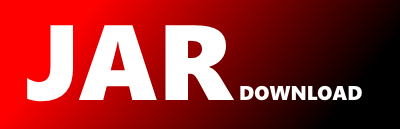
com.staros.worker.WorkerGroup Maven / Gradle / Ivy
// Copyright 2021-present StarRocks, Inc. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.staros.worker;
import com.staros.util.LogUtils;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
/**
* Worker Group manages a certain number of worker in one service.
*/
public class WorkerGroup {
private static final Logger LOG = LogManager.getLogger(WorkerGroup.class);
private final String serviceId;
private final long groupId;
private List workers;
public WorkerGroup(String serviceId, long groupId) {
// TODO: load from storage
this.serviceId = serviceId;
this.groupId = groupId;
this.workers = new ArrayList();
}
public String getServiceId() {
return serviceId;
}
public long getGroupId() {
return groupId;
}
public void addWorker(Worker worker) {
checkWorker(worker);
for (Worker w : workers) {
if (w.equals(worker)) {
LogUtils.fatal(LOG, "worker {}({}) already exist in worker group.", worker.getWorkerId(), worker.getIpPort());
}
}
workers.add(worker);
}
public void removeWorker(Worker worker) {
checkWorker(worker);
for (Worker w : workers) {
if (w.equals(worker)) {
workers.remove(worker);
return;
}
}
LogUtils.fatal(LOG, "worker {}({}) not exist in worker group when remove.", worker.getWorkerId(), worker.getIpPort());
}
public void updateWorker(Worker worker) {
checkWorker(worker);
for (int i = 0; i < workers.size(); ++i) {
if (workers.get(i).equals(worker)) {
workers.set(i, worker);
return;
}
}
LogUtils.fatal(LOG, "worker {}({}) not exist in worker group when update.", worker.getWorkerId(), worker.getIpPort());
}
public List getWorkerList() {
return workers;
}
public List getAllWorkerIds(boolean onlyAlive) {
return workers.stream()
.filter(x -> !onlyAlive || x.isAlive())
.map(Worker::getWorkerId)
.collect(Collectors.toList());
}
public int getWorkerCount() {
return workers.size();
}
private void checkWorker(Worker worker) {
if (!worker.getServiceId().equals(serviceId)) {
LogUtils.fatal(LOG, "worker's service id {} not match service id {} in worker group.",
worker.getServiceId(), serviceId);
}
if (worker.getGroupId() != groupId) {
LogUtils.fatal(LOG, "worker's group id {} not match group id {} in worker group.", worker.getGroupId(), groupId);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy