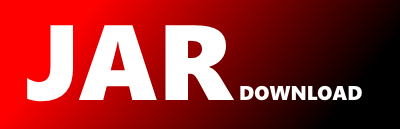
com.staros.heartbeat.HeartbeatManager Maven / Gradle / Ivy
// Copyright 2021-present StarRocks, Inc. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// https://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.staros.heartbeat;
import com.staros.util.AbstractServer;
import com.staros.util.Config;
import com.staros.util.Utils;
import com.staros.worker.Worker;
import com.staros.worker.WorkerManager;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.List;
import java.util.concurrent.ScheduledThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
public class HeartbeatManager extends AbstractServer {
private static final Logger LOG = LogManager.getLogger(HeartbeatManager.class);
private final int heartbeatInterval;
private final WorkerManager workerManager;
private ScheduledThreadPoolExecutor executors;
public HeartbeatManager(WorkerManager workerManager) {
int interval = Config.WORKER_HEARTBEAT_INTERVAL_SEC;
if (interval < 1 || interval > 60) {
LOG.warn("worker heartbeat interval {} is not suitable, change it to default 10.", interval);
interval = 10;
}
this.heartbeatInterval = interval;
this.workerManager = workerManager;
}
@Override
public void doStart() {
this.executors = new ScheduledThreadPoolExecutor(1);
this.executors.execute(this::runOnceHeartbeatCheck);
}
@Override
public void doStop() {
// force stop all active tasks
executors.shutdownNow();
Utils.shutdownExecutorService(executors);
}
private void runOnceHeartbeatCheck() {
if (!isRunning()) {
return;
}
try {
LOG.debug("running heartbeat once.");
List allWorkerIds = workerManager.getAllWorkerIds();
for (long id : allWorkerIds) {
Worker worker = workerManager.getWorker(id);
if (worker == null) {
continue;
}
// TODO: send epoch, which should be obtained from star manager ha module
this.executors.execute(worker::heartbeat);
}
} catch (Exception exception) {
LOG.warn("Fail to submit tasks to executor. Error:", exception);
}
try { // schedule next running
this.executors.schedule(this::runOnceHeartbeatCheck, heartbeatInterval, TimeUnit.SECONDS);
} catch (Exception exception) {
if (isRunning()) {
LOG.fatal("Fail to schedule next round of heartbeat check, error:", exception);
} else {
LOG.info("HeartbeatManager is in shutdown.");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy