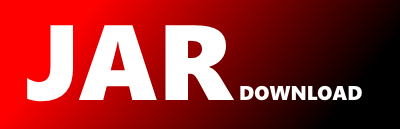
com.starkinfra.Key Maven / Gradle / Ivy
Show all versions of sdk Show documentation
package com.starkinfra;
import com.starkbank.ellipticcurve.PrivateKey;
import java.io.File;
import java.io.PrintWriter;
import java.io.FileNotFoundException;
public final class Key {
public final String privatePem;
public final String publicPem;
public Key(String privatePem) {
PrivateKey privateKey = PrivateKey.fromPem(privatePem);
this.privatePem = privatePem;
this.publicPem = privateKey.publicKey().toPem();
}
/**
* Create a key pair
*
* Generates a secp256k1 ECDSA private/public key pair to be used in the API authentications
*
* Return:
* @return new Key object
*/
public static Key create() {
return new Key((new PrivateKey()).toPem());
}
/**
* Create a key pair
*
* Generates a secp256k1 ECDSA private/public key pair to be used in the API authentications
*
* Parameters:
* @param savePath [string]: path to save the keys .pem files. No files will be saved if this parameter isn't provided
*
* Return:
* @return new Key object
* @throws FileNotFoundException error when the savePath is not found
*/
public static Key create(String savePath) throws FileNotFoundException {
Key key = Key.create();
File directory = new File(savePath);
if (! directory.exists()){
directory.mkdirs();
}
try (PrintWriter out = new PrintWriter(new File(savePath, "privateKey.pem"))) {
out.println(key.privatePem);
}
try (PrintWriter out = new PrintWriter(new File(savePath, "publicKey.pem"))) {
out.println(key.publicPem);
}
return key;
}
}