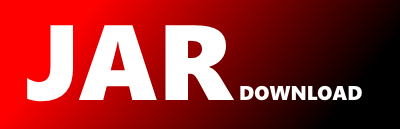
com.starmcc.qmframework.redis.QmRedisClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qm-framework Show documentation
Show all versions of qm-framework Show documentation
springboot based on a series of packaging framework.
QmFramework to implements AOP,
rewrite the requestBody,
global exception catching,version checking,
request body parameter symmetric encryption mechanism,
controller packaging and the toolkit collection.
thank you for using it.
package com.starmcc.qmframework.redis;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.util.CollectionUtils;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
/**
* Redis客户端
*
* @Author qm
* @Date 2018年11月24日 上午2:33:49
*/
public final class QmRedisClient {
private static QmRedisClient qmRedisClient;
private RedisTemplate redisTemplate;
private QmRedisClient() {
}
public QmRedisClient(RedisTemplate redisTemplate) {
qmRedisClient = this;
qmRedisClient.redisTemplate = redisTemplate;
}
/**
* 指定缓存失效时间
*
* @param key 键
* @param time 时间(秒)
* @return
*/
public static boolean expire(String key, long time) {
try {
if (time > 0) {
qmRedisClient.redisTemplate.expire(key, time, TimeUnit.SECONDS);
}
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
/**
* 根据key 获取过期时间
*
* @param key 键 不能为null
* @return 时间(秒) 返回0代表为永久有效
*/
public static long getExpire(String key) {
return qmRedisClient.redisTemplate.getExpire(key, TimeUnit.SECONDS);
}
/**
* 判断key是否存在
*
* @param key 键
* @return true 存在 false不存在
*/
public static boolean hasKey(String key) {
try {
return qmRedisClient.redisTemplate.hasKey(key);
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
// =============================common============================
/**
* 删除缓存
*
* @param key 可以传一个值 或多个
*/
public static boolean del(String... key) {
try {
if (key != null && key.length > 0) {
if (key.length == 1) {
return qmRedisClient.redisTemplate.delete(key[0]);
} else {
Long res = qmRedisClient.redisTemplate.delete(CollectionUtils.arrayToList(key));
if (res < 1) {
return false;
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
/**
* 普通缓存获取
*
* @param key 键
* @return 值
*/
public static Object get(String key) {
return key == null ? null : qmRedisClient.redisTemplate.opsForValue().get(key);
}
/**
* 普通缓存放入
*
* @param key 键
* @param value 值
* @return true成功 false失败
*/
public static boolean set(String key, Object value) {
try {
qmRedisClient.redisTemplate.opsForValue().set(key, value);
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
/**
* 普通缓存放入并设置时间
*
* @param key 键
* @param value 值
* @param time 时间(秒) time要大于0 如果time小于等于0 将设置无限期
* @return true成功 false 失败
*/
public static boolean set(String key, Object value, long time) {
try {
if (time > 0) {
qmRedisClient.redisTemplate.opsForValue().set(key, value, time, TimeUnit.SECONDS);
} else {
set(key, value);
}
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
// ============================String=============================
/**
* 递增
*
* @param key 键
* @param delta 要增加几(大于0)
* @return
*/
public static long incr(String key, long delta) {
if (delta < 0) {
throw new RuntimeException("递增因子必须大于0");
}
return qmRedisClient.redisTemplate.opsForValue().increment(key, delta);
}
/**
* 递减
*
* @param key 键
* @param delta 要减少几(小于0)
* @return
*/
public static long decr(String key, long delta) {
if (delta < 0) {
throw new RuntimeException("递减因子必须大于0");
}
return qmRedisClient.redisTemplate.opsForValue().increment(key, -delta);
}
/**
* HashGet
*
* @param key 键 不能为null
* @param item 项 不能为null
* @return 值
*/
public static Object hget(String key, String item) {
return qmRedisClient.redisTemplate.opsForHash().get(key, item);
}
/**
* 获取hashKey对应的所有键值
*
* @param key 键
* @return 对应的多个键值
*/
public static Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy