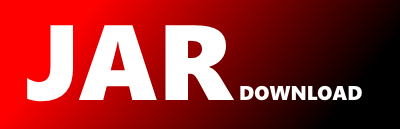
com.starkinfra.IssuingTransaction Maven / Gradle / Ivy
Show all versions of sdk Show documentation
package com.starkinfra;
import com.starkinfra.utils.Rest;
import com.starkinfra.utils.Resource;
import com.starkinfra.utils.Generator;
import com.starkinfra.utils.SubResource;
import java.util.Map;
import java.util.List;
import java.util.HashMap;
import java.util.ArrayList;
public final class IssuingTransaction extends Resource {
/**
* IssuingTransaction object
*
* The IssuingTransaction objects created in your Workspace to represent each balance shift.
*
* Parameters:
* id [string]: unique id returned when IssuingTransaction is created. ex: "5656565656565656"
* amount [Long]: IssuingTransaction value in cents. ex: 1234 (= R$ 12.34)
* balance [Long]: balance amount of the workspace at the instant of the Transaction in cents. ex: 200 (= R$ 2.00)
* description [string]: IssuingTransaction description. ex: "Buying food"
* source [string]: source of the transaction. ex: "issuing-purchase/5656565656565656"
* tags [string]: list of strings inherited from the source resource. ex: ["tony", "stark"]
* created [string]: creation datetime for the IssuingTransaction. ex: "2020-03-10 10:30:00.000000+00:00"
*
*/
static ClassData data = new ClassData(IssuingTransaction.class, "IssuingTransaction");
public Long amount;
public Long balance;
public String description;
public String source;
public String[] tags;
public String created;
/**
* IssuingTransaction object
*
* The IssuingTransaction objects created in your Workspace to represent each balance shift.
*
* Parameters:
* @param id [string]: unique id returned when IssuingTransaction is created. ex: "5656565656565656"
* @param amount [Long]: IssuingTransaction value in cents. ex: 1234 (= R$ 12.34)
* @param balance [Long]: balance amount of the workspace at the instant of the Transaction in cents. ex: 200 (= R$ 2.00)
* @param description [string]: IssuingTransaction description. ex: "Buying food"
* @param source [string]: source of the transaction. ex: "issuing-purchase/5656565656565656"
* @param tags [string]: list of strings inherited from the source resource. ex: ["tony", "stark"]
* @param created [string]: creation datetime for the IssuingTransaction. ex: "2020-03-10 10:30:00.000000+00:00"
*/
public IssuingTransaction(String id, Long amount, Long balance, String description,
String source, String[] tags, String created
) {
super(id);
this.amount = amount;
this.balance = balance;
this.description = description;
this.source = source;
this.tags = tags;
this.created = created;
}
/**
* IssuingTransaction object
*
* The IssuingTransaction objects created in your Workspace to represent each balance shift.
*
* Attributes (return-only):
* id [string]: unique id returned when IssuingTransaction is created. ex: "5656565656565656"
* amount [Long]: IssuingTransaction value in cents. ex: 1234 (= R$ 12.34)
* balance [Long]: balance amount of the workspace at the instant of the Transaction in cents. ex: 200 (= R$ 2.00)
* description [string]: IssuingTransaction description. ex: "Buying food"
* source [string]: source of the transaction. ex: "issuing-purchase/5656565656565656"
* tags [string]: list of strings inherited from the source resource. ex: ["tony", "stark"]
* created [string]: creation datetime for the IssuingTransaction. ex: "2020-03-10 10:30:00.000000+00:00"
* @throws Exception error in the request
*/
public IssuingTransaction(Map data) throws Exception {
super(null);
HashMap dataCopy = new HashMap<>(data);
this.amount = null;
this.balance = null;
this.description = null;
this.source = null;
this.tags = null;
this.created = null;
if (!dataCopy.isEmpty()) {
throw new Exception("Unknown parameters used in constructor: [" + String.join(", ", dataCopy.keySet()) + "]");
}
}
/**
* Retrieve a specific IssuingTransaction
*
* Receive a single IssuingTransaction object previously created in the Stark Infra API by its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return IssuingTransaction object with updated attributes
* @throws Exception error in the request
*/
public static IssuingTransaction get(String id, User user) throws Exception{
return Rest.getId(data, id, user);
}
/**
* Retrieve a specific IssuingTransaction
*
* Receive a single IssuingTransaction object previously created in the Stark Infra API by its id
*
* Parameters:
* @param id [string]: object unique id. ex: "5656565656565656"
*
* Return:
* @return IssuingTransaction object with updated attributes
* @throws Exception error in the request
*/
public static IssuingTransaction get(String id) throws Exception{
return Rest.getId(data, id, null);
}
/**
* Retrieve a specific IssuingTransaction
*
* Receive a single IssuingTransaction object previously created in the Stark Infra API by its id
*
* Return:
* @return IssuingTransaction object with updated attributes
* @throws Exception error in the request
*/
public static IssuingTransaction get() throws Exception{
return Rest.getId(data, null, null);
}
/**
* Retrieve IssuingTransaction
*
* Receive a generator of IssuingTransaction objects previously created in the Stark Infra API
*
* Parameters:
* @param params map of parameters for the query
* tags [list of strings, default null]: tags to filter retrieved objects. ex: ["tony", "stark"]
* externalIds [list of strings, default []]: external IDs. ex: ["5656565656565656", "4545454545454545"]
* after [string, default null] date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* status [string, default ""]: filter for status of retrieved objects. ex: "approved", "canceled", "denied", "confirmed" or "voided"
* ids [list of strings, default []]: transaction IDs
* limit [integer, default null]: maximum number of objects to be retrieved. Unlimited if null. ex: 35
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return generator of IssuingTransaction objects with updated attributes
* @throws Exception error in the transaction
*/
public static Generator query(Map params, User user) throws Exception{
return Rest.getStream(data, params, user);
}
/**
* Retrieve IssuingTransaction
*
* Receive a generator of IssuingTransaction objects previously created in the Stark Infra API
*
* Parameters:
* @param params map of parameters for the query
* tags [list of strings, default null]: tags to filter retrieved objects. ex: ["tony", "stark"]
* externalIds [list of strings, default []]: external IDs. ex: ["5656565656565656", "4545454545454545"]
* after [string, default null] date filter for objects created only after specified date. ex: "2020-03-10"
* before [string, default null]: date filter for objects created only before specified date. ex: "2020-03-10"
* status [string, default ""]: filter for status of retrieved objects. ex: "approved", "canceled", "denied", "confirmed" or "voided"
* ids [list of strings, default []]: transaction IDs
* limit [integer, default null]: maximum number of objects to be retrieved. Unlimited if null. ex: 35
*
* Return:
* @return generator of IssuingTransaction objects with updated attributes
* @throws Exception error in the transaction
*/
public static Generator query(Map params) throws Exception{
return Rest.getStream(data, params, null);
}
/**
* Retrieve IssuingTransactions
*
* Receive a generator of IssuingTransaction objects previously created in the Stark Infra API
*
* Parameters:
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return generator of IssuingTransaction objects with updated attributes
* @throws Exception error in the transaction
*/
public static Generator query(User user) throws Exception{
return Rest.getStream(data, new HashMap<>(), user);
}
/**
* Retrieve IssuingTransactions
*
* Receive a generator of IssuingTransaction objects previously created in the Stark Infra API
*
* Return:
* @return generator of IssuingTransaction objects with updated attributes
* @throws Exception error in the transaction
*/
public static Generator query() throws Exception{
return Rest.getStream(data, new HashMap<>(), null);
}
public final static class Page {
public List issuingTransactions;
public String cursor;
public Page(List issuingTransactions, String cursor) {
this.issuingTransactions = issuingTransactions;
this.cursor = cursor;
}
}
/**
* Retrieve paged IssuingTransactions
*
* Receive a list of up to 100 IssuingTransaction objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page transactions.
*
* Return:
* IssuingTransaction.Page.transactions: list of IssuingTransaction objects with updated attributes
* IssuingTransaction.Page.cursor: cursor to retrieve the next page of IssuingTransaction objects
* @throws Exception error in the request
*/
public static IssuingTransaction.Page page() throws Exception {
return page(new HashMap<>(), null);
}
/**
* Retrieve paged IssuingTransactions
*
* Receive a list of up to 100 IssuingTransaction objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page transactions.
*
* Parameters:
* @param params map of parameters
* tags [list of strings, default null]: tags to filter retrieved objects. ex: ["tony", "stark"]
* externalIds [list of strings, default []]: external IDs. ex: ["5656565656565656", "4545454545454545"]
* after [string, default null] date filter for objects created only after specified date. ex: "2022-03-22"
* before [string, default null]: date filter for objects created only before specified date. ex: "2022-03-22"
* status [string, default ""]: filter for status of retrieved objects. ex: "approved", "canceled", "denied", "confirmed" or "voided"
* ids [list of strings, default []]: transaction IDs
* limit [integer, default 100]: maximum number of objects to be retrieved. It must be an integer between 1 and 100. ex: 35
* cursor [string, default null]: cursor returned on the previous page function call
*
* Return:
* IssuingTransaction.Page.transactions: list of IssuingTransaction objects with updated attributes
* IssuingTransaction.Page.cursor: cursor to retrieve the next page of IssuingTransaction objects
* @throws Exception error in the request
*/
public static IssuingTransaction.Page page(Map params) throws Exception {
return page(params, null);
}
/**
* Retrieve paged IssuingTransactions
*
* Receive a list of up to 100 IssuingTransaction objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page transactions.
*
* Parameters:
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* IssuingTransaction.Page.transactions: list of IssuingTransaction objects with updated attributes
* IssuingTransaction.Page.cursor: cursor to retrieve the next page of IssuingTransaction objects
* @throws Exception error in the request
*/
public static IssuingTransaction.Page page(User user) throws Exception {
return page(new HashMap<>(), user);
}
/**
* Retrieve paged IssuingTransactions
*
* Receive a list of up to 100 IssuingTransaction objects previously created in the Stark Infra API and the cursor to the next page.
* Use this function instead of query if you want to manually page transactions.
*
* Parameters:
* @param params map of parameters
* tags [list of strings, default null]: tags to filter retrieved objects. ex: ["tony", "stark"]
* externalIds [list of strings, default []]: external IDs. ex: ["5656565656565656", "4545454545454545"]
* after [string, default null] date filter for objects created only after specified date. ex: "2022-03-22"
* before [string, default null]: date filter for objects created only before specified date. ex: "2022-03-22"
* status [string, default ""]: filter for status of retrieved objects. ex: "approved", "canceled", "denied", "confirmed" or "voided"
* ids [list of strings, default []]: transaction IDs
* limit [integer, default 100]: maximum number of objects to be retrieved. It must be an integer between 1 and 100. ex: 35
* cursor [string, default null]: cursor returned on the previous page function call
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* IssuingTransaction.Page.transactions: list of IssuingTransaction objects with updated attributes
* IssuingTransaction.Page.cursor: cursor to retrieve the next page of IssuingTransaction objects
* @throws Exception error in the request
*/
public static IssuingTransaction.Page page(Map params, User user) throws Exception {
com.starkinfra.utils.Page page = Rest.getPage(data, params, user);
List issuingTransactions = new ArrayList<>();
for (SubResource issuingTransaction: page.entities) {
issuingTransactions.add((IssuingTransaction) issuingTransaction);
}
return new Page(issuingTransactions, page.cursor);
}
}