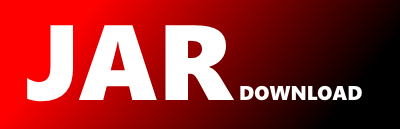
com.staros.filestore.S3FileStore Maven / Gradle / Ivy
// Copyright 2021-present StarRocks, Inc. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.staros.filestore;
import com.staros.proto.FileStoreInfo;
import com.staros.proto.FileStoreType;
import com.staros.proto.S3FileStoreInfo;
public class S3FileStore implements FileStore {
private String bucket;
private String region;
private String endpoint;
private String accessKey;
private String accessKeySecret;
public S3FileStore(String bucket, String region, String endpoint, String accessKey, String accessKeySecret) {
this.bucket = bucket;
this.region = region;
this.endpoint = endpoint;
this.accessKey = accessKey;
this.accessKeySecret = accessKeySecret;
}
// return file store type, 'S3','HDFS' etc
public FileStoreType type() {
return FileStoreType.S3;
}
// return s3 file store key
public String key() {
return bucket;
}
// return file store root path
public String rootPath() {
return String.format("s3://%s/", bucket);
}
public boolean isValid() {
return true;
}
public FileStoreInfo toProtobuf() {
S3FileStoreInfo.Builder s3fsBuilder = S3FileStoreInfo.newBuilder();
s3fsBuilder.setBucket(bucket);
s3fsBuilder.setRegion(region);
s3fsBuilder.setEndpoint(endpoint);
s3fsBuilder.setAccessKey(accessKey);
s3fsBuilder.setAccessKeySecret(accessKeySecret);
S3FileStoreInfo s3FsInfo = s3fsBuilder.build();
FileStoreInfo.Builder builder = FileStoreInfo.newBuilder();
builder.setFsType(FileStoreType.S3);
builder.setFsKey(key());
builder.setS3FsInfo(s3FsInfo);
return builder.build();
}
public static FileStore fromProtobuf(FileStoreInfo fsInfo) {
S3FileStoreInfo s3FsInfo = fsInfo.getS3FsInfo();
String bucket = s3FsInfo.getBucket();
String region = s3FsInfo.getRegion();
String endpoint = s3FsInfo.getEndpoint();
String ak = s3FsInfo.getAccessKey();
String sk = s3FsInfo.getAccessKeySecret();
return new S3FileStore(bucket, region, endpoint, ak, sk);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy