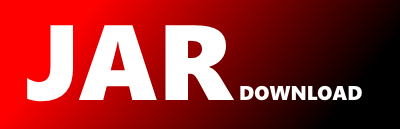
com.staros.manager.StarManagerServer Maven / Gradle / Ivy
// Copyright 2021-present StarRocks, Inc. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.staros.manager;
import com.staros.journal.DummyJournalSystem;
import com.staros.journal.JournalSystem;
import com.staros.util.Config;
import com.staros.util.LogConfig;
import io.grpc.Server;
import io.grpc.ServerBuilder;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.io.IOException;
import java.util.concurrent.atomic.AtomicBoolean;
public class StarManagerServer {
private static final Logger LOG = LogManager.getLogger(StarManagerServer.class);
private StarManager manager;
private StarManagerService service;
private Server server;
private AtomicBoolean started; // FOR TEST
// FOR TEST
public StarManagerServer() {
this(new DummyJournalSystem());
}
public StarManagerServer(JournalSystem journalSystem) {
this.manager = new StarManager(journalSystem);
this.service = new StarManagerService(manager);
this.started = new AtomicBoolean(false);
}
public void start(int port) throws IOException {
LOG.info("starting star manager on port " + port + " ...");
server = ServerBuilder.forPort(port).addService(service).build().start();
started.set(true);
}
public void shutdown() {
LOG.info("star manager shutting down...");
server.shutdown();
getStarManager().stopBackgroundThreads();
}
public void blockUntilShutdown() throws InterruptedException {
if (server != null) {
server.awaitTermination();
}
}
// FOR TEST ONLY
public int getServerPort() {
return server.getPort();
}
// FOR TEST
public void blockUntilStart() {
int times = 1;
while (!started.get()) {
if (times++ >= 100) {
System.out.println("wait star manager server to start for 5 seconds, abort test!");
System.exit(-1);
}
try {
Thread.sleep(50); // 50ms
} catch (InterruptedException e) {
// do nothing
}
}
}
public StarManager getStarManager() {
return manager;
}
public static void main(String[] args) throws IOException, InterruptedException {
String confFile = "./starmgr.conf";
// process config file
new Config().init(confFile);
// start log4j based on config
LogConfig.initLogging();
// start server
StarManagerServer server = new StarManagerServer();
server.getStarManager().becomeLeader();
server.start(Config.STARMGR_RPC_PORT);
server.blockUntilShutdown();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy