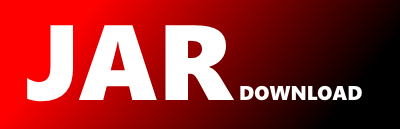
com.starkinfra.IssuingStock Maven / Gradle / Ivy
Show all versions of sdk Show documentation
package com.starkinfra;
import com.starkinfra.utils.Rest;
import com.starkinfra.utils.Resource;
import com.starkinfra.utils.Generator;
import com.starkinfra.utils.SubResource;
import java.util.Map;
import java.util.List;
import java.util.HashMap;
import java.util.ArrayList;
public final class IssuingStock extends Resource {
/**
* IssuingStock object
*
* The IssuingStock object represents the current stock of a certain IssuingDesign linked to an Embosser available to your workspace.
*
* Parameters:
* id [string]: unique id returned when IssuingStock is created. ex: "5656565656565656"
* balance [integer]: [EXPANDABLE] current stock balance. ex: 1000
* designId [string]: IssuingDesign unique id. ex: "5656565656565656"
* embosserId [string]: Embosser unique id. ex: "5656565656565656"
* updated [string]: latest update datetime for the IssuingStock. ex: "2020-03-10 10:30:00.000000+00:00"
* created [string]: creation datetime for the IssuingStock. ex: "2020-03-10 10:30:00.000000+00:00"
*
*/
static ClassData data = new ClassData(IssuingStock.class, "IssuingStock");
public Integer balance;
public String designId;
public String embosserId;
public String updated;
public String created;
/**
* IssuingStock object
*
* The IssuingStock object represents the current stock of a certain IssuingDesign linked to an Embosser available to your workspace.
*
* Parameters:
* @param balance [integer]: [EXPANDABLE] current stock balance. ex: 1000
* @param designId [string]: IssuingDesign unique id. ex: "5656565656565656"
* @param embosserId [string]: Embosser unique id. ex: "5656565656565656"
* @param updated [string]: latest update datetime for the IssuingStock. ex: "2020-03-10 10:30:00.000000+00:00"
* @param created [string]: creation datetime for the IssuingStock. ex: "2020-03-10 10:30:00.000000+00:00"
*/
public IssuingStock(
String id, Integer balance, String designId, String embosserId, String updated, String created
) {
super(id);
this.balance = balance;
this.designId = designId;
this.embosserId = embosserId;
this.updated = updated;
this.created = created;
}
/**
* IssuingStock object
*
* The IssuingStock object represents the current stock of a certain IssuingDesign linked to an Embosser available to your workspace.
*
* Attributes (return-only):
* id [string]: unique id returned when IssuingStock is created. ex: "5656565656565656"
* balance [integer]: [EXPANDABLE] current stock balance. ex: 1000
* designId [string]: IssuingDesign unique id. ex: "5656565656565656"
* embosserId [string]: Embosser unique id. ex: "5656565656565656"
* updated [string]: latest update datetime for the IssuingStock. ex: "2020-03-10 10:30:00.000000+00:00"
* created [string]: creation datetime for the IssuingStock. ex: "2020-03-10 10:30:00.000000+00:00"
* @throws Exception error in the request
*/
@SuppressWarnings("unchecked")
public IssuingStock(Map data) throws Exception {
super(null);
HashMap dataCopy = new HashMap<>(data);
this.balance = null;
this.designId = null;
this.embosserId = null;
this.updated = null;
this.created = null;
if (!dataCopy.isEmpty()) {
throw new Exception("Unknown parameters used in constructor: [" + String.join(", ", dataCopy.keySet()) + "]");
}
}
/**
* Retrieve IssuingStocks
*
* Receive a generator of IssuingStock objects previously created in the Stark Infra API
*
* Parameters:
* @param params map of parameters for the query
* limit [integer, default null]: maximum number of objects to be retrieved. Unlimited if null. ex: 35
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* designIds [list of strings, default null]: IssuingDesign unique ids. ex: ["5656565656565656", "4545454545454545"]
* embosserIds [list of strings, default null]: Embosser unique ids. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* expand [list of strings, default null]: fields to expand information. ex: ["balance"]
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return generator of IssuingStock objects with updated attributes
* @throws Exception error in the request
*/
public static Generator query(Map params, User user) throws Exception{
return Rest.getStream(data, params, user);
}
/**
* Retrieve IssuingStocks
*
* Receive a generator of IssuingStock objects previously created in the Stark Infra API
*
* Parameters:
* @param params map of parameters for the query
* limit [integer, default null]: maximum number of objects to be retrieved. Unlimited if null. ex: 35
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* designIds [list of strings, default null]: IssuingDesign unique ids. ex: ["5656565656565656", "4545454545454545"]
* embosserIds [list of strings, default null]: Embosser unique ids. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* expand [list of strings, default null]: fields to expand information. ex: ["balance"]
*
* Return:
* @return generator of IssuingStock objects with updated attributes
* @throws Exception error in the request
*/
public static Generator query(Map params) throws Exception{
return Rest.getStream(data, params, null);
}
/**
* Retrieve IssuingStocks
*
* Receive a generator of IssuingStock objects previously created in the Stark Infra API
*
* Parameters:
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return generator of IssuingStock objects with updated attributes
* @throws Exception error in the request
*/
public static Generator query(User user) throws Exception{
return Rest.getStream(data, new HashMap<>(), user);
}
/**
* Retrieve IssuingStocks
*
* Receive a generator of IssuingStock objects previously created in the Stark Infra API
*
* Return:
* @return generator of IssuingStock objects with updated attributes
* @throws Exception error in the request
*/
public static Generator query() throws Exception{
return Rest.getStream(data, new HashMap<>(), null);
}
public final static class Page {
public List stocks;
public String cursor;
public Page(List stocks, String cursor) {
this.stocks = stocks;
this.cursor = cursor;
}
}
/**
* Retrieve paged IssuingStocks
*
* Receive a list of up to 100 IssuingStock objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your IssuingStocks.
*
* Return:
* IssuingStock.Page.Stocks: list of IssuingStock objects with updated attributes
* IssuingStock.Page.cursor: cursor to retrieve the next page of IssuingStock objects
* @throws Exception error in the request
*/
public static Page page() throws Exception {
return page(new HashMap<>(), null);
}
/**
* Retrieve paged IssuingStocks
*
* Receive a list of up to 100 IssuingStock objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your IssuingStocks.
*
* Parameters:
* @param params map of parameters
* cursor [string, default null]: cursor returned on the previous page function call
* limit [integer, default 100]: maximum number of objects to be retrieved. Max = 100. ex: 35
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* designIds [list of strings, default null]: IssuingDesign unique ids. ex: ["5656565656565656", "4545454545454545"]
* embosserIds [list of strings, default null]: Embosser unique ids. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* expand [list of strings, default null]: fields to expand information. ex: ["balance"]
*
* Return:
* IssuingStock.Page.stocks: list of IssuingStock objects with updated attributes
* IssuingStock.Page.cursor: cursor to retrieve the next page of IssuingStock objects
* @throws Exception error in the request
*/
public static Page page(Map params) throws Exception {
return page(params, null);
}
/**
* Retrieve paged IssuingStocks
*
* Receive a list of up to 100 IssuingStock objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your IssuingStocks.
*
* Parameters:
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* IssuingStock.Page.stocks: list of IssuingStock objects with updated attributes
* IssuingStock.Page.cursor: cursor to retrieve the next page of IssuingStock objects
* @throws Exception error in the request
*/
public static Page page(User user) throws Exception {
return page(new HashMap<>(), user);
}
/**
* Retrieve paged IssuingStocks
*
* Receive a list of up to 100 IssuingStock objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your IssuingStocks.
*
* Parameters:
* @param params map of parameters
* cursor [string, default null]: cursor returned on the previous page function call
* limit [integer, default 100]: maximum number of objects to be retrieved. Max = 100. ex: 35
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* designIds [list of strings, default null]: IssuingDesign unique ids. ex: ["5656565656565656", "4545454545454545"]
* embosserIds [list of strings, default null]: Embosser unique ids. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* expand [list of strings, default null]: fields to expand information. ex: ["balance"]
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* IssuingStock.Page.stocks: list of IssuingStock objects with updated attributes
* IssuingStock.Page.cursor: cursor to retrieve the next page of IssuingStock objects
* @throws Exception error in the request
*/
public static Page page(Map params, User user) throws Exception {
com.starkinfra.utils.Page page = Rest.getPage(data, params, user);
List stocks = new ArrayList<>();
for (SubResource issuingStock: page.entities) {
stocks.add((IssuingStock) issuingStock);
}
return new Page(stocks, page.cursor);
}
/**
* Retrieve a specific IssuingStock
*
* Receive a single IssuingStock object previously created in the Stark Infra API by its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
* @param params map of parameters
* expand [list of strings, default null]: fields to expand information. ex: ["balance"]
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return IssuingStock object with updated attributes
* @throws Exception error in the request
*/
public static IssuingStock get(String id, Map params, User user) throws Exception {
return Rest.getId(data, id, params, user);
}
/**
* Retrieve a specific IssuingStock
*
* Receive a single IssuingStock object previously created in the Stark Infra API by its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
* @param params map of parameters
* expand [list of strings, default null]: fields to expand information. ex: ["balance"]
*
* Return:
* @return IssuingStock object with updated attributes
* @throws Exception error in the request
*/
public static IssuingStock get(String id, Map params) throws Exception{
return Rest.getId(data, id, params, null);
}
/**
* Retrieve a specific IssuingStock
*
* Receive a single IssuingStock object previously created in the Stark Infra API by its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return IssuingStock object with updated attributes
* @throws Exception error in the request
*/
public static IssuingStock get(String id, User user) throws Exception {
return Rest.getId(data, id, user);
}
/**
* Retrieve a specific IssuingStock
*
* Receive a single IssuingStock object previously created in the Stark Infra API by its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
*
* Return:
* @return IssuingStock object with updated attributes
* @throws Exception error in the request
*/
public static IssuingStock get(String id) throws Exception{
return Rest.getId(data, id, null);
}
public final static class Log extends Resource {
static ClassData data = new ClassData(IssuingStock.Log.class, "IssuingStockLog");
public IssuingStock stock;
public String type;
public Integer count;
public String created;
/**
* IssuingStock Log object
*
* Every time an IssuingStock entity is modified, a corresponding IssuingStock Log
* is generated for the entity. This log is never generated by the user,
* but it can be retrieved to check additional information on the IssuingStock.
*
* Attributes:
* @param id [string]: unique id returned when the log is created. ex: "5656565656565656"
* @param stock [IssuingStock object]: IssuingStock entity to which the log refers to.
* @param type [string]: type of the IssuingStock event which triggered the log creation. Options: "created", "spent", "restocked", "lost"
* @param count [integer]: shift in stock balance. ex: 10
* @param created [string]: creation datetime for the log. ex: "2020-03-10 10:30:00.000000+00:00"
*/
public Log(String id, IssuingStock stock, String type, Integer count, String created ) {
super(id);
this.stock = stock;
this.type = type;
this.count = count;
this.created = created;
}
/**
* Retrieve a specific IssuingStock Log
*
* Receive a single IssuingStock Log object previously created by the Stark Infra API by passing its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
*
* Return:
* @return IssuingStock Log object with updated attributes
* @throws Exception error in the stock
*/
public static IssuingStock.Log get(String id) throws Exception {
return IssuingStock.Log.get(id, null);
}
/**
* Retrieve a specific IssuingStock Log
*
* Receive a single IssuingStock Log object previously created by the Stark Infra API by passing its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return IssuingStock Log object with updated attributes
* @throws Exception error in the stock
*/
public static IssuingStock.Log get(String id, User user) throws Exception {
return Rest.getId(data, id, user);
}
/**
* Retrieve IssuingStock Logs
*
* Receive a generator of IssuingStock.Log objects previously created in the Stark Infra API.
* Use this function instead of page if you want to stream the objects without worrying about cursors and pagination.
*
* Parameters:
* @param params map of parameters for the query
* limit [integer, default null]: maximum number of objects to be retrieved. Unlimited if null. ex: 35
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* types [list of strings, default null]: filter retrieved objects by types. ex: ["created", "spent", "restocked", "lost"]
* stockIds [list of strings, default null]: list of IssuingStock ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
*
* Return:
* @return generator of IssuingStock Log objects with updated attributes
* @throws Exception error in the stock
*/
public static Generator query(Map params) throws Exception {
return IssuingStock.Log.query(params, null);
}
/**
* Retrieve IssuingStock Logs
*
* Receive a generator of IssuingStock.Log objects previously created in the Stark Infra API.
* Use this function instead of page if you want to stream the objects without worrying about cursors and pagination.
*
* Parameters:
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return generator of IssuingStock Log objects with updated attributes
* @throws Exception error in the stock
*/
public static Generator query(User user) throws Exception {
return IssuingStock.Log.query(new HashMap<>(), user);
}
/**
* Retrieve IssuingStock Logs
*
* Receive a generator of IssuingStock.Log objects previously created in the Stark Infra API.
* Use this function instead of page if you want to stream the objects without worrying about cursors and pagination.
*
* Return:
* @return generator of IssuingStock Log objects with updated attributes
* @throws Exception error in the stock
*/
public static Generator query() throws Exception {
return IssuingStock.Log.query(new HashMap<>(), null);
}
/**
* Retrieve IssuingStock Logs
*
* Receive a generator of IssuingStock.Log objects previously created in the Stark Infra API.
* Use this function instead of page if you want to stream the objects without worrying about cursors and pagination.
*
* Parameters:
* @param params map of parameters for the query
* limit [integer, default null]: maximum number of objects to be retrieved. Unlimited if null. ex: 35
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* types [list of strings, default null]: filter retrieved objects by types. ex: ["created", "spent", "restocked", "lost"]
* stockIds [list of strings, default null]: list of IssuingStock ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return generator of IssuingStock Log objects with updated attributes
* @throws Exception error in the stock
*/
public static Generator query(Map params, User user) throws Exception {
return Rest.getStream(data, params, user);
}
public final static class Page {
public List logs;
public String cursor;
public Page(List logs, String cursor) {
this.logs = logs;
this.cursor = cursor;
}
}
/**
* Retrieve paged IssuingStock.Logs
*
* Receive a list of up to 100 IssuingStock.Log objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your stocks.
*
* Parameters:
* @param params map of parameters for the query
* cursor [string, default null]: cursor returned on the previous page function call
* limit [integer, default 100]: maximum number of objects to be retrieved. It must be an integer between 1 and 100. ex: 50
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* types [list of strings, default null]: filter retrieved objects by types. ex: ["created", "spent", "restocked", "lost"]
* stockIds [list of strings, default null]: list of IssuingStock ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
*
* Return:
* @return IssuingStock.Log.Page object:
* IssuingStock.Log.Page.logs: list of IssuingStock.Log objects with updated attributes
* IssuingStock.Log.Page.cursor: cursor to retrieve the next page of IssuingStock.Log objects
* @throws Exception error in the stock
*/
public static IssuingStock.Log.Page page(Map params) throws Exception {
return IssuingStock.Log.page(params, null);
}
/**
* Retrieve paged IssuingStock.Logs
*
* Receive a list of up to 100 IssuingStock.Log objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your stocks.
*
* Parameters:
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return IssuingStock.Log.Page object:
* IssuingStock.Log.Page.logs: list of IssuingStock.Log objects with updated attributes
* IssuingStock.Log.Page.cursor: cursor to retrieve the next page of IssuingStock.Log objects
* @throws Exception error in the stock
*/
public static IssuingStock.Log.Page page(User user) throws Exception {
return IssuingStock.Log.page(new HashMap<>(), user);
}
/**
* Retrieve paged IssuingStock.Logs
*
* Receive a list of up to 100 IssuingStock.Log objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your stocks.
*
* Return:
* @return IssuingStock.Log.Page object:
* IssuingStock.Log.Page.logs: list of IssuingStock.Log objects with updated attributes
* IssuingStock.Log.Page.cursor: cursor to retrieve the next page of IssuingStock.Log objects
* @throws Exception error in the stock
*/
public static IssuingStock.Log.Page page() throws Exception {
return IssuingStock.Log.page(new HashMap<>(), null);
}
/**
* Retrieve paged IssuingStock.Logs
*
* Receive a list of up to 100 IssuingStock.Log objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page your stocks.
*
* Parameters:
* @param params map of parameters for the query
* cursor [string, default null]: cursor returned on the previous page function call
* limit [integer, default 100]: maximum number of objects to be retrieved. It must be an integer between 1 and 100. ex: 50
* after [string, default null]: date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* types [list of strings, default null]: filter retrieved objects by types. ex: ["created", "spent", "restocked", "lost"]
* stockIds [list of strings, default null]: list of IssuingStock ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* ids [list of strings, default null]: list of ids to filter retrieved objects. ex: ["5656565656565656", "4545454545454545"]
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return IssuingStock.Log.Page object:
* IssuingStock.Log.Page.logs: list of IssuingStock.Log objects with updated attributes
* IssuingStock.Log.Page.cursor: cursor to retrieve the next page of IssuingStock.Log objects
* @throws Exception error in the stock
*/
public static IssuingStock.Log.Page page(Map params, User user) throws Exception {
com.starkinfra.utils.Page page = Rest.getPage(data, params, user);
List logs = new ArrayList<>();
for (SubResource log: page.entities) {
logs.add((IssuingStock.Log) log);
}
return new Page(logs, page.cursor);
}
}
}