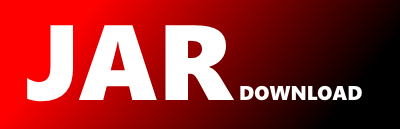
com.staros.filestore.FileStore Maven / Gradle / Ivy
// Copyright 2021-present StarRocks, Inc. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.staros.filestore;
import com.staros.proto.FileStoreInfo;
import com.staros.proto.FileStoreType;
public abstract class FileStore {
protected String key;
protected String name;
protected boolean enabled = true;
protected boolean isDefault = false;
protected String comment = "";
public FileStore(String key, String name) {
this.key = key;
this.name = name;
}
public FileStore(FileStoreInfo fsInfo) {
this.key = fsInfo.getFsKey();
this.name = fsInfo.getFsName();
this.enabled = fsInfo.getEnabled();
this.isDefault = fsInfo.getIsDefault();
this.comment = fsInfo.getComment();
}
// Return file store key
// Each file store has one unique key
public String key() {
return key;
}
// Return file store name
// Name is not ensured to be unique
public String name() {
return name;
}
public String getComment() {
return comment;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public boolean getEnabled() {
return enabled;
}
public void setIsDefault(boolean isDefault) {
this.isDefault = isDefault;
}
public boolean isDefault() {
return isDefault;
}
// Return file store type, 'S3','HDFS' etc
public abstract FileStoreType type();
// Return file store root path
public abstract String rootPath();
public abstract boolean isValid();
// Serialize file store to protobuf format
public FileStoreInfo toProtobuf() {
FileStoreInfo.Builder builder = FileStoreInfo.newBuilder();
return builder.setFsKey(key)
.setFsName(name)
.setEnabled(enabled)
.setIsDefault(isDefault)
.setComment(comment).build();
}
public void mergeFrom(FileStore other) {
if (!other.getComment().isEmpty()) {
this.comment = other.comment;
}
this.enabled = other.enabled;
this.isDefault = other.isDefault;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy