang.String getApplicationName() {
java.lang.Object ref = applicationName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
public com.google.protobuf.ByteString
getApplicationNameBytes() {
java.lang.Object ref = applicationName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
public Builder setApplicationName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
applicationName_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
public Builder clearApplicationName() {
applicationName_ = getDefaultInstance().getApplicationName();
onChanged();
return this;
}
/**
*
* REQUIRED. The name of the application. Example: "stackify-agent"
*
*
* string application_name = 2;
*/
public Builder setApplicationNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
applicationName_ = value;
onChanged();
return this;
}
private java.lang.Object applicationLocation_ = "";
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
public java.lang.String getApplicationLocation() {
java.lang.Object ref = applicationLocation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationLocation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
public com.google.protobuf.ByteString
getApplicationLocationBytes() {
java.lang.Object ref = applicationLocation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationLocation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
public Builder setApplicationLocation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
applicationLocation_ = value;
onChanged();
return this;
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
public Builder clearApplicationLocation() {
applicationLocation_ = getDefaultInstance().getApplicationLocation();
onChanged();
return this;
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 3;
*/
public Builder setApplicationLocationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
applicationLocation_ = value;
onChanged();
return this;
}
private java.lang.Object configuredApplicationName_ = "";
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
public java.lang.String getConfiguredApplicationName() {
java.lang.Object ref = configuredApplicationName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configuredApplicationName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
public com.google.protobuf.ByteString
getConfiguredApplicationNameBytes() {
java.lang.Object ref = configuredApplicationName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configuredApplicationName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
public Builder setConfiguredApplicationName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
configuredApplicationName_ = value;
onChanged();
return this;
}
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
public Builder clearConfiguredApplicationName() {
configuredApplicationName_ = getDefaultInstance().getConfiguredApplicationName();
onChanged();
return this;
}
/**
*
* Optional. The name of the application. This overrides the AppName field. Example: "my-stackify-agent"
*
*
* string configured_application_name = 4;
*/
public Builder setConfiguredApplicationNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
configuredApplicationName_ = value;
onChanged();
return this;
}
private java.lang.Object configuredEnvironmentName_ = "";
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
public java.lang.String getConfiguredEnvironmentName() {
java.lang.Object ref = configuredEnvironmentName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configuredEnvironmentName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
public com.google.protobuf.ByteString
getConfiguredEnvironmentNameBytes() {
java.lang.Object ref = configuredEnvironmentName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
configuredEnvironmentName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
public Builder setConfiguredEnvironmentName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
configuredEnvironmentName_ = value;
onChanged();
return this;
}
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
public Builder clearConfiguredEnvironmentName() {
configuredEnvironmentName_ = getDefaultInstance().getConfiguredEnvironmentName();
onChanged();
return this;
}
/**
*
* Optional. The environment name. If the device is monitored by Stackify, we will use the environment associated to the device. If the device is not monitored by Stackify, this should be specified. Example: "Prod"
*
*
* string configured_environment_name = 5;
*/
public Builder setConfiguredEnvironmentNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
configuredEnvironmentName_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Log.Error.EnvironmentDetail)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Log.Error.EnvironmentDetail)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EnvironmentDetail parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EnvironmentDetail(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ErrorItemOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Log.Error.ErrorItem)
com.google.protobuf.MessageOrBuilder {
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
java.lang.String getMessage();
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
com.google.protobuf.ByteString
getMessageBytes();
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
java.lang.String getErrorType();
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
com.google.protobuf.ByteString
getErrorTypeBytes();
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
java.lang.String getErrorTypeCode();
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
com.google.protobuf.ByteString
getErrorTypeCodeBytes();
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
int getDataCount();
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
boolean containsData(
java.lang.String key);
/**
* Use {@link #getDataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getData();
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
java.util.Map
getDataMap();
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
java.lang.String getDataOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
java.lang.String getDataOrThrow(
java.lang.String key);
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
java.lang.String getSourceMethod();
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
com.google.protobuf.ByteString
getSourceMethodBytes();
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
java.util.List
getStacktraceList();
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame getStacktrace(int index);
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
int getStacktraceCount();
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
java.util.List extends com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder>
getStacktraceOrBuilderList();
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder getStacktraceOrBuilder(
int index);
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
boolean hasInnerError();
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getInnerError();
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder getInnerErrorOrBuilder();
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.ErrorItem}
*/
public static final class ErrorItem extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Log.Error.ErrorItem)
ErrorItemOrBuilder {
private static final long serialVersionUID = 0L;
// Use ErrorItem.newBuilder() to construct.
private ErrorItem(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ErrorItem() {
message_ = "";
errorType_ = "";
errorTypeCode_ = "";
sourceMethod_ = "";
stacktrace_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ErrorItem();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ErrorItem(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
message_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
errorType_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
errorTypeCode_ = s;
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
data_ = com.google.protobuf.MapField.newMapField(
DataDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
data__ = input.readMessage(
DataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
data_.getMutableMap().put(
data__.getKey(), data__.getValue());
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
sourceMethod_ = s;
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
stacktrace_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
stacktrace_.add(
input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.parser(), extensionRegistry));
break;
}
case 58: {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder subBuilder = null;
if (innerError_ != null) {
subBuilder = innerError_.toBuilder();
}
innerError_ = input.readMessage(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(innerError_);
innerError_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) != 0)) {
stacktrace_ = java.util.Collections.unmodifiableList(stacktrace_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder.class);
}
public interface TraceFrameOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Log.Error.ErrorItem.TraceFrame)
com.google.protobuf.MessageOrBuilder {
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
java.lang.String getCodeFilename();
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
com.google.protobuf.ByteString
getCodeFilenameBytes();
/**
*
* Optional. Line number. Example: 16
*
*
* int32 line_number = 2;
*/
int getLineNumber();
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
java.lang.String getMethod();
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
com.google.protobuf.ByteString
getMethodBytes();
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.ErrorItem.TraceFrame}
*/
public static final class TraceFrame extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Log.Error.ErrorItem.TraceFrame)
TraceFrameOrBuilder {
private static final long serialVersionUID = 0L;
// Use TraceFrame.newBuilder() to construct.
private TraceFrame(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TraceFrame() {
codeFilename_ = "";
method_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new TraceFrame();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TraceFrame(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
codeFilename_ = s;
break;
}
case 16: {
lineNumber_ = input.readInt32();
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
method_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder.class);
}
public static final int CODE_FILENAME_FIELD_NUMBER = 1;
private volatile java.lang.Object codeFilename_;
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
public java.lang.String getCodeFilename() {
java.lang.Object ref = codeFilename_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
codeFilename_ = s;
return s;
}
}
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
public com.google.protobuf.ByteString
getCodeFilenameBytes() {
java.lang.Object ref = codeFilename_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
codeFilename_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LINE_NUMBER_FIELD_NUMBER = 2;
private int lineNumber_;
/**
*
* Optional. Line number. Example: 16
*
*
* int32 line_number = 2;
*/
public int getLineNumber() {
return lineNumber_;
}
public static final int METHOD_FIELD_NUMBER = 3;
private volatile java.lang.Object method_;
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
method_ = s;
return s;
}
}
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getCodeFilenameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, codeFilename_);
}
if (lineNumber_ != 0) {
output.writeInt32(2, lineNumber_);
}
if (!getMethodBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, method_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getCodeFilenameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, codeFilename_);
}
if (lineNumber_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, lineNumber_);
}
if (!getMethodBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, method_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame) obj;
if (!getCodeFilename()
.equals(other.getCodeFilename())) return false;
if (getLineNumber()
!= other.getLineNumber()) return false;
if (!getMethod()
.equals(other.getMethod())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CODE_FILENAME_FIELD_NUMBER;
hash = (53 * hash) + getCodeFilename().hashCode();
hash = (37 * hash) + LINE_NUMBER_FIELD_NUMBER;
hash = (53 * hash) + getLineNumber();
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + getMethod().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.ErrorItem.TraceFrame}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Log.Error.ErrorItem.TraceFrame)
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
codeFilename_ = "";
lineNumber_ = 0;
method_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame(this);
result.codeFilename_ = codeFilename_;
result.lineNumber_ = lineNumber_;
result.method_ = method_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.getDefaultInstance()) return this;
if (!other.getCodeFilename().isEmpty()) {
codeFilename_ = other.codeFilename_;
onChanged();
}
if (other.getLineNumber() != 0) {
setLineNumber(other.getLineNumber());
}
if (!other.getMethod().isEmpty()) {
method_ = other.method_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object codeFilename_ = "";
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
public java.lang.String getCodeFilename() {
java.lang.Object ref = codeFilename_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
codeFilename_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
public com.google.protobuf.ByteString
getCodeFilenameBytes() {
java.lang.Object ref = codeFilename_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
codeFilename_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
public Builder setCodeFilename(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
codeFilename_ = value;
onChanged();
return this;
}
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
public Builder clearCodeFilename() {
codeFilename_ = getDefaultInstance().getCodeFilename();
onChanged();
return this;
}
/**
*
* Optional. File name. Example: "SomeSweetClass.java"
*
*
* string code_filename = 1;
*/
public Builder setCodeFilenameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
codeFilename_ = value;
onChanged();
return this;
}
private int lineNumber_ ;
/**
*
* Optional. Line number. Example: 16
*
*
* int32 line_number = 2;
*/
public int getLineNumber() {
return lineNumber_;
}
/**
*
* Optional. Line number. Example: 16
*
*
* int32 line_number = 2;
*/
public Builder setLineNumber(int value) {
lineNumber_ = value;
onChanged();
return this;
}
/**
*
* Optional. Line number. Example: 16
*
*
* int32 line_number = 2;
*/
public Builder clearLineNumber() {
lineNumber_ = 0;
onChanged();
return this;
}
private java.lang.Object method_ = "";
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
method_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
public Builder setMethod(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
method_ = value;
onChanged();
return this;
}
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
public Builder clearMethod() {
method_ = getDefaultInstance().getMethod();
onChanged();
return this;
}
/**
*
* Fully qualified method name. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string method = 3;
*/
public Builder setMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
method_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Log.Error.ErrorItem.TraceFrame)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Log.Error.ErrorItem.TraceFrame)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TraceFrame parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TraceFrame(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int MESSAGE_FIELD_NUMBER = 1;
private volatile java.lang.Object message_;
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ERROR_TYPE_FIELD_NUMBER = 2;
private volatile java.lang.Object errorType_;
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
public java.lang.String getErrorType() {
java.lang.Object ref = errorType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorType_ = s;
return s;
}
}
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
public com.google.protobuf.ByteString
getErrorTypeBytes() {
java.lang.Object ref = errorType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ERROR_TYPE_CODE_FIELD_NUMBER = 3;
private volatile java.lang.Object errorTypeCode_;
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
public java.lang.String getErrorTypeCode() {
java.lang.Object ref = errorTypeCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorTypeCode_ = s;
return s;
}
}
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
public com.google.protobuf.ByteString
getErrorTypeCodeBytes() {
java.lang.Object ref = errorTypeCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorTypeCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 4;
private static final class DataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_DataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> data_;
private com.google.protobuf.MapField
internalGetData() {
if (data_ == null) {
return com.google.protobuf.MapField.emptyMapField(
DataDefaultEntryHolder.defaultEntry);
}
return data_;
}
public int getDataCount() {
return internalGetData().getMap().size();
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public boolean containsData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetData().getMap().containsKey(key);
}
/**
* Use {@link #getDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getData() {
return getDataMap();
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public java.util.Map getDataMap() {
return internalGetData().getMap();
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public java.lang.String getDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public java.lang.String getDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int SOURCE_METHOD_FIELD_NUMBER = 5;
private volatile java.lang.Object sourceMethod_;
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
public java.lang.String getSourceMethod() {
java.lang.Object ref = sourceMethod_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceMethod_ = s;
return s;
}
}
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
public com.google.protobuf.ByteString
getSourceMethodBytes() {
java.lang.Object ref = sourceMethod_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STACKTRACE_FIELD_NUMBER = 6;
private java.util.List stacktrace_;
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public java.util.List getStacktraceList() {
return stacktrace_;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public java.util.List extends com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder>
getStacktraceOrBuilderList() {
return stacktrace_;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public int getStacktraceCount() {
return stacktrace_.size();
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame getStacktrace(int index) {
return stacktrace_.get(index);
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder getStacktraceOrBuilder(
int index) {
return stacktrace_.get(index);
}
public static final int INNER_ERROR_FIELD_NUMBER = 7;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem innerError_;
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public boolean hasInnerError() {
return innerError_ != null;
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getInnerError() {
return innerError_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance() : innerError_;
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder getInnerErrorOrBuilder() {
return getInnerError();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, message_);
}
if (!getErrorTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, errorType_);
}
if (!getErrorTypeCodeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, errorTypeCode_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetData(),
DataDefaultEntryHolder.defaultEntry,
4);
if (!getSourceMethodBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, sourceMethod_);
}
for (int i = 0; i < stacktrace_.size(); i++) {
output.writeMessage(6, stacktrace_.get(i));
}
if (innerError_ != null) {
output.writeMessage(7, getInnerError());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, message_);
}
if (!getErrorTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, errorType_);
}
if (!getErrorTypeCodeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, errorTypeCode_);
}
for (java.util.Map.Entry entry
: internalGetData().getMap().entrySet()) {
com.google.protobuf.MapEntry
data__ = DataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, data__);
}
if (!getSourceMethodBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, sourceMethod_);
}
for (int i = 0; i < stacktrace_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, stacktrace_.get(i));
}
if (innerError_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getInnerError());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem) obj;
if (!getMessage()
.equals(other.getMessage())) return false;
if (!getErrorType()
.equals(other.getErrorType())) return false;
if (!getErrorTypeCode()
.equals(other.getErrorTypeCode())) return false;
if (!internalGetData().equals(
other.internalGetData())) return false;
if (!getSourceMethod()
.equals(other.getSourceMethod())) return false;
if (!getStacktraceList()
.equals(other.getStacktraceList())) return false;
if (hasInnerError() != other.hasInnerError()) return false;
if (hasInnerError()) {
if (!getInnerError()
.equals(other.getInnerError())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (37 * hash) + ERROR_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getErrorType().hashCode();
hash = (37 * hash) + ERROR_TYPE_CODE_FIELD_NUMBER;
hash = (53 * hash) + getErrorTypeCode().hashCode();
if (!internalGetData().getMap().isEmpty()) {
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetData().hashCode();
}
hash = (37 * hash) + SOURCE_METHOD_FIELD_NUMBER;
hash = (53 * hash) + getSourceMethod().hashCode();
if (getStacktraceCount() > 0) {
hash = (37 * hash) + STACKTRACE_FIELD_NUMBER;
hash = (53 * hash) + getStacktraceList().hashCode();
}
if (hasInnerError()) {
hash = (37 * hash) + INNER_ERROR_FIELD_NUMBER;
hash = (53 * hash) + getInnerError().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.ErrorItem}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Log.Error.ErrorItem)
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 4:
return internalGetData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 4:
return internalGetMutableData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getStacktraceFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
message_ = "";
errorType_ = "";
errorTypeCode_ = "";
internalGetMutableData().clear();
sourceMethod_ = "";
if (stacktraceBuilder_ == null) {
stacktrace_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
stacktraceBuilder_.clear();
}
if (innerErrorBuilder_ == null) {
innerError_ = null;
} else {
innerError_ = null;
innerErrorBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem(this);
int from_bitField0_ = bitField0_;
result.message_ = message_;
result.errorType_ = errorType_;
result.errorTypeCode_ = errorTypeCode_;
result.data_ = internalGetData();
result.data_.makeImmutable();
result.sourceMethod_ = sourceMethod_;
if (stacktraceBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
stacktrace_ = java.util.Collections.unmodifiableList(stacktrace_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.stacktrace_ = stacktrace_;
} else {
result.stacktrace_ = stacktraceBuilder_.build();
}
if (innerErrorBuilder_ == null) {
result.innerError_ = innerError_;
} else {
result.innerError_ = innerErrorBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance()) return this;
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (!other.getErrorType().isEmpty()) {
errorType_ = other.errorType_;
onChanged();
}
if (!other.getErrorTypeCode().isEmpty()) {
errorTypeCode_ = other.errorTypeCode_;
onChanged();
}
internalGetMutableData().mergeFrom(
other.internalGetData());
if (!other.getSourceMethod().isEmpty()) {
sourceMethod_ = other.sourceMethod_;
onChanged();
}
if (stacktraceBuilder_ == null) {
if (!other.stacktrace_.isEmpty()) {
if (stacktrace_.isEmpty()) {
stacktrace_ = other.stacktrace_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureStacktraceIsMutable();
stacktrace_.addAll(other.stacktrace_);
}
onChanged();
}
} else {
if (!other.stacktrace_.isEmpty()) {
if (stacktraceBuilder_.isEmpty()) {
stacktraceBuilder_.dispose();
stacktraceBuilder_ = null;
stacktrace_ = other.stacktrace_;
bitField0_ = (bitField0_ & ~0x00000002);
stacktraceBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getStacktraceFieldBuilder() : null;
} else {
stacktraceBuilder_.addAllMessages(other.stacktrace_);
}
}
}
if (other.hasInnerError()) {
mergeInnerError(other.getInnerError());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object message_ = "";
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
*
* Optional. The exception message. Example: "java.lang.NullPointerException: Something important was null that can't be null"
*
*
* string message = 1;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private java.lang.Object errorType_ = "";
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
public java.lang.String getErrorType() {
java.lang.Object ref = errorType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
public com.google.protobuf.ByteString
getErrorTypeBytes() {
java.lang.Object ref = errorType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
public Builder setErrorType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
errorType_ = value;
onChanged();
return this;
}
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
public Builder clearErrorType() {
errorType_ = getDefaultInstance().getErrorType();
onChanged();
return this;
}
/**
*
* The exception type. Example: "java.lang.RuntimeException"
*
*
* string error_type = 2;
*/
public Builder setErrorTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
errorType_ = value;
onChanged();
return this;
}
private java.lang.Object errorTypeCode_ = "";
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
public java.lang.String getErrorTypeCode() {
java.lang.Object ref = errorTypeCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
errorTypeCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
public com.google.protobuf.ByteString
getErrorTypeCodeBytes() {
java.lang.Object ref = errorTypeCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
errorTypeCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
public Builder setErrorTypeCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
errorTypeCode_ = value;
onChanged();
return this;
}
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
public Builder clearErrorTypeCode() {
errorTypeCode_ = getDefaultInstance().getErrorTypeCode();
onChanged();
return this;
}
/**
*
* Optional. The exception type code. Example: 40143
*
*
* string error_type_code = 3;
*/
public Builder setErrorTypeCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
errorTypeCode_ = value;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> data_;
private com.google.protobuf.MapField
internalGetData() {
if (data_ == null) {
return com.google.protobuf.MapField.emptyMapField(
DataDefaultEntryHolder.defaultEntry);
}
return data_;
}
private com.google.protobuf.MapField
internalGetMutableData() {
onChanged();;
if (data_ == null) {
data_ = com.google.protobuf.MapField.newMapField(
DataDefaultEntryHolder.defaultEntry);
}
if (!data_.isMutable()) {
data_ = data_.copy();
}
return data_;
}
public int getDataCount() {
return internalGetData().getMap().size();
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public boolean containsData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetData().getMap().containsKey(key);
}
/**
* Use {@link #getDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getData() {
return getDataMap();
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public java.util.Map getDataMap() {
return internalGetData().getMap();
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public java.lang.String getDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public java.lang.String getDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearData() {
internalGetMutableData().getMutableMap()
.clear();
return this;
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public Builder removeData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableData().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableData() {
return internalGetMutableData().getMutableMap();
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public Builder putData(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableData().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Optional. Additional data from the loggers diagnostic context. Example: {"Key1": "Value1", "Key2":"Value2"}
*
*
* map<string, string> data = 4;
*/
public Builder putAllData(
java.util.Map values) {
internalGetMutableData().getMutableMap()
.putAll(values);
return this;
}
private java.lang.Object sourceMethod_ = "";
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
public java.lang.String getSourceMethod() {
java.lang.Object ref = sourceMethod_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceMethod_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
public com.google.protobuf.ByteString
getSourceMethodBytes() {
java.lang.Object ref = sourceMethod_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
public Builder setSourceMethod(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceMethod_ = value;
onChanged();
return this;
}
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
public Builder clearSourceMethod() {
sourceMethod_ = getDefaultInstance().getSourceMethod();
onChanged();
return this;
}
/**
*
* Optional. Fully qualified method from the first stack trace frame. Example: "com.stackify.error.test.SomeSweetClass.doSomething"
*
*
* string source_method = 5;
*/
public Builder setSourceMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceMethod_ = value;
onChanged();
return this;
}
private java.util.List stacktrace_ =
java.util.Collections.emptyList();
private void ensureStacktraceIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
stacktrace_ = new java.util.ArrayList(stacktrace_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder> stacktraceBuilder_;
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public java.util.List getStacktraceList() {
if (stacktraceBuilder_ == null) {
return java.util.Collections.unmodifiableList(stacktrace_);
} else {
return stacktraceBuilder_.getMessageList();
}
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public int getStacktraceCount() {
if (stacktraceBuilder_ == null) {
return stacktrace_.size();
} else {
return stacktraceBuilder_.getCount();
}
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame getStacktrace(int index) {
if (stacktraceBuilder_ == null) {
return stacktrace_.get(index);
} else {
return stacktraceBuilder_.getMessage(index);
}
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder setStacktrace(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame value) {
if (stacktraceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStacktraceIsMutable();
stacktrace_.set(index, value);
onChanged();
} else {
stacktraceBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder setStacktrace(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder builderForValue) {
if (stacktraceBuilder_ == null) {
ensureStacktraceIsMutable();
stacktrace_.set(index, builderForValue.build());
onChanged();
} else {
stacktraceBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder addStacktrace(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame value) {
if (stacktraceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStacktraceIsMutable();
stacktrace_.add(value);
onChanged();
} else {
stacktraceBuilder_.addMessage(value);
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder addStacktrace(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame value) {
if (stacktraceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureStacktraceIsMutable();
stacktrace_.add(index, value);
onChanged();
} else {
stacktraceBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder addStacktrace(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder builderForValue) {
if (stacktraceBuilder_ == null) {
ensureStacktraceIsMutable();
stacktrace_.add(builderForValue.build());
onChanged();
} else {
stacktraceBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder addStacktrace(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder builderForValue) {
if (stacktraceBuilder_ == null) {
ensureStacktraceIsMutable();
stacktrace_.add(index, builderForValue.build());
onChanged();
} else {
stacktraceBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder addAllStacktrace(
java.lang.Iterable extends com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame> values) {
if (stacktraceBuilder_ == null) {
ensureStacktraceIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, stacktrace_);
onChanged();
} else {
stacktraceBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder clearStacktrace() {
if (stacktraceBuilder_ == null) {
stacktrace_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
stacktraceBuilder_.clear();
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public Builder removeStacktrace(int index) {
if (stacktraceBuilder_ == null) {
ensureStacktraceIsMutable();
stacktrace_.remove(index);
onChanged();
} else {
stacktraceBuilder_.remove(index);
}
return this;
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder getStacktraceBuilder(
int index) {
return getStacktraceFieldBuilder().getBuilder(index);
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder getStacktraceOrBuilder(
int index) {
if (stacktraceBuilder_ == null) {
return stacktrace_.get(index); } else {
return stacktraceBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public java.util.List extends com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder>
getStacktraceOrBuilderList() {
if (stacktraceBuilder_ != null) {
return stacktraceBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(stacktrace_);
}
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder addStacktraceBuilder() {
return getStacktraceFieldBuilder().addBuilder(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.getDefaultInstance());
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder addStacktraceBuilder(
int index) {
return getStacktraceFieldBuilder().addBuilder(
index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.getDefaultInstance());
}
/**
*
* Lists of stack trace frames.
*
*
* repeated .stackify.LogGroup.Log.Error.ErrorItem.TraceFrame stacktrace = 6;
*/
public java.util.List
getStacktraceBuilderList() {
return getStacktraceFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder>
getStacktraceFieldBuilder() {
if (stacktraceBuilder_ == null) {
stacktraceBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrame.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.TraceFrameOrBuilder>(
stacktrace_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
stacktrace_ = null;
}
return stacktraceBuilder_;
}
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem innerError_;
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder> innerErrorBuilder_;
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public boolean hasInnerError() {
return innerErrorBuilder_ != null || innerError_ != null;
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getInnerError() {
if (innerErrorBuilder_ == null) {
return innerError_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance() : innerError_;
} else {
return innerErrorBuilder_.getMessage();
}
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public Builder setInnerError(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem value) {
if (innerErrorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
innerError_ = value;
onChanged();
} else {
innerErrorBuilder_.setMessage(value);
}
return this;
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public Builder setInnerError(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder builderForValue) {
if (innerErrorBuilder_ == null) {
innerError_ = builderForValue.build();
onChanged();
} else {
innerErrorBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public Builder mergeInnerError(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem value) {
if (innerErrorBuilder_ == null) {
if (innerError_ != null) {
innerError_ =
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.newBuilder(innerError_).mergeFrom(value).buildPartial();
} else {
innerError_ = value;
}
onChanged();
} else {
innerErrorBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public Builder clearInnerError() {
if (innerErrorBuilder_ == null) {
innerError_ = null;
onChanged();
} else {
innerError_ = null;
innerErrorBuilder_ = null;
}
return this;
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder getInnerErrorBuilder() {
onChanged();
return getInnerErrorFieldBuilder().getBuilder();
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder getInnerErrorOrBuilder() {
if (innerErrorBuilder_ != null) {
return innerErrorBuilder_.getMessageOrBuilder();
} else {
return innerError_ == null ?
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance() : innerError_;
}
}
/**
*
* Optional. Same as Msgs[*]/Ex/Error object.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem inner_error = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder>
getInnerErrorFieldBuilder() {
if (innerErrorBuilder_ == null) {
innerErrorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder>(
getInnerError(),
getParentForChildren(),
isClean());
innerError_ = null;
}
return innerErrorBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Log.Error.ErrorItem)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Log.Error.ErrorItem)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ErrorItem parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ErrorItem(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface WebRequestDetailOrBuilder extends
// @@protoc_insertion_point(interface_extends:stackify.LogGroup.Log.Error.WebRequestDetail)
com.google.protobuf.MessageOrBuilder {
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
java.lang.String getUserIpAddress();
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
com.google.protobuf.ByteString
getUserIpAddressBytes();
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
java.lang.String getHttpMethod();
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
com.google.protobuf.ByteString
getHttpMethodBytes();
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
java.lang.String getRequestProtocol();
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
com.google.protobuf.ByteString
getRequestProtocolBytes();
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
java.lang.String getRequestUrl();
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
com.google.protobuf.ByteString
getRequestUrlBytes();
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
java.lang.String getRequestUrlRoot();
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
com.google.protobuf.ByteString
getRequestUrlRootBytes();
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
java.lang.String getReferralUrl();
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
com.google.protobuf.ByteString
getReferralUrlBytes();
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
int getHeadersCount();
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
boolean containsHeaders(
java.lang.String key);
/**
* Use {@link #getHeadersMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getHeaders();
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
java.util.Map
getHeadersMap();
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
java.lang.String getHeadersOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
java.lang.String getHeadersOrThrow(
java.lang.String key);
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
int getCookiesCount();
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
boolean containsCookies(
java.lang.String key);
/**
* Use {@link #getCookiesMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getCookies();
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
java.util.Map
getCookiesMap();
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
java.lang.String getCookiesOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
java.lang.String getCookiesOrThrow(
java.lang.String key);
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
int getQuerystringCount();
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
boolean containsQuerystring(
java.lang.String key);
/**
* Use {@link #getQuerystringMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getQuerystring();
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
java.util.Map
getQuerystringMap();
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
java.lang.String getQuerystringOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
java.lang.String getQuerystringOrThrow(
java.lang.String key);
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
int getPostDataCount();
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
boolean containsPostData(
java.lang.String key);
/**
* Use {@link #getPostDataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getPostData();
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
java.util.Map
getPostDataMap();
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
java.lang.String getPostDataOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
java.lang.String getPostDataOrThrow(
java.lang.String key);
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
int getSessionDataCount();
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
boolean containsSessionData(
java.lang.String key);
/**
* Use {@link #getSessionDataMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getSessionData();
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
java.util.Map
getSessionDataMap();
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
java.lang.String getSessionDataOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
java.lang.String getSessionDataOrThrow(
java.lang.String key);
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
java.lang.String getPostDataRaw();
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
com.google.protobuf.ByteString
getPostDataRawBytes();
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
java.lang.String getMvcAction();
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
com.google.protobuf.ByteString
getMvcActionBytes();
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
java.lang.String getMvcController();
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
com.google.protobuf.ByteString
getMvcControllerBytes();
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
java.lang.String getMvcArea();
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
com.google.protobuf.ByteString
getMvcAreaBytes();
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.WebRequestDetail}
*/
public static final class WebRequestDetail extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:stackify.LogGroup.Log.Error.WebRequestDetail)
WebRequestDetailOrBuilder {
private static final long serialVersionUID = 0L;
// Use WebRequestDetail.newBuilder() to construct.
private WebRequestDetail(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private WebRequestDetail() {
userIpAddress_ = "";
httpMethod_ = "";
requestProtocol_ = "";
requestUrl_ = "";
requestUrlRoot_ = "";
referralUrl_ = "";
postDataRaw_ = "";
mvcAction_ = "";
mvcController_ = "";
mvcArea_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new WebRequestDetail();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private WebRequestDetail(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
userIpAddress_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
httpMethod_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
requestProtocol_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
requestUrl_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
requestUrlRoot_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
referralUrl_ = s;
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
headers_ = com.google.protobuf.MapField.newMapField(
HeadersDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000001;
}
com.google.protobuf.MapEntry
headers__ = input.readMessage(
HeadersDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
headers_.getMutableMap().put(
headers__.getKey(), headers__.getValue());
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
cookies_ = com.google.protobuf.MapField.newMapField(
CookiesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
cookies__ = input.readMessage(
CookiesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
cookies_.getMutableMap().put(
cookies__.getKey(), cookies__.getValue());
break;
}
case 74: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
querystring_ = com.google.protobuf.MapField.newMapField(
QuerystringDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000004;
}
com.google.protobuf.MapEntry
querystring__ = input.readMessage(
QuerystringDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
querystring_.getMutableMap().put(
querystring__.getKey(), querystring__.getValue());
break;
}
case 82: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
postData_ = com.google.protobuf.MapField.newMapField(
PostDataDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000008;
}
com.google.protobuf.MapEntry
postData__ = input.readMessage(
PostDataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
postData_.getMutableMap().put(
postData__.getKey(), postData__.getValue());
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
sessionData_ = com.google.protobuf.MapField.newMapField(
SessionDataDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000010;
}
com.google.protobuf.MapEntry
sessionData__ = input.readMessage(
SessionDataDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
sessionData_.getMutableMap().put(
sessionData__.getKey(), sessionData__.getValue());
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
postDataRaw_ = s;
break;
}
case 106: {
java.lang.String s = input.readStringRequireUtf8();
mvcAction_ = s;
break;
}
case 114: {
java.lang.String s = input.readStringRequireUtf8();
mvcController_ = s;
break;
}
case 122: {
java.lang.String s = input.readStringRequireUtf8();
mvcArea_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 7:
return internalGetHeaders();
case 8:
return internalGetCookies();
case 9:
return internalGetQuerystring();
case 10:
return internalGetPostData();
case 11:
return internalGetSessionData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder.class);
}
public static final int USER_IP_ADDRESS_FIELD_NUMBER = 1;
private volatile java.lang.Object userIpAddress_;
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
public java.lang.String getUserIpAddress() {
java.lang.Object ref = userIpAddress_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userIpAddress_ = s;
return s;
}
}
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
public com.google.protobuf.ByteString
getUserIpAddressBytes() {
java.lang.Object ref = userIpAddress_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userIpAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HTTP_METHOD_FIELD_NUMBER = 2;
private volatile java.lang.Object httpMethod_;
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
public java.lang.String getHttpMethod() {
java.lang.Object ref = httpMethod_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
httpMethod_ = s;
return s;
}
}
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
public com.google.protobuf.ByteString
getHttpMethodBytes() {
java.lang.Object ref = httpMethod_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
httpMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_PROTOCOL_FIELD_NUMBER = 3;
private volatile java.lang.Object requestProtocol_;
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
public java.lang.String getRequestProtocol() {
java.lang.Object ref = requestProtocol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestProtocol_ = s;
return s;
}
}
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
public com.google.protobuf.ByteString
getRequestProtocolBytes() {
java.lang.Object ref = requestProtocol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestProtocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_URL_FIELD_NUMBER = 4;
private volatile java.lang.Object requestUrl_;
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
public java.lang.String getRequestUrl() {
java.lang.Object ref = requestUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestUrl_ = s;
return s;
}
}
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
public com.google.protobuf.ByteString
getRequestUrlBytes() {
java.lang.Object ref = requestUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REQUEST_URL_ROOT_FIELD_NUMBER = 5;
private volatile java.lang.Object requestUrlRoot_;
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
public java.lang.String getRequestUrlRoot() {
java.lang.Object ref = requestUrlRoot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestUrlRoot_ = s;
return s;
}
}
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
public com.google.protobuf.ByteString
getRequestUrlRootBytes() {
java.lang.Object ref = requestUrlRoot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestUrlRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REFERRAL_URL_FIELD_NUMBER = 6;
private volatile java.lang.Object referralUrl_;
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
public java.lang.String getReferralUrl() {
java.lang.Object ref = referralUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referralUrl_ = s;
return s;
}
}
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
public com.google.protobuf.ByteString
getReferralUrlBytes() {
java.lang.Object ref = referralUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referralUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int HEADERS_FIELD_NUMBER = 7;
private static final class HeadersDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_HeadersEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> headers_;
private com.google.protobuf.MapField
internalGetHeaders() {
if (headers_ == null) {
return com.google.protobuf.MapField.emptyMapField(
HeadersDefaultEntryHolder.defaultEntry);
}
return headers_;
}
public int getHeadersCount() {
return internalGetHeaders().getMap().size();
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public boolean containsHeaders(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetHeaders().getMap().containsKey(key);
}
/**
* Use {@link #getHeadersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getHeaders() {
return getHeadersMap();
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public java.util.Map getHeadersMap() {
return internalGetHeaders().getMap();
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public java.lang.String getHeadersOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetHeaders().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public java.lang.String getHeadersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetHeaders().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int COOKIES_FIELD_NUMBER = 8;
private static final class CookiesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_CookiesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> cookies_;
private com.google.protobuf.MapField
internalGetCookies() {
if (cookies_ == null) {
return com.google.protobuf.MapField.emptyMapField(
CookiesDefaultEntryHolder.defaultEntry);
}
return cookies_;
}
public int getCookiesCount() {
return internalGetCookies().getMap().size();
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public boolean containsCookies(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetCookies().getMap().containsKey(key);
}
/**
* Use {@link #getCookiesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getCookies() {
return getCookiesMap();
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public java.util.Map getCookiesMap() {
return internalGetCookies().getMap();
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public java.lang.String getCookiesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCookies().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public java.lang.String getCookiesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCookies().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int QUERYSTRING_FIELD_NUMBER = 9;
private static final class QuerystringDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_QuerystringEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> querystring_;
private com.google.protobuf.MapField
internalGetQuerystring() {
if (querystring_ == null) {
return com.google.protobuf.MapField.emptyMapField(
QuerystringDefaultEntryHolder.defaultEntry);
}
return querystring_;
}
public int getQuerystringCount() {
return internalGetQuerystring().getMap().size();
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public boolean containsQuerystring(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetQuerystring().getMap().containsKey(key);
}
/**
* Use {@link #getQuerystringMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getQuerystring() {
return getQuerystringMap();
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public java.util.Map getQuerystringMap() {
return internalGetQuerystring().getMap();
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public java.lang.String getQuerystringOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetQuerystring().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public java.lang.String getQuerystringOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetQuerystring().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int POST_DATA_FIELD_NUMBER = 10;
private static final class PostDataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_PostDataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> postData_;
private com.google.protobuf.MapField
internalGetPostData() {
if (postData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
PostDataDefaultEntryHolder.defaultEntry);
}
return postData_;
}
public int getPostDataCount() {
return internalGetPostData().getMap().size();
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public boolean containsPostData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetPostData().getMap().containsKey(key);
}
/**
* Use {@link #getPostDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getPostData() {
return getPostDataMap();
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public java.util.Map getPostDataMap() {
return internalGetPostData().getMap();
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public java.lang.String getPostDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPostData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public java.lang.String getPostDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPostData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int SESSION_DATA_FIELD_NUMBER = 11;
private static final class SessionDataDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_SessionDataEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> sessionData_;
private com.google.protobuf.MapField
internalGetSessionData() {
if (sessionData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
SessionDataDefaultEntryHolder.defaultEntry);
}
return sessionData_;
}
public int getSessionDataCount() {
return internalGetSessionData().getMap().size();
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public boolean containsSessionData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetSessionData().getMap().containsKey(key);
}
/**
* Use {@link #getSessionDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getSessionData() {
return getSessionDataMap();
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public java.util.Map getSessionDataMap() {
return internalGetSessionData().getMap();
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public java.lang.String getSessionDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetSessionData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public java.lang.String getSessionDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetSessionData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int POST_DATA_RAW_FIELD_NUMBER = 12;
private volatile java.lang.Object postDataRaw_;
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
public java.lang.String getPostDataRaw() {
java.lang.Object ref = postDataRaw_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
postDataRaw_ = s;
return s;
}
}
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
public com.google.protobuf.ByteString
getPostDataRawBytes() {
java.lang.Object ref = postDataRaw_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
postDataRaw_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MVC_ACTION_FIELD_NUMBER = 13;
private volatile java.lang.Object mvcAction_;
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
public java.lang.String getMvcAction() {
java.lang.Object ref = mvcAction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mvcAction_ = s;
return s;
}
}
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
public com.google.protobuf.ByteString
getMvcActionBytes() {
java.lang.Object ref = mvcAction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mvcAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MVC_CONTROLLER_FIELD_NUMBER = 14;
private volatile java.lang.Object mvcController_;
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
public java.lang.String getMvcController() {
java.lang.Object ref = mvcController_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mvcController_ = s;
return s;
}
}
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
public com.google.protobuf.ByteString
getMvcControllerBytes() {
java.lang.Object ref = mvcController_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mvcController_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MVC_AREA_FIELD_NUMBER = 15;
private volatile java.lang.Object mvcArea_;
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
public java.lang.String getMvcArea() {
java.lang.Object ref = mvcArea_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mvcArea_ = s;
return s;
}
}
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
public com.google.protobuf.ByteString
getMvcAreaBytes() {
java.lang.Object ref = mvcArea_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mvcArea_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getUserIpAddressBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, userIpAddress_);
}
if (!getHttpMethodBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, httpMethod_);
}
if (!getRequestProtocolBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, requestProtocol_);
}
if (!getRequestUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, requestUrl_);
}
if (!getRequestUrlRootBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, requestUrlRoot_);
}
if (!getReferralUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, referralUrl_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetHeaders(),
HeadersDefaultEntryHolder.defaultEntry,
7);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetCookies(),
CookiesDefaultEntryHolder.defaultEntry,
8);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetQuerystring(),
QuerystringDefaultEntryHolder.defaultEntry,
9);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetPostData(),
PostDataDefaultEntryHolder.defaultEntry,
10);
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetSessionData(),
SessionDataDefaultEntryHolder.defaultEntry,
11);
if (!getPostDataRawBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, postDataRaw_);
}
if (!getMvcActionBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, mvcAction_);
}
if (!getMvcControllerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, mvcController_);
}
if (!getMvcAreaBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, mvcArea_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getUserIpAddressBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, userIpAddress_);
}
if (!getHttpMethodBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, httpMethod_);
}
if (!getRequestProtocolBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, requestProtocol_);
}
if (!getRequestUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, requestUrl_);
}
if (!getRequestUrlRootBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, requestUrlRoot_);
}
if (!getReferralUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, referralUrl_);
}
for (java.util.Map.Entry entry
: internalGetHeaders().getMap().entrySet()) {
com.google.protobuf.MapEntry
headers__ = HeadersDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, headers__);
}
for (java.util.Map.Entry entry
: internalGetCookies().getMap().entrySet()) {
com.google.protobuf.MapEntry
cookies__ = CookiesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, cookies__);
}
for (java.util.Map.Entry entry
: internalGetQuerystring().getMap().entrySet()) {
com.google.protobuf.MapEntry
querystring__ = QuerystringDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, querystring__);
}
for (java.util.Map.Entry entry
: internalGetPostData().getMap().entrySet()) {
com.google.protobuf.MapEntry
postData__ = PostDataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, postData__);
}
for (java.util.Map.Entry entry
: internalGetSessionData().getMap().entrySet()) {
com.google.protobuf.MapEntry
sessionData__ = SessionDataDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, sessionData__);
}
if (!getPostDataRawBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, postDataRaw_);
}
if (!getMvcActionBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, mvcAction_);
}
if (!getMvcControllerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, mvcController_);
}
if (!getMvcAreaBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, mvcArea_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail) obj;
if (!getUserIpAddress()
.equals(other.getUserIpAddress())) return false;
if (!getHttpMethod()
.equals(other.getHttpMethod())) return false;
if (!getRequestProtocol()
.equals(other.getRequestProtocol())) return false;
if (!getRequestUrl()
.equals(other.getRequestUrl())) return false;
if (!getRequestUrlRoot()
.equals(other.getRequestUrlRoot())) return false;
if (!getReferralUrl()
.equals(other.getReferralUrl())) return false;
if (!internalGetHeaders().equals(
other.internalGetHeaders())) return false;
if (!internalGetCookies().equals(
other.internalGetCookies())) return false;
if (!internalGetQuerystring().equals(
other.internalGetQuerystring())) return false;
if (!internalGetPostData().equals(
other.internalGetPostData())) return false;
if (!internalGetSessionData().equals(
other.internalGetSessionData())) return false;
if (!getPostDataRaw()
.equals(other.getPostDataRaw())) return false;
if (!getMvcAction()
.equals(other.getMvcAction())) return false;
if (!getMvcController()
.equals(other.getMvcController())) return false;
if (!getMvcArea()
.equals(other.getMvcArea())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + USER_IP_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getUserIpAddress().hashCode();
hash = (37 * hash) + HTTP_METHOD_FIELD_NUMBER;
hash = (53 * hash) + getHttpMethod().hashCode();
hash = (37 * hash) + REQUEST_PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + getRequestProtocol().hashCode();
hash = (37 * hash) + REQUEST_URL_FIELD_NUMBER;
hash = (53 * hash) + getRequestUrl().hashCode();
hash = (37 * hash) + REQUEST_URL_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getRequestUrlRoot().hashCode();
hash = (37 * hash) + REFERRAL_URL_FIELD_NUMBER;
hash = (53 * hash) + getReferralUrl().hashCode();
if (!internalGetHeaders().getMap().isEmpty()) {
hash = (37 * hash) + HEADERS_FIELD_NUMBER;
hash = (53 * hash) + internalGetHeaders().hashCode();
}
if (!internalGetCookies().getMap().isEmpty()) {
hash = (37 * hash) + COOKIES_FIELD_NUMBER;
hash = (53 * hash) + internalGetCookies().hashCode();
}
if (!internalGetQuerystring().getMap().isEmpty()) {
hash = (37 * hash) + QUERYSTRING_FIELD_NUMBER;
hash = (53 * hash) + internalGetQuerystring().hashCode();
}
if (!internalGetPostData().getMap().isEmpty()) {
hash = (37 * hash) + POST_DATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetPostData().hashCode();
}
if (!internalGetSessionData().getMap().isEmpty()) {
hash = (37 * hash) + SESSION_DATA_FIELD_NUMBER;
hash = (53 * hash) + internalGetSessionData().hashCode();
}
hash = (37 * hash) + POST_DATA_RAW_FIELD_NUMBER;
hash = (53 * hash) + getPostDataRaw().hashCode();
hash = (37 * hash) + MVC_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getMvcAction().hashCode();
hash = (37 * hash) + MVC_CONTROLLER_FIELD_NUMBER;
hash = (53 * hash) + getMvcController().hashCode();
hash = (37 * hash) + MVC_AREA_FIELD_NUMBER;
hash = (53 * hash) + getMvcArea().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error.WebRequestDetail}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Log.Error.WebRequestDetail)
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetailOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 7:
return internalGetHeaders();
case 8:
return internalGetCookies();
case 9:
return internalGetQuerystring();
case 10:
return internalGetPostData();
case 11:
return internalGetSessionData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 7:
return internalGetMutableHeaders();
case 8:
return internalGetMutableCookies();
case 9:
return internalGetMutableQuerystring();
case 10:
return internalGetMutablePostData();
case 11:
return internalGetMutableSessionData();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
userIpAddress_ = "";
httpMethod_ = "";
requestProtocol_ = "";
requestUrl_ = "";
requestUrlRoot_ = "";
referralUrl_ = "";
internalGetMutableHeaders().clear();
internalGetMutableCookies().clear();
internalGetMutableQuerystring().clear();
internalGetMutablePostData().clear();
internalGetMutableSessionData().clear();
postDataRaw_ = "";
mvcAction_ = "";
mvcController_ = "";
mvcArea_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail(this);
int from_bitField0_ = bitField0_;
result.userIpAddress_ = userIpAddress_;
result.httpMethod_ = httpMethod_;
result.requestProtocol_ = requestProtocol_;
result.requestUrl_ = requestUrl_;
result.requestUrlRoot_ = requestUrlRoot_;
result.referralUrl_ = referralUrl_;
result.headers_ = internalGetHeaders();
result.headers_.makeImmutable();
result.cookies_ = internalGetCookies();
result.cookies_.makeImmutable();
result.querystring_ = internalGetQuerystring();
result.querystring_.makeImmutable();
result.postData_ = internalGetPostData();
result.postData_.makeImmutable();
result.sessionData_ = internalGetSessionData();
result.sessionData_.makeImmutable();
result.postDataRaw_ = postDataRaw_;
result.mvcAction_ = mvcAction_;
result.mvcController_ = mvcController_;
result.mvcArea_ = mvcArea_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.getDefaultInstance()) return this;
if (!other.getUserIpAddress().isEmpty()) {
userIpAddress_ = other.userIpAddress_;
onChanged();
}
if (!other.getHttpMethod().isEmpty()) {
httpMethod_ = other.httpMethod_;
onChanged();
}
if (!other.getRequestProtocol().isEmpty()) {
requestProtocol_ = other.requestProtocol_;
onChanged();
}
if (!other.getRequestUrl().isEmpty()) {
requestUrl_ = other.requestUrl_;
onChanged();
}
if (!other.getRequestUrlRoot().isEmpty()) {
requestUrlRoot_ = other.requestUrlRoot_;
onChanged();
}
if (!other.getReferralUrl().isEmpty()) {
referralUrl_ = other.referralUrl_;
onChanged();
}
internalGetMutableHeaders().mergeFrom(
other.internalGetHeaders());
internalGetMutableCookies().mergeFrom(
other.internalGetCookies());
internalGetMutableQuerystring().mergeFrom(
other.internalGetQuerystring());
internalGetMutablePostData().mergeFrom(
other.internalGetPostData());
internalGetMutableSessionData().mergeFrom(
other.internalGetSessionData());
if (!other.getPostDataRaw().isEmpty()) {
postDataRaw_ = other.postDataRaw_;
onChanged();
}
if (!other.getMvcAction().isEmpty()) {
mvcAction_ = other.mvcAction_;
onChanged();
}
if (!other.getMvcController().isEmpty()) {
mvcController_ = other.mvcController_;
onChanged();
}
if (!other.getMvcArea().isEmpty()) {
mvcArea_ = other.mvcArea_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object userIpAddress_ = "";
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
public java.lang.String getUserIpAddress() {
java.lang.Object ref = userIpAddress_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
userIpAddress_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
public com.google.protobuf.ByteString
getUserIpAddressBytes() {
java.lang.Object ref = userIpAddress_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
userIpAddress_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
public Builder setUserIpAddress(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
userIpAddress_ = value;
onChanged();
return this;
}
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
public Builder clearUserIpAddress() {
userIpAddress_ = getDefaultInstance().getUserIpAddress();
onChanged();
return this;
}
/**
*
* Optional. IP address of the user. Example: "127.0.0.1"
*
*
* string user_ip_address = 1;
*/
public Builder setUserIpAddressBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
userIpAddress_ = value;
onChanged();
return this;
}
private java.lang.Object httpMethod_ = "";
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
public java.lang.String getHttpMethod() {
java.lang.Object ref = httpMethod_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
httpMethod_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
public com.google.protobuf.ByteString
getHttpMethodBytes() {
java.lang.Object ref = httpMethod_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
httpMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
public Builder setHttpMethod(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
httpMethod_ = value;
onChanged();
return this;
}
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
public Builder clearHttpMethod() {
httpMethod_ = getDefaultInstance().getHttpMethod();
onChanged();
return this;
}
/**
*
* Optional. HTTP method (GET, POST, PUT, DELETE, etc.). Example: "GET"
*
*
* string http_method = 2;
*/
public Builder setHttpMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
httpMethod_ = value;
onChanged();
return this;
}
private java.lang.Object requestProtocol_ = "";
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
public java.lang.String getRequestProtocol() {
java.lang.Object ref = requestProtocol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestProtocol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
public com.google.protobuf.ByteString
getRequestProtocolBytes() {
java.lang.Object ref = requestProtocol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestProtocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
public Builder setRequestProtocol(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestProtocol_ = value;
onChanged();
return this;
}
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
public Builder clearRequestProtocol() {
requestProtocol_ = getDefaultInstance().getRequestProtocol();
onChanged();
return this;
}
/**
*
* Optional. HTTP request protocol. Example: "HTTP/1.1"
*
*
* string request_protocol = 3;
*/
public Builder setRequestProtocolBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestProtocol_ = value;
onChanged();
return this;
}
private java.lang.Object requestUrl_ = "";
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
public java.lang.String getRequestUrl() {
java.lang.Object ref = requestUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
public com.google.protobuf.ByteString
getRequestUrlBytes() {
java.lang.Object ref = requestUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
public Builder setRequestUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestUrl_ = value;
onChanged();
return this;
}
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
public Builder clearRequestUrl() {
requestUrl_ = getDefaultInstance().getRequestUrl();
onChanged();
return this;
}
/**
*
* Optional. HTTP request URL. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string request_url = 4;
*/
public Builder setRequestUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestUrl_ = value;
onChanged();
return this;
}
private java.lang.Object requestUrlRoot_ = "";
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
public java.lang.String getRequestUrlRoot() {
java.lang.Object ref = requestUrlRoot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
requestUrlRoot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
public com.google.protobuf.ByteString
getRequestUrlRootBytes() {
java.lang.Object ref = requestUrlRoot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
requestUrlRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
public Builder setRequestUrlRoot(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
requestUrlRoot_ = value;
onChanged();
return this;
}
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
public Builder clearRequestUrlRoot() {
requestUrlRoot_ = getDefaultInstance().getRequestUrlRoot();
onChanged();
return this;
}
/**
*
* Optional. Request URI. Example: "/Metrics/IdentifyApp"
*
*
* string request_url_root = 5;
*/
public Builder setRequestUrlRootBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
requestUrlRoot_ = value;
onChanged();
return this;
}
private java.lang.Object referralUrl_ = "";
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
public java.lang.String getReferralUrl() {
java.lang.Object ref = referralUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referralUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
public com.google.protobuf.ByteString
getReferralUrlBytes() {
java.lang.Object ref = referralUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
referralUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
public Builder setReferralUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
referralUrl_ = value;
onChanged();
return this;
}
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
public Builder clearReferralUrl() {
referralUrl_ = getDefaultInstance().getReferralUrl();
onChanged();
return this;
}
/**
*
* Optional. Value of the "referer" header. Example: "https://api.stackify.com/Metrics/IdentifyApp"
*
*
* string referral_url = 6;
*/
public Builder setReferralUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
referralUrl_ = value;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> headers_;
private com.google.protobuf.MapField
internalGetHeaders() {
if (headers_ == null) {
return com.google.protobuf.MapField.emptyMapField(
HeadersDefaultEntryHolder.defaultEntry);
}
return headers_;
}
private com.google.protobuf.MapField
internalGetMutableHeaders() {
onChanged();;
if (headers_ == null) {
headers_ = com.google.protobuf.MapField.newMapField(
HeadersDefaultEntryHolder.defaultEntry);
}
if (!headers_.isMutable()) {
headers_ = headers_.copy();
}
return headers_;
}
public int getHeadersCount() {
return internalGetHeaders().getMap().size();
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public boolean containsHeaders(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetHeaders().getMap().containsKey(key);
}
/**
* Use {@link #getHeadersMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getHeaders() {
return getHeadersMap();
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public java.util.Map getHeadersMap() {
return internalGetHeaders().getMap();
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public java.lang.String getHeadersOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetHeaders().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public java.lang.String getHeadersOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetHeaders().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearHeaders() {
internalGetMutableHeaders().getMutableMap()
.clear();
return this;
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public Builder removeHeaders(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableHeaders().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableHeaders() {
return internalGetMutableHeaders().getMutableMap();
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public Builder putHeaders(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableHeaders().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Optional. HTTP header name/value pairs. Example: {"Content-Type": "application/json", "Content-Encoding":"gzip"}
*
*
* map<string, string> headers = 7;
*/
public Builder putAllHeaders(
java.util.Map values) {
internalGetMutableHeaders().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> cookies_;
private com.google.protobuf.MapField
internalGetCookies() {
if (cookies_ == null) {
return com.google.protobuf.MapField.emptyMapField(
CookiesDefaultEntryHolder.defaultEntry);
}
return cookies_;
}
private com.google.protobuf.MapField
internalGetMutableCookies() {
onChanged();;
if (cookies_ == null) {
cookies_ = com.google.protobuf.MapField.newMapField(
CookiesDefaultEntryHolder.defaultEntry);
}
if (!cookies_.isMutable()) {
cookies_ = cookies_.copy();
}
return cookies_;
}
public int getCookiesCount() {
return internalGetCookies().getMap().size();
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public boolean containsCookies(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetCookies().getMap().containsKey(key);
}
/**
* Use {@link #getCookiesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getCookies() {
return getCookiesMap();
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public java.util.Map getCookiesMap() {
return internalGetCookies().getMap();
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public java.lang.String getCookiesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCookies().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public java.lang.String getCookiesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetCookies().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearCookies() {
internalGetMutableCookies().getMutableMap()
.clear();
return this;
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public Builder removeCookies(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableCookies().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableCookies() {
return internalGetMutableCookies().getMutableMap();
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public Builder putCookies(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableCookies().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Optional. HTTP cookie name/value pairs. We mask all cookie values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> cookies = 8;
*/
public Builder putAllCookies(
java.util.Map values) {
internalGetMutableCookies().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> querystring_;
private com.google.protobuf.MapField
internalGetQuerystring() {
if (querystring_ == null) {
return com.google.protobuf.MapField.emptyMapField(
QuerystringDefaultEntryHolder.defaultEntry);
}
return querystring_;
}
private com.google.protobuf.MapField
internalGetMutableQuerystring() {
onChanged();;
if (querystring_ == null) {
querystring_ = com.google.protobuf.MapField.newMapField(
QuerystringDefaultEntryHolder.defaultEntry);
}
if (!querystring_.isMutable()) {
querystring_ = querystring_.copy();
}
return querystring_;
}
public int getQuerystringCount() {
return internalGetQuerystring().getMap().size();
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public boolean containsQuerystring(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetQuerystring().getMap().containsKey(key);
}
/**
* Use {@link #getQuerystringMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getQuerystring() {
return getQuerystringMap();
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public java.util.Map getQuerystringMap() {
return internalGetQuerystring().getMap();
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public java.lang.String getQuerystringOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetQuerystring().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public java.lang.String getQuerystringOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetQuerystring().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearQuerystring() {
internalGetMutableQuerystring().getMutableMap()
.clear();
return this;
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public Builder removeQuerystring(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableQuerystring().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableQuerystring() {
return internalGetMutableQuerystring().getMutableMap();
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public Builder putQuerystring(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableQuerystring().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Optional. HTTP query string name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> querystring = 9;
*/
public Builder putAllQuerystring(
java.util.Map values) {
internalGetMutableQuerystring().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> postData_;
private com.google.protobuf.MapField
internalGetPostData() {
if (postData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
PostDataDefaultEntryHolder.defaultEntry);
}
return postData_;
}
private com.google.protobuf.MapField
internalGetMutablePostData() {
onChanged();;
if (postData_ == null) {
postData_ = com.google.protobuf.MapField.newMapField(
PostDataDefaultEntryHolder.defaultEntry);
}
if (!postData_.isMutable()) {
postData_ = postData_.copy();
}
return postData_;
}
public int getPostDataCount() {
return internalGetPostData().getMap().size();
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public boolean containsPostData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetPostData().getMap().containsKey(key);
}
/**
* Use {@link #getPostDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getPostData() {
return getPostDataMap();
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public java.util.Map getPostDataMap() {
return internalGetPostData().getMap();
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public java.lang.String getPostDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPostData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public java.lang.String getPostDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetPostData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearPostData() {
internalGetMutablePostData().getMutableMap()
.clear();
return this;
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public Builder removePostData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutablePostData().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutablePostData() {
return internalGetMutablePostData().getMutableMap();
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public Builder putPostData(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutablePostData().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Optional. HTTP post data name/value pairs. Example: {"name": "value"}
*
*
* map<string, string> post_data = 10;
*/
public Builder putAllPostData(
java.util.Map values) {
internalGetMutablePostData().getMutableMap()
.putAll(values);
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> sessionData_;
private com.google.protobuf.MapField
internalGetSessionData() {
if (sessionData_ == null) {
return com.google.protobuf.MapField.emptyMapField(
SessionDataDefaultEntryHolder.defaultEntry);
}
return sessionData_;
}
private com.google.protobuf.MapField
internalGetMutableSessionData() {
onChanged();;
if (sessionData_ == null) {
sessionData_ = com.google.protobuf.MapField.newMapField(
SessionDataDefaultEntryHolder.defaultEntry);
}
if (!sessionData_.isMutable()) {
sessionData_ = sessionData_.copy();
}
return sessionData_;
}
public int getSessionDataCount() {
return internalGetSessionData().getMap().size();
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public boolean containsSessionData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetSessionData().getMap().containsKey(key);
}
/**
* Use {@link #getSessionDataMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getSessionData() {
return getSessionDataMap();
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public java.util.Map getSessionDataMap() {
return internalGetSessionData().getMap();
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public java.lang.String getSessionDataOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetSessionData().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public java.lang.String getSessionDataOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetSessionData().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearSessionData() {
internalGetMutableSessionData().getMutableMap()
.clear();
return this;
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public Builder removeSessionData(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableSessionData().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableSessionData() {
return internalGetMutableSessionData().getMutableMap();
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public Builder putSessionData(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableSessionData().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Optional. HTTP session name/value pairs. We mask all session values with "X-MASKED-X". Example: {"name": "X-MASKED-X"}
*
*
* map<string, string> session_data = 11;
*/
public Builder putAllSessionData(
java.util.Map values) {
internalGetMutableSessionData().getMutableMap()
.putAll(values);
return this;
}
private java.lang.Object postDataRaw_ = "";
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
public java.lang.String getPostDataRaw() {
java.lang.Object ref = postDataRaw_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
postDataRaw_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
public com.google.protobuf.ByteString
getPostDataRawBytes() {
java.lang.Object ref = postDataRaw_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
postDataRaw_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
public Builder setPostDataRaw(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
postDataRaw_ = value;
onChanged();
return this;
}
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
public Builder clearPostDataRaw() {
postDataRaw_ = getDefaultInstance().getPostDataRaw();
onChanged();
return this;
}
/**
*
* Optional. HTTP raw post data. Example: "name=value"
*
*
* string post_data_raw = 12;
*/
public Builder setPostDataRawBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
postDataRaw_ = value;
onChanged();
return this;
}
private java.lang.Object mvcAction_ = "";
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
public java.lang.String getMvcAction() {
java.lang.Object ref = mvcAction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mvcAction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
public com.google.protobuf.ByteString
getMvcActionBytes() {
java.lang.Object ref = mvcAction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mvcAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
public Builder setMvcAction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mvcAction_ = value;
onChanged();
return this;
}
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
public Builder clearMvcAction() {
mvcAction_ = getDefaultInstance().getMvcAction();
onChanged();
return this;
}
/**
*
* Optional. MVC area. Example: "API"
*
*
* string mvc_action = 13;
*/
public Builder setMvcActionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mvcAction_ = value;
onChanged();
return this;
}
private java.lang.Object mvcController_ = "";
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
public java.lang.String getMvcController() {
java.lang.Object ref = mvcController_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mvcController_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
public com.google.protobuf.ByteString
getMvcControllerBytes() {
java.lang.Object ref = mvcController_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mvcController_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
public Builder setMvcController(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mvcController_ = value;
onChanged();
return this;
}
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
public Builder clearMvcController() {
mvcController_ = getDefaultInstance().getMvcController();
onChanged();
return this;
}
/**
*
* Optional. MVC controller. Example: "Metrics"
*
*
* string mvc_controller = 14;
*/
public Builder setMvcControllerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mvcController_ = value;
onChanged();
return this;
}
private java.lang.Object mvcArea_ = "";
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
public java.lang.String getMvcArea() {
java.lang.Object ref = mvcArea_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mvcArea_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
public com.google.protobuf.ByteString
getMvcAreaBytes() {
java.lang.Object ref = mvcArea_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
mvcArea_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
public Builder setMvcArea(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mvcArea_ = value;
onChanged();
return this;
}
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
public Builder clearMvcArea() {
mvcArea_ = getDefaultInstance().getMvcArea();
onChanged();
return this;
}
/**
*
* Optional. MVC action. Example: "IdentifyApp"
*
*
* string mvc_area = 15;
*/
public Builder setMvcAreaBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mvcArea_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Log.Error.WebRequestDetail)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Log.Error.WebRequestDetail)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public WebRequestDetail parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new WebRequestDetail(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int ENVIRONMENT_DETAIL_FIELD_NUMBER = 1;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail environmentDetail_;
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public boolean hasEnvironmentDetail() {
return environmentDetail_ != null;
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail getEnvironmentDetail() {
return environmentDetail_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.getDefaultInstance() : environmentDetail_;
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetailOrBuilder getEnvironmentDetailOrBuilder() {
return getEnvironmentDetail();
}
public static final int DATE_MILLIS_FIELD_NUMBER = 2;
private long dateMillis_;
/**
*
* Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 2;
*/
public long getDateMillis() {
return dateMillis_;
}
public static final int ERROR_ITEM_FIELD_NUMBER = 3;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem errorItem_;
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public boolean hasErrorItem() {
return errorItem_ != null;
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getErrorItem() {
return errorItem_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance() : errorItem_;
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder getErrorItemOrBuilder() {
return getErrorItem();
}
public static final int WEB_REQUEST_DETAIL_FIELD_NUMBER = 4;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail webRequestDetail_;
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public boolean hasWebRequestDetail() {
return webRequestDetail_ != null;
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail getWebRequestDetail() {
return webRequestDetail_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.getDefaultInstance() : webRequestDetail_;
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetailOrBuilder getWebRequestDetailOrBuilder() {
return getWebRequestDetail();
}
public static final int SERVER_VARIABLES_FIELD_NUMBER = 5;
private static final class ServerVariablesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_ServerVariablesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> serverVariables_;
private com.google.protobuf.MapField
internalGetServerVariables() {
if (serverVariables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ServerVariablesDefaultEntryHolder.defaultEntry);
}
return serverVariables_;
}
public int getServerVariablesCount() {
return internalGetServerVariables().getMap().size();
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public boolean containsServerVariables(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetServerVariables().getMap().containsKey(key);
}
/**
* Use {@link #getServerVariablesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getServerVariables() {
return getServerVariablesMap();
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public java.util.Map getServerVariablesMap() {
return internalGetServerVariables().getMap();
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public java.lang.String getServerVariablesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetServerVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public java.lang.String getServerVariablesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetServerVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int CUSTOMER_NAME_FIELD_NUMBER = 6;
private volatile java.lang.Object customerName_;
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
public java.lang.String getCustomerName() {
java.lang.Object ref = customerName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerName_ = s;
return s;
}
}
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
public com.google.protobuf.ByteString
getCustomerNameBytes() {
java.lang.Object ref = customerName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USERNAME_FIELD_NUMBER = 7;
private volatile java.lang.Object username_;
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
username_ = s;
return s;
}
}
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (environmentDetail_ != null) {
output.writeMessage(1, getEnvironmentDetail());
}
if (dateMillis_ != 0L) {
output.writeInt64(2, dateMillis_);
}
if (errorItem_ != null) {
output.writeMessage(3, getErrorItem());
}
if (webRequestDetail_ != null) {
output.writeMessage(4, getWebRequestDetail());
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetServerVariables(),
ServerVariablesDefaultEntryHolder.defaultEntry,
5);
if (!getCustomerNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, customerName_);
}
if (!getUsernameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, username_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (environmentDetail_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getEnvironmentDetail());
}
if (dateMillis_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, dateMillis_);
}
if (errorItem_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getErrorItem());
}
if (webRequestDetail_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getWebRequestDetail());
}
for (java.util.Map.Entry entry
: internalGetServerVariables().getMap().entrySet()) {
com.google.protobuf.MapEntry
serverVariables__ = ServerVariablesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, serverVariables__);
}
if (!getCustomerNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, customerName_);
}
if (!getUsernameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, username_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error) obj;
if (hasEnvironmentDetail() != other.hasEnvironmentDetail()) return false;
if (hasEnvironmentDetail()) {
if (!getEnvironmentDetail()
.equals(other.getEnvironmentDetail())) return false;
}
if (getDateMillis()
!= other.getDateMillis()) return false;
if (hasErrorItem() != other.hasErrorItem()) return false;
if (hasErrorItem()) {
if (!getErrorItem()
.equals(other.getErrorItem())) return false;
}
if (hasWebRequestDetail() != other.hasWebRequestDetail()) return false;
if (hasWebRequestDetail()) {
if (!getWebRequestDetail()
.equals(other.getWebRequestDetail())) return false;
}
if (!internalGetServerVariables().equals(
other.internalGetServerVariables())) return false;
if (!getCustomerName()
.equals(other.getCustomerName())) return false;
if (!getUsername()
.equals(other.getUsername())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasEnvironmentDetail()) {
hash = (37 * hash) + ENVIRONMENT_DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getEnvironmentDetail().hashCode();
}
hash = (37 * hash) + DATE_MILLIS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDateMillis());
if (hasErrorItem()) {
hash = (37 * hash) + ERROR_ITEM_FIELD_NUMBER;
hash = (53 * hash) + getErrorItem().hashCode();
}
if (hasWebRequestDetail()) {
hash = (37 * hash) + WEB_REQUEST_DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getWebRequestDetail().hashCode();
}
if (!internalGetServerVariables().getMap().isEmpty()) {
hash = (37 * hash) + SERVER_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + internalGetServerVariables().hashCode();
}
hash = (37 * hash) + CUSTOMER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getCustomerName().hashCode();
hash = (37 * hash) + USERNAME_FIELD_NUMBER;
hash = (53 * hash) + getUsername().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Log.Error}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Log.Error)
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.ErrorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 5:
return internalGetServerVariables();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 5:
return internalGetMutableServerVariables();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (environmentDetailBuilder_ == null) {
environmentDetail_ = null;
} else {
environmentDetail_ = null;
environmentDetailBuilder_ = null;
}
dateMillis_ = 0L;
if (errorItemBuilder_ == null) {
errorItem_ = null;
} else {
errorItem_ = null;
errorItemBuilder_ = null;
}
if (webRequestDetailBuilder_ == null) {
webRequestDetail_ = null;
} else {
webRequestDetail_ = null;
webRequestDetailBuilder_ = null;
}
internalGetMutableServerVariables().clear();
customerName_ = "";
username_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_Error_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error(this);
int from_bitField0_ = bitField0_;
if (environmentDetailBuilder_ == null) {
result.environmentDetail_ = environmentDetail_;
} else {
result.environmentDetail_ = environmentDetailBuilder_.build();
}
result.dateMillis_ = dateMillis_;
if (errorItemBuilder_ == null) {
result.errorItem_ = errorItem_;
} else {
result.errorItem_ = errorItemBuilder_.build();
}
if (webRequestDetailBuilder_ == null) {
result.webRequestDetail_ = webRequestDetail_;
} else {
result.webRequestDetail_ = webRequestDetailBuilder_.build();
}
result.serverVariables_ = internalGetServerVariables();
result.serverVariables_.makeImmutable();
result.customerName_ = customerName_;
result.username_ = username_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.getDefaultInstance()) return this;
if (other.hasEnvironmentDetail()) {
mergeEnvironmentDetail(other.getEnvironmentDetail());
}
if (other.getDateMillis() != 0L) {
setDateMillis(other.getDateMillis());
}
if (other.hasErrorItem()) {
mergeErrorItem(other.getErrorItem());
}
if (other.hasWebRequestDetail()) {
mergeWebRequestDetail(other.getWebRequestDetail());
}
internalGetMutableServerVariables().mergeFrom(
other.internalGetServerVariables());
if (!other.getCustomerName().isEmpty()) {
customerName_ = other.customerName_;
onChanged();
}
if (!other.getUsername().isEmpty()) {
username_ = other.username_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail environmentDetail_;
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetailOrBuilder> environmentDetailBuilder_;
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public boolean hasEnvironmentDetail() {
return environmentDetailBuilder_ != null || environmentDetail_ != null;
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail getEnvironmentDetail() {
if (environmentDetailBuilder_ == null) {
return environmentDetail_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.getDefaultInstance() : environmentDetail_;
} else {
return environmentDetailBuilder_.getMessage();
}
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public Builder setEnvironmentDetail(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail value) {
if (environmentDetailBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
environmentDetail_ = value;
onChanged();
} else {
environmentDetailBuilder_.setMessage(value);
}
return this;
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public Builder setEnvironmentDetail(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder builderForValue) {
if (environmentDetailBuilder_ == null) {
environmentDetail_ = builderForValue.build();
onChanged();
} else {
environmentDetailBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public Builder mergeEnvironmentDetail(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail value) {
if (environmentDetailBuilder_ == null) {
if (environmentDetail_ != null) {
environmentDetail_ =
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.newBuilder(environmentDetail_).mergeFrom(value).buildPartial();
} else {
environmentDetail_ = value;
}
onChanged();
} else {
environmentDetailBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public Builder clearEnvironmentDetail() {
if (environmentDetailBuilder_ == null) {
environmentDetail_ = null;
onChanged();
} else {
environmentDetail_ = null;
environmentDetailBuilder_ = null;
}
return this;
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder getEnvironmentDetailBuilder() {
onChanged();
return getEnvironmentDetailFieldBuilder().getBuilder();
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetailOrBuilder getEnvironmentDetailOrBuilder() {
if (environmentDetailBuilder_ != null) {
return environmentDetailBuilder_.getMessageOrBuilder();
} else {
return environmentDetail_ == null ?
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.getDefaultInstance() : environmentDetail_;
}
}
/**
*
* Device, application and environment details.
*
*
* .stackify.LogGroup.Log.Error.EnvironmentDetail environment_detail = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetailOrBuilder>
getEnvironmentDetailFieldBuilder() {
if (environmentDetailBuilder_ == null) {
environmentDetailBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetail.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.EnvironmentDetailOrBuilder>(
getEnvironmentDetail(),
getParentForChildren(),
isClean());
environmentDetail_ = null;
}
return environmentDetailBuilder_;
}
private long dateMillis_ ;
/**
*
* Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 2;
*/
public long getDateMillis() {
return dateMillis_;
}
/**
*
* Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 2;
*/
public Builder setDateMillis(long value) {
dateMillis_ = value;
onChanged();
return this;
}
/**
*
* Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 2;
*/
public Builder clearDateMillis() {
dateMillis_ = 0L;
onChanged();
return this;
}
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem errorItem_;
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder> errorItemBuilder_;
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public boolean hasErrorItem() {
return errorItemBuilder_ != null || errorItem_ != null;
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem getErrorItem() {
if (errorItemBuilder_ == null) {
return errorItem_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance() : errorItem_;
} else {
return errorItemBuilder_.getMessage();
}
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public Builder setErrorItem(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem value) {
if (errorItemBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
errorItem_ = value;
onChanged();
} else {
errorItemBuilder_.setMessage(value);
}
return this;
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public Builder setErrorItem(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder builderForValue) {
if (errorItemBuilder_ == null) {
errorItem_ = builderForValue.build();
onChanged();
} else {
errorItemBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public Builder mergeErrorItem(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem value) {
if (errorItemBuilder_ == null) {
if (errorItem_ != null) {
errorItem_ =
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.newBuilder(errorItem_).mergeFrom(value).buildPartial();
} else {
errorItem_ = value;
}
onChanged();
} else {
errorItemBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public Builder clearErrorItem() {
if (errorItemBuilder_ == null) {
errorItem_ = null;
onChanged();
} else {
errorItem_ = null;
errorItemBuilder_ = null;
}
return this;
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder getErrorItemBuilder() {
onChanged();
return getErrorItemFieldBuilder().getBuilder();
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder getErrorItemOrBuilder() {
if (errorItemBuilder_ != null) {
return errorItemBuilder_.getMessageOrBuilder();
} else {
return errorItem_ == null ?
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.getDefaultInstance() : errorItem_;
}
}
/**
*
* Exception details.
*
*
* .stackify.LogGroup.Log.Error.ErrorItem error_item = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder>
getErrorItemFieldBuilder() {
if (errorItemBuilder_ == null) {
errorItemBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItem.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.ErrorItemOrBuilder>(
getErrorItem(),
getParentForChildren(),
isClean());
errorItem_ = null;
}
return errorItemBuilder_;
}
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail webRequestDetail_;
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetailOrBuilder> webRequestDetailBuilder_;
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public boolean hasWebRequestDetail() {
return webRequestDetailBuilder_ != null || webRequestDetail_ != null;
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail getWebRequestDetail() {
if (webRequestDetailBuilder_ == null) {
return webRequestDetail_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.getDefaultInstance() : webRequestDetail_;
} else {
return webRequestDetailBuilder_.getMessage();
}
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public Builder setWebRequestDetail(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail value) {
if (webRequestDetailBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
webRequestDetail_ = value;
onChanged();
} else {
webRequestDetailBuilder_.setMessage(value);
}
return this;
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public Builder setWebRequestDetail(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder builderForValue) {
if (webRequestDetailBuilder_ == null) {
webRequestDetail_ = builderForValue.build();
onChanged();
} else {
webRequestDetailBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public Builder mergeWebRequestDetail(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail value) {
if (webRequestDetailBuilder_ == null) {
if (webRequestDetail_ != null) {
webRequestDetail_ =
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.newBuilder(webRequestDetail_).mergeFrom(value).buildPartial();
} else {
webRequestDetail_ = value;
}
onChanged();
} else {
webRequestDetailBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public Builder clearWebRequestDetail() {
if (webRequestDetailBuilder_ == null) {
webRequestDetail_ = null;
onChanged();
} else {
webRequestDetail_ = null;
webRequestDetailBuilder_ = null;
}
return this;
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder getWebRequestDetailBuilder() {
onChanged();
return getWebRequestDetailFieldBuilder().getBuilder();
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetailOrBuilder getWebRequestDetailOrBuilder() {
if (webRequestDetailBuilder_ != null) {
return webRequestDetailBuilder_.getMessageOrBuilder();
} else {
return webRequestDetail_ == null ?
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.getDefaultInstance() : webRequestDetail_;
}
}
/**
*
* Optional. Web request details.
*
*
* .stackify.LogGroup.Log.Error.WebRequestDetail web_request_detail = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetailOrBuilder>
getWebRequestDetailFieldBuilder() {
if (webRequestDetailBuilder_ == null) {
webRequestDetailBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetail.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.WebRequestDetailOrBuilder>(
getWebRequestDetail(),
getParentForChildren(),
isClean());
webRequestDetail_ = null;
}
return webRequestDetailBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> serverVariables_;
private com.google.protobuf.MapField
internalGetServerVariables() {
if (serverVariables_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ServerVariablesDefaultEntryHolder.defaultEntry);
}
return serverVariables_;
}
private com.google.protobuf.MapField
internalGetMutableServerVariables() {
onChanged();;
if (serverVariables_ == null) {
serverVariables_ = com.google.protobuf.MapField.newMapField(
ServerVariablesDefaultEntryHolder.defaultEntry);
}
if (!serverVariables_.isMutable()) {
serverVariables_ = serverVariables_.copy();
}
return serverVariables_;
}
public int getServerVariablesCount() {
return internalGetServerVariables().getMap().size();
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public boolean containsServerVariables(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetServerVariables().getMap().containsKey(key);
}
/**
* Use {@link #getServerVariablesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getServerVariables() {
return getServerVariablesMap();
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public java.util.Map getServerVariablesMap() {
return internalGetServerVariables().getMap();
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public java.lang.String getServerVariablesOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetServerVariables().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public java.lang.String getServerVariablesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetServerVariables().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearServerVariables() {
internalGetMutableServerVariables().getMutableMap()
.clear();
return this;
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public Builder removeServerVariables(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableServerVariables().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableServerVariables() {
return internalGetMutableServerVariables().getMutableMap();
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public Builder putServerVariables(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableServerVariables().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Optional. Server data name/value pairs. Example: {"java.runtime.name": "OpenJDK Runtime Environment", "os.name": "Linux", "user.timezone": "UTC", "java.vendor": "Oracle Corporation"}
*
*
* map<string, string> server_variables = 5;
*/
public Builder putAllServerVariables(
java.util.Map values) {
internalGetMutableServerVariables().getMutableMap()
.putAll(values);
return this;
}
private java.lang.Object customerName_ = "";
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
public java.lang.String getCustomerName() {
java.lang.Object ref = customerName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
customerName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
public com.google.protobuf.ByteString
getCustomerNameBytes() {
java.lang.Object ref = customerName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
customerName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
public Builder setCustomerName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
customerName_ = value;
onChanged();
return this;
}
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
public Builder clearCustomerName() {
customerName_ = getDefaultInstance().getCustomerName();
onChanged();
return this;
}
/**
*
* Optional. Customer/client name. Example: "Stackify"
*
*
* string customer_name = 6;
*/
public Builder setCustomerNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
customerName_ = value;
onChanged();
return this;
}
private java.lang.Object username_ = "";
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
public java.lang.String getUsername() {
java.lang.Object ref = username_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
username_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
public com.google.protobuf.ByteString
getUsernameBytes() {
java.lang.Object ref = username_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
username_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
public Builder setUsername(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
username_ = value;
onChanged();
return this;
}
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
public Builder clearUsername() {
username_ = getDefaultInstance().getUsername();
onChanged();
return this;
}
/**
*
* Optional. User name. Example: "test-user@stackify.com
*
*
* string username = 7;
*/
public Builder setUsernameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
username_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Log.Error)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Log.Error)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Error parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Error(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int MESSAGE_FIELD_NUMBER = 1;
private volatile java.lang.Object message_;
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
}
}
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATA_FIELD_NUMBER = 2;
private volatile java.lang.Object data_;
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
}
}
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int THREAD_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object threadName_;
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
public java.lang.String getThreadName() {
java.lang.Object ref = threadName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
threadName_ = s;
return s;
}
}
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
public com.google.protobuf.ByteString
getThreadNameBytes() {
java.lang.Object ref = threadName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
threadName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DATE_MILLIS_FIELD_NUMBER = 4;
private long dateMillis_;
/**
*
* REQUIRED Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 4;
*/
public long getDateMillis() {
return dateMillis_;
}
public static final int LEVEL_FIELD_NUMBER = 5;
private volatile java.lang.Object level_;
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
public java.lang.String getLevel() {
java.lang.Object ref = level_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
level_ = s;
return s;
}
}
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
public com.google.protobuf.ByteString
getLevelBytes() {
java.lang.Object ref = level_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
level_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRANSACTION_ID_FIELD_NUMBER = 6;
private volatile java.lang.Object transactionId_;
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
public java.lang.String getTransactionId() {
java.lang.Object ref = transactionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
transactionId_ = s;
return s;
}
}
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
public com.google.protobuf.ByteString
getTransactionIdBytes() {
java.lang.Object ref = transactionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
transactionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_METHOD_FIELD_NUMBER = 7;
private volatile java.lang.Object sourceMethod_;
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
public java.lang.String getSourceMethod() {
java.lang.Object ref = sourceMethod_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceMethod_ = s;
return s;
}
}
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
public com.google.protobuf.ByteString
getSourceMethodBytes() {
java.lang.Object ref = sourceMethod_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_LINE_FIELD_NUMBER = 8;
private int sourceLine_;
/**
*
* Optional. Line number. Example: 57
*
*
* int32 source_line = 8;
*/
public int getSourceLine() {
return sourceLine_;
}
public static final int ID_FIELD_NUMBER = 9;
private volatile java.lang.Object id_;
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TAGS_FIELD_NUMBER = 10;
private com.google.protobuf.LazyStringList tags_;
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public com.google.protobuf.ProtocolStringList
getTagsList() {
return tags_;
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public int getTagsCount() {
return tags_.size();
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
public static final int ERROR_FIELD_NUMBER = 11;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error error_;
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public boolean hasError() {
return error_ != null;
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error getError() {
return error_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.getDefaultInstance() : error_;
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.ErrorOrBuilder getErrorOrBuilder() {
return getError();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getMessageBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, message_);
}
if (!getDataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, data_);
}
if (!getThreadNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, threadName_);
}
if (dateMillis_ != 0L) {
output.writeInt64(4, dateMillis_);
}
if (!getLevelBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, level_);
}
if (!getTransactionIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, transactionId_);
}
if (!getSourceMethodBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, sourceMethod_);
}
if (sourceLine_ != 0) {
output.writeInt32(8, sourceLine_);
}
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 9, id_);
}
for (int i = 0; i < tags_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, tags_.getRaw(i));
}
if (error_ != null) {
output.writeMessage(11, getError());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getMessageBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, message_);
}
if (!getDataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, data_);
}
if (!getThreadNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, threadName_);
}
if (dateMillis_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, dateMillis_);
}
if (!getLevelBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, level_);
}
if (!getTransactionIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, transactionId_);
}
if (!getSourceMethodBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, sourceMethod_);
}
if (sourceLine_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(8, sourceLine_);
}
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(9, id_);
}
{
int dataSize = 0;
for (int i = 0; i < tags_.size(); i++) {
dataSize += computeStringSizeNoTag(tags_.getRaw(i));
}
size += dataSize;
size += 1 * getTagsList().size();
}
if (error_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getError());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup.Log other = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log) obj;
if (!getMessage()
.equals(other.getMessage())) return false;
if (!getData()
.equals(other.getData())) return false;
if (!getThreadName()
.equals(other.getThreadName())) return false;
if (getDateMillis()
!= other.getDateMillis()) return false;
if (!getLevel()
.equals(other.getLevel())) return false;
if (!getTransactionId()
.equals(other.getTransactionId())) return false;
if (!getSourceMethod()
.equals(other.getSourceMethod())) return false;
if (getSourceLine()
!= other.getSourceLine()) return false;
if (!getId()
.equals(other.getId())) return false;
if (!getTagsList()
.equals(other.getTagsList())) return false;
if (hasError() != other.hasError()) return false;
if (hasError()) {
if (!getError()
.equals(other.getError())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
hash = (37 * hash) + DATA_FIELD_NUMBER;
hash = (53 * hash) + getData().hashCode();
hash = (37 * hash) + THREAD_NAME_FIELD_NUMBER;
hash = (53 * hash) + getThreadName().hashCode();
hash = (37 * hash) + DATE_MILLIS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDateMillis());
hash = (37 * hash) + LEVEL_FIELD_NUMBER;
hash = (53 * hash) + getLevel().hashCode();
hash = (37 * hash) + TRANSACTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getTransactionId().hashCode();
hash = (37 * hash) + SOURCE_METHOD_FIELD_NUMBER;
hash = (53 * hash) + getSourceMethod().hashCode();
hash = (37 * hash) + SOURCE_LINE_FIELD_NUMBER;
hash = (53 * hash) + getSourceLine();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
if (getTagsCount() > 0) {
hash = (37 * hash) + TAGS_FIELD_NUMBER;
hash = (53 * hash) + getTagsList().hashCode();
}
if (hasError()) {
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup.Log prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup.Log}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup.Log)
com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.Log.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
message_ = "";
data_ = "";
threadName_ = "";
dateMillis_ = 0L;
level_ = "";
transactionId_ = "";
sourceMethod_ = "";
sourceLine_ = 0;
id_ = "";
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (errorBuilder_ == null) {
error_ = null;
} else {
error_ = null;
errorBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_Log_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.Log.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log build() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log result = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log(this);
int from_bitField0_ = bitField0_;
result.message_ = message_;
result.data_ = data_;
result.threadName_ = threadName_;
result.dateMillis_ = dateMillis_;
result.level_ = level_;
result.transactionId_ = transactionId_;
result.sourceMethod_ = sourceMethod_;
result.sourceLine_ = sourceLine_;
result.id_ = id_;
if (((bitField0_ & 0x00000001) != 0)) {
tags_ = tags_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.tags_ = tags_;
if (errorBuilder_ == null) {
result.error_ = error_;
} else {
result.error_ = errorBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup.Log) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup.Log)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup.Log other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.Log.getDefaultInstance()) return this;
if (!other.getMessage().isEmpty()) {
message_ = other.message_;
onChanged();
}
if (!other.getData().isEmpty()) {
data_ = other.data_;
onChanged();
}
if (!other.getThreadName().isEmpty()) {
threadName_ = other.threadName_;
onChanged();
}
if (other.getDateMillis() != 0L) {
setDateMillis(other.getDateMillis());
}
if (!other.getLevel().isEmpty()) {
level_ = other.level_;
onChanged();
}
if (!other.getTransactionId().isEmpty()) {
transactionId_ = other.transactionId_;
onChanged();
}
if (!other.getSourceMethod().isEmpty()) {
sourceMethod_ = other.sourceMethod_;
onChanged();
}
if (other.getSourceLine() != 0) {
setSourceLine(other.getSourceLine());
}
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.tags_.isEmpty()) {
if (tags_.isEmpty()) {
tags_ = other.tags_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureTagsIsMutable();
tags_.addAll(other.tags_);
}
onChanged();
}
if (other.hasError()) {
mergeError(other.getError());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup.Log parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup.Log) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object message_ = "";
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
public java.lang.String getMessage() {
java.lang.Object ref = message_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
message_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
public com.google.protobuf.ByteString
getMessageBytes() {
java.lang.Object ref = message_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
message_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
public Builder setMessage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
public Builder clearMessage() {
message_ = getDefaultInstance().getMessage();
onChanged();
return this;
}
/**
*
* REQUIRED The log message. Example: "Example debug message"
*
*
* string message = 1;
*/
public Builder setMessageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
message_ = value;
onChanged();
return this;
}
private java.lang.Object data_ = "";
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
public java.lang.String getData() {
java.lang.Object ref = data_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
data_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
public com.google.protobuf.ByteString
getDataBytes() {
java.lang.Object ref = data_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
data_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
public Builder setData(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
data_ = value;
onChanged();
return this;
}
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
public Builder clearData() {
data_ = getDefaultInstance().getData();
onChanged();
return this;
}
/**
*
* Optional. Additional JSON metadata about the log event. Special characters need to be escaped. Example: "{"Key1":"Value1","Key2":"Value2"}"
*
*
* string data = 2;
*/
public Builder setDataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
data_ = value;
onChanged();
return this;
}
private java.lang.Object threadName_ = "";
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
public java.lang.String getThreadName() {
java.lang.Object ref = threadName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
threadName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
public com.google.protobuf.ByteString
getThreadNameBytes() {
java.lang.Object ref = threadName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
threadName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
public Builder setThreadName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
threadName_ = value;
onChanged();
return this;
}
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
public Builder clearThreadName() {
threadName_ = getDefaultInstance().getThreadName();
onChanged();
return this;
}
/**
*
* Optional. The thread name. Example: "main"
*
*
* string thread_name = 3;
*/
public Builder setThreadNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
threadName_ = value;
onChanged();
return this;
}
private long dateMillis_ ;
/**
*
* REQUIRED Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 4;
*/
public long getDateMillis() {
return dateMillis_;
}
/**
*
* REQUIRED Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 4;
*/
public Builder setDateMillis(long value) {
dateMillis_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED Unix/POSIX/Epoch time with millisecond precision. Example: 1417535434194
*
*
* int64 date_millis = 4;
*/
public Builder clearDateMillis() {
dateMillis_ = 0L;
onChanged();
return this;
}
private java.lang.Object level_ = "";
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
public java.lang.String getLevel() {
java.lang.Object ref = level_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
level_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
public com.google.protobuf.ByteString
getLevelBytes() {
java.lang.Object ref = level_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
level_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
public Builder setLevel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
level_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
public Builder clearLevel() {
level_ = getDefaultInstance().getLevel();
onChanged();
return this;
}
/**
*
* REQUIRED The log level. Example: "debug"
*
*
* string level = 5;
*/
public Builder setLevelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
level_ = value;
onChanged();
return this;
}
private java.lang.Object transactionId_ = "";
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
public java.lang.String getTransactionId() {
java.lang.Object ref = transactionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
transactionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
public com.google.protobuf.ByteString
getTransactionIdBytes() {
java.lang.Object ref = transactionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
transactionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
public Builder setTransactionId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
transactionId_ = value;
onChanged();
return this;
}
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
public Builder clearTransactionId() {
transactionId_ = getDefaultInstance().getTransactionId();
onChanged();
return this;
}
/**
*
* Optional. Transaction identifier. Example: "c06570b2-ba9d-40be-8078-1394cf331159"
*
*
* string transaction_id = 6;
*/
public Builder setTransactionIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
transactionId_ = value;
onChanged();
return this;
}
private java.lang.Object sourceMethod_ = "";
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
public java.lang.String getSourceMethod() {
java.lang.Object ref = sourceMethod_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sourceMethod_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
public com.google.protobuf.ByteString
getSourceMethodBytes() {
java.lang.Object ref = sourceMethod_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
sourceMethod_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
public Builder setSourceMethod(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sourceMethod_ = value;
onChanged();
return this;
}
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
public Builder clearSourceMethod() {
sourceMethod_ = getDefaultInstance().getSourceMethod();
onChanged();
return this;
}
/**
*
* Optional. Fully qualified method name. Example: "com.stackify.error.test.StackifyErrorAppenderTest.main"
*
*
* string source_method = 7;
*/
public Builder setSourceMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sourceMethod_ = value;
onChanged();
return this;
}
private int sourceLine_ ;
/**
*
* Optional. Line number. Example: 57
*
*
* int32 source_line = 8;
*/
public int getSourceLine() {
return sourceLine_;
}
/**
*
* Optional. Line number. Example: 57
*
*
* int32 source_line = 8;
*/
public Builder setSourceLine(int value) {
sourceLine_ = value;
onChanged();
return this;
}
/**
*
* Optional. Line number. Example: 57
*
*
* int32 source_line = 8;
*/
public Builder clearSourceLine() {
sourceLine_ = 0;
onChanged();
return this;
}
private java.lang.Object id_ = "";
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Optional. Used for linking traces w/ log messages.
*
*
* string id = 9;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTagsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
tags_ = new com.google.protobuf.LazyStringArrayList(tags_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public com.google.protobuf.ProtocolStringList
getTagsList() {
return tags_.getUnmodifiableView();
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public int getTagsCount() {
return tags_.size();
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public java.lang.String getTags(int index) {
return tags_.get(index);
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public com.google.protobuf.ByteString
getTagsBytes(int index) {
return tags_.getByteString(index);
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public Builder setTags(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.set(index, value);
onChanged();
return this;
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public Builder addTags(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public Builder addAllTags(
java.lang.Iterable values) {
ensureTagsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tags_);
onChanged();
return this;
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public Builder clearTags() {
tags_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Optional.
*
*
* repeated string tags = 10;
*/
public Builder addTagsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureTagsIsMutable();
tags_.add(value);
onChanged();
return this;
}
private com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error error_;
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.ErrorOrBuilder> errorBuilder_;
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public boolean hasError() {
return errorBuilder_ != null || error_ != null;
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error getError() {
if (errorBuilder_ == null) {
return error_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.getDefaultInstance() : error_;
} else {
return errorBuilder_.getMessage();
}
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public Builder setError(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error value) {
if (errorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
error_ = value;
onChanged();
} else {
errorBuilder_.setMessage(value);
}
return this;
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public Builder setError(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder builderForValue) {
if (errorBuilder_ == null) {
error_ = builderForValue.build();
onChanged();
} else {
errorBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public Builder mergeError(com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error value) {
if (errorBuilder_ == null) {
if (error_ != null) {
error_ =
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.newBuilder(error_).mergeFrom(value).buildPartial();
} else {
error_ = value;
}
onChanged();
} else {
errorBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public Builder clearError() {
if (errorBuilder_ == null) {
error_ = null;
onChanged();
} else {
error_ = null;
errorBuilder_ = null;
}
return this;
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder getErrorBuilder() {
onChanged();
return getErrorFieldBuilder().getBuilder();
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.ErrorOrBuilder getErrorOrBuilder() {
if (errorBuilder_ != null) {
return errorBuilder_.getMessageOrBuilder();
} else {
return error_ == null ?
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.getDefaultInstance() : error_;
}
}
/**
*
* Optional. Exception details.
*
*
* .stackify.LogGroup.Log.Error error = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.ErrorOrBuilder>
getErrorFieldBuilder() {
if (errorBuilder_ == null) {
errorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Error.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.ErrorOrBuilder>(
getError(),
getParentForChildren(),
isClean());
error_ = null;
}
return errorBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup.Log)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup.Log)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup.Log DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup.Log();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup.Log getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Log parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Log(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int ENVIRONMENT_FIELD_NUMBER = 1;
private volatile java.lang.Object environment_;
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
public java.lang.String getEnvironment() {
java.lang.Object ref = environment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
environment_ = s;
return s;
}
}
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
public com.google.protobuf.ByteString
getEnvironmentBytes() {
java.lang.Object ref = environment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
environment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVER_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object serverName_;
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
public java.lang.String getServerName() {
java.lang.Object ref = serverName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serverName_ = s;
return s;
}
}
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
public com.google.protobuf.ByteString
getServerNameBytes() {
java.lang.Object ref = serverName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPLICATION_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object applicationName_;
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
public java.lang.String getApplicationName() {
java.lang.Object ref = applicationName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationName_ = s;
return s;
}
}
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
public com.google.protobuf.ByteString
getApplicationNameBytes() {
java.lang.Object ref = applicationName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int APPLICATION_LOCATION_FIELD_NUMBER = 4;
private volatile java.lang.Object applicationLocation_;
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
public java.lang.String getApplicationLocation() {
java.lang.Object ref = applicationLocation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationLocation_ = s;
return s;
}
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
public com.google.protobuf.ByteString
getApplicationLocationBytes() {
java.lang.Object ref = applicationLocation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationLocation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOGGER_FIELD_NUMBER = 5;
private volatile java.lang.Object logger_;
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
public java.lang.String getLogger() {
java.lang.Object ref = logger_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
logger_ = s;
return s;
}
}
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
public com.google.protobuf.ByteString
getLoggerBytes() {
java.lang.Object ref = logger_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logger_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PLATFORM_FIELD_NUMBER = 6;
private volatile java.lang.Object platform_;
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
public java.lang.String getPlatform() {
java.lang.Object ref = platform_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
platform_ = s;
return s;
}
}
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
public com.google.protobuf.ByteString
getPlatformBytes() {
java.lang.Object ref = platform_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platform_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOGS_FIELD_NUMBER = 7;
private java.util.List logs_;
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public java.util.List getLogsList() {
return logs_;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public java.util.List extends com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder>
getLogsOrBuilderList() {
return logs_;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public int getLogsCount() {
return logs_.size();
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log getLogs(int index) {
return logs_.get(index);
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder getLogsOrBuilder(
int index) {
return logs_.get(index);
}
public static final int CONTAINER_FIELD_NUMBER = 8;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Container container_;
/**
* .stackify.LogGroup.Container container = 8;
*/
public boolean hasContainer() {
return container_ != null;
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Container getContainer() {
return container_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Container.getDefaultInstance() : container_;
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.ContainerOrBuilder getContainerOrBuilder() {
return getContainer();
}
public static final int KUBERNETES_FIELD_NUMBER = 9;
private com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes kubernetes_;
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public boolean hasKubernetes() {
return kubernetes_ != null;
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes getKubernetes() {
return kubernetes_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.getDefaultInstance() : kubernetes_;
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.KubernetesOrBuilder getKubernetesOrBuilder() {
return getKubernetes();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getEnvironmentBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, environment_);
}
if (!getServerNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, serverName_);
}
if (!getApplicationNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, applicationName_);
}
if (!getApplicationLocationBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, applicationLocation_);
}
if (!getLoggerBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, logger_);
}
if (!getPlatformBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, platform_);
}
for (int i = 0; i < logs_.size(); i++) {
output.writeMessage(7, logs_.get(i));
}
if (container_ != null) {
output.writeMessage(8, getContainer());
}
if (kubernetes_ != null) {
output.writeMessage(9, getKubernetes());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getEnvironmentBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, environment_);
}
if (!getServerNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, serverName_);
}
if (!getApplicationNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, applicationName_);
}
if (!getApplicationLocationBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, applicationLocation_);
}
if (!getLoggerBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, logger_);
}
if (!getPlatformBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, platform_);
}
for (int i = 0; i < logs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, logs_.get(i));
}
if (container_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getContainer());
}
if (kubernetes_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getKubernetes());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.stackify.api.common.proto.StackifyProto.LogGroup)) {
return super.equals(obj);
}
com.stackify.api.common.proto.StackifyProto.LogGroup other = (com.stackify.api.common.proto.StackifyProto.LogGroup) obj;
if (!getEnvironment()
.equals(other.getEnvironment())) return false;
if (!getServerName()
.equals(other.getServerName())) return false;
if (!getApplicationName()
.equals(other.getApplicationName())) return false;
if (!getApplicationLocation()
.equals(other.getApplicationLocation())) return false;
if (!getLogger()
.equals(other.getLogger())) return false;
if (!getPlatform()
.equals(other.getPlatform())) return false;
if (!getLogsList()
.equals(other.getLogsList())) return false;
if (hasContainer() != other.hasContainer()) return false;
if (hasContainer()) {
if (!getContainer()
.equals(other.getContainer())) return false;
}
if (hasKubernetes() != other.hasKubernetes()) return false;
if (hasKubernetes()) {
if (!getKubernetes()
.equals(other.getKubernetes())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ENVIRONMENT_FIELD_NUMBER;
hash = (53 * hash) + getEnvironment().hashCode();
hash = (37 * hash) + SERVER_NAME_FIELD_NUMBER;
hash = (53 * hash) + getServerName().hashCode();
hash = (37 * hash) + APPLICATION_NAME_FIELD_NUMBER;
hash = (53 * hash) + getApplicationName().hashCode();
hash = (37 * hash) + APPLICATION_LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getApplicationLocation().hashCode();
hash = (37 * hash) + LOGGER_FIELD_NUMBER;
hash = (53 * hash) + getLogger().hashCode();
hash = (37 * hash) + PLATFORM_FIELD_NUMBER;
hash = (53 * hash) + getPlatform().hashCode();
if (getLogsCount() > 0) {
hash = (37 * hash) + LOGS_FIELD_NUMBER;
hash = (53 * hash) + getLogsList().hashCode();
}
if (hasContainer()) {
hash = (37 * hash) + CONTAINER_FIELD_NUMBER;
hash = (53 * hash) + getContainer().hashCode();
}
if (hasKubernetes()) {
hash = (37 * hash) + KUBERNETES_FIELD_NUMBER;
hash = (53 * hash) + getKubernetes().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.stackify.api.common.proto.StackifyProto.LogGroup prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code stackify.LogGroup}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:stackify.LogGroup)
com.stackify.api.common.proto.StackifyProto.LogGroupOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.stackify.api.common.proto.StackifyProto.LogGroup.class, com.stackify.api.common.proto.StackifyProto.LogGroup.Builder.class);
}
// Construct using com.stackify.api.common.proto.StackifyProto.LogGroup.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLogsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
environment_ = "";
serverName_ = "";
applicationName_ = "";
applicationLocation_ = "";
logger_ = "";
platform_ = "";
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
logsBuilder_.clear();
}
if (containerBuilder_ == null) {
container_ = null;
} else {
container_ = null;
containerBuilder_ = null;
}
if (kubernetesBuilder_ == null) {
kubernetes_ = null;
} else {
kubernetes_ = null;
kubernetesBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.stackify.api.common.proto.StackifyProto.internal_static_stackify_LogGroup_descriptor;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup getDefaultInstanceForType() {
return com.stackify.api.common.proto.StackifyProto.LogGroup.getDefaultInstance();
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup build() {
com.stackify.api.common.proto.StackifyProto.LogGroup result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup buildPartial() {
com.stackify.api.common.proto.StackifyProto.LogGroup result = new com.stackify.api.common.proto.StackifyProto.LogGroup(this);
int from_bitField0_ = bitField0_;
result.environment_ = environment_;
result.serverName_ = serverName_;
result.applicationName_ = applicationName_;
result.applicationLocation_ = applicationLocation_;
result.logger_ = logger_;
result.platform_ = platform_;
if (logsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.logs_ = logs_;
} else {
result.logs_ = logsBuilder_.build();
}
if (containerBuilder_ == null) {
result.container_ = container_;
} else {
result.container_ = containerBuilder_.build();
}
if (kubernetesBuilder_ == null) {
result.kubernetes_ = kubernetes_;
} else {
result.kubernetes_ = kubernetesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.stackify.api.common.proto.StackifyProto.LogGroup) {
return mergeFrom((com.stackify.api.common.proto.StackifyProto.LogGroup)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.stackify.api.common.proto.StackifyProto.LogGroup other) {
if (other == com.stackify.api.common.proto.StackifyProto.LogGroup.getDefaultInstance()) return this;
if (!other.getEnvironment().isEmpty()) {
environment_ = other.environment_;
onChanged();
}
if (!other.getServerName().isEmpty()) {
serverName_ = other.serverName_;
onChanged();
}
if (!other.getApplicationName().isEmpty()) {
applicationName_ = other.applicationName_;
onChanged();
}
if (!other.getApplicationLocation().isEmpty()) {
applicationLocation_ = other.applicationLocation_;
onChanged();
}
if (!other.getLogger().isEmpty()) {
logger_ = other.logger_;
onChanged();
}
if (!other.getPlatform().isEmpty()) {
platform_ = other.platform_;
onChanged();
}
if (logsBuilder_ == null) {
if (!other.logs_.isEmpty()) {
if (logs_.isEmpty()) {
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLogsIsMutable();
logs_.addAll(other.logs_);
}
onChanged();
}
} else {
if (!other.logs_.isEmpty()) {
if (logsBuilder_.isEmpty()) {
logsBuilder_.dispose();
logsBuilder_ = null;
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00000001);
logsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLogsFieldBuilder() : null;
} else {
logsBuilder_.addAllMessages(other.logs_);
}
}
}
if (other.hasContainer()) {
mergeContainer(other.getContainer());
}
if (other.hasKubernetes()) {
mergeKubernetes(other.getKubernetes());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.stackify.api.common.proto.StackifyProto.LogGroup parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.stackify.api.common.proto.StackifyProto.LogGroup) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object environment_ = "";
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
public java.lang.String getEnvironment() {
java.lang.Object ref = environment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
environment_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
public com.google.protobuf.ByteString
getEnvironmentBytes() {
java.lang.Object ref = environment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
environment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
public Builder setEnvironment(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
environment_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
public Builder clearEnvironment() {
environment_ = getDefaultInstance().getEnvironment();
onChanged();
return this;
}
/**
*
* REQUIRED The environment name. Example: "Prod"
*
*
* string environment = 1;
*/
public Builder setEnvironmentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
environment_ = value;
onChanged();
return this;
}
private java.lang.Object serverName_ = "";
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
public java.lang.String getServerName() {
java.lang.Object ref = serverName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
serverName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
public com.google.protobuf.ByteString
getServerNameBytes() {
java.lang.Object ref = serverName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
serverName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
public Builder setServerName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
serverName_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
public Builder clearServerName() {
serverName_ = getDefaultInstance().getServerName();
onChanged();
return this;
}
/**
*
* REQUIRED The name of the device. Example: "PROD-RS-Debian-7"
*
*
* string server_name = 2;
*/
public Builder setServerNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
serverName_ = value;
onChanged();
return this;
}
private java.lang.Object applicationName_ = "";
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
public java.lang.String getApplicationName() {
java.lang.Object ref = applicationName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
public com.google.protobuf.ByteString
getApplicationNameBytes() {
java.lang.Object ref = applicationName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
public Builder setApplicationName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
applicationName_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
public Builder clearApplicationName() {
applicationName_ = getDefaultInstance().getApplicationName();
onChanged();
return this;
}
/**
*
* REQUIRED The name of the application. Example: "stackify-agent"
*
*
* string application_name = 3;
*/
public Builder setApplicationNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
applicationName_ = value;
onChanged();
return this;
}
private java.lang.Object applicationLocation_ = "";
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
public java.lang.String getApplicationLocation() {
java.lang.Object ref = applicationLocation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
applicationLocation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
public com.google.protobuf.ByteString
getApplicationLocationBytes() {
java.lang.Object ref = applicationLocation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
applicationLocation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
public Builder setApplicationLocation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
applicationLocation_ = value;
onChanged();
return this;
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
public Builder clearApplicationLocation() {
applicationLocation_ = getDefaultInstance().getApplicationLocation();
onChanged();
return this;
}
/**
*
* Optional. The full directory path for the application. Example: "/usr/local/stackify/stackify-agent"
*
*
* string application_location = 4;
*/
public Builder setApplicationLocationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
applicationLocation_ = value;
onChanged();
return this;
}
private java.lang.Object logger_ = "";
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
public java.lang.String getLogger() {
java.lang.Object ref = logger_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
logger_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
public com.google.protobuf.ByteString
getLoggerBytes() {
java.lang.Object ref = logger_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
logger_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
public Builder setLogger(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
logger_ = value;
onChanged();
return this;
}
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
public Builder clearLogger() {
logger_ = getDefaultInstance().getLogger();
onChanged();
return this;
}
/**
*
* REQUIRED The name and version of the logging project generating this request. Example: "stackify-log-log4j12-1.0.12"
*
*
* string logger = 5;
*/
public Builder setLoggerBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
logger_ = value;
onChanged();
return this;
}
private java.lang.Object platform_ = "";
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
public java.lang.String getPlatform() {
java.lang.Object ref = platform_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
platform_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
public com.google.protobuf.ByteString
getPlatformBytes() {
java.lang.Object ref = platform_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
platform_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
public Builder setPlatform(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
platform_ = value;
onChanged();
return this;
}
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
public Builder clearPlatform() {
platform_ = getDefaultInstance().getPlatform();
onChanged();
return this;
}
/**
*
* The logging language. Example: "java"
*
*
* string platform = 6;
*/
public Builder setPlatformBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
platform_ = value;
onChanged();
return this;
}
private java.util.List logs_ =
java.util.Collections.emptyList();
private void ensureLogsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
logs_ = new java.util.ArrayList(logs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder> logsBuilder_;
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public java.util.List getLogsList() {
if (logsBuilder_ == null) {
return java.util.Collections.unmodifiableList(logs_);
} else {
return logsBuilder_.getMessageList();
}
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public int getLogsCount() {
if (logsBuilder_ == null) {
return logs_.size();
} else {
return logsBuilder_.getCount();
}
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log getLogs(int index) {
if (logsBuilder_ == null) {
return logs_.get(index);
} else {
return logsBuilder_.getMessage(index);
}
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder setLogs(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.set(index, value);
onChanged();
} else {
logsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder setLogs(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.set(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder addLogs(com.stackify.api.common.proto.StackifyProto.LogGroup.Log value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(value);
onChanged();
} else {
logsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder addLogs(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(index, value);
onChanged();
} else {
logsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder addLogs(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder addLogs(
int index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder addAllLogs(
java.lang.Iterable extends com.stackify.api.common.proto.StackifyProto.LogGroup.Log> values) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, logs_);
onChanged();
} else {
logsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder clearLogs() {
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
logsBuilder_.clear();
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public Builder removeLogs(int index) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.remove(index);
onChanged();
} else {
logsBuilder_.remove(index);
}
return this;
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder getLogsBuilder(
int index) {
return getLogsFieldBuilder().getBuilder(index);
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder getLogsOrBuilder(
int index) {
if (logsBuilder_ == null) {
return logs_.get(index); } else {
return logsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public java.util.List extends com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder>
getLogsOrBuilderList() {
if (logsBuilder_ != null) {
return logsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logs_);
}
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder addLogsBuilder() {
return getLogsFieldBuilder().addBuilder(
com.stackify.api.common.proto.StackifyProto.LogGroup.Log.getDefaultInstance());
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder addLogsBuilder(
int index) {
return getLogsFieldBuilder().addBuilder(
index, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.getDefaultInstance());
}
/**
* repeated .stackify.LogGroup.Log logs = 7;
*/
public java.util.List
getLogsBuilderList() {
return getLogsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder>
getLogsFieldBuilder() {
if (logsBuilder_ == null) {
logsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Log, com.stackify.api.common.proto.StackifyProto.LogGroup.Log.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.LogOrBuilder>(
logs_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
logs_ = null;
}
return logsBuilder_;
}
private com.stackify.api.common.proto.StackifyProto.LogGroup.Container container_;
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Container, com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.ContainerOrBuilder> containerBuilder_;
/**
* .stackify.LogGroup.Container container = 8;
*/
public boolean hasContainer() {
return containerBuilder_ != null || container_ != null;
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Container getContainer() {
if (containerBuilder_ == null) {
return container_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Container.getDefaultInstance() : container_;
} else {
return containerBuilder_.getMessage();
}
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public Builder setContainer(com.stackify.api.common.proto.StackifyProto.LogGroup.Container value) {
if (containerBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
container_ = value;
onChanged();
} else {
containerBuilder_.setMessage(value);
}
return this;
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public Builder setContainer(
com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder builderForValue) {
if (containerBuilder_ == null) {
container_ = builderForValue.build();
onChanged();
} else {
containerBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public Builder mergeContainer(com.stackify.api.common.proto.StackifyProto.LogGroup.Container value) {
if (containerBuilder_ == null) {
if (container_ != null) {
container_ =
com.stackify.api.common.proto.StackifyProto.LogGroup.Container.newBuilder(container_).mergeFrom(value).buildPartial();
} else {
container_ = value;
}
onChanged();
} else {
containerBuilder_.mergeFrom(value);
}
return this;
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public Builder clearContainer() {
if (containerBuilder_ == null) {
container_ = null;
onChanged();
} else {
container_ = null;
containerBuilder_ = null;
}
return this;
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder getContainerBuilder() {
onChanged();
return getContainerFieldBuilder().getBuilder();
}
/**
* .stackify.LogGroup.Container container = 8;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.ContainerOrBuilder getContainerOrBuilder() {
if (containerBuilder_ != null) {
return containerBuilder_.getMessageOrBuilder();
} else {
return container_ == null ?
com.stackify.api.common.proto.StackifyProto.LogGroup.Container.getDefaultInstance() : container_;
}
}
/**
* .stackify.LogGroup.Container container = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Container, com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.ContainerOrBuilder>
getContainerFieldBuilder() {
if (containerBuilder_ == null) {
containerBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Container, com.stackify.api.common.proto.StackifyProto.LogGroup.Container.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.ContainerOrBuilder>(
getContainer(),
getParentForChildren(),
isClean());
container_ = null;
}
return containerBuilder_;
}
private com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes kubernetes_;
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes, com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.KubernetesOrBuilder> kubernetesBuilder_;
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public boolean hasKubernetes() {
return kubernetesBuilder_ != null || kubernetes_ != null;
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes getKubernetes() {
if (kubernetesBuilder_ == null) {
return kubernetes_ == null ? com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.getDefaultInstance() : kubernetes_;
} else {
return kubernetesBuilder_.getMessage();
}
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public Builder setKubernetes(com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes value) {
if (kubernetesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kubernetes_ = value;
onChanged();
} else {
kubernetesBuilder_.setMessage(value);
}
return this;
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public Builder setKubernetes(
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder builderForValue) {
if (kubernetesBuilder_ == null) {
kubernetes_ = builderForValue.build();
onChanged();
} else {
kubernetesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public Builder mergeKubernetes(com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes value) {
if (kubernetesBuilder_ == null) {
if (kubernetes_ != null) {
kubernetes_ =
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.newBuilder(kubernetes_).mergeFrom(value).buildPartial();
} else {
kubernetes_ = value;
}
onChanged();
} else {
kubernetesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public Builder clearKubernetes() {
if (kubernetesBuilder_ == null) {
kubernetes_ = null;
onChanged();
} else {
kubernetes_ = null;
kubernetesBuilder_ = null;
}
return this;
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder getKubernetesBuilder() {
onChanged();
return getKubernetesFieldBuilder().getBuilder();
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
public com.stackify.api.common.proto.StackifyProto.LogGroup.KubernetesOrBuilder getKubernetesOrBuilder() {
if (kubernetesBuilder_ != null) {
return kubernetesBuilder_.getMessageOrBuilder();
} else {
return kubernetes_ == null ?
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.getDefaultInstance() : kubernetes_;
}
}
/**
* .stackify.LogGroup.Kubernetes kubernetes = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes, com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.KubernetesOrBuilder>
getKubernetesFieldBuilder() {
if (kubernetesBuilder_ == null) {
kubernetesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes, com.stackify.api.common.proto.StackifyProto.LogGroup.Kubernetes.Builder, com.stackify.api.common.proto.StackifyProto.LogGroup.KubernetesOrBuilder>(
getKubernetes(),
getParentForChildren(),
isClean());
kubernetes_ = null;
}
return kubernetesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:stackify.LogGroup)
}
// @@protoc_insertion_point(class_scope:stackify.LogGroup)
private static final com.stackify.api.common.proto.StackifyProto.LogGroup DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.stackify.api.common.proto.StackifyProto.LogGroup();
}
public static com.stackify.api.common.proto.StackifyProto.LogGroup getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LogGroup parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LogGroup(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.stackify.api.common.proto.StackifyProto.LogGroup getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Container_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Container_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Kubernetes_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Kubernetes_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_ServerVariablesEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_ServerVariablesEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_ErrorItem_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_ErrorItem_DataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_ErrorItem_DataEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_HeadersEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_HeadersEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_CookiesEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_CookiesEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_QuerystringEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_QuerystringEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_PostDataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_PostDataEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_SessionDataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_SessionDataEntry_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\024stackify-agent.proto\022\010stackify\"\272\024\n\010Log" +
"Group\022\023\n\013environment\030\001 \001(\t\022\023\n\013server_nam" +
"e\030\002 \001(\t\022\030\n\020application_name\030\003 \001(\t\022\034\n\024app" +
"lication_location\030\004 \001(\t\022\016\n\006logger\030\005 \001(\t\022" +
"\020\n\010platform\030\006 \001(\t\022$\n\004logs\030\007 \003(\0132\026.stacki" +
"fy.LogGroup.Log\022/\n\tcontainer\030\010 \001(\0132\034.sta" +
"ckify.LogGroup.Container\0221\n\nkubernetes\030\t" +
" \001(\0132\035.stackify.LogGroup.Kubernetes\032x\n\tC" +
"ontainer\022\020\n\010image_id\030\001 \001(\t\022\030\n\020image_repo" +
"sitory\030\002 \001(\t\022\021\n\timage_tag\030\003 \001(\t\022\024\n\014conta" +
"iner_id\030\004 \001(\t\022\026\n\016container_name\030\005 \001(\t\032K\n" +
"\nKubernetes\022\020\n\010pod_name\030\001 \001(\t\022\025\n\rpod_nam" +
"espace\030\002 \001(\t\022\024\n\014cluster_name\030\003 \001(\t\032\330\020\n\003L" +
"og\022\017\n\007message\030\001 \001(\t\022\014\n\004data\030\002 \001(\t\022\023\n\013thr" +
"ead_name\030\003 \001(\t\022\023\n\013date_millis\030\004 \001(\003\022\r\n\005l" +
"evel\030\005 \001(\t\022\026\n\016transaction_id\030\006 \001(\t\022\025\n\rso" +
"urce_method\030\007 \001(\t\022\023\n\013source_line\030\010 \001(\005\022\n" +
"\n\002id\030\t \001(\t\022\014\n\004tags\030\n \003(\t\022+\n\005error\030\013 \001(\0132" +
"\034.stackify.LogGroup.Log.Error\032\355\016\n\005Error\022" +
"J\n\022environment_detail\030\001 \001(\0132..stackify.L" +
"ogGroup.Log.Error.EnvironmentDetail\022\023\n\013d" +
"ate_millis\030\002 \001(\003\022:\n\nerror_item\030\003 \001(\0132&.s" +
"tackify.LogGroup.Log.Error.ErrorItem\022I\n\022" +
"web_request_detail\030\004 \001(\0132-.stackify.LogG" +
"roup.Log.Error.WebRequestDetail\022K\n\020serve" +
"r_variables\030\005 \003(\01321.stackify.LogGroup.Lo" +
"g.Error.ServerVariablesEntry\022\025\n\rcustomer" +
"_name\030\006 \001(\t\022\020\n\010username\030\007 \001(\t\0326\n\024ServerV" +
"ariablesEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(" +
"\t:\0028\001\032\252\001\n\021EnvironmentDetail\022\023\n\013device_na" +
"me\030\001 \001(\t\022\030\n\020application_name\030\002 \001(\t\022\034\n\024ap" +
"plication_location\030\003 \001(\t\022#\n\033configured_a" +
"pplication_name\030\004 \001(\t\022#\n\033configured_envi" +
"ronment_name\030\005 \001(\t\032\233\003\n\tErrorItem\022\017\n\007mess" +
"age\030\001 \001(\t\022\022\n\nerror_type\030\002 \001(\t\022\027\n\017error_t" +
"ype_code\030\003 \001(\t\022>\n\004data\030\004 \003(\01320.stackify." +
"LogGroup.Log.Error.ErrorItem.DataEntry\022\025" +
"\n\rsource_method\030\005 \001(\t\022E\n\nstacktrace\030\006 \003(" +
"\01321.stackify.LogGroup.Log.Error.ErrorIte" +
"m.TraceFrame\022;\n\013inner_error\030\007 \001(\0132&.stac" +
"kify.LogGroup.Log.Error.ErrorItem\032+\n\tDat" +
"aEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001\032H" +
"\n\nTraceFrame\022\025\n\rcode_filename\030\001 \001(\t\022\023\n\013l" +
"ine_number\030\002 \001(\005\022\016\n\006method\030\003 \001(\t\032\202\007\n\020Web" +
"RequestDetail\022\027\n\017user_ip_address\030\001 \001(\t\022\023" +
"\n\013http_method\030\002 \001(\t\022\030\n\020request_protocol\030" +
"\003 \001(\t\022\023\n\013request_url\030\004 \001(\t\022\030\n\020request_ur" +
"l_root\030\005 \001(\t\022\024\n\014referral_url\030\006 \001(\t\022K\n\007he" +
"aders\030\007 \003(\0132:.stackify.LogGroup.Log.Erro" +
"r.WebRequestDetail.HeadersEntry\022K\n\007cooki" +
"es\030\010 \003(\0132:.stackify.LogGroup.Log.Error.W" +
"ebRequestDetail.CookiesEntry\022S\n\013querystr" +
"ing\030\t \003(\0132>.stackify.LogGroup.Log.Error." +
"WebRequestDetail.QuerystringEntry\022N\n\tpos" +
"t_data\030\n \003(\0132;.stackify.LogGroup.Log.Err" +
"or.WebRequestDetail.PostDataEntry\022T\n\014ses" +
"sion_data\030\013 \003(\0132>.stackify.LogGroup.Log." +
"Error.WebRequestDetail.SessionDataEntry\022" +
"\025\n\rpost_data_raw\030\014 \001(\t\022\022\n\nmvc_action\030\r \001" +
"(\t\022\026\n\016mvc_controller\030\016 \001(\t\022\020\n\010mvc_area\030\017" +
" \001(\t\032.\n\014HeadersEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005val" +
"ue\030\002 \001(\t:\0028\001\032.\n\014CookiesEntry\022\013\n\003key\030\001 \001(" +
"\t\022\r\n\005value\030\002 \001(\t:\0028\001\0322\n\020QuerystringEntry" +
"\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001\032/\n\rPost" +
"DataEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028" +
"\001\0322\n\020SessionDataEntry\022\013\n\003key\030\001 \001(\t\022\r\n\005va" +
"lue\030\002 \001(\t:\0028\001B.\n\035com.stackify.api.common" +
".protoB\rStackifyProtob\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_stackify_LogGroup_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_stackify_LogGroup_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_descriptor,
new java.lang.String[] { "Environment", "ServerName", "ApplicationName", "ApplicationLocation", "Logger", "Platform", "Logs", "Container", "Kubernetes", });
internal_static_stackify_LogGroup_Container_descriptor =
internal_static_stackify_LogGroup_descriptor.getNestedTypes().get(0);
internal_static_stackify_LogGroup_Container_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Container_descriptor,
new java.lang.String[] { "ImageId", "ImageRepository", "ImageTag", "ContainerId", "ContainerName", });
internal_static_stackify_LogGroup_Kubernetes_descriptor =
internal_static_stackify_LogGroup_descriptor.getNestedTypes().get(1);
internal_static_stackify_LogGroup_Kubernetes_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Kubernetes_descriptor,
new java.lang.String[] { "PodName", "PodNamespace", "ClusterName", });
internal_static_stackify_LogGroup_Log_descriptor =
internal_static_stackify_LogGroup_descriptor.getNestedTypes().get(2);
internal_static_stackify_LogGroup_Log_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_descriptor,
new java.lang.String[] { "Message", "Data", "ThreadName", "DateMillis", "Level", "TransactionId", "SourceMethod", "SourceLine", "Id", "Tags", "Error", });
internal_static_stackify_LogGroup_Log_Error_descriptor =
internal_static_stackify_LogGroup_Log_descriptor.getNestedTypes().get(0);
internal_static_stackify_LogGroup_Log_Error_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_descriptor,
new java.lang.String[] { "EnvironmentDetail", "DateMillis", "ErrorItem", "WebRequestDetail", "ServerVariables", "CustomerName", "Username", });
internal_static_stackify_LogGroup_Log_Error_ServerVariablesEntry_descriptor =
internal_static_stackify_LogGroup_Log_Error_descriptor.getNestedTypes().get(0);
internal_static_stackify_LogGroup_Log_Error_ServerVariablesEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_ServerVariablesEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_descriptor =
internal_static_stackify_LogGroup_Log_Error_descriptor.getNestedTypes().get(1);
internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_EnvironmentDetail_descriptor,
new java.lang.String[] { "DeviceName", "ApplicationName", "ApplicationLocation", "ConfiguredApplicationName", "ConfiguredEnvironmentName", });
internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor =
internal_static_stackify_LogGroup_Log_Error_descriptor.getNestedTypes().get(2);
internal_static_stackify_LogGroup_Log_Error_ErrorItem_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor,
new java.lang.String[] { "Message", "ErrorType", "ErrorTypeCode", "Data", "SourceMethod", "Stacktrace", "InnerError", });
internal_static_stackify_LogGroup_Log_Error_ErrorItem_DataEntry_descriptor =
internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor.getNestedTypes().get(0);
internal_static_stackify_LogGroup_Log_Error_ErrorItem_DataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_ErrorItem_DataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_descriptor =
internal_static_stackify_LogGroup_Log_Error_ErrorItem_descriptor.getNestedTypes().get(1);
internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_ErrorItem_TraceFrame_descriptor,
new java.lang.String[] { "CodeFilename", "LineNumber", "Method", });
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor =
internal_static_stackify_LogGroup_Log_Error_descriptor.getNestedTypes().get(3);
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor,
new java.lang.String[] { "UserIpAddress", "HttpMethod", "RequestProtocol", "RequestUrl", "RequestUrlRoot", "ReferralUrl", "Headers", "Cookies", "Querystring", "PostData", "SessionData", "PostDataRaw", "MvcAction", "MvcController", "MvcArea", });
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_HeadersEntry_descriptor =
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor.getNestedTypes().get(0);
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_HeadersEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_HeadersEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_CookiesEntry_descriptor =
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor.getNestedTypes().get(1);
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_CookiesEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_CookiesEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_QuerystringEntry_descriptor =
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor.getNestedTypes().get(2);
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_QuerystringEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_QuerystringEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_PostDataEntry_descriptor =
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor.getNestedTypes().get(3);
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_PostDataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_PostDataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_SessionDataEntry_descriptor =
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_descriptor.getNestedTypes().get(4);
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_SessionDataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_stackify_LogGroup_Log_Error_WebRequestDetail_SessionDataEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy