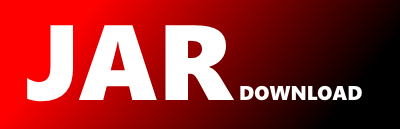
com.sleepycat.je.evictor.EvictorStatDefinition Maven / Gradle / Ivy
The newest version!
/*-
* Copyright (C) 2002, 2018, Oracle and/or its affiliates. All rights reserved.
*
* This file was distributed by Oracle as part of a version of Oracle Berkeley
* DB Java Edition made available at:
*
* http://www.oracle.com/technetwork/database/database-technologies/berkeleydb/downloads/index.html
*
* Please see the LICENSE file included in the top-level directory of the
* appropriate version of Oracle Berkeley DB Java Edition for a copy of the
* license and additional information.
*/
package com.sleepycat.je.evictor;
import com.sleepycat.je.utilint.StatDefinition;
import com.sleepycat.je.utilint.StatDefinition.StatType;
/**
* Per-stat Metadata for JE evictor statistics.
*/
public class EvictorStatDefinition {
public static final String GROUP_NAME = "Cache";
public static final String GROUP_DESC =
"The main cache resides in the Java heap and holds" +
" data, keys, Btree internal nodes, locks and JE metadata.";
/**
* The StatDefinitions for N_BYTES_EVICTED_XXX stats are generated by
* {@link Evictor.EvictionSource}.
*/
public static final String N_BYTES_EVICTED_EVICTORTHREAD_NAME =
"nBytesEvictedEVICTORTHREAD";
public static final String N_BYTES_EVICTED_EVICTORTHREAD_DESC =
"Number of bytes evicted by evictor pool threads.";
public static final String N_BYTES_EVICTED_MANUAL_NAME =
"nBytesEvictedMANUAL";
public static final String N_BYTES_EVICTED_MANUAL_DESC =
"Number of bytes evicted by the Environment.evictMemory or during" +
" Environment startup.";
public static final String N_BYTES_EVICTED_CRITICAL_NAME =
"nBytesEvictedCRITICAL";
public static final String N_BYTES_EVICTED_CRITICAL_DESC =
"Number of bytes evicted in the application thread because the cache" +
" is over budget.";
public static final String N_BYTES_EVICTED_CACHEMODE_NAME =
"nBytesEvictedCACHEMODE";
public static final String N_BYTES_EVICTED_CACHEMODE_DESC =
"Number of bytes evicted by operations for which " +
"CacheMode.EVICT_BIN is specified.";
public static final String N_BYTES_EVICTED_DAEMON_NAME =
"nBytesEvictedDAEMON";
public static final String N_BYTES_EVICTED_DAEMON_DESC =
"Number of bytes evicted by JE deamon threads.";
public static final String EVICTOR_EVICTION_RUNS_NAME =
"nEvictionRuns";
public static final String EVICTOR_EVICTION_RUNS_DESC =
"Number of times the background eviction thread is awoken.";
public static final StatDefinition EVICTOR_EVICTION_RUNS =
new StatDefinition(
EVICTOR_EVICTION_RUNS_NAME,
EVICTOR_EVICTION_RUNS_DESC);
public static final String EVICTOR_NODES_TARGETED_NAME =
"nNodesTargeted";
public static final String EVICTOR_NODES_TARGETED_DESC =
"Number of nodes (INs) selected as eviction targets.";
public static final StatDefinition EVICTOR_NODES_TARGETED =
new StatDefinition(
EVICTOR_NODES_TARGETED_NAME,
EVICTOR_NODES_TARGETED_DESC);
public static final String EVICTOR_NODES_EVICTED_NAME =
"nNodesEvicted";
public static final String EVICTOR_NODES_EVICTED_DESC =
"Number of target nodes (INs) evicted from the main cache.";
public static final StatDefinition EVICTOR_NODES_EVICTED =
new StatDefinition(
EVICTOR_NODES_EVICTED_NAME,
EVICTOR_NODES_EVICTED_DESC);
public static final String EVICTOR_ROOT_NODES_EVICTED_NAME =
"nRootNodesEvicted";
public static final String EVICTOR_ROOT_NODES_EVICTED_DESC =
"Number of database root nodes (INs) evicted.";
public static final StatDefinition EVICTOR_ROOT_NODES_EVICTED =
new StatDefinition(
EVICTOR_ROOT_NODES_EVICTED_NAME,
EVICTOR_ROOT_NODES_EVICTED_DESC);
public static final String EVICTOR_DIRTY_NODES_EVICTED_NAME =
"nDirtyNodesEvicted";
public static final String EVICTOR_DIRTY_NODES_EVICTED_DESC =
"Number of dirty target nodes logged and evicted.";
public static final StatDefinition EVICTOR_DIRTY_NODES_EVICTED =
new StatDefinition(
EVICTOR_DIRTY_NODES_EVICTED_NAME,
EVICTOR_DIRTY_NODES_EVICTED_DESC);
public static final String EVICTOR_LNS_EVICTED_NAME =
"nLNsEvicted";
public static final String EVICTOR_LNS_EVICTED_DESC =
"Number of LNs evicted as a result of LRU-based eviction (but not " +
"CacheMode.EVICT_LN).";
public static final StatDefinition EVICTOR_LNS_EVICTED =
new StatDefinition(
EVICTOR_LNS_EVICTED_NAME,
EVICTOR_LNS_EVICTED_DESC);
public static final String EVICTOR_NODES_STRIPPED_NAME =
"nNodesStripped";
public static final String EVICTOR_NODES_STRIPPED_DESC =
"Number of target BINs for which space was reclaimed by deleting " +
"space for expired LNs or evicting resident LNs.";
public static final StatDefinition EVICTOR_NODES_STRIPPED =
new StatDefinition(
EVICTOR_NODES_STRIPPED_NAME,
EVICTOR_NODES_STRIPPED_DESC);
public static final String EVICTOR_NODES_MUTATED_NAME =
"nNodesMutated";
public static final String EVICTOR_NODES_MUTATED_DESC =
"Number of target BINs mutated to BIN-deltas.";
public static final StatDefinition EVICTOR_NODES_MUTATED =
new StatDefinition(
EVICTOR_NODES_MUTATED_NAME,
EVICTOR_NODES_MUTATED_DESC);
public static final String EVICTOR_NODES_PUT_BACK_NAME =
"nNodesPutBack";
public static final String EVICTOR_NODES_PUT_BACK_DESC =
"Number of target nodes (INs) moved to the cold end of the LRU " +
"list without any action taken on them.";
public static final StatDefinition EVICTOR_NODES_PUT_BACK =
new StatDefinition(
EVICTOR_NODES_PUT_BACK_NAME,
EVICTOR_NODES_PUT_BACK_DESC);
public static final String EVICTOR_NODES_MOVED_TO_PRI2_LRU_NAME =
"nNodesMovedToDirtyLRU";
public static final String EVICTOR_NODES_MOVED_TO_PRI2_LRU_DESC =
"Number of nodes (INs) moved from the mixed/priority-1 to the " +
"dirty/priority-2 LRU list.";
public static final StatDefinition EVICTOR_NODES_MOVED_TO_PRI2_LRU =
new StatDefinition(
EVICTOR_NODES_MOVED_TO_PRI2_LRU_NAME,
EVICTOR_NODES_MOVED_TO_PRI2_LRU_DESC);
public static final String EVICTOR_NODES_SKIPPED_NAME =
"nNodesSkipped";
public static final String EVICTOR_NODES_SKIPPED_DESC =
"Number of nodes (INs) that did not require any action.";
public static final StatDefinition EVICTOR_NODES_SKIPPED =
new StatDefinition(
EVICTOR_NODES_SKIPPED_NAME,
EVICTOR_NODES_SKIPPED_DESC);
public static final String EVICTOR_SHARED_CACHE_ENVS_NAME =
"nSharedCacheEnvironments";
public static final String EVICTOR_SHARED_CACHE_ENVS_DESC =
"Number of Environments sharing the main cache.";
public static final StatDefinition EVICTOR_SHARED_CACHE_ENVS =
new StatDefinition(
EVICTOR_SHARED_CACHE_ENVS_NAME,
EVICTOR_SHARED_CACHE_ENVS_DESC,
StatType.CUMULATIVE);
public static final String LN_FETCH_NAME =
"nLNsFetch";
public static final String LN_FETCH_DESC =
"Number of LNs (data records) requested by btree operations.";
public static final StatDefinition LN_FETCH =
new StatDefinition(
LN_FETCH_NAME,
LN_FETCH_DESC);
/*
* Number of times IN.fetchIN() or IN.fetchINWithNoLatch() was called
* to fetch a UIN.
*/
public static final String UPPER_IN_FETCH_NAME =
"nUpperINsFetch";
public static final String UPPER_IN_FETCH_DESC =
"Number of Upper INs (non-bottom internal nodes) requested by btree " +
"operations.";
public static final StatDefinition UPPER_IN_FETCH =
new StatDefinition(
UPPER_IN_FETCH_NAME,
UPPER_IN_FETCH_DESC);
/*
* Number of times IN.fetchIN() or IN.fetchINWithNoLatch() was called
* to fetch a BIN.
*/
public static final String BIN_FETCH_NAME =
"nBINsFetch";
public static final String BIN_FETCH_DESC =
"Number of BINs (bottom internal nodes) and BIN-deltas requested by " +
"btree operations.";
public static final StatDefinition BIN_FETCH =
new StatDefinition(
BIN_FETCH_NAME,
BIN_FETCH_DESC);
public static final String LN_FETCH_MISS_NAME =
"nLNsFetchMiss";
public static final String LN_FETCH_MISS_DESC =
"Number of LNs (data records) requested by btree operations that " +
"were not in main cache.";
public static final StatDefinition LN_FETCH_MISS =
new StatDefinition(
LN_FETCH_MISS_NAME,
LN_FETCH_MISS_DESC);
/*
* Number of times IN.fetchIN() or IN.fetchINWithNoLatch() was called
* to fetch a UIN and that UIN was not already cached.
*/
public static final String UPPER_IN_FETCH_MISS_NAME =
"nUpperINsFetchMiss";
public static final String UPPER_IN_FETCH_MISS_DESC =
"Number of Upper INs (non-bottom internal nodes) requested by btree " +
"operations that were not in main cache.";
public static final StatDefinition UPPER_IN_FETCH_MISS =
new StatDefinition(
UPPER_IN_FETCH_MISS_NAME,
UPPER_IN_FETCH_MISS_DESC);
/*
* Number of times IN.fetchIN() or IN.fetchINWithNoLatch() was called
* to fetch a BIN and that BIN was not already cached.
*/
public static final String BIN_FETCH_MISS_NAME =
"nBINsFetchMiss";
public static final String BIN_FETCH_MISS_DESC =
"Number of full BINs (bottom internal nodes) and BIN-deltas fetched " +
"to satisfy btree operations that were not in main cache.";
public static final StatDefinition BIN_FETCH_MISS =
new StatDefinition(
BIN_FETCH_MISS_NAME,
BIN_FETCH_MISS_DESC);
/*
* BIN_FETCH_MISS / BIN_FETCH
*/
public static final String BIN_FETCH_MISS_RATIO_NAME =
"nBINsFetchMissRatio";
public static final String BIN_FETCH_MISS_RATIO_DESC =
"The BIN fetch miss ratio (nBINsFetchMiss / nBINsFetch)";
public static final StatDefinition BIN_FETCH_MISS_RATIO =
new StatDefinition(
BIN_FETCH_MISS_RATIO_NAME,
BIN_FETCH_MISS_RATIO_DESC,
StatType.CUMULATIVE);
/*
* Number of times IN.fetchIN() or IN.fetchINWithNoLatch() was called
* to fetch a BIN, that BIN was not already cached, and a BIN-delta was
* fetched from disk.
*/
public static final String BIN_DELTA_FETCH_MISS_NAME =
"nBINDeltasFetchMiss";
public static final String BIN_DELTA_FETCH_MISS_DESC =
"Number of BIN-deltas (partial BINs) fetched to satisfy btree " +
"operations that were not in main cache.";
public static final StatDefinition BIN_DELTA_FETCH_MISS =
new StatDefinition(
BIN_DELTA_FETCH_MISS_NAME,
BIN_DELTA_FETCH_MISS_DESC);
/*
* The number of operations performed blindly in BIN deltas
*/
public static final String BIN_DELTA_BLIND_OPS_NAME =
"nBinDeltaBlindOps";
public static final String BIN_DELTA_BLIND_OPS_DESC =
"The number of operations performed blindly in BIN deltas";
public static final StatDefinition BIN_DELTA_BLIND_OPS =
new StatDefinition(
BIN_DELTA_BLIND_OPS_NAME,
BIN_DELTA_BLIND_OPS_DESC);
/*
* Number of calls to BIN.mutateToFullBIN()
*/
public static final String FULL_BIN_MISS_NAME =
"nFullBINsMiss";
public static final String FULL_BIN_MISS_DESC =
"Number of times a BIN-delta had to be mutated to a full BIN (and as" +
" a result a full BIN had to be read in from the log).";
public static final StatDefinition FULL_BIN_MISS =
new StatDefinition(
FULL_BIN_MISS_NAME,
FULL_BIN_MISS_DESC);
/*
* The number of UINs in the memory-resident tree at the time the
* stats were collected. This is an INSTANT stat.
*/
public static final String CACHED_UPPER_INS_NAME =
"nCachedUpperINs";
public static final String CACHED_UPPER_INS_DESC =
"Number of upper INs (non-bottom internal nodes) in main cache.";
public static final StatDefinition CACHED_UPPER_INS =
new StatDefinition(
CACHED_UPPER_INS_NAME,
CACHED_UPPER_INS_DESC,
StatType.CUMULATIVE);
/*
* The number of BINs (full or deltas) in the memory-resident tree at the
* time the stats were collected. This is an INSTANT stat.
*/
public static final String CACHED_BINS_NAME =
"nCachedBINs";
public static final String CACHED_BINS_DESC =
"Number of BINs (bottom internal nodes) and BIN-deltas in main cache.";
public static final StatDefinition CACHED_BINS =
new StatDefinition(
CACHED_BINS_NAME,
CACHED_BINS_DESC,
StatType.CUMULATIVE);
/*
* The number of delta-BINs in the memory-resident tree at the time the
* stats were collected. This is an INSTANT stat.
*/
public static final String CACHED_BIN_DELTAS_NAME =
"nCachedBINDeltas";
public static final String CACHED_BIN_DELTAS_DESC =
"Number of BIN-deltas (partial BINs) in main cache. This is a subset" +
" of the nCachedBINs value.";
public static final StatDefinition CACHED_BIN_DELTAS =
new StatDefinition(
CACHED_BIN_DELTAS_NAME,
CACHED_BIN_DELTAS_DESC,
StatType.CUMULATIVE);
/*
* Number of eviction tasks that were submitted to the background evictor
* pool, but were refused because all eviction threads were busy.
*/
public static final String THREAD_UNAVAILABLE_NAME =
"nThreadUnavailable";
public static final String THREAD_UNAVAILABLE_DESC =
"Number of eviction tasks that were submitted to the background " +
"evictor pool, but were refused because all eviction threads " +
"were busy.";
public static final StatDefinition THREAD_UNAVAILABLE =
new StatDefinition(
THREAD_UNAVAILABLE_NAME,
THREAD_UNAVAILABLE_DESC);
public static final String CACHED_IN_SPARSE_TARGET_NAME =
"nINSparseTarget";
public static final String CACHED_IN_SPARSE_TARGET_DESC =
"Number of INs that use a compact sparse array representation to " +
"point to child nodes in the main cache.";
public static final StatDefinition CACHED_IN_SPARSE_TARGET =
new StatDefinition(
CACHED_IN_SPARSE_TARGET_NAME,
CACHED_IN_SPARSE_TARGET_DESC,
StatType.CUMULATIVE);
public static final String CACHED_IN_NO_TARGET_NAME =
"nINNoTarget";
public static final String CACHED_IN_NO_TARGET_DESC =
"Number of INs that use a compact representation when none of its " +
"child nodes are in the main cache.";
public static final StatDefinition CACHED_IN_NO_TARGET =
new StatDefinition(
CACHED_IN_NO_TARGET_NAME,
CACHED_IN_NO_TARGET_DESC,
StatType.CUMULATIVE);
public static final String CACHED_IN_COMPACT_KEY_NAME =
"nINCompactKey";
public static final String CACHED_IN_COMPACT_KEY_DESC =
"Number of INs that use a compact key representation to minimize the" +
" key object representation overhead.";
public static final StatDefinition CACHED_IN_COMPACT_KEY =
new StatDefinition(
CACHED_IN_COMPACT_KEY_NAME,
CACHED_IN_COMPACT_KEY_DESC,
StatType.CUMULATIVE);
public static final String PRI1_LRU_SIZE_NAME =
"lruMixedSize";
public static final String PRI1_LRU_SIZE_DESC =
"Number of INs in the mixed/priority-1 LRU ";
public static final StatDefinition PRI1_LRU_SIZE =
new StatDefinition(
PRI1_LRU_SIZE_NAME,
PRI1_LRU_SIZE_DESC,
StatType.CUMULATIVE);
public static final String PRI2_LRU_SIZE_NAME =
"lruDirtySize";
public static final String PRI2_LRU_SIZE_DESC =
"Number of INs in the dirty/priority-2 LRU ";
public static final StatDefinition PRI2_LRU_SIZE =
new StatDefinition(
PRI2_LRU_SIZE_NAME,
PRI2_LRU_SIZE_DESC,
StatType.CUMULATIVE);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy