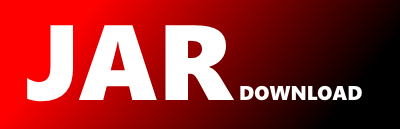
com.starkinfra.PixDomain Maven / Gradle / Ivy
Show all versions of sdk Show documentation
package com.starkinfra;
import com.starkinfra.utils.Rest;
import com.starkinfra.utils.Generator;
import com.starkinfra.utils.SubResource;
import java.util.List;
import java.util.HashMap;
public final class PixDomain extends SubResource {
/**
* PixDomain object
*
* The PixDomain object displays the domain and certificate information of registered
* SPI participants able to issue dynamic QR Codes.
* They are used in the validation of the URLs contained in the dynamic QR Codes.
*
* Parameters:
* certificates [list of Certificate objects]: list of Certificate objects of the SPI participant in PEM format.
* name [string]: current active domain (URL) of the SPI participant.
*
*/
static ClassData data = new ClassData(PixDomain.class, "PixDomain");
public List certificates;
public String name;
/**
* PixDomain object
*
* The PixDomain object displays the certificate information of registered
* SPI participants able to issue dynamic QR Codes.
* They are used in the validation of the URLs contained in the dynamic QR Codes.
*
* Parameters:
* @param certificates [list of Certificate objects]: list of Certificate objects of the SPI participant in PEM format.
* @param name [string]: current active domain (URL) of the SPI participant.
*/
public PixDomain(List certificates, String name) {
this.certificates = certificates;
this.name = name;
}
/**
* Retrieve PixDomains
*
* Receive a generator of PixDomain objects.
*
* Parameters:
* @param user [Organization/Project object, default null]: Organization or Project object. Not necessary if starkinfra.Settings.user was set before function call
*
* Return:
* @return generator of PixDomain objects with updated attributes
* @throws Exception error in the request
*/
public static Generator query(User user) throws Exception {
return Rest.getStream(data, new HashMap(), user);
}
/**
* Retrieve PixDomains
*
* Receive a generator of PixDomain objects.
*
* Return:
* @return generator of PixDomain objects with updated attributes
* @throws Exception error in the request
*/
public static Generator query() throws Exception {
return PixDomain.query(null);
}
/**
* PixDomain Certificate object
*
* The Certificate object displays the certificate's content from a specific domain.
*
* Attributes (return-only):
* content [string]: certificate's content of the Pix participant in PEM format.
*/
public final static class Certificate extends SubResource {
public String content;
static ClassData data = new ClassData(Certificate.class, "Certificate");
/***
* PixDomain Certificate object
*
* The Certificate object displays the certificate's content from a specific domain.
*
* Parameters:
* @param content [string]: certificate of the Pix participant in PEM format.
*/
public Certificate(String content) {
this.content = content;
}
}
}